In C++, a loop allows you to execute a block of code multiple times based on a specific condition, making it efficient for repetitive tasks.
Here's a simple example of a `for` loop that prints numbers from 1 to 5:
#include <iostream>
int main() {
for (int i = 1; i <= 5; i++) {
std::cout << i << std::endl;
}
return 0;
}
Understanding Loops in C++
What is a Loop?
A loop is a fundamental programming structure that allows you to execute a block of code repeatedly based on a specified condition. In C++, loops simplify the task of performing repetitive operations, enhancing the efficiency and maintainability of your code. Rather than writing the same lines of code multiple times, you can use loops to reduce redundancy and improve readability.
Types of Loops
In C++, there are primarily three types of loops, each suited for different scenarios:
- For Loops: Designed for scenarios where you know how many times you want to execute a block of code.
- While Loops: Best used when the number of iterations is not predetermined and depends on a condition being true.
- Do-While Loops: Similar to while loops, but they execute the code block at least once before checking the condition.
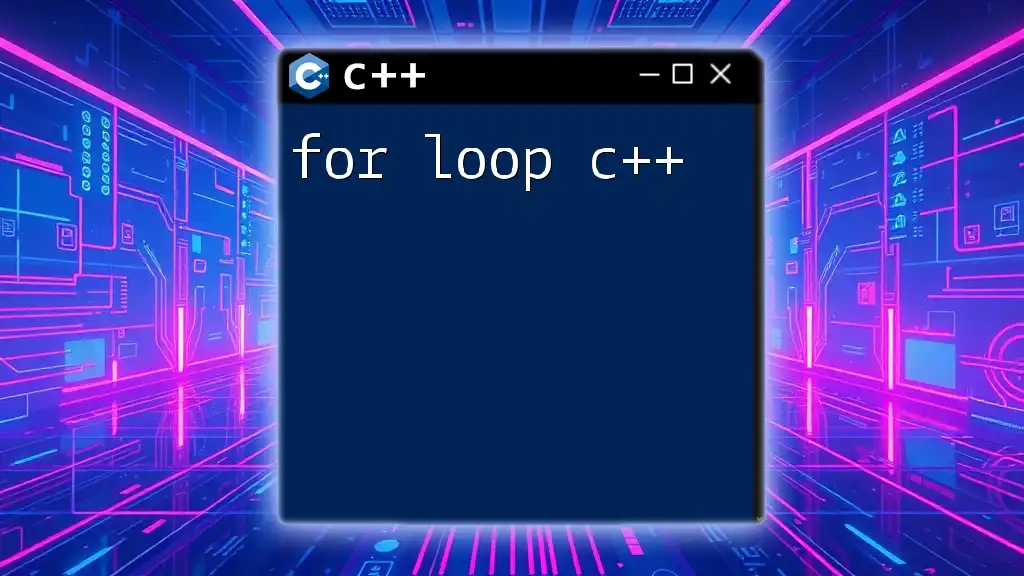
How to Use a For Loop in C++
Syntax of a For Loop
The syntax of a for loop in C++ is quite straightforward:
for(initialization; condition; increment/decrement) {
// code block to be executed
}
This structure allows for clarity in how the loop operates, as all parts of the loop are visible at a glance.
Breaking Down the Syntax
-
Initialization: This is where you declare and initialize your loop control variable. It only executes once at the start of the loop.
-
Condition: Before each iteration, this condition is evaluated. If it returns true, the loop executes. If false, the loop terminates.
-
Increment/Decrement: This step adjusts the loop control variable after each iteration, allowing the loop to progress toward its termination condition.
Example of a Basic For Loop
Here’s a simple example of a for loop:
#include <iostream>
using namespace std;
int main() {
for(int i = 0; i < 5; i++) {
cout << "Iteration: " << i << endl;
}
return 0;
}
In this example, the loop runs five times, outputting the current value of `i` during each iteration. Each iteration increments `i` with `i++`, demonstrating how the loop control variable progresses.
