In C++, the `fork` command is used to create a new process by duplicating the calling process, allowing for concurrent execution of multiple processes.
Here's a simple code snippet demonstrating how to use `fork` in C++:
#include <iostream>
#include <unistd.h>
int main() {
pid_t pid = fork(); // Create a new process
if (pid == -1) {
std::cerr << "Fork failed" << std::endl;
return 1; // Error occurred
} else if (pid == 0) {
// This block is executed by the child process
std::cout << "Hello from the child process!" << std::endl;
} else {
// This block is executed by the parent process
std::cout << "Hello from the parent process!" << std::endl;
}
return 0;
}
What is Fork in C++?
The fork() function is a crucial element in C++ programming for creating new processes. It allows a program to split itself into two processes: the original (parent) and the newly created process (child). This function is part of the POSIX standard and is commonly used in Unix/Linux environments to enable multitasking and parallel processing.
Understanding the difference between processes and threads is vital when discussing fork. A process is an independent execution environment with its own memory, while threads are lighter, sharing memory space within a process. fork() effectively allows a program to create individual processes that run concurrently.
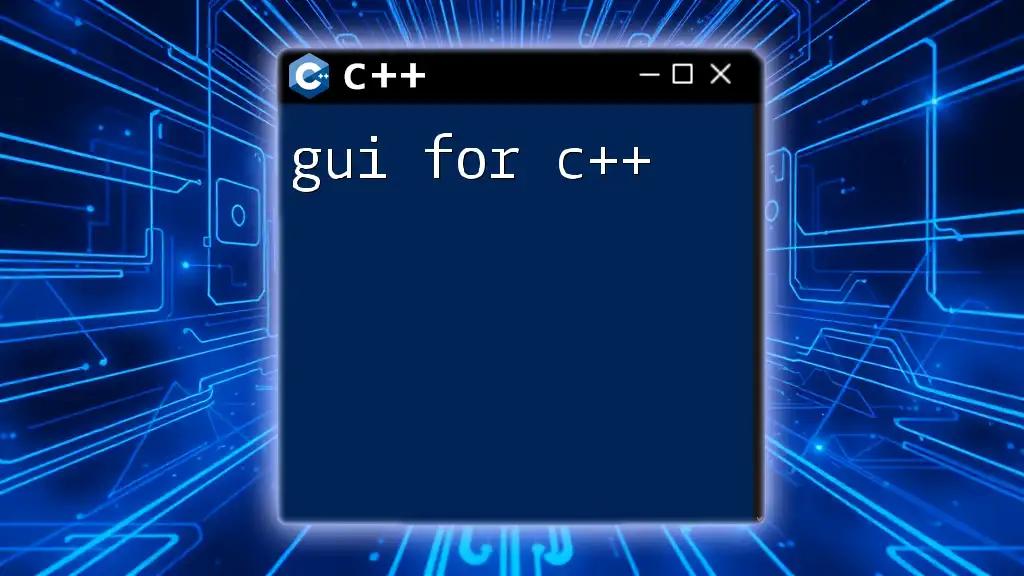
How Fork Works
When you invoke fork(), it performs a series of actions:
- It creates a new process by duplicating the calling process.
- After the call, two processes continue execution: the original (parent) and the duplicated (child).
- Both processes receive a unique process ID (PID).
Return values from fork() are critical to understanding its operation:
- If fork() returns 0, the code is executing in the child process.
- If it returns a positive value, the code is executing in the parent, and the value represents the child’s PID.
- A return value of -1 indicates an error, highlighting that the process could not be created.
