A "for loop" in C++ is a control flow statement that allows you to execute a block of code repeatedly for a specified number of iterations.
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
What is a For Loop?
A for loop is a fundamental construct in programming that allows developers to execute a block of code multiple times, based on a counter or condition. It is widely used for iterations where the number of times to execute the loop is known beforehand.
Why Use a For Loop? For loops are particularly favored for their concise syntax and straightforward logic. They provide fine control over the initiation, condition, and progression of the loop, making them ideal for tasks like iterating through arrays, lists, or performing repetitive calculations.
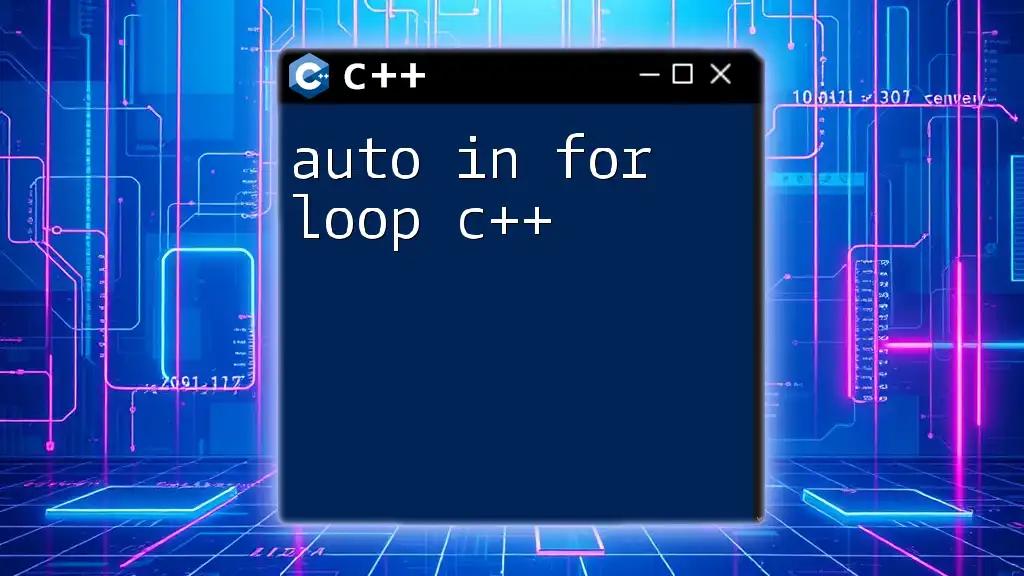
Structure of a For Loop
Syntax of a For Loop
The typical structure of a for loop in C++ can be encapsulated in the following syntax:
for (initialization; condition; increment) {
// code to execute
}
This structure comprises three main components:
- Initialization: This sets a loop control variable and runs once at the beginning.
- Condition: This boolean expression determines if the loop should continue executing.
- Increment/Decrement: This modifies the loop control variable, allowing or stopping further iterations based on the condition.
Components in Detail
Initialization In a for loop, you usually define and initialize a loop counter. For example:
for (int i = 0; i < 10; i++) {
// code
}
Here, `int i = 0` initializes the loop control variable `i` to zero.
Condition The loop continues to execute as long as this condition evaluates to `true`. For instance:
for (int i = 0; i < 10; i++) {
// loop executes until i is less than 10
}
When `i` reaches 10, the condition fails, and the loop terminates.
Increment/Decrement The increment (or decrement) statement is executed at the end of each iteration. For example:
for (int i = 0; i < 10; i++) {
// the loop increments i by 1 each time
}
This means that in each iteration, `i` is increased by one until the condition is no longer met.