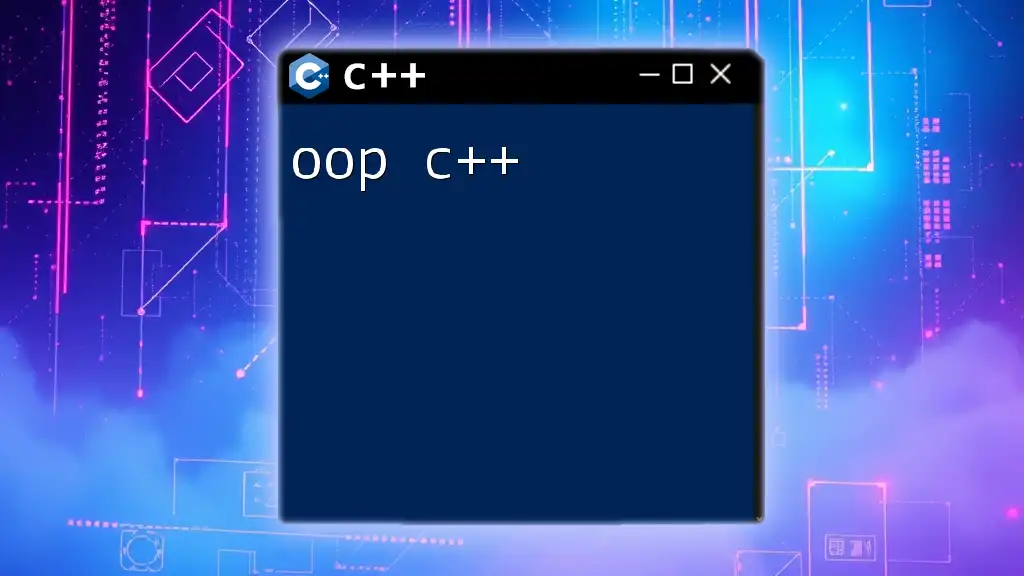
Advanced Usage of For Loops
Nested For Loops
Nested loops allow for complex operations and are especially useful in scenarios like working with multi-dimensional arrays or generating combinations. A nested for loop is simply a for loop inside another for loop.
Here’s an example:
#include <iostream>
using namespace std;
int main() {
for(int i = 1; i <= 3; i++) {
for(int j = 1; j <= 2; j++) {
cout << "i: " << i << ", j: " << j << endl;
}
}
return 0;
}
In this example, for each value of `i`, the inner loop executes completely. This setup would print `i` and `j` together, demonstrating how nested loops can handle multiple levels of iteration.
Using For Loops with Arrays
For loops are particularly powerful when iterating through arrays, allowing you to access each element in a structured manner.
Here's how you might iterate through an array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {10, 20, 30, 40, 50};
for(int i = 0; i < 5; i++) {
cout << "Element: " << arr[i] << endl;
}
return 0;
}
This snippet traverses the array `arr`, printing each element. The loop runs five times, corresponding to the number of elements in the array, demonstrating the synergy between loops and arrays in C++.
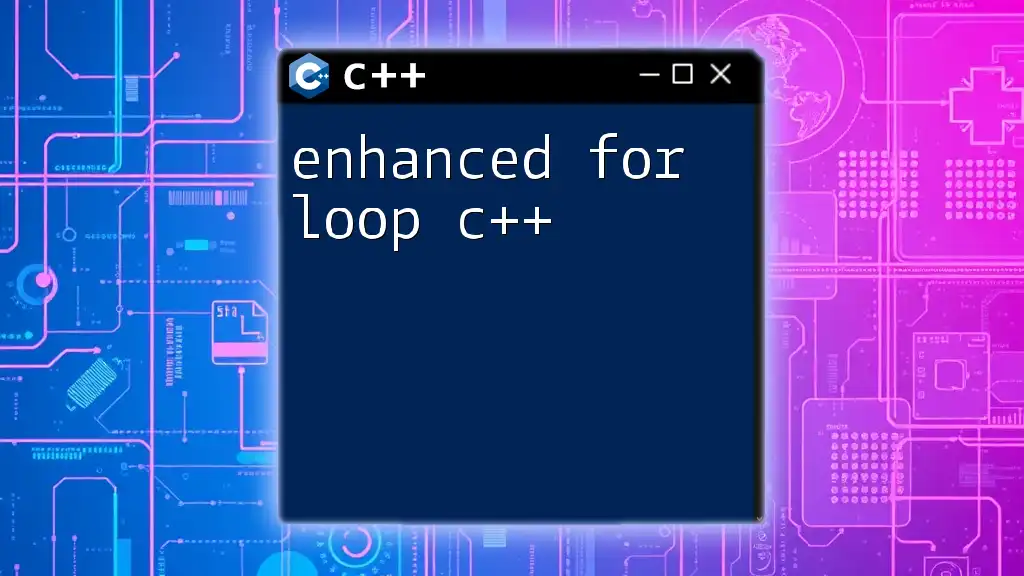
Comparing For Loop and Other Loop Types
While Loop vs. For Loop
While loops are advantageous when the number of iterations isn’t known beforehand. While loops check for a condition before executing the loop body. Here is a basic while loop example:
#include <iostream>
using namespace std;
int main() {
int i = 0;
while(i < 5) {
cout << "Iteration: " << i << endl;
i++;
}
return 0;
}
In this piece of code, the loop continues as long as `i` is less than 5, mimicking the for loop's behavior but utilizing a different structure. While for loops are often preferred for known iteration counts, while loops offer flexibility when conditions vary.
