MPI (Message Passing Interface) for C++ is a standardized and portable message-passing system designed to enable parallel programming in distributed computing environments.
#include <mpi.h>
#include <iostream>
int main(int argc, char** argv) {
MPI_Init(&argc, &argv); // Initialize MPI
int world_size;
MPI_Comm_size(MPI_COMM_WORLD, &world_size); // Get the size of the communicator
int world_rank;
MPI_Comm_rank(MPI_COMM_WORLD, &world_rank); // Get the rank of the process
std::cout << "Hello from process " << world_rank << " out of " << world_size << std::endl;
MPI_Finalize(); // Finalize MPI
return 0;
}
What is MPI?
The Message Passing Interface (MPI) is a standard for parallel programming that enables the efficient communication of data among processes in a distributed or parallel computing environment. MPI is critical for applications that require high-performance computing across multiple nodes, making it a preferred choice for developers working with complex computational tasks.
Why Use MPI with C++?
Using MPI in conjunction with C++ allows for better performance improvements in parallel applications. The ability to easily share data and synchronize processes across computing nodes enables developers to scale their applications seamlessly. Real-world applications include scientific simulations, data analysis, and computational models, where precise communication and calculation are essential for accuracy and efficiency.
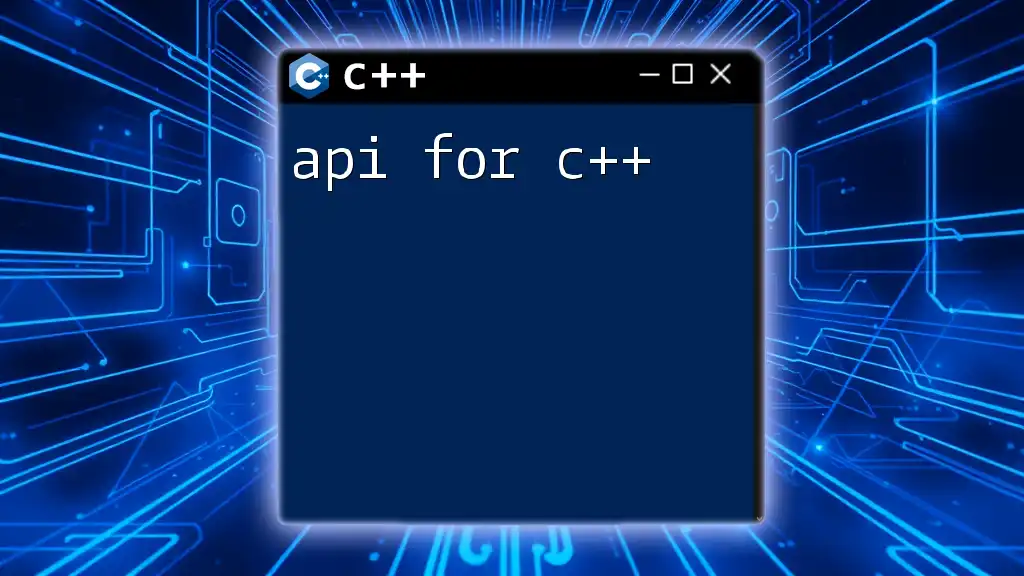
Setting Up MPI for C++
Installing MPI
Before diving into coding with MPI, you need to have an MPI implementation set up on your machine. Two popular options are Open MPI and MPICH. Follow these steps to install MPI on different platforms:
-
For Linux (Ubuntu), you can install Open MPI using:
sudo apt-get install libopenmpi-dev openmpi-bin
-
For Windows, it is recommended to use the MS-MPI version and follow the instructions on the official Microsoft website for downloading and setup.
-
For macOS, you might use Homebrew to install Open MPI:
brew install open-mpi
Setting Up a C++ Development Environment
Once MPI is installed, you need to configure your C++ development environment:
- Set up your preferred IDE (such as Visual Studio or Code::Blocks) to recognize the MPI libraries.
- Ensure your compilation commands are set up to link against MPI. For instance, you would typically use:
mpic++ -o my_mpi_program my_mpi_program.cpp
Example Code for a Simple MPI Program
To get started, let’s look at a simple MPI program that initializes the environment, fetches the rank of a process, and outputs a message.
#include <mpi.h>
#include <iostream>
int main(int argc, char** argv) {
MPI_Init(&argc, &argv);
int rank;
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
std::cout << "Hello from process " << rank << std::endl;
MPI_Finalize();
return 0;
}
This code showcases the essential steps of initializing MPI, retrieving the rank of the process, and finalizing the MPI environment.
