The factorial of a non-negative integer is the product of all positive integers up to that number, and can be computed in C++ using a simple recursive function as shown below:
#include <iostream>
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
int main() {
int number = 5;
std::cout << "Factorial of " << number << " is " << factorial(number) << std::endl;
return 0;
}
Understanding Factorial
Definition of Factorial
A factorial, denoted as n!, is a mathematical operation that multiplies a whole number \( n \) by all the positive integers below it. Mathematically, the factorial can be expressed using the following formula:
\[ n! = n \times (n-1) \times (n-2) \times ... \times 1 \]
For example, the factorial of 5 (5!) can be calculated as:
\[ 5! = 5 \times 4 \times 3 \times 2 \times 1 = 120 \]
Factorials are essential in various fields, particularly in combinatorics, where they are used to calculate permutations and combinations.
Visual Representation of Factorial
To visualize factorial calculations, you can create a simple list. For example:
- 4!:
- \( 4 \)
- \( 4 \times 3 \)
- \( 4 \times 3 \times 2 \)
- \( 4 \times 3 \times 2 \times 1 = 24 \)
This visualization helps in understanding how multiplication accumulates as you move down the number line.
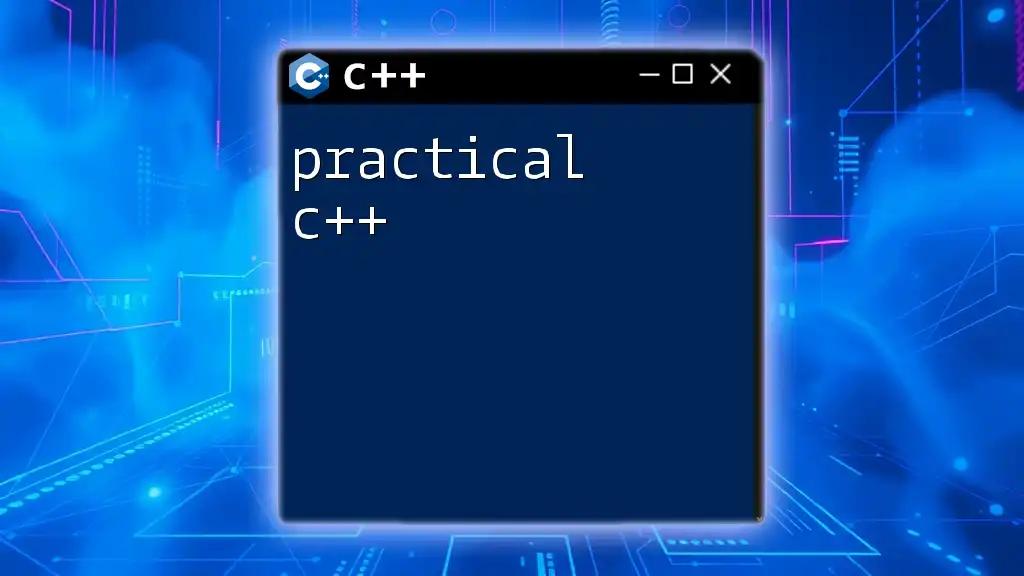
Factorial Function in C++
Understanding Functions in C++
In C++, a function is a self-contained block of code that performs a specific task. Using functions makes code modular, reusable, and easier to debug. Creating a factorial function allows you to encapsulate the logic necessary to calculate factorial values.
Creating a Factorial Function in C++
To handle factorial calculations, you need to define a function in C++. Below is the syntax for a simple factorial function:
- Define the function with a return type of `int`.
- Parameterize it to accept an integer \( n \).
- Handle the condition where \( n \) is less than or equal to 1.