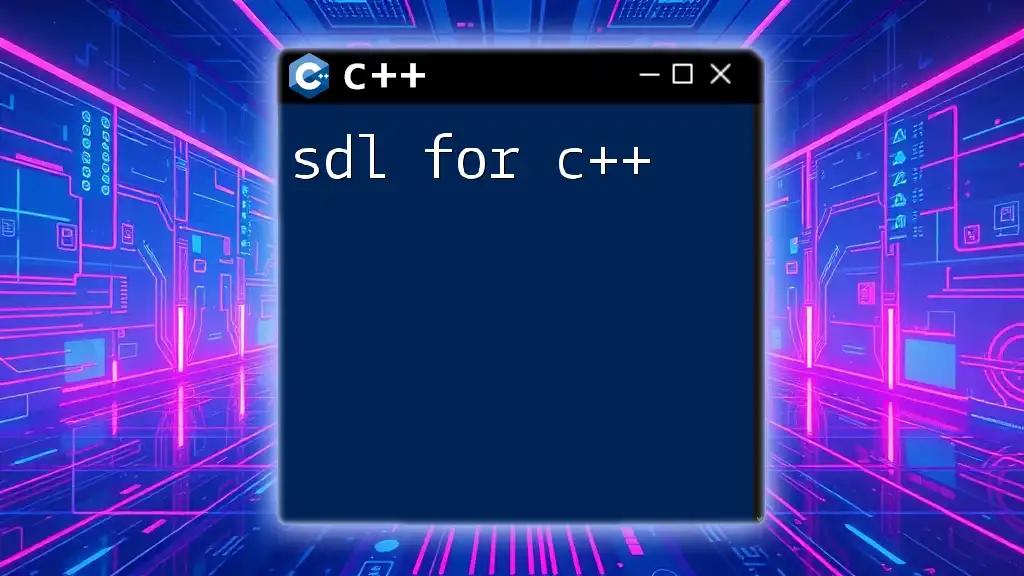
Basic Syntax of Fork
The syntax for using fork() in C++ is straightforward:
pid_t fork(void);
To utilize this function, you must include specific headers at the beginning of your program:
#include <unistd.h>
#include <sys/types.h>
These headers provide the necessary functionality and types required to work with processes.
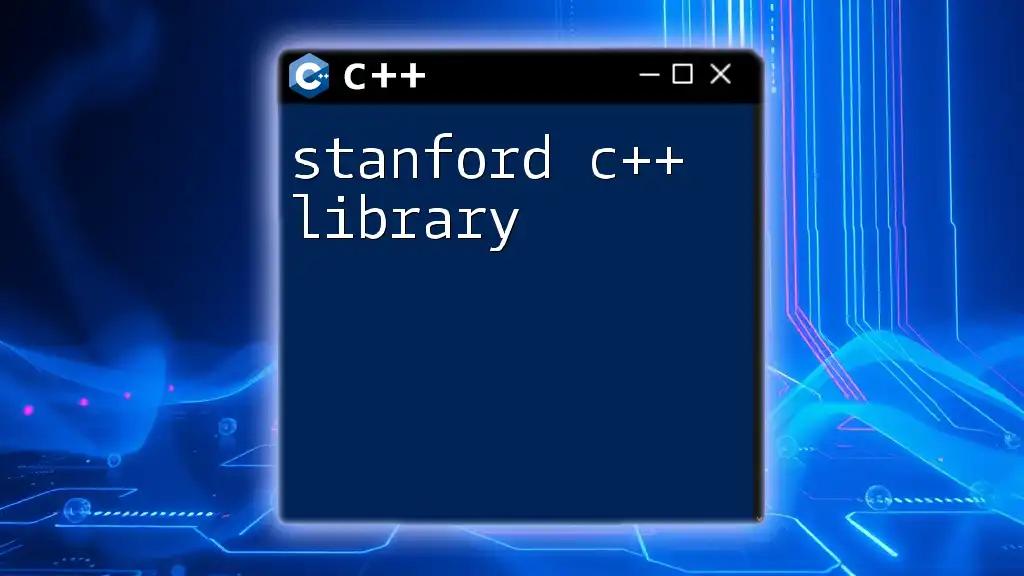
Example of Using Fork
Here’s a basic example that demonstrates the functionality of fork():
#include <iostream>
#include <unistd.h>
int main() {
pid_t pid = fork();
if (pid < 0) {
std::cerr << "Fork failed." << std::endl;
return 1;
} else if (pid == 0) {
std::cout << "Hello from the Child Process!" << std::endl;
} else {
std::cout << "Hello from the Parent Process!" << std::endl;
}
return 0;
}
In this code, after calling fork(), two separate processes are created:
- In the child process, the output will display "Hello from the Child Process!".
- In the parent process, the output will show "Hello from the Parent Process!".
This code snippet illustrates how you can identify the role of each process based on the return value of fork().