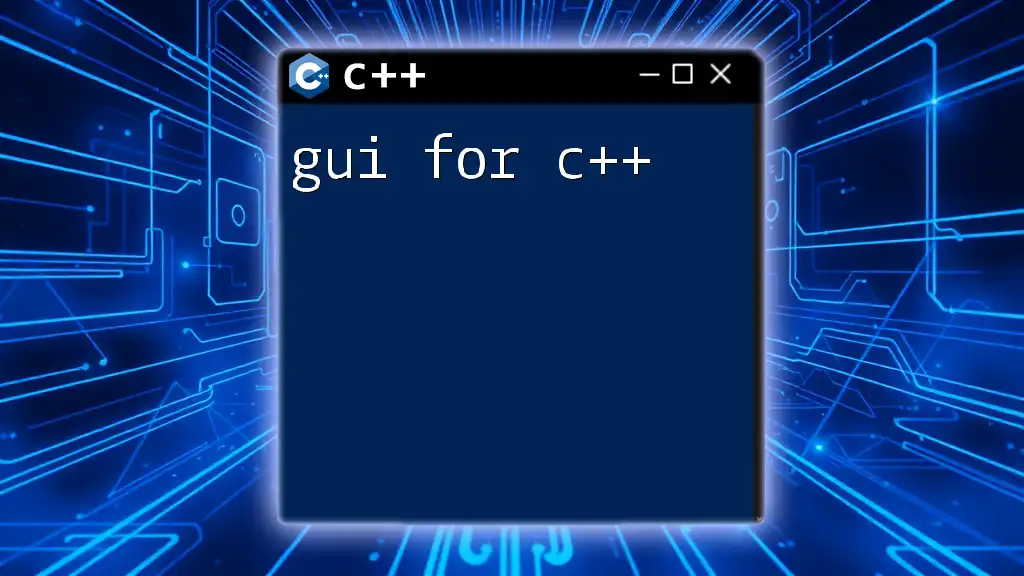
Understanding the Basics of MPI
Key Concepts in MPI
Processes
In an MPI program, processes are the fundamental units of execution. Each process runs independently and can communicate with other processes using MPI functions. Understanding how processes interact is crucial for effective parallel programming.
Communicators
A communicator is a set of processes that can communicate with each other. `MPI_COMM_WORLD` is the default communicator that includes all the processes that were launched when the MPI program started. Using communicators correctly is essential for managing which processes can exchange messages.
MPI Functions and Syntax
Initialization and Finalization
Two crucial functions in MPI are `MPI_Init` and `MPI_Finalize`:
-
`MPI_Init`: Initializes the MPI environment and must be called before any other MPI functions. It sets up the necessary resources for running MPI applications.
-
`MPI_Finalize`: Cleans up the MPI environment. This function must be called before the program exits to ensure that all resources are properly released.
Sending and Receiving Messages
Communication in MPI can be categorized into point-to-point and collective communication.
For point-to-point communication, sending and receiving messages is typically handled with two functions:
- `MPI_Send`: Used by a process to send a message to another process.
- `MPI_Recv`: Used by a process to receive a message from another process.
An example of the send operation looks like this:
MPI_Send(&data, count, datatype, destination, tag, MPI_COMM_WORLD);
This code snippet sends `data` to a specified `destination` process, where `count` defines how many elements are being sent and `datatype` indicates the type of data being sent.
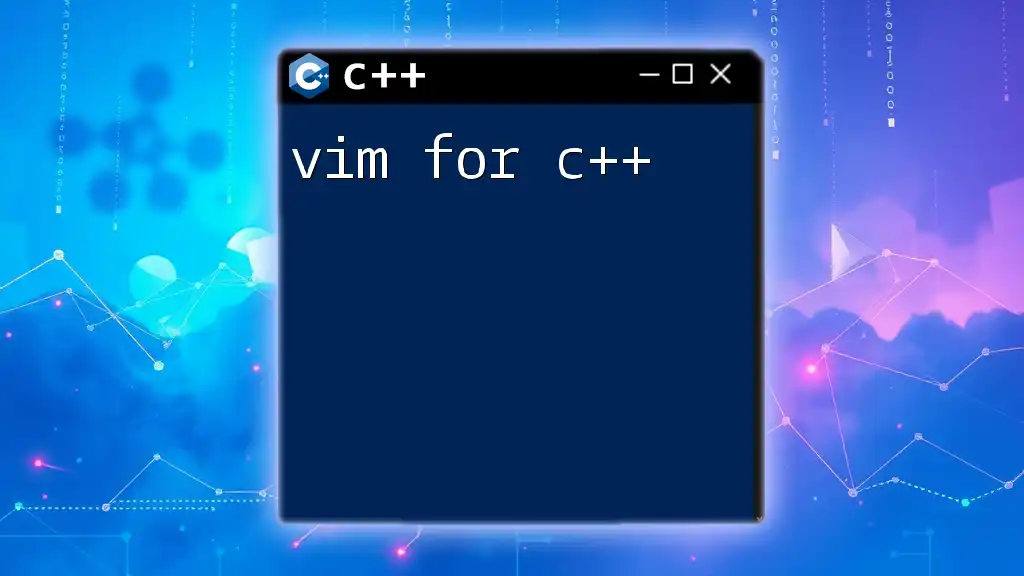
Advanced MPI Concepts
Point-to-Point Communication
In point-to-point communication, data is sent from one process to another directly. This method is crucial when processes need to exchange information or share results specifically with one another, creating a streamlined flow of data.
