In C++, the `auto` keyword can be used in a for loop to automatically deduce the type of the loop variable, making the code cleaner and easier to read.
Here’s an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding For Loops in C++
What is a For Loop?
A for loop is a control flow statement for specifying iteration, allowing code to be executed repeatedly. The structure and simplicity of for loops make them one of the most commonly used types of loops in C++ programming. The goal of a for loop is to perform a block of code multiple times until a specific condition is met. A typical use case might include iterating through an array or a collection.
Basic Syntax of a For Loop
The syntax of a basic for loop is as follows:
for (initialization; condition; increment) {
// Code to execute
}
- Initialization is where you declare and set your loop variable.
- Condition is a boolean expression checked before every iteration. If it evaluates to true, the loop continues; otherwise, it ends.
- Increment is the expression that modifies the loop variable after each iteration.
Understanding this syntax is essential to implementing loops effectively.
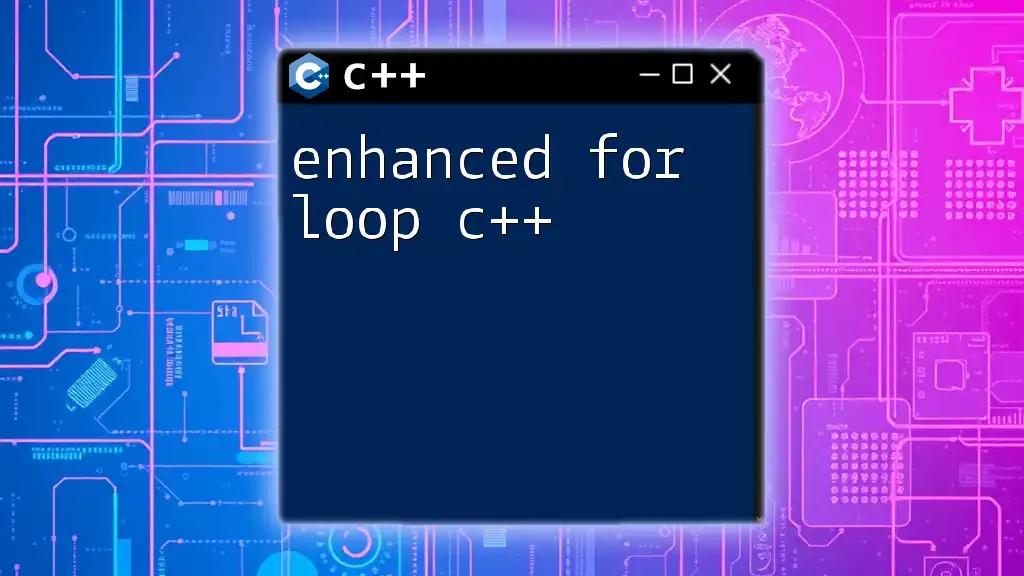
Introduction to the `auto` Keyword
What is the `auto` Keyword?
In C++, the `auto` keyword is a powerful feature that allows the compiler to automatically deduce the type of a variable. By using `auto`, programmers can avoid the verbosity of explicitly declaring variable types, thus simplifying code and minimizing the chances of errors in type definitions.
Why Use `auto` in For Loops?
Using `auto` in for loops streamlines code and enhances readability. This is especially beneficial when dealing with complex data types or in cases where the type is evident from the context. Additionally, using auto can help reduce the amount of code you write, as demonstrated below:
Instead of declaring a type explicitly:
std::vector<int>::iterator it = vec.begin();
You can use:
auto it = vec.begin();
This not only makes the code cleaner but also reduces the likelihood of type mismatches.