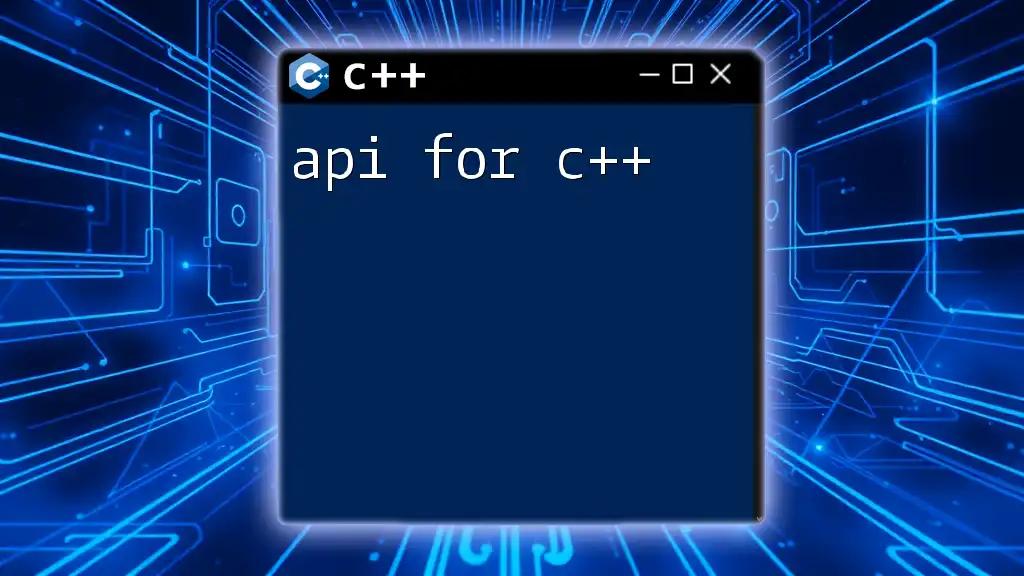
Handling Multiple Forks
You can use fork() multiple times to create several child processes. Here’s an example demonstrating this concept:
#include <iostream>
#include <unistd.h>
int main() {
for (int i = 0; i < 3; i++) {
pid_t pid = fork();
if (pid < 0) {
std::cerr << "Fork failed." << std::endl;
return 1;
} else if (pid == 0) {
std::cout << "Child " << i + 1 << " process with PID " << getpid() << std::endl;
return 0; // Ensure child processes terminate here
}
}
std::cout << "I am the Parent Process." << std::endl;
return 0;
}
In this code:
- A loop creates three child processes.
- Each child process outputs its identifier (PID).
- The parent process confirms its existence after spawning child processes.
This illustrates how multiple fork() calls can provide concurrent process execution.
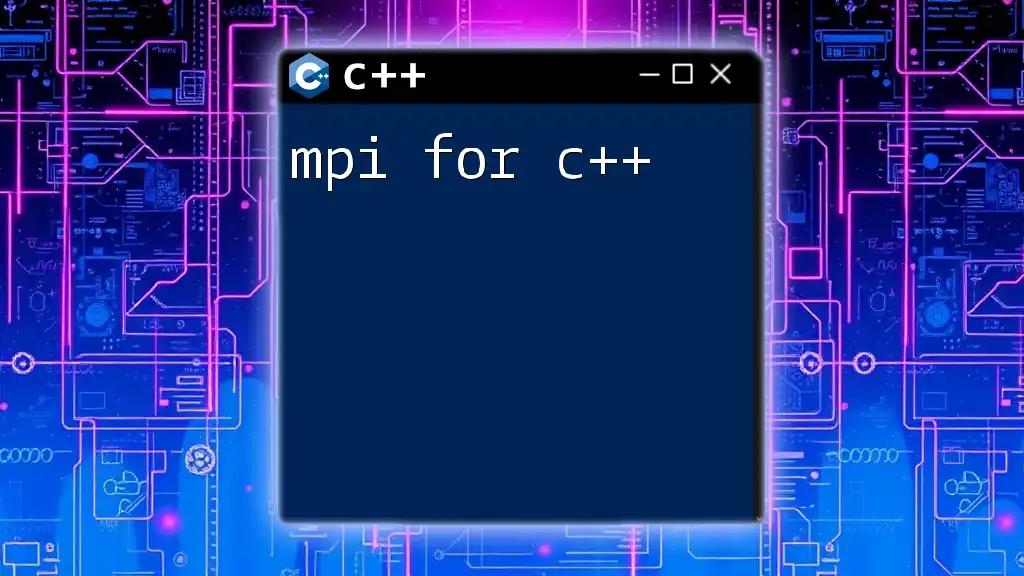
Handling Errors and Return Values
Error handling is an essential aspect of using fork(). When invoking the function, a return value of -1 indicates a failure in creating a child process, often due to system resource limitations or exceeding the maximum allowable number of processes.