Collective Communication
Collective communication involves multiple processes participating in data exchange collectively. Some major collective operations include:
Broadcast and Scatter
Broadcasting is when one process sends the same data to all other processes. This can be achieved using `MPI_Bcast`, which simplifies the process of uniformly distributing data across all tasks.
MPI_Bcast(&data, count, datatype, root, MPI_COMM_WORLD);
In contrast, scatter distributes data from one process to all other processes, where each receives a unique segment of the data. Master processes often utilize this to allocate tasks or data slices.
Gather and Reduce
Gather operations involve collecting data from multiple processes into one single process. Using `MPI_Gather`, you can easily aggregate data:
MPI_Gather(sendbuf, sendcount, sendtype, recvbuf, recvcount, recvtype, root, MPI_COMM_WORLD);
Reduce operations are utilized to combine information and return a single value to a designated root process, such as calculating sums or maxima from distributed data.
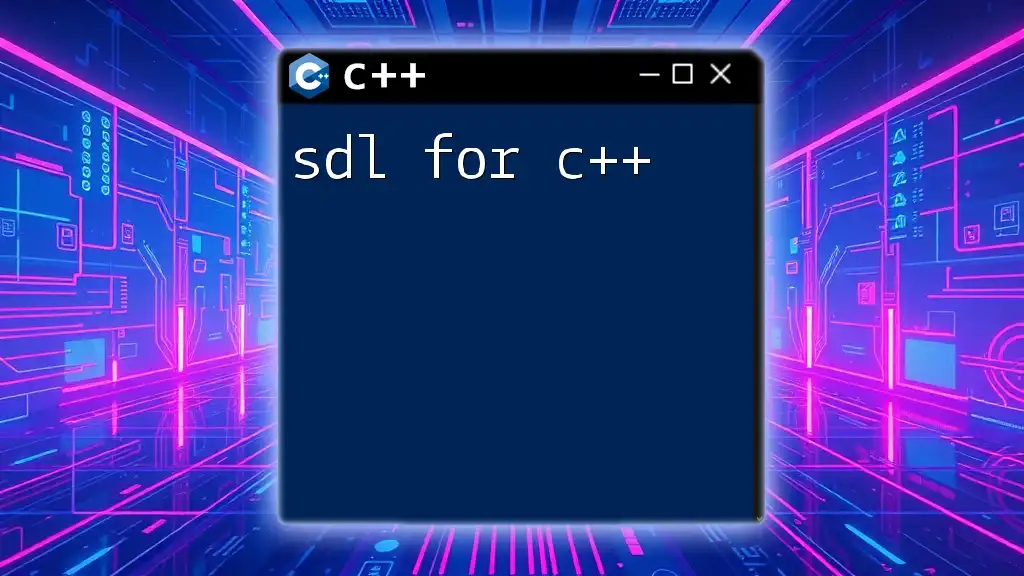
Error Handling in MPI
Common MPI Errors
Errors can arise from various issues such as communication failures or incorrect message formats. MPI utilizes specific error codes to manage these issues effectively.
Debugging MPI Programs
Debugging distributed applications can be challenging due to their concurrent nature. However, tools such as `TotalView`, `DDT`, and `GDB` can help in tracing and analyzing issues. Good practices include checking for error returns from MPI calls and using logging to track execution flow.