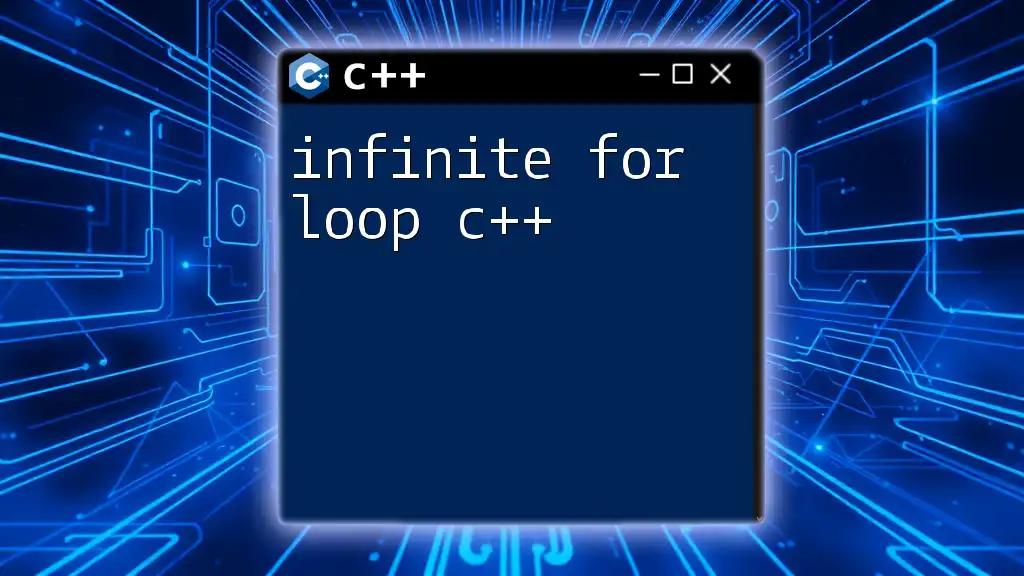
Using `auto` in For Loops
Basic Example of `auto` in a For Loop
The most straightforward application of `auto` in a for loop is allowing for seamless iteration over elements in a collection. Here’s an example of a simple for loop using `auto`:
std::vector<int> vec = {1, 2, 3, 4, 5};
for (auto i : vec) {
std::cout << i << " ";
}
In this code, auto enables the compiler to deduce that the type of `i` is `int`, based on the contents of the vector. This makes the code concise and easy to understand.
Iterating Through Collections with `auto`
Using `auto` with Standard Library Containers
Utilizing auto becomes particularly advantageous when working with the C++ Standard Library. Here’s an example of how to iterate through a vector of strings:
std::vector<std::string> fruits = {"apple", "banana", "cherry"};
for (auto fruit : fruits) {
std::cout << fruit << std::endl;
}
In this case, auto helps infer the type of `fruit` as `std::string`, effectively reducing redundancy while keeping the code clear.
Nested For Loops with `auto`
When iterating through multidimensional arrays or vectors, auto can simplify nested loops as well. For instance:
std::vector<std::vector<int>> matrix = {{1, 2}, {3, 4}};
for (auto row : matrix) {
for (auto val : row) {
std::cout << val << " ";
}
std::cout << std::endl;
}
In this example, using auto clarifies the purpose of the variables without cluttering the code with explicit types, thereby enhancing readability.
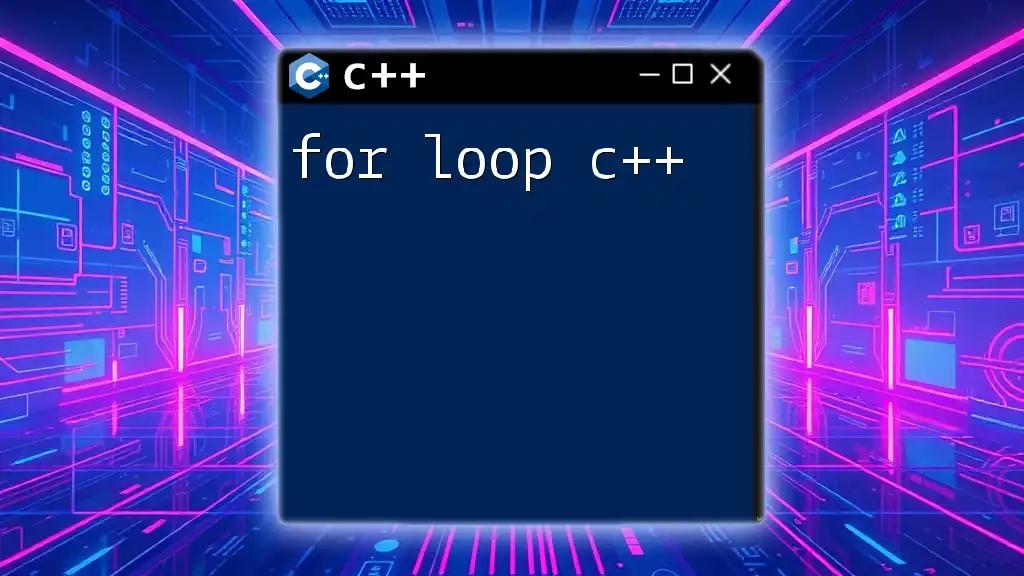
Performance Considerations
Is `auto` Slower?
A common concern among programmers is whether using `auto` affects performance. In fact, auto does not introduce performance overhead. The auto keyword causes the type to be deduced at compile time, meaning there is no runtime penalty associated with type inference. The generated code is typically just as efficient as explicitly typing out the variable declaration.
When Not to Use `auto`
However, there are situations where using `auto` may lead to confusion or reduce code clarity. For instance, in complex expressions, it might not be immediately apparent what type is being assigned to a variable. In such instances, an explicit type declaration can enhance readability and maintainability.

Best Practices for Using `auto`
Understanding when to use auto can significantly improve your coding efficiency:
- Use auto when the type is obvious from the context, such as iterating through collections or using `std::make_shared`.