Here is a code snippet for a basic factorial function:
int factorial(int n) {
if (n <= 1)
return 1;
return n * factorial(n - 1);
}
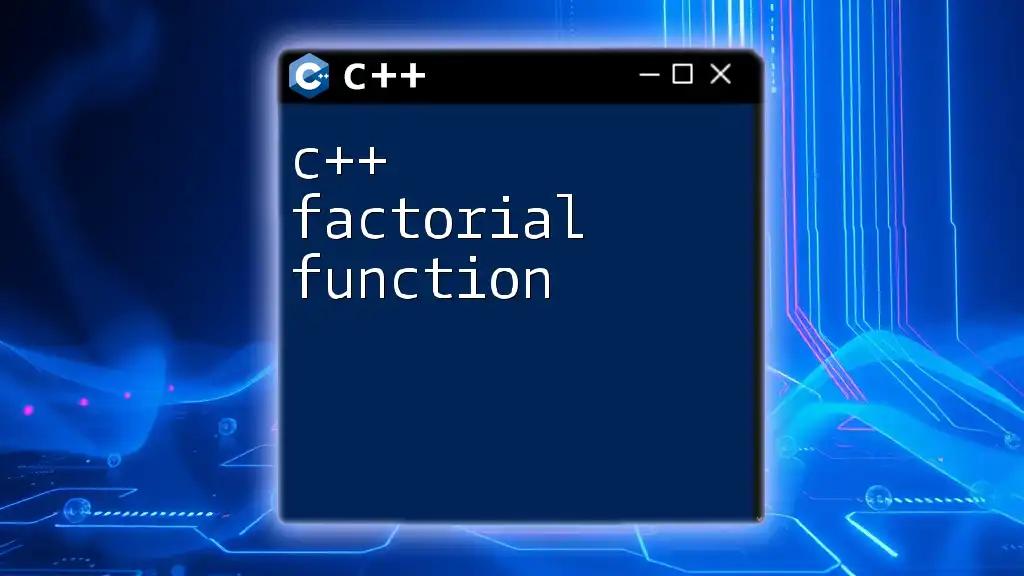
Iterative vs Recursive Approach
The Recursive Method
Recursion is a technique where a function calls itself to solve smaller subproblems. In the case of a factorial function, the largest number can be broken down until it reaches the base case (where \( n \) is 1). The recursive method can be succinctly implemented, but care must be taken with the depth of recursion, considering that it might lead to a stack overflow if the input is too large.
Here is an example of the recursive approach for calculating factorial:
int factorialRecursive(int n) {
if (n < 0)
throw invalid_argument("Negative numbers do not have a factorial.");
if (n <= 1)
return 1;
return n * factorialRecursive(n - 1);
}
The Iterative Method
Unlike recursion, the iterative approach uses loops to perform repetition. This method is generally more efficient in terms of memory as it avoids the overhead associated with multiple function calls.
Here is how an iterative factorial function looks:
int factorialIterative(int n) {
if (n < 0)
throw invalid_argument("Negative numbers do not have a factorial.");
int result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
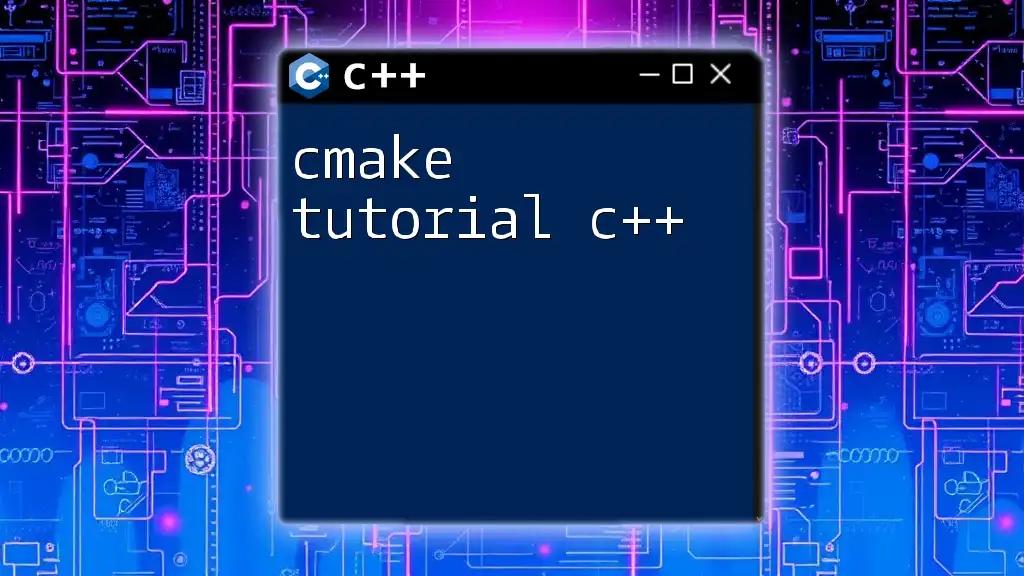
Comparing Both Approaches
Performance Analysis
When comparing the recursive and iterative methods, it’s crucial to evaluate both time and space complexity:
-
Time Complexity:
- Recursive functions generally run in \( O(n) \) time.
- Iterative functions also run in \( O(n) \) time but may execute faster due to less overhead.
-
Space Complexity:
- Recursive approach utilizes \( O(n) \) space due to the call stack.
- Iterative approach uses \( O(1) \) space since it maintains only a single state variable.
In practice, the iterative approach is often preferred for larger values of \( n \) due to its efficiency and the risk of stack overflow with deep recursion.