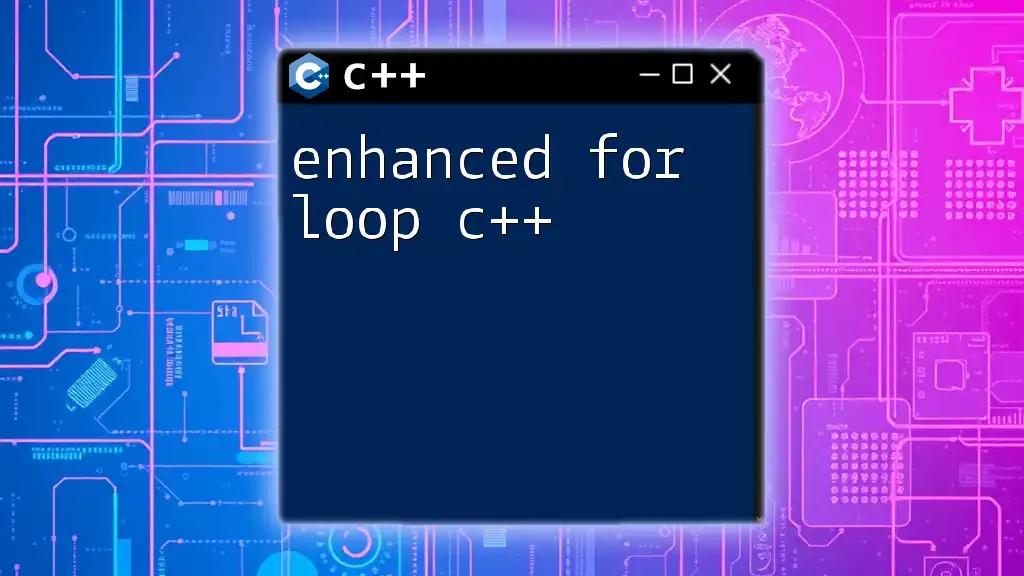
Types of For Loops
Standard For Loop
The standard for loop is the most commonly used format. It achieves most tasks efficiently. Here's an example showing how it can output numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl; // Outputs: 0 1 2 3 4
}
For Loop with Multiple Variables
In C++, you can even iterate through multiple variables within your loop. This can be useful for coordinated conditions or counters. Here’s an example:
for (int i = 0, j = 5; i < j; i++, j--) {
std::cout << i << " " << j << std::endl;
}
In this scenario, `i` increases while `j` decreases, demonstrating combined control over two variables.
For Each Loop (Range-based For Loop)
Introduced in C++11, the range-based for loop simplifies iterations over collections like arrays or vectors. It abstracts away the index manipulation. Consider this example with a vector:
std::vector<int> vec = {1, 2, 3, 4, 5};
for (auto num : vec) {
std::cout << num << " "; // Outputs: 1 2 3 4 5
}
Here, `auto num` automatically deduces the type, streamlining the syntax.
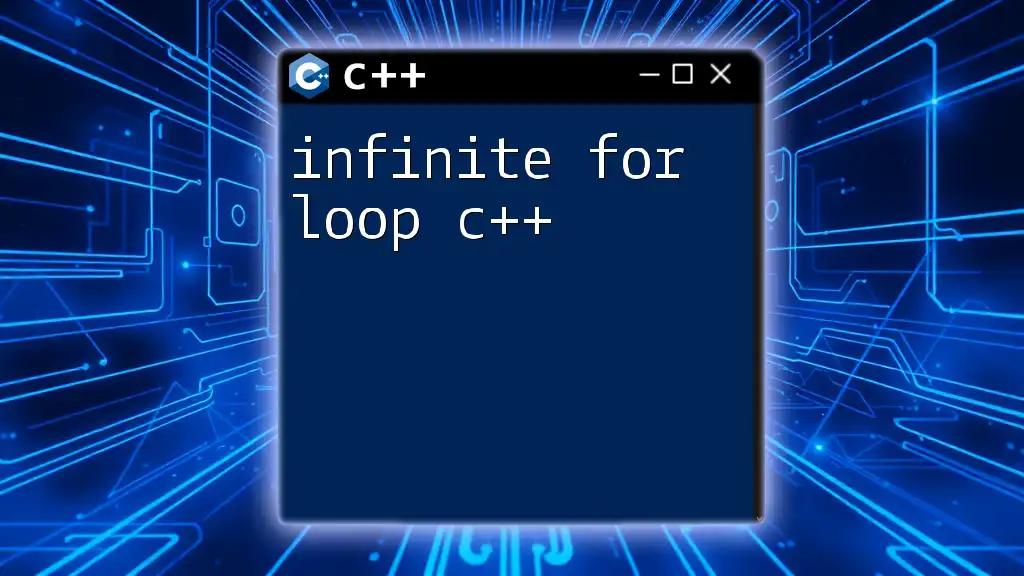
Common Use Cases
Iterating Over Arrays
For loops are exceptional for traversing arrays since the number of elements is often defined. Here’s an example:
int arr[] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " "; // Outputs: 1 2 3 4 5
}
This example outputs each element in the `arr` array by iterating through its indices.
Nested For Loops
When you need to perform operations on multi-dimensional data or combinations, nested for loops come in handy. Below is a demonstration of creating a multiplication table:
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++) {
std::cout << i * j << "\t";
}
std::cout << std::endl;
}
This code snippet will create a nicely formatted output displaying products of integers 1 through 10.
