CMake is a powerful cross-platform tool that simplifies the build process of C++ projects by using configuration files to generate native makefiles or IDE project files.
Here's a simple example of a CMakeLists.txt file:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
set(CMAKE_CXX_STANDARD 11)
add_executable(MyExecutable main.cpp)
What is CMake?
CMake is an open-source, cross-platform tool designed to manage the build process of software projects using a compiler-independent approach. It generates build files for native build systems like Make, Ninja, or Visual Studio. This flexibility allows developers to write their C++ code in one place while seamlessly compiling and linking it across different platforms.
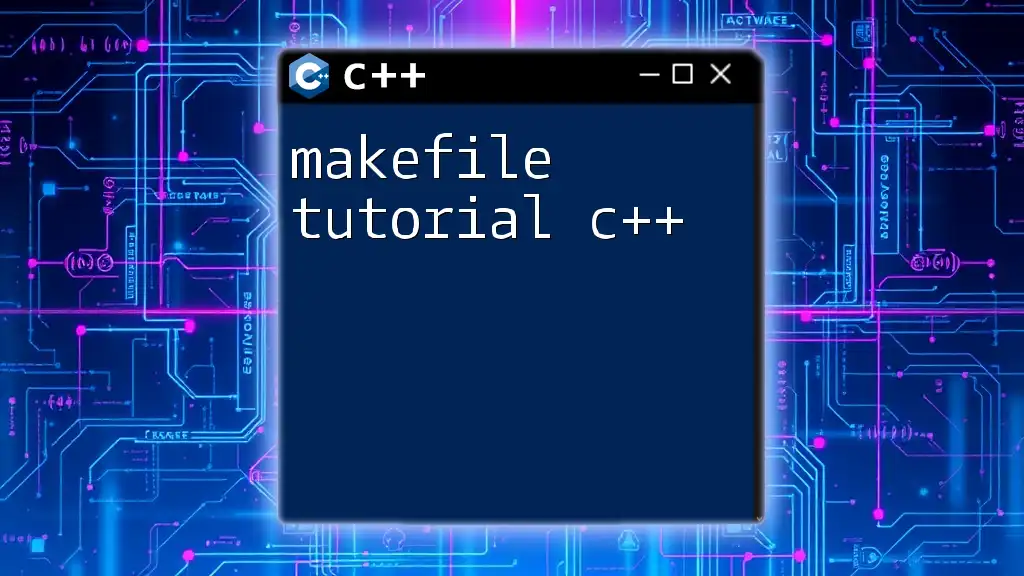
Why Use CMake for C++ Projects?
Using CMake streamlines the development process significantly. It allows for easier configuration and simplifies project management, especially when dealing with complex projects that involve multiple files and directories. Additionally, CMake provides essential capabilities for integrating external libraries and building on various operating systems without needing to change your codebase.
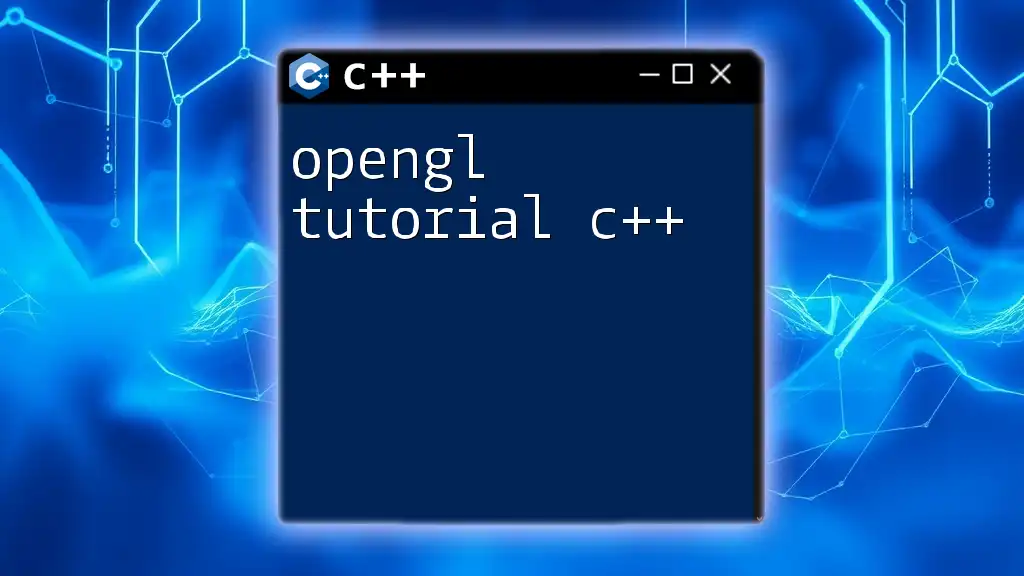
Installing CMake
Before you get started with this CMake tutorial for C++, you need to install CMake on your machine. Here’s how to do it on various platforms:
- Windows: Download the installer from the official CMake website and follow the instructions.