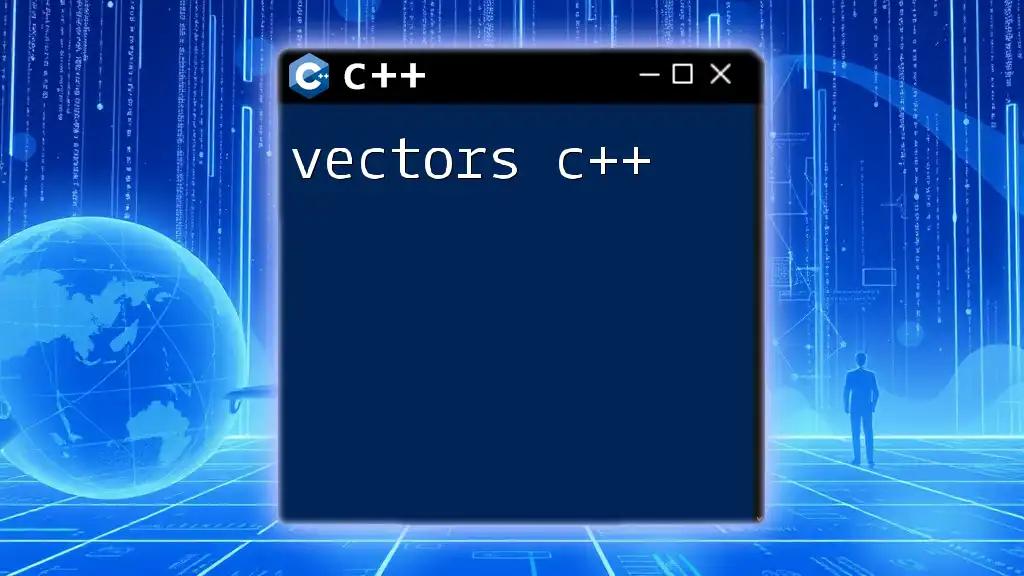
Handling Edge Cases in C++
Input Validation
When designing a factorial function, the first step is to ensure input validation. It is crucial to check that the function does not receive negative numbers; otherwise, it could lead to incorrect calculations. Throwing an exception is a standard method to handle such scenarios.
For example, you can add this validation in your functions:
if (n < 0)
throw invalid_argument("Input must be a non-negative integer.");
Large Numbers and Factorial Overflow
In C++, standard integer types can only represent numbers up to a specific limit, leading to overflow errors for relatively small values of \( n \) (e.g., \( 20! \) exceeds the maximum value of a `long long`). To handle large factorial calculations, consider:
- Using a `long long` data type to increase the range of values.
- Employing libraries like GMP (GNU Multiple Precision Arithmetic Library) for arbitrary-precision arithmetic.
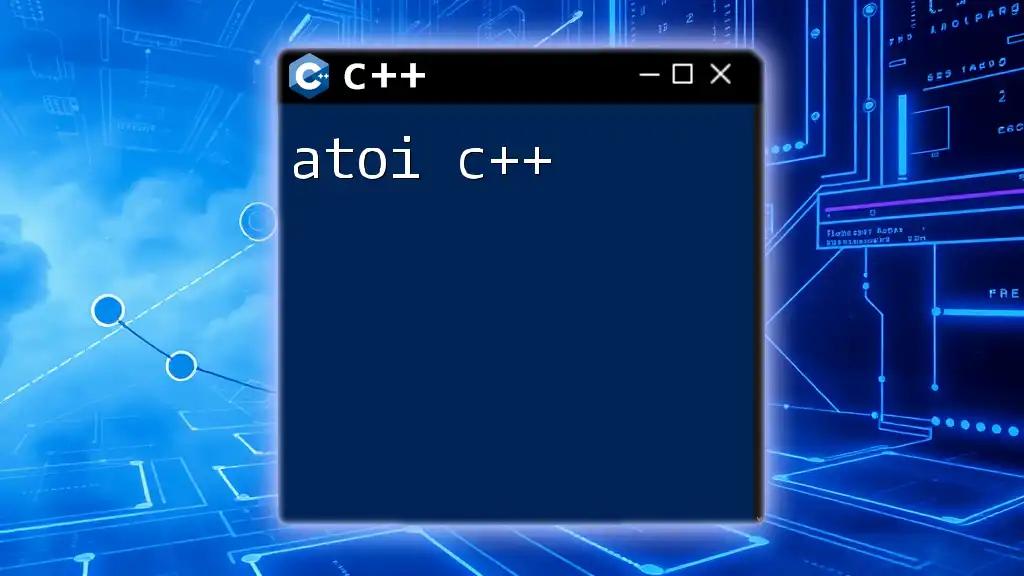
Practical Applications of Factorials
Real-World Examples
Factorials find applications across various disciplines. They are extensively used in:
- Combinatorics: Calculating permutations and combinations, where the number of ways to arrange or select items is critical. For example, the number of ways to choose \( r \) items from \( n \) items can be calculated using the formula:
\[ C(n, r) = \frac{n!}{r!(n-r)!} \]
- Probability Theory: In calculating probabilities involving combinations of events, the factorial serves as a foundation for understanding arrangements.