To troubleshoot issues related to fork(), always check the return value. Here’s how you might handle errors:
pid_t pid = fork();
if (pid < 0) {
perror("Fork failed"); // Use perror for error details
exit(EXIT_FAILURE);
}
Utilizing `perror()` provides an informative error message that can help diagnose problems with process creation.

Fork and Process Synchronization
When employing fork(), one must consider process synchronization—especially in applications that require coordinated execution. If a parent process initiates a child process, it can call `wait()` to ensure that it waits for the child to complete before proceeding. Here's a simple example:
#include <iostream>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid = fork();
if (pid == 0) {
// Child process
std::cout << "Child process, PID: " << getpid() << std::endl;
} else {
// Parent process
wait(NULL); // Wait for child process to finish
std::cout << "Parent process, PID: " << getpid() << std::endl;
}
return 0;
}
In this scenario, the parent process waits until the child process completes its execution. This synchronization prevents conflicts that might arise from concurrent operations.
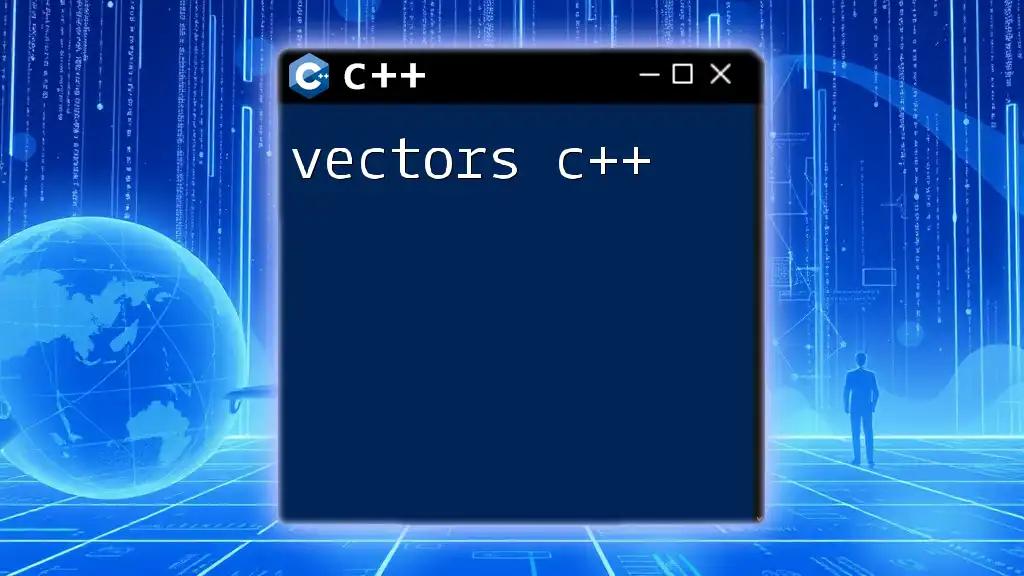
Common Use Cases for Fork
The fork() function is widely used across various applications, including:
-
Server Applications: In networking, servers use fork() to handle multiple client requests simultaneously, creating a new child process for each client.
-
Parallel Processing: In scientific computing, complex tasks can be divided into subtasks handled by separate processes for efficiency.
-
Utilities and Command-Line Tools: Many command-line utilities utilize fork() to perform tasks in parallel, improving performance and responsiveness.
