The `for_each` algorithm in C++ applies a specified function to each element of a container, providing an efficient way to process elements without explicitly writing a loop.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int n) { std::cout << n << " "; });
return 0;
}
Understanding `for_each`
Definition and Purpose
`for_each` is a function template defined in the C++ Standard Library (STL) that applies a given function (or callable) to a range of elements specified by iterators. It greatly simplifies the process of iterating over elements, promoting cleaner and more readable code. The primary purpose of using `for_each` is to replace traditional loops with a more elegant syntax.
Syntax of `for_each`
The basic syntax of `for_each` is as follows:
std::for_each(begin_iterator, end_iterator, function);
Where:
- `begin_iterator`: This is the starting point of the range you want to iterate over.
- `end_iterator`: This marks the endpoint of the range. The element pointed to by end_iterator will not be included in the operation.
- `function`: This can be a function pointer, a lambda function, or any callable object that defines how to operate on each element in the specified range.
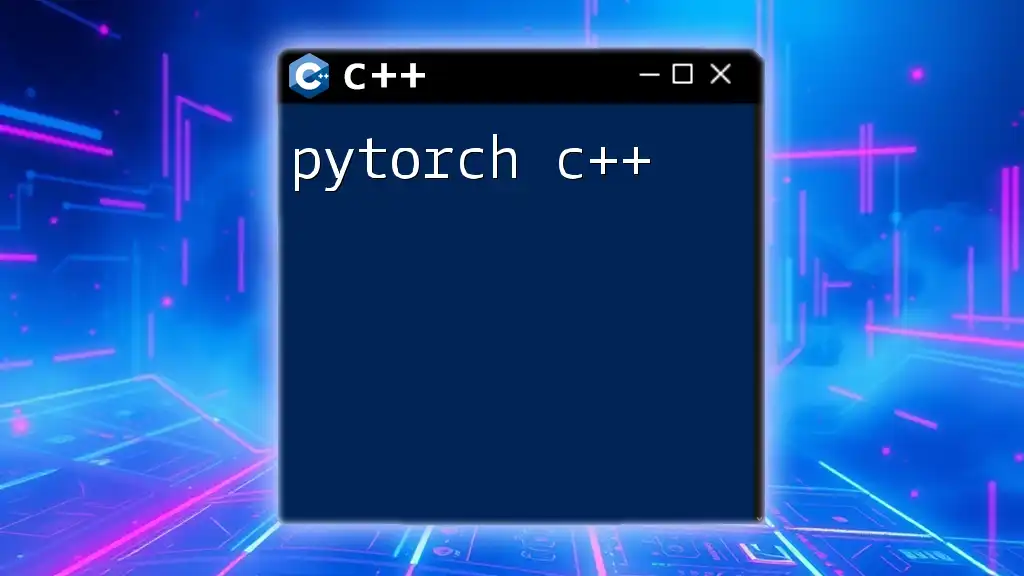
Practical Usage of `for_each`
Using Lambda Functions with `for_each`
In modern C++, lambda functions provide a flexible way to create inline, unnamed functions directly in your code. This feature allows a seamless and intuitive way to apply `for_each`.
Here’s how you can use a lambda function with `for_each`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::for_each(vec.begin(), vec.end(), [](int n) { std::cout << n << " "; });
return 0;
}
In this example, we declare a vector of integers and use `for_each` to print each element. The lambda `[](int n) { std::cout << n << " "; }` defines an operation that’s applied to every element in `vec`. The output will be: `1 2 3 4 5`.
Applying `for_each` on Containers
`for_each` can be effectively applied to various STL containers, such as lists, vectors, and arrays.
Here’s an example using `for_each` with a vector of strings:
std::vector<std::string> names = {"Alice", "Bob", "Charlie"};
std::for_each(names.begin(), names.end(), [](const std::string& name) {
std::cout << name << std::endl;
});
This will output:
Alice
Bob
Charlie
Modifying Elements with `for_each`
Beyond simply iterating, `for_each` can also be used to modify the elements in a container. When changing values, it is essential to pass elements by reference.