Do-While Loop Explained
The do-while loop is unique because it guarantees that the loop body is executed at least once, as the condition is checked after the loop executes. Its syntax looks similar to the following:
#include <iostream>
using namespace std;
int main() {
int i = 0;
do {
cout << "Iteration: " << i << endl;
i++;
} while(i < 5);
return 0;
}
This loop will also print values from 0 to 4, but if the condition `i < 5` were false on the first check, the loop still executes once, in contrast to the while loop. Understanding these types of loops offers flexibility in how you handle iterations in your code.
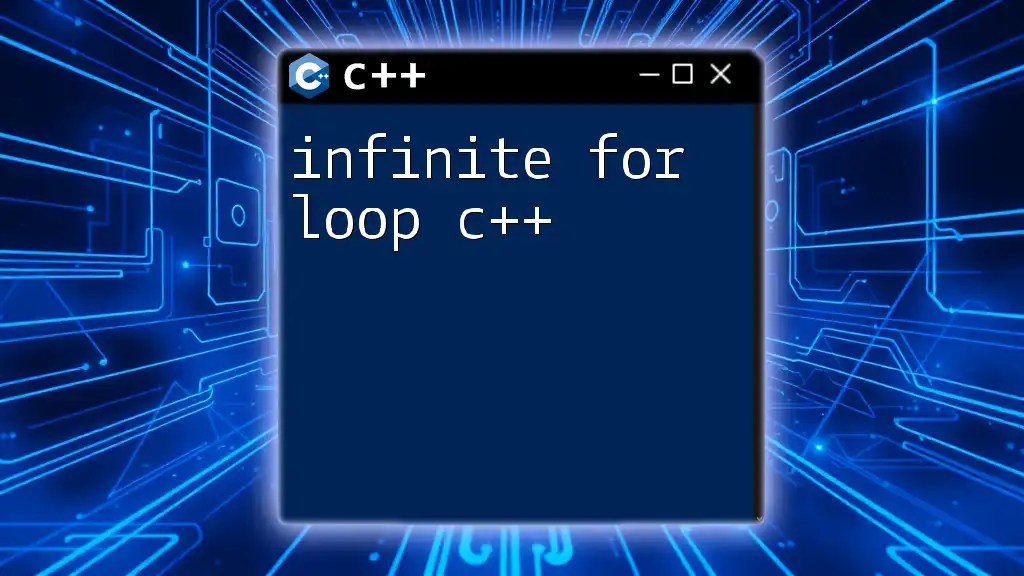
Best Practices for Using Loops in C++
Avoiding Infinite Loops
One of the most common pitfalls in using loops is creating an infinite loop, where the termination condition is never met. To avoid this:
- Always ensure that the loop variables progress appropriately.
- Debug your loops by printing values if the loop doesn’t behave as expected.
Loop Efficiency
Efficient looping can significantly affect performance, especially when dealing with large data sets or complex operations. Consider the following:
- Minimize the work done inside the loop.
- Avoid unnecessary calculations that could be performed before entering the loop.
- Use appropriate data structures that facilitate faster access times.
Readability and Maintenance
Clear and concise code improves maintainability. Make your loops easy to read by following these guidelines:
- Consistently use meaningful variable names.
- Comment on complex logic to improve understanding.
- Adopt a uniform structure to aid in visual comprehension.
