In C++, you can calculate the value of π (pi) using the `atan` function from the `<cmath>` library, as shown in the following code snippet:
#include <iostream>
#include <cmath>
int main() {
double pi = 4 * atan(1);
std::cout << "Value of Pi: " << pi << std::endl;
return 0;
}
What is Pi?
Pi (π) is a mathematical constant that represents the ratio of a circle's circumference to its diameter. With an approximate value of 3.14159, Pi is an irrational number, meaning it cannot be exactly expressed as a fraction, and its decimal representation goes on forever without repeating. Its significance stretches across various fields, including mathematics, engineering, physics, and computer science, making it an essential constant in both theoretical concepts and practical applications.
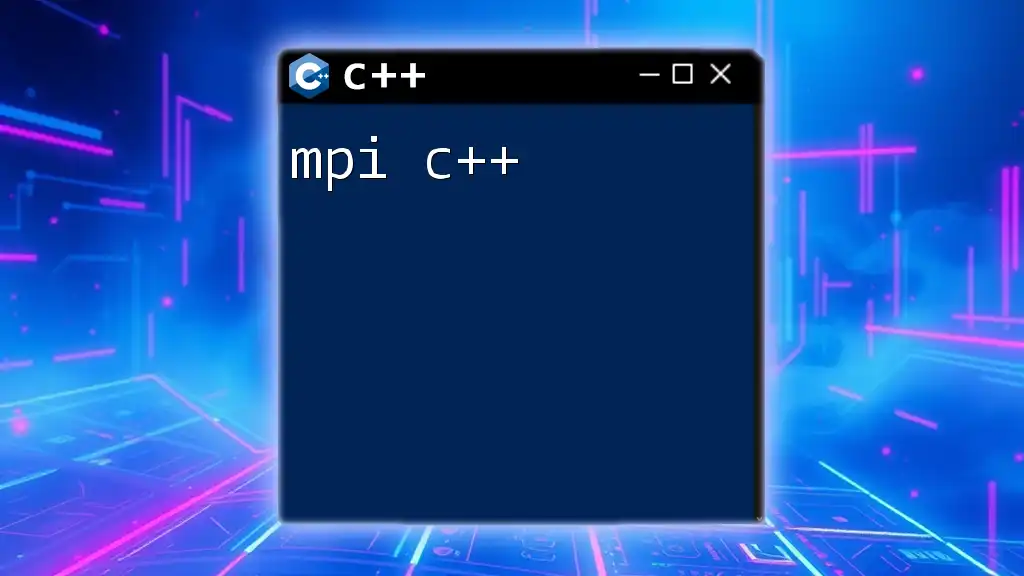
Pi and C++
C++ leverages mathematics in its programming constructs, and Pi is no exception. In C++, Pi is utilized primarily in calculations involving geometry, particularly those related to circles and curves. Its importance lies not only in its mathematical relevance but also in how it simplifies the coding process, allowing programmers to concentrate on logic rather than worrying about numerical precision.
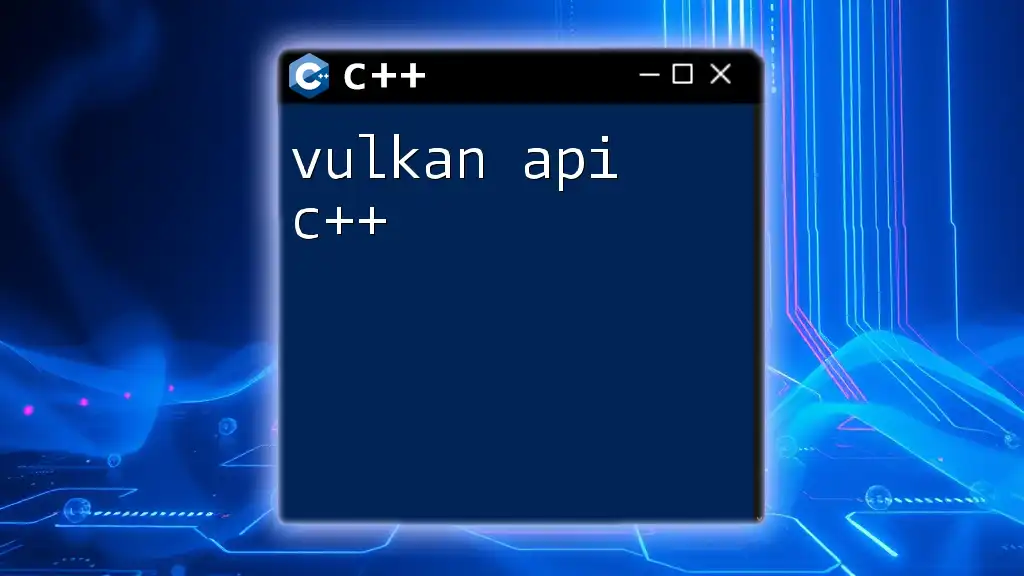
Understanding the Pi Constant in C++
What is “M_PI”?