For instance, consider modifying a vector's values:
std::vector<int> numbers = {1, 2, 3};
std::for_each(numbers.begin(), numbers.end(), [](int& n) { n *= 2; });
In this scenario, each element in `numbers` is multiplied by two. If you were to print the vector afterward, it would display: `2 4 6`.
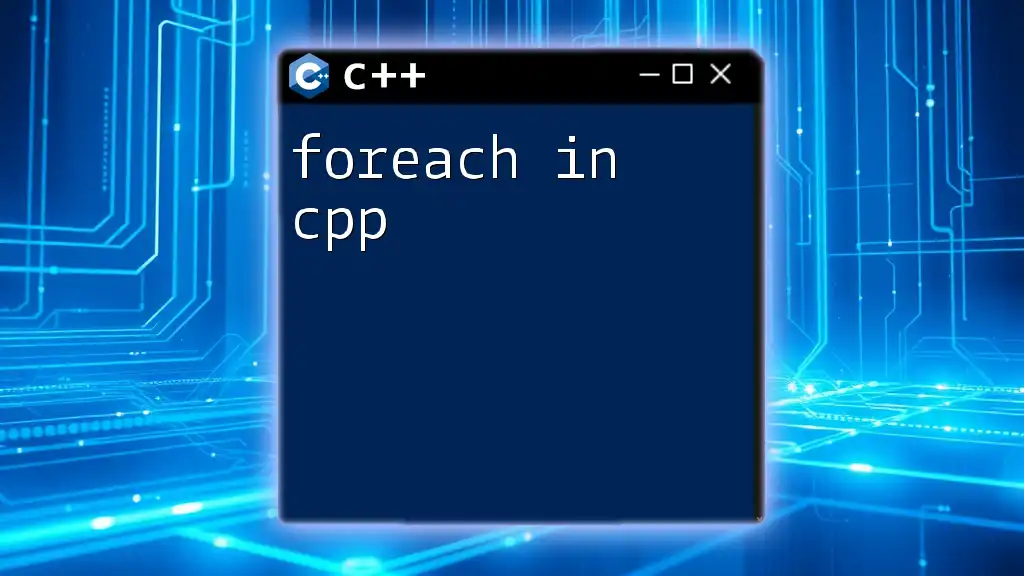
Advanced Techniques with `for_each`
Custom Function Objects
Function objects, or functors, are objects that can be called as if they were functions. They provide a way to encapsulate behavior.
Here’s an example of a custom functor:
struct Print {
void operator()(int n) const { std::cout << n << " "; }
};
std::for_each(vec.begin(), vec.end(), Print());
In this case, we define a struct `Print` and overload the call operator to print integers. It's a powerful approach for encapsulating state and behavior.
Combining `for_each` with Other Algorithms
The versatility of `for_each` shines even brighter when combined with other STL algorithms. For example, you could sort a vector and then print the results using `for_each`:
std::sort(vec.begin(), vec.end());
std::for_each(vec.begin(), vec.end(), [](int n) { std::cout << n << " "; });
Here, the vector is first sorted, and then the sorted elements are printed.
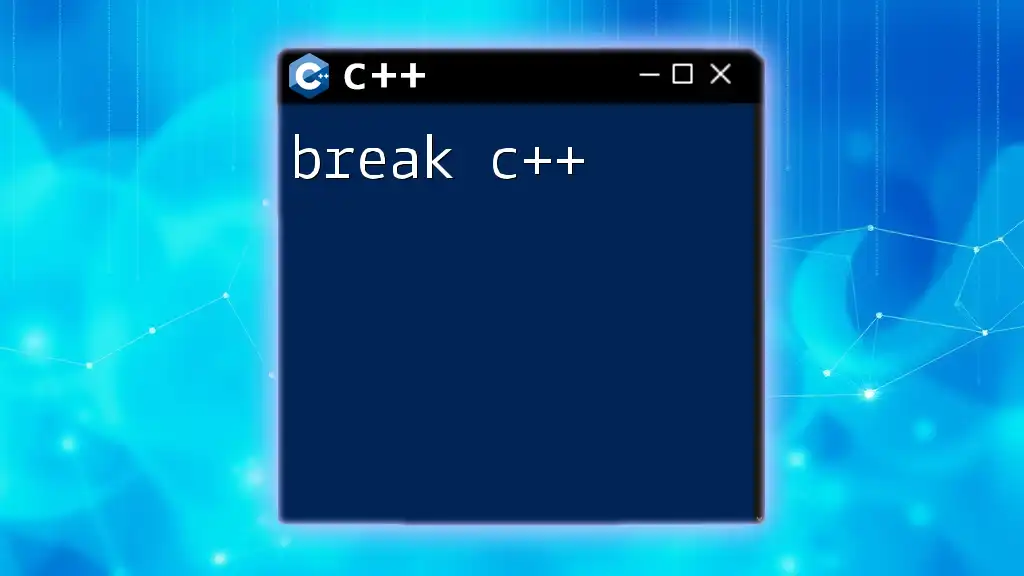
Common Mistakes and How to Avoid Them
Not Handling Iterators Properly
One common issue when working with iterators is iterator invalidation. Modifying a container while iterating through it with `for_each` can lead to undefined behavior. Always ensure that the container remains unchanged in size during the iteration.