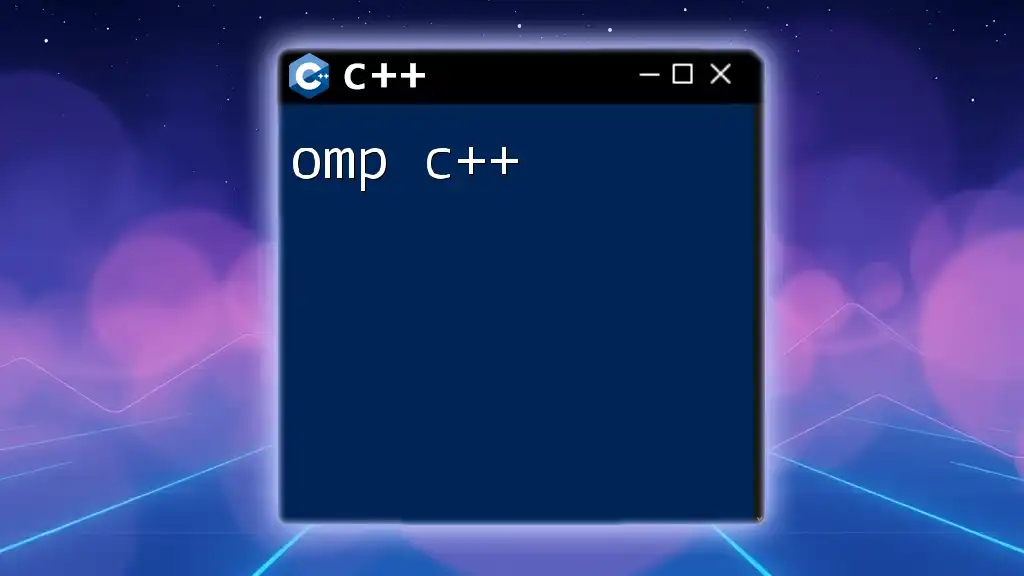
Conclusion
In this guide, we explored the multiple facets of loops in C++. Understanding the purpose and application of different loop constructs allows you to write more efficient and effective code. Loops are essential in any programmer's toolkit; their flexibility and power enable you to manage repetitive tasks effortlessly.
Additional Resources
For further reading, consider diving into online documentation and tutorials that expand on these concepts. Tools like online compilers, such as repl.it or Ideone, can offer opportunities to practice and test your loop constructs in real-time.
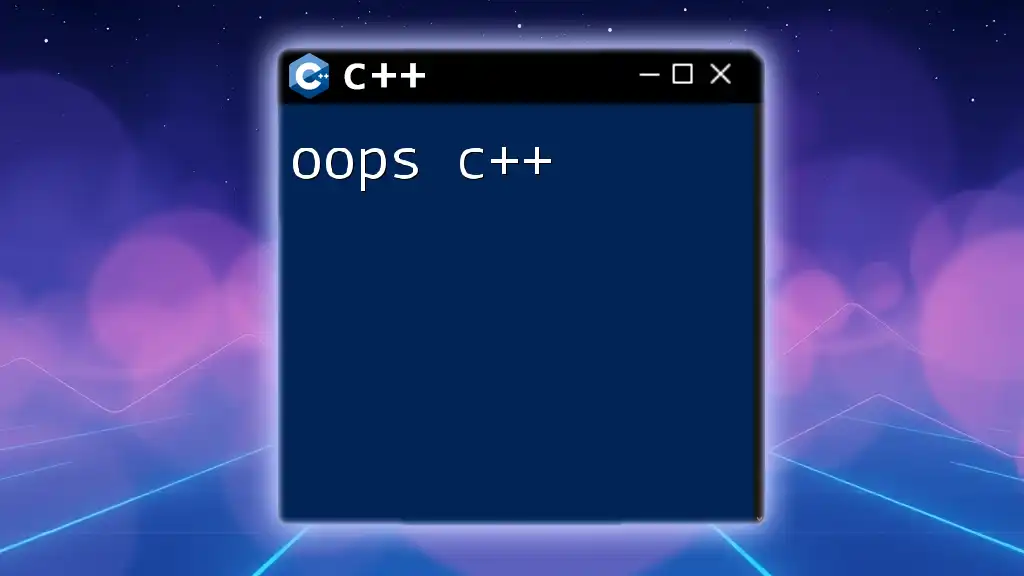
Call to Action
Now that you have a solid understanding of loop c++, try implementing these concepts in your projects. Experiment with loops in various scenarios, and feel free to share your experiences or questions!