In C++, Pi can be found predefined in the `<cmath>` library as the constant M_PI. This predefined constant provides a reliable and precise value for Pi, preventing users from needing to define it repeatedly. Utilizing predefined constants like M_PI ensures better readability and maintainability of code, as it allows developers to use standardized values instead of hardcoding them.
How to Write Pi in C++
For those who prefer or need to define Pi themselves, here's how to declare it as a constant in C++:
const double PI = 3.141592653589793;
This ensures that the value of PI is recognized throughout your program and can be used consistently in any mathematical calculations.
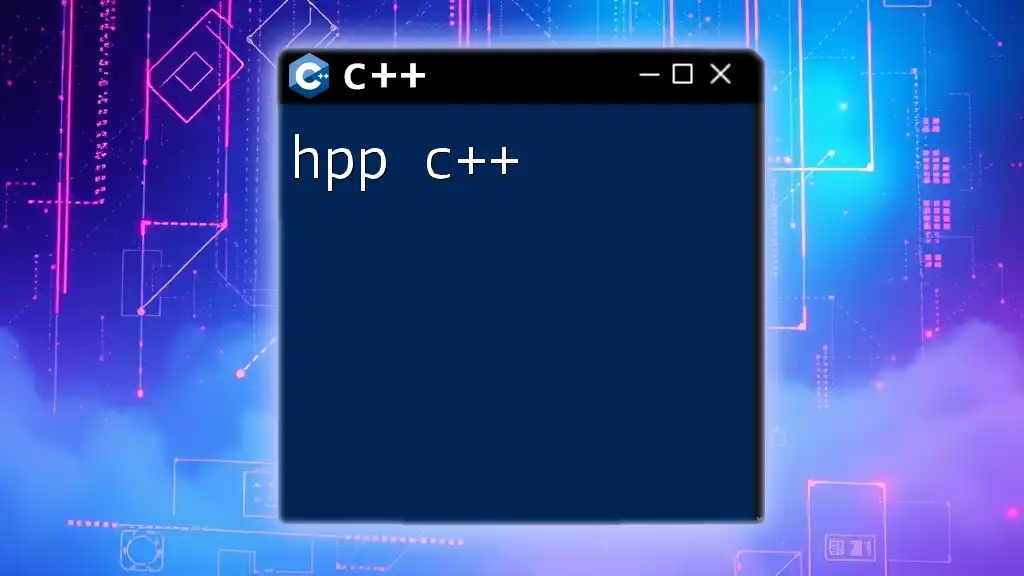
How to Use Pi in C++
Basic Usage of Pi
Utilizing Pi in C++ can be straightforward, especially for basic geometric calculations. Consider the following example of calculating the circumference of a circle:
double radius = 5.0;
double circumference = 2 * PI * radius;
In this code, we calculate the circumference using the formula \( C = 2\pi r \). Here, substituting the value of PI makes the equation clear and precise.
Advanced Usage of Pi
For more complex calculations, such as finding the area of a circle or the volume of a sphere, you can use Pi in the following examples:
double area = PI * radius * radius; // Area of a circle
double volume = (4.0 / 3.0) * PI * radius * radius * radius; // Volume of a sphere
These snippets demonstrate how Pancakes can simplify mathematical expressions and improve computational clarity.