Inefficient Function Calls
When defining the function to be called by `for_each`, consider efficiency. For instance, pass parameters by reference to avoid unnecessary copies. This is particularly important for large data structures.
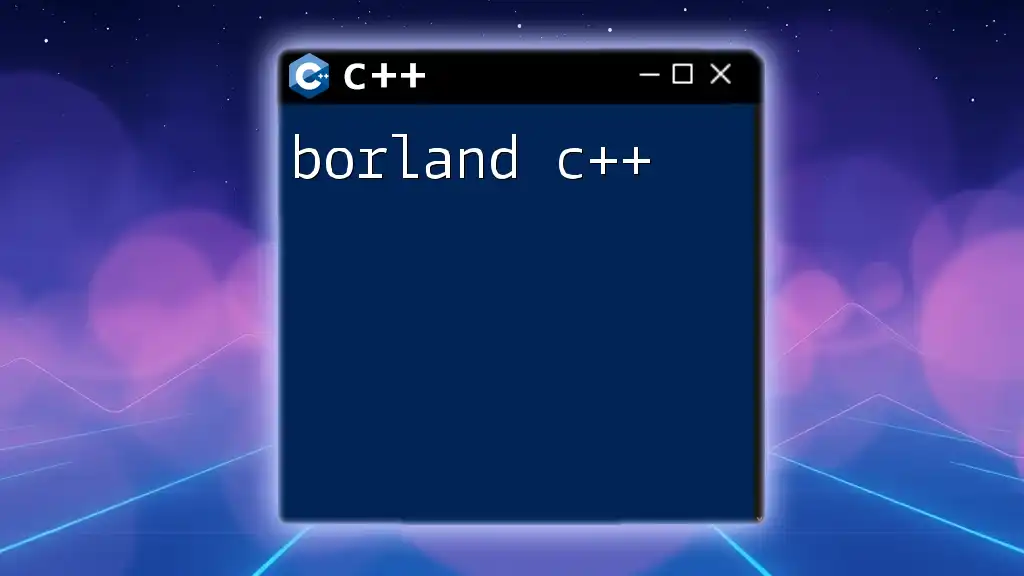
Testing and Debugging with `for_each`
Testing implementations of `for_each` can be straightforward. To ensure that it behaves as expected, consider writing unit tests for different scenarios, such as testing edge cases (like empty containers) or verifying modifications after applying the function.
Debugging `for_each` might involve checking both the function’s functionality and how it interacts with the iterators. If unexpected behaviors occur, consider isolating components and checking the values of the iterators before and after the function application.