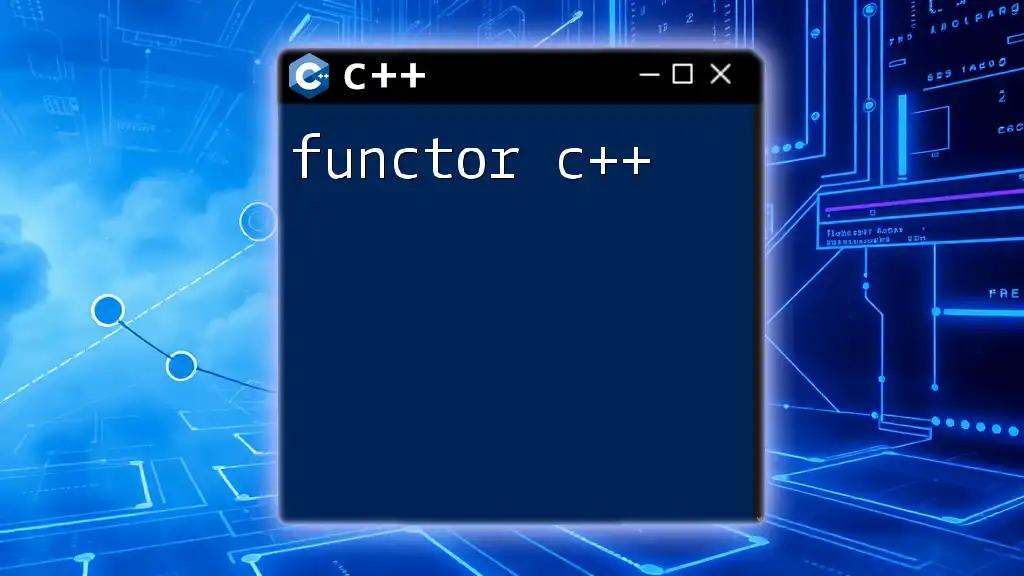
Best Practices When Using Fork
When using fork(), it’s crucial to adopt best practices to maintain system stability and performance:
-
Resource Management: Ensure that child processes are managed effectively to prevent wastage of resources.
-
Avoiding Zombie Processes: Implement proper wait mechanisms to prevent zombie processes, which occur when a child process has completed, but the parent has not yet read its exit status.
-
Proper Exit Handling: Always call `exit()` within the child process to ensure it does not inadvertently execute the parent’s code after forking.
-
Signal Handling: Think about the implications of signals (like SIGCHLD) for process termination and how they may affect your application’s behavior.
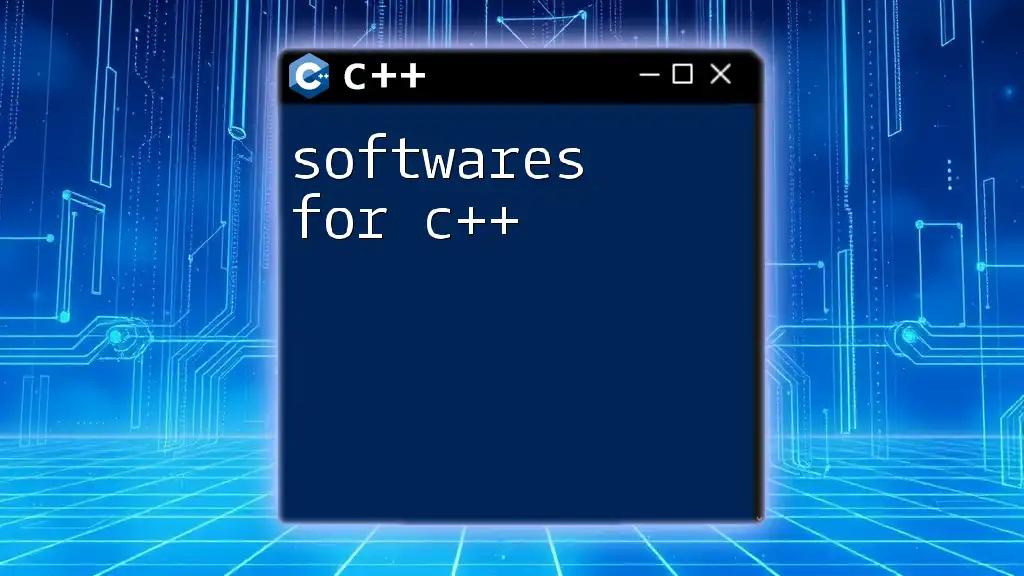
Conclusion
The fork c++ function is an indispensable tool for creating processes in C++ programming, enabling multitasking and efficient CPU usage. Understanding its operation, error handling, and best practices is vital for any developer aiming to write robust and scalable applications. As you explore and practice using fork(), you will encounter diverse scenarios that highlight its versatility and power in process management.