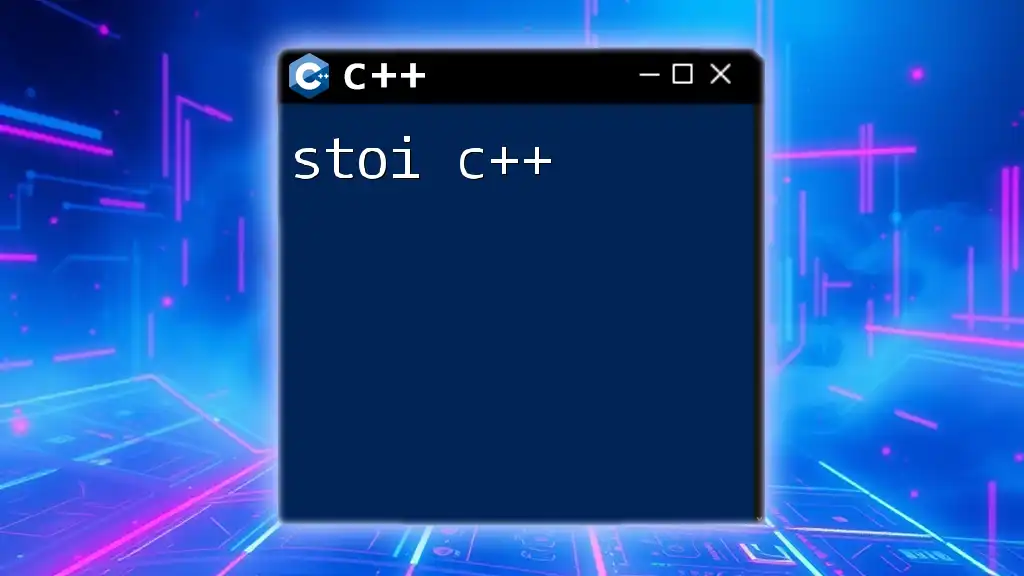
C++ Math Library and Pi
Using <cmath> for Mathematical Calculations
C++ includes a robust math library through the `<cmath>` header, which provides a wide range of mathematical functions that can work seamlessly with Pi. This elevates the precision and functionality of mathematical operations in your programs.
Examples of CMATH and Pi
Here is a simple example of using the `sin` function from the `<cmath>` library in conjunction with Pi:
#include <cmath>
double sin_value = sin(PI / 2.0); // Using sin with Pi
In this case, computing the sine of π/2 demonstrates how utilizing Pi in various mathematical functions simplifies the process while ensuring accuracy.
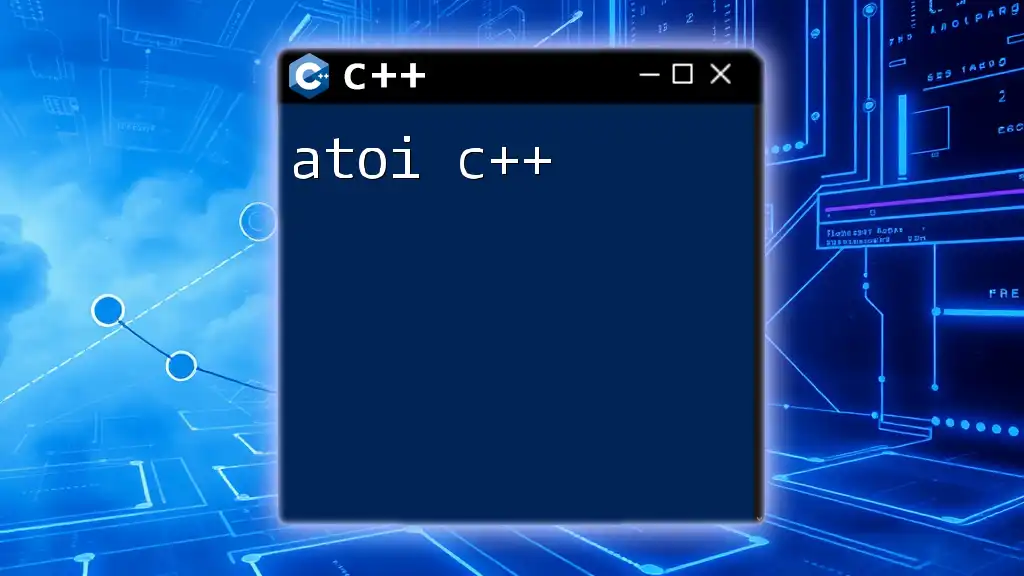
C++ Best Practices with Pi
Constants vs. Hardcoding Values
One of the best practices in programming is to avoid hardcoding values, which can lead to errors and make code less readable. Instead of writing:
double perimeter = 2 * 3.14 * radius; // Hardcoding
You can improve your code's clarity by using a defined constant:
double perimeter = 2 * PI * radius; // Using constant
This clear approach not only enhances maintainability but also ensures consistency across your calculations.