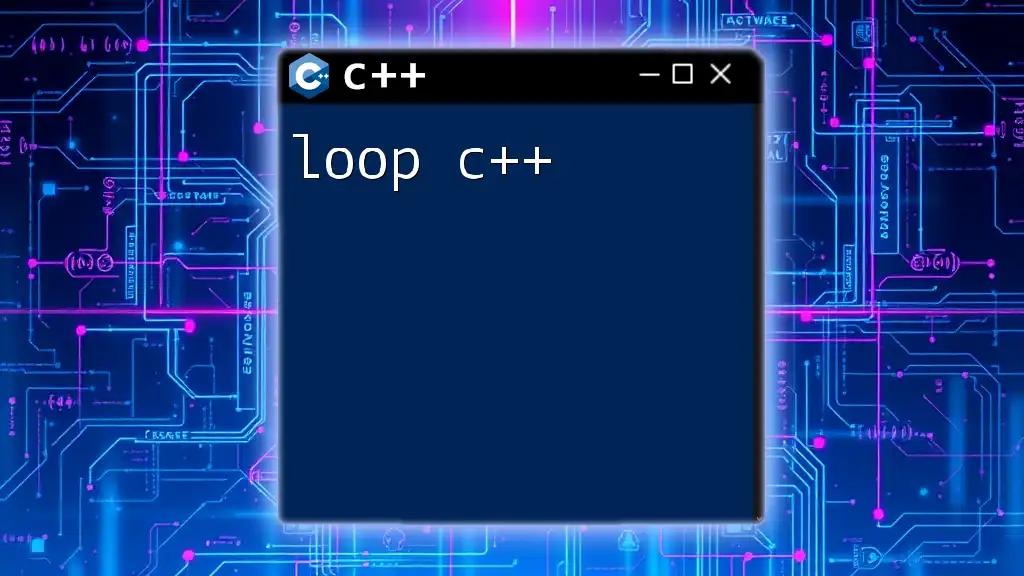
Best Practices
Keep Loop Simple
To ensure that your code remains legible, it’s essential to keep your loops simple. Avoid placing complex logic inside the loop. Instead, consider using functions or breaking the task into manageable parts.
Control Variables and Scope
Control variables should be precisely scoped to the loop itself, minimizing unintended side effects. Consider the following example:
for (int i = 0; i < 5; i++) {
int x = i; // x is scoped only within this loop
}
// std::cout << x; // Error: x cannot be accessed here
This prohibits the variable `x` from causing confusion or issues outside its intended scope.
Performance Considerations
Understanding algorithm efficiency can significantly impact your program's performance. Always consider how the bounds of your loop and the increments might affect execution speed.
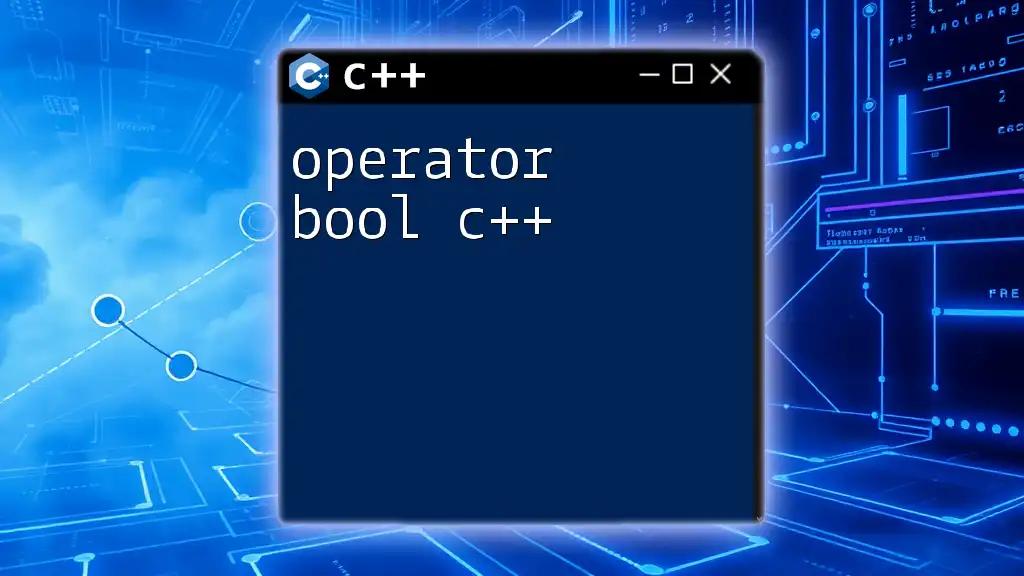
Debugging Common Issues
Infinite Loops
A common pitfall, infinite loops occur when the condition is never met. Typically, this happens due to missing incrementing logic or incorrect condition checks. Here’s an example that demonstrates this error:
// This code will run indefinitely
for (int i = 0; i < 10; /* missing increment here */) {
std::cout << i << std::endl; // Will not terminate
}
To avoid infinite loops, always verify that your condition will be eventually false.
Off-by-One Errors
Off-by-one errors are a frequent programming miscalculation. They occur when you miscalculate the range or bounds of your loop. For instance:
for (int i = 0; i <= 10; i++) {
std::cout << i << std::endl; // Outputs: 0 to 10, but could be intended 0 to 9.