- macOS: Use Homebrew to install CMake:
brew install cmake
- Linux: Most Linux distributions have CMake available in their package management systems. Use:
sudo apt-get install cmake
Make sure you also have a C++ compiler installed on your system (e.g., `g++` for Linux, `clang` for macOS, or MSVC on Windows).
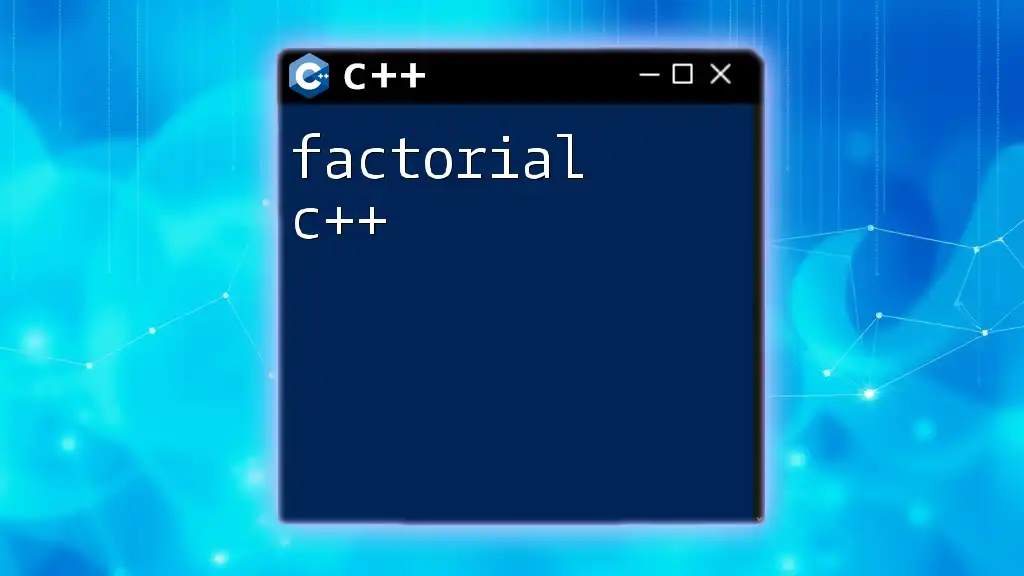
Basic CMake Commands
Familiarizing yourself with essential CMake commands is crucial for a streamlined workflow. Here are some of the primary commands you will utilize:
- `cmake`: This command is used to generate the build system.
- `cmake-build`: Use this command to build your project.
- `cmake-gui`: For those who prefer a graphical interface, CMake provides this GUI for configuring projects visually.
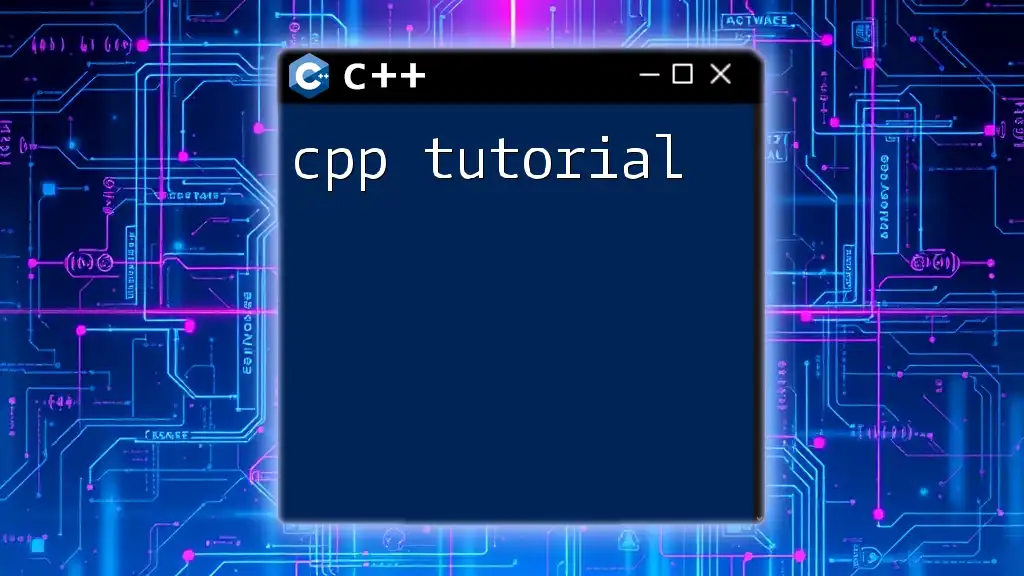
Creating Your First CMake Project
Creating the Project Structure
Start by creating a directory for your project. A well-organized directory structure is vital for maintainability. Here’s a recommended layout:
MyProject/
├── CMakeLists.txt
├── src/
│ └── main.cpp
└── include/
Writing Your First CMakeLists.txt
Inside your project directory, create a file named CMakeLists.txt. This file serves as the blueprint for your build configuration. Here’s a basic structure you can follow:
cmake_minimum_required(VERSION 3.10)
project(HelloWorld)
add_executable(hello src/main.cpp)
- `cmake_minimum_required` specifies the minimum version of CMake required.
- `project` defines the name of your project.
- `add_executable` indicates which source file should be compiled into an executable.