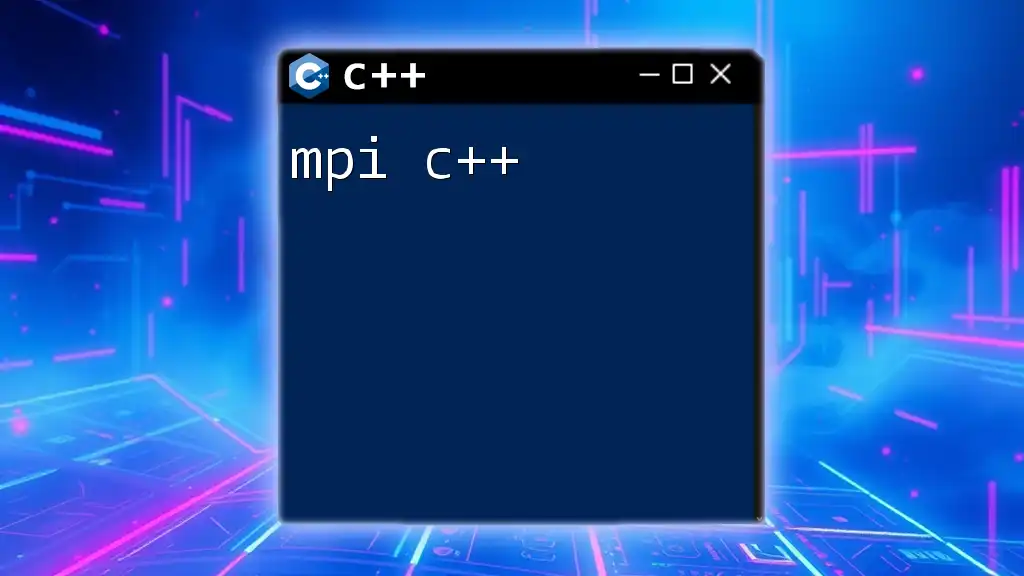
Best Practices for Writing MPI Programs
Performance Considerations
To maximize performance in MPI applications, consider the following tips:
- Minimize communication: Strive for fewer data exchanges; combine messages when feasible to reduce overhead.
- Prefetch data: Reduce latency by transferring data in advance when possible.
Writing Scalable MPI Applications
To ensure scalability, focus on developing algorithms that can dynamically adjust based on the number of processes available. Always design your code to handle increases in workload without significant changes in structure.
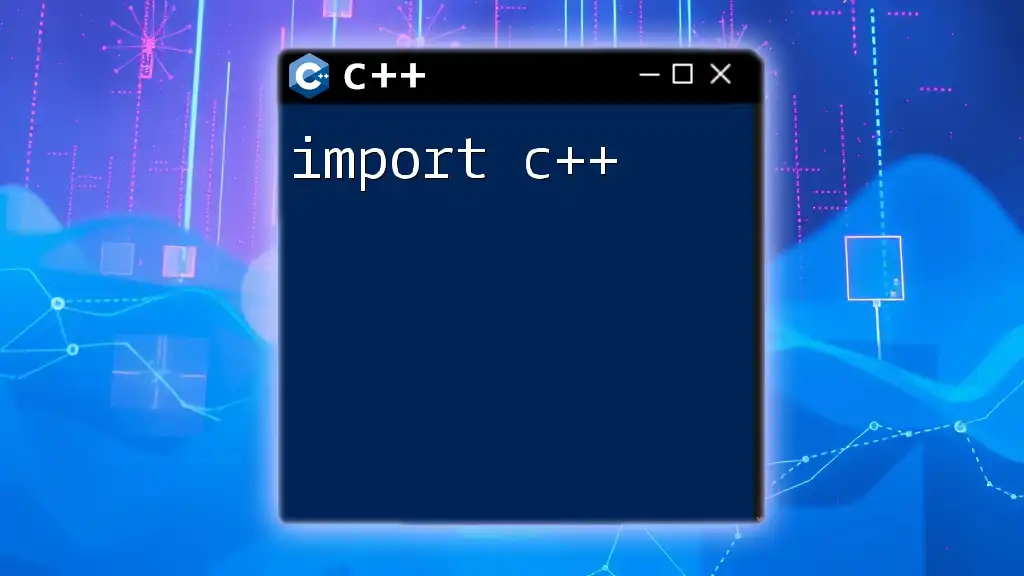
Real-World Applications and Case Studies
Scientific Computing
MPI is widely used in scientific computing for simulations in fields like physics and chemistry. For example, researchers modeling climate systems or molecular interactions depend on MPI to handle large data sets and intricate calculations efficiently.
Data Analysis in Big Data
In the realm of big data, MPI proves invaluable. It enables rapid processing of massive datasets by distributing the workload across multiple nodes. Companies harness MPI to perform complex analyses on extensive data stores, ensuring quick turnaround times and insightful results.