Utilizing the M_PI Constant
Using the defined constant M_PI is often preferred due to its reliability and the accuracy it provides over manually defined values. For example:
#include <cmath>
double circleArea = M_PI * radius * radius; // Calculate area using M_PI
In this snippet, using M_PI ensures that you are working with the most precise representation of Pi available in the library, enhancing your program's reliability.
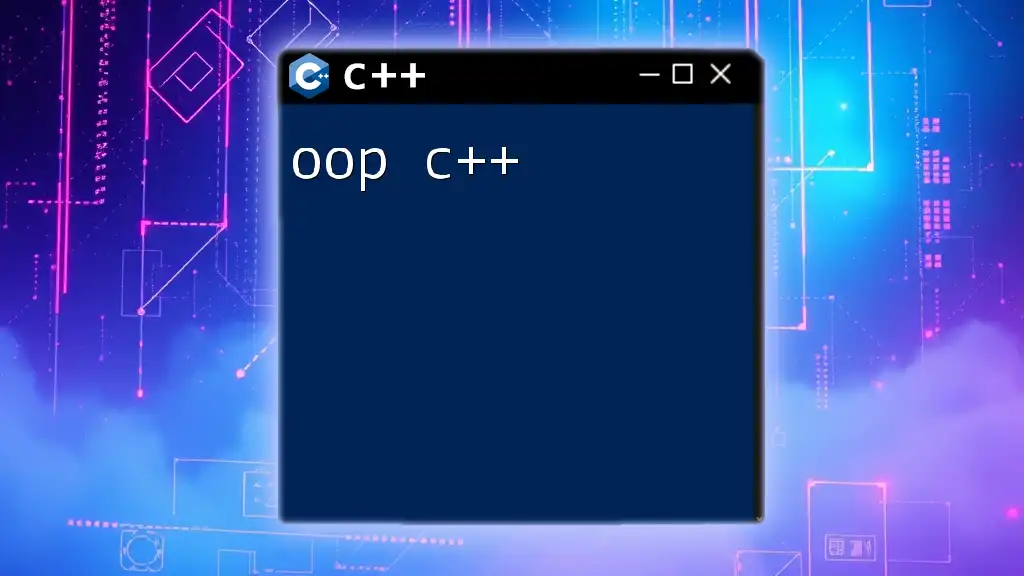
Real-World Applications of Pi in C++
Engineering and Physics Simulations
In engineering and physics, Pi plays a critical role in simulations involving circular motion or wave patterns. For instance, when modeling a pendulum's movement, the periodic function may heavily rely on the properties defined by Pi.
Computer Graphics
Pi also finds significant application in graphics programming. When creating circular shapes or arcs, understanding how to manipulate Pi can greatly enhance visual representations. For example, in rendering a circle, the equation might involve Pi to define the border's curvature.