}
It's crucial to double-check your conditions and ranges to ensure they align with your intentions.
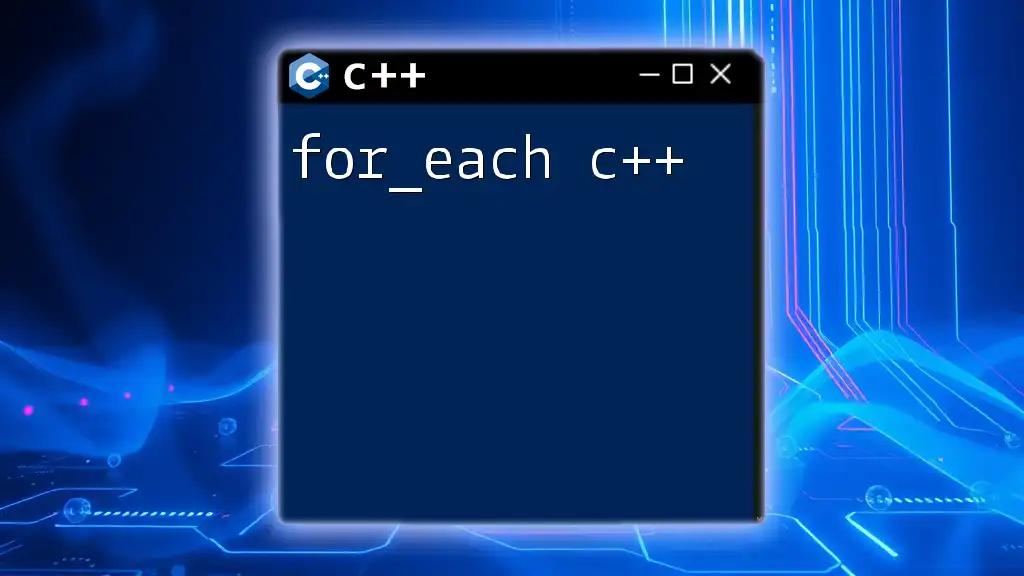
Conclusion
In summary, the for loop in C++ is a versatile and powerful tool for executing repetitive tasks. By mastering the structure, types, and best practices associated with for loops, you can enhance your programming skills significantly. Incorporate these insights into your programming practice, and you'll become proficient in efficiently using for loops in C++.
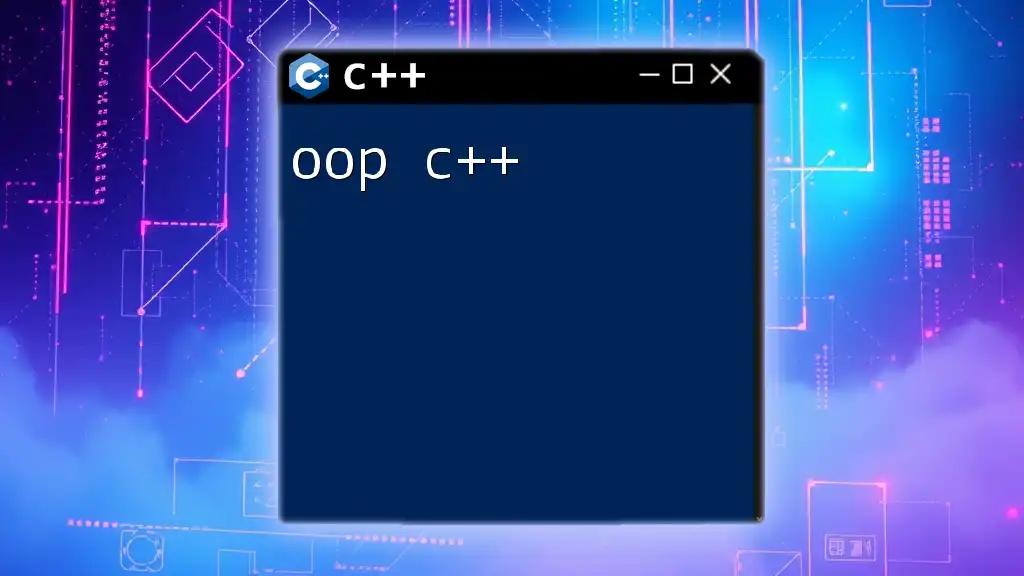
Further Learning Resources
As you continue your journey in mastering C++:
- Explore programming books focused on C++.
- Look for online courses that dive deeper into advanced loop types and logic.
- Participate in coding forums or communities to exchange knowledge and stimulate further learning.
Engaging with these resources will help solidify your understanding and skills with for loops, leading to more efficient and effective programming in C++.