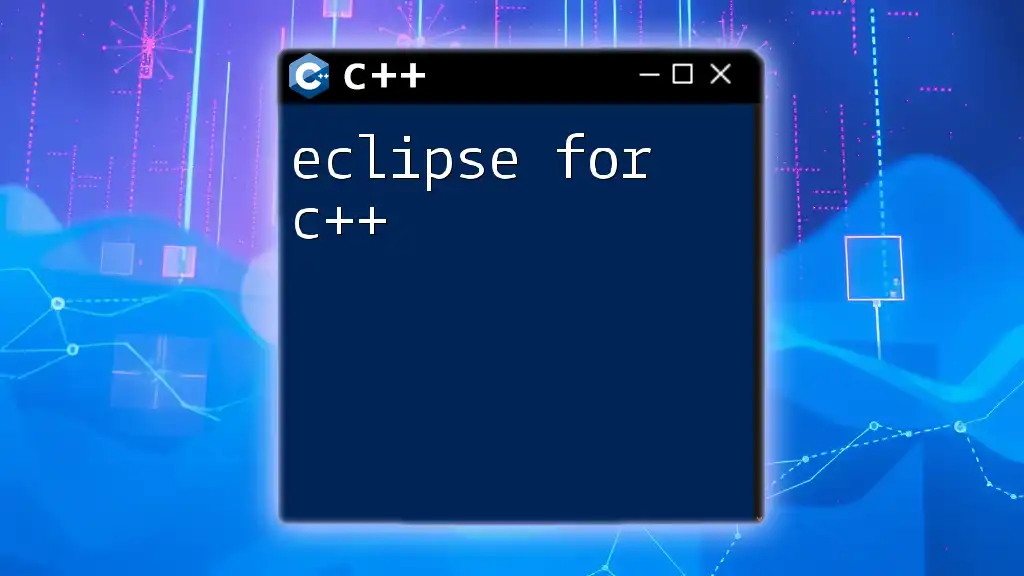
Conclusion
Embracing MPI for C++ opens up a world of possibility in parallel computing. By understanding its foundational concepts, leveraging its full range of communication capabilities, and adhering to best practices, developers can build powerful, scalable applications that make use of multicore and distributed systems.
Additional Resources
If you’re looking to dive deeper into MPI for C++, numerous resources are available, including textbooks like "Using MPI" by Gropp et al., and online platforms offering courses on parallel programming. Additionally, engaging with online communities and forums can provide ongoing support and insights as you continue your journey into parallel programming with MPI.
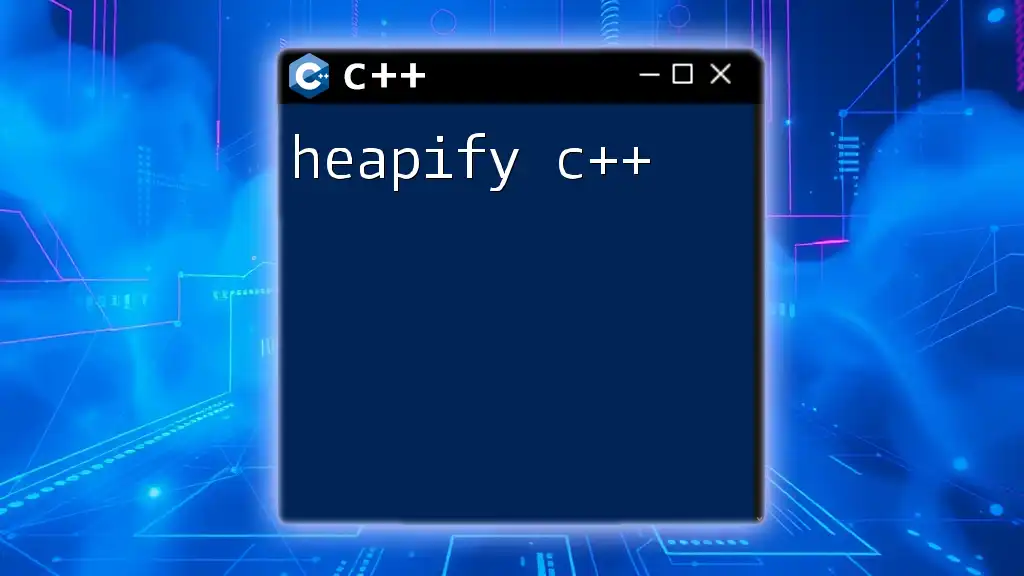
Call to Action
Try implementing a simple MPI project today! Experiment with sending and receiving messages or run a basic simulation using multiple processes. If you’re interested in comprehensive training or need technical support while working with MPI for C++, feel free to reach out.