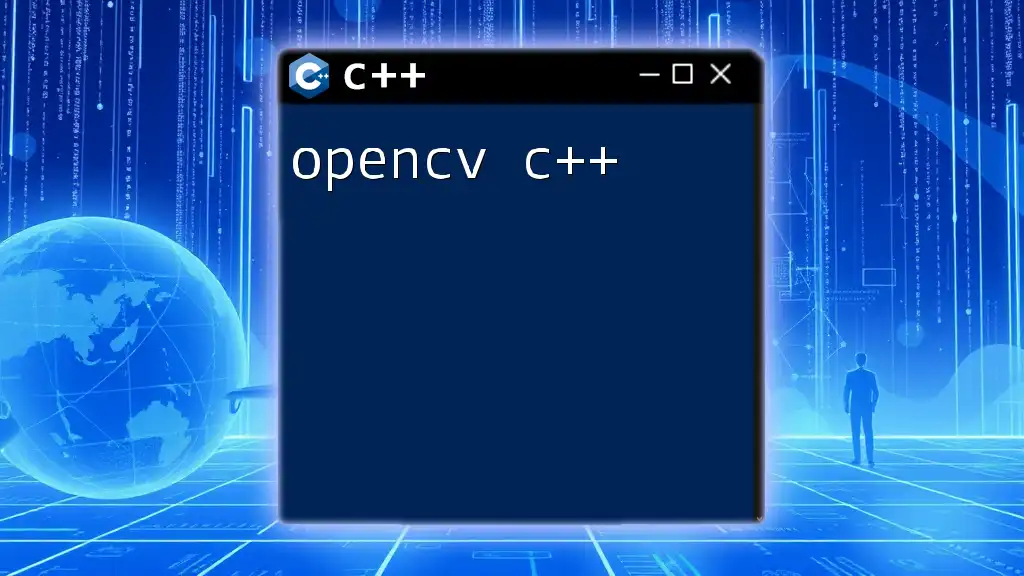
Conclusion
In conclusion, `for_each` offers a highly effective way to iterate over elements in C++, making code cleaner and more expressive. By employing `for_each`, developers can significantly improve the readability of their code while simultaneously increasing productivity. This function is a vital tool in any C++ programmer's arsenal, crucial for working with the Standard Template Library (STL).
Call to Action
Challenge yourself to implement `for_each` in your next C++ project. Experiment with different containers, functions, and combinations with other STL algorithms. Your code quality will surely see an improvement!
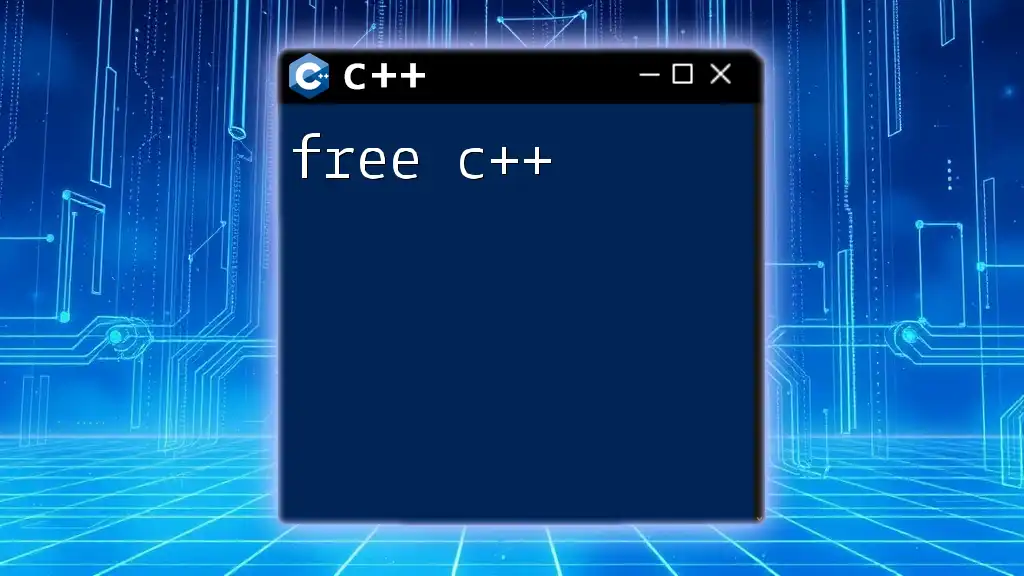
Additional Resources
- For further reading, consider exploring books like “Effective C++” by Scott Meyers or “C++ Primer” by Stanley B. Lippman.
- Online platforms like Stack Overflow, C++ reference documentation, and coding forums can provide additional support and answers to common questions regarding `for_each` and general C++ programming.
Comments Section
We invite you to share your experiences with `for_each` in your projects. What challenges did you face, and how did you overcome them? Feel free to ask questions or provide suggestions for further reading!