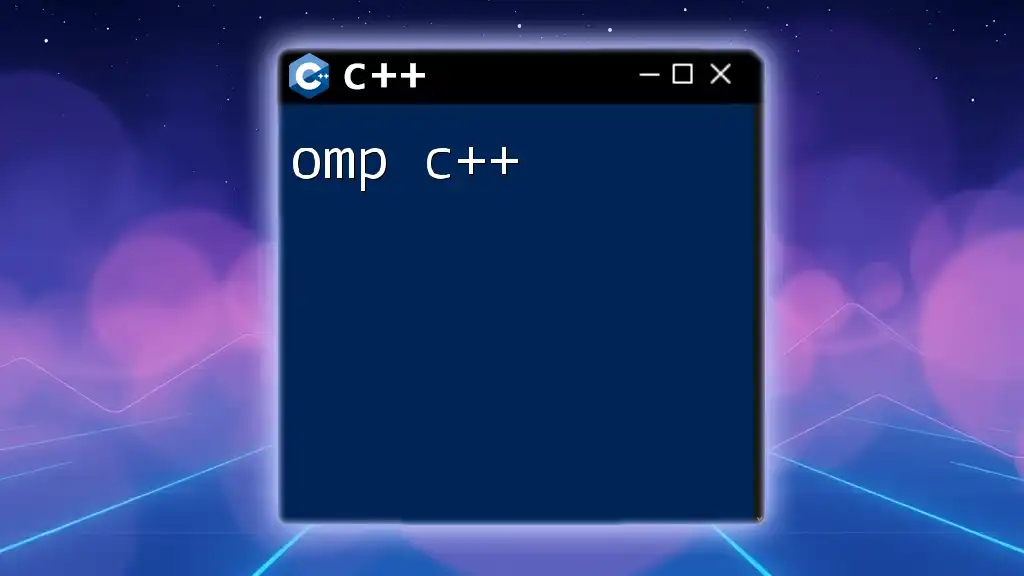
Conclusion
Understanding how to use pi in C++ can significantly improve the efficiency and clarity of your code. With its active role in various calculations and simulations, Pi serves as more than just a mathematical curiosity; it is a fundamental tool for any programmer. By taking advantage of constants like M_PI and ensuring best practices in code, you can achieve precise and readable programming standards. Whether you're developing algorithms or embarking on exciting engineering projects, mastering the use of Pi will undoubtedly enhance your programming toolkit.
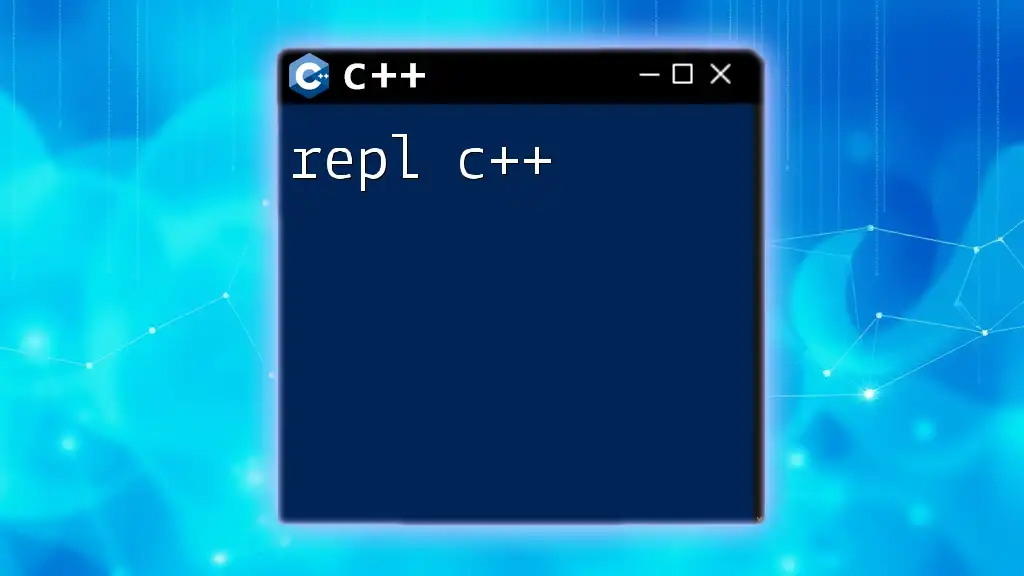
Additional Resources
For those interested in deepening their understanding of C++ and the application of mathematical constants like Pi, numerous online resources provide excellent tutorials, documentation, and communities of practice. Happy coding!