- Avoid auto in scenarios where the type is not evident, as this can lead to confusion.
- Use `auto&` when you want to avoid unnecessary copies, particularly with large data structures.
By following these best practices, you can balance the benefits of code brevity with the need for clarity.

Conclusion
Using auto in for loop C++ adds significant advantages in terms of simplicity and readability. Embracing this keyword not only makes your code cleaner but also fosters a better understanding of C++ syntax and structure. So, as you advance in your journey with C++, consider integrating auto into your programming toolkit for more efficient coding.
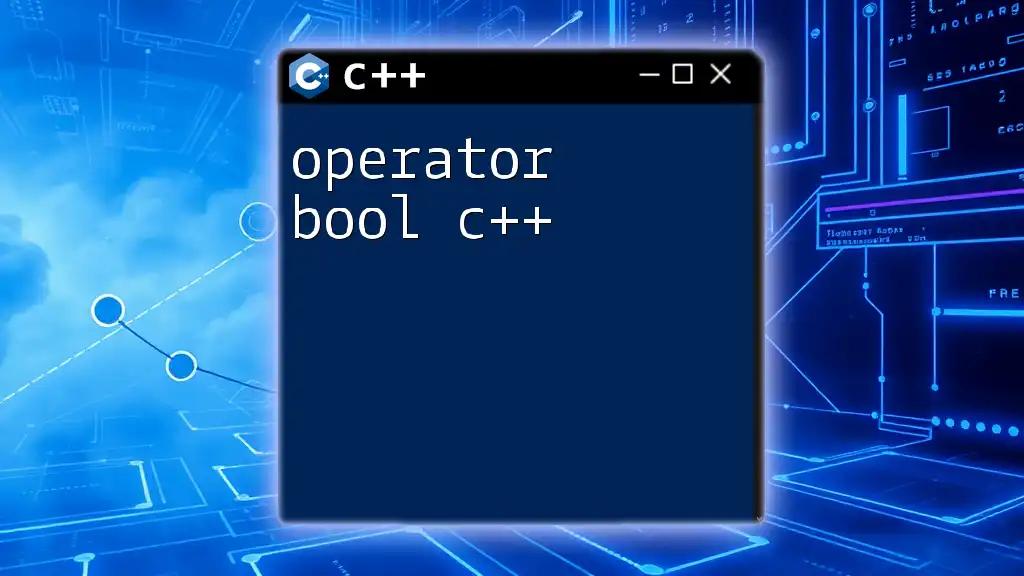
Additional Resources
For further readings, consider exploring advanced C++ features and best practices around loops and type inference. Engaging with community tutorials and shared code can greatly enhance your understanding of these crucial programming concepts.
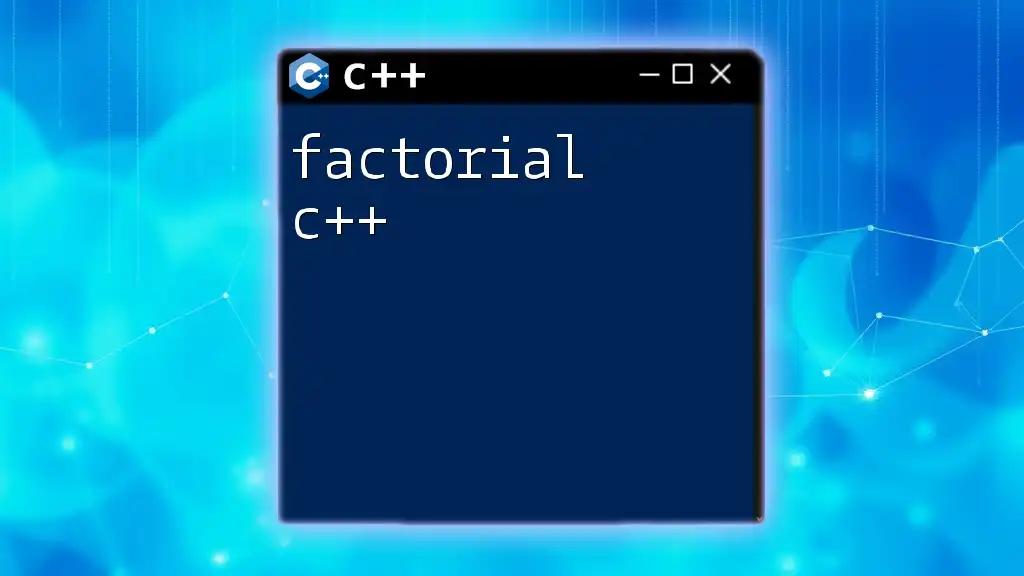
Call to Action
Feel free to leave your questions or share your own experiences with using auto in for loop in C++. And don't forget to sign up for more tutorials and guides on mastering C++ programming!