Code Example Demonstrating Use of Factorial in Combinatorial Calculations
To combine our factorial function with combinatorial applications, a code snippet might look as follows:
int combinations(int n, int r) {
if (r > n) return 0;
return factorial(n) / (factorial(r) * factorial(n - r));
}
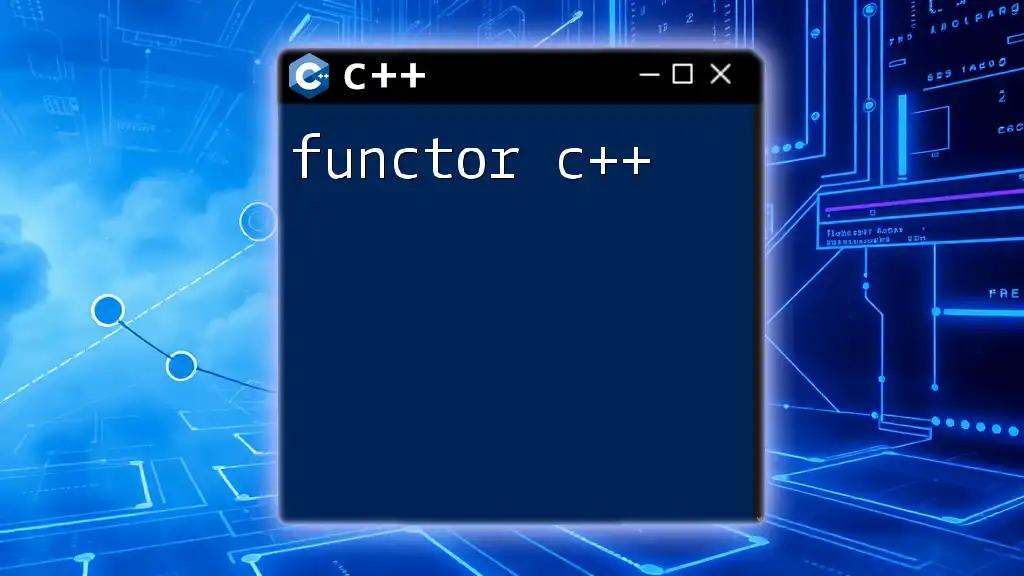
Conclusion
Understanding how to efficiently compute factorial values in C++ through both recursive and iterative techniques is fundamental for any programmer. Mastering these concepts not only enhances problem-solving skills but also lays a strong foundation for tackling complex algorithms in computer science. We encourage you to practice writing these functions and explore the wide range of applications for the factorial in programming and mathematics. Dive deeper into functions and other essential C++ concepts for a more robust programming skill set!
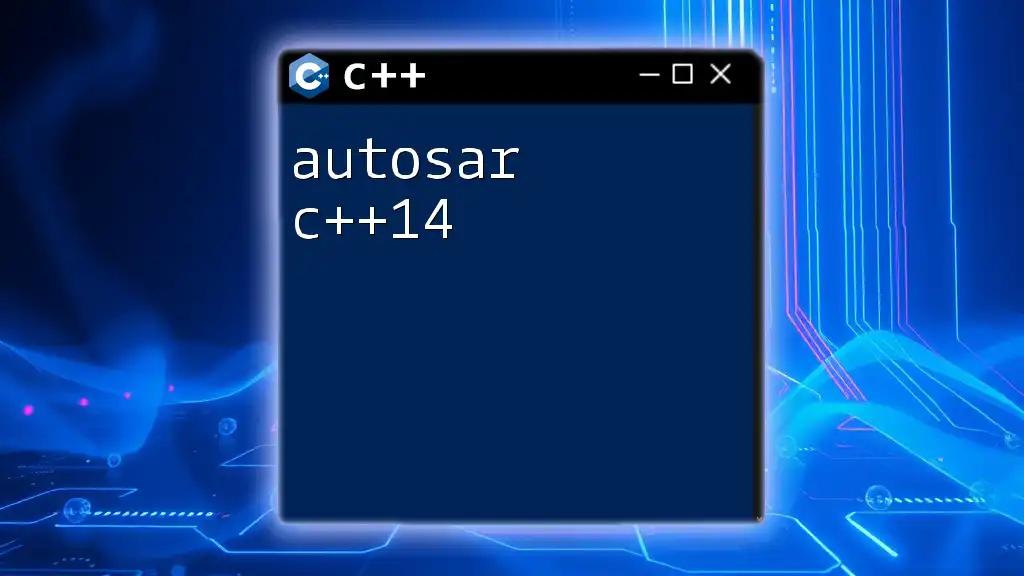
Additional Resources
For further reading and a deeper understanding, refer to reputable C++ documentation such as the C++ Standard Library or tutorials on advanced mathematical computations in C++. Engaging with these resources can greatly enhance your ability to leverage the C++ programming language effectively.