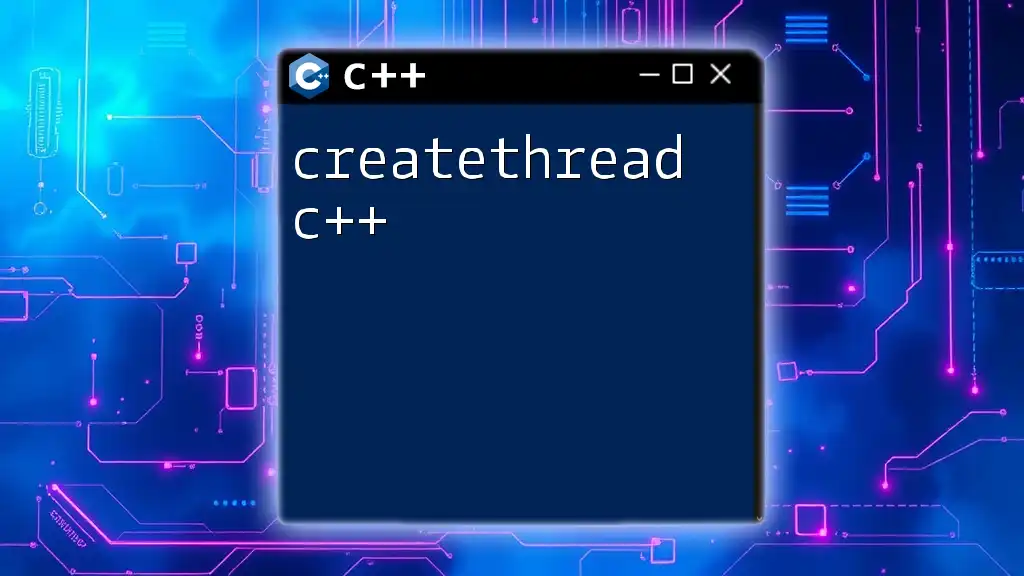
Configuring CMake
Defining Variables
Using variables helps you manage your project more efficiently. In your CMakeLists.txt, you can define variables like this:
set(SOURCES src/main.cpp)
add_executable(hello ${SOURCES})
This approach not only simplifies your commands but makes updates to your project easier.
Setting Compiler Options
Choosing the correct compiler options is essential for the development process. You can specify compiler flags and C++ standards in CMakeLists.txt:
set(CMAKE_CXX_STANDARD 17)
set(CMAKE_CXX_STANDARD_REQUIRED True)
Setting the C++ standard to the latest version ensures compatibility with modern language features.
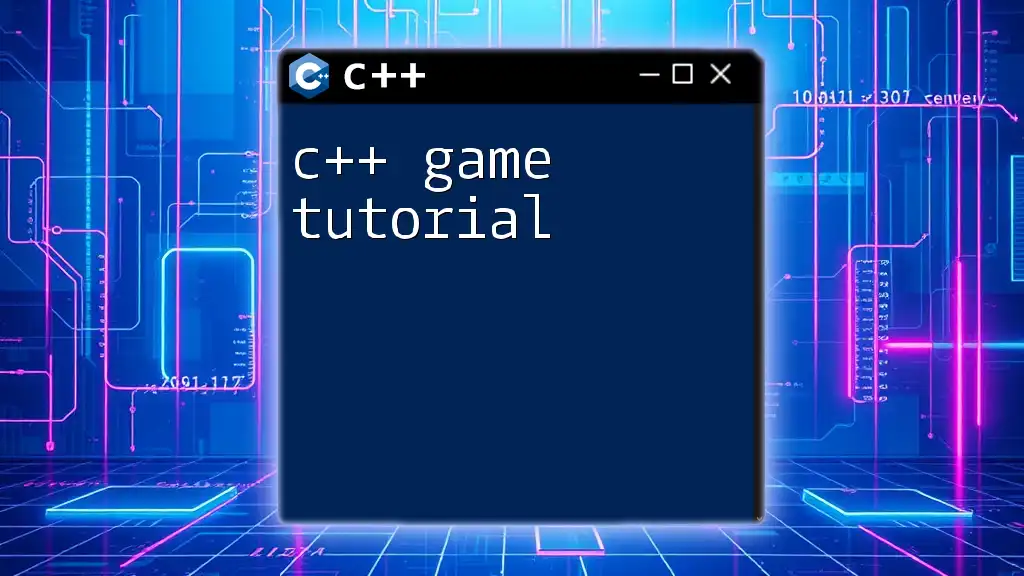
Adding Libraries to Your Project
Linking External Libraries
To enhance your project capabilities, you’ll often need to link external libraries. For example, if you want to use OpenCV, you can find and link it like this:
find_package(OpenCV REQUIRED)
target_link_libraries(hello ${OpenCV_LIBS})
Creating Static and Shared Libraries
Understanding the difference between static and shared libraries is vital when developing larger projects. You can create a static library as follows:
add_library(mylib STATIC src/mylib.cpp)
target_include_directories(mylib PUBLIC include)
This example makes it straightforward to encapsulate functionality in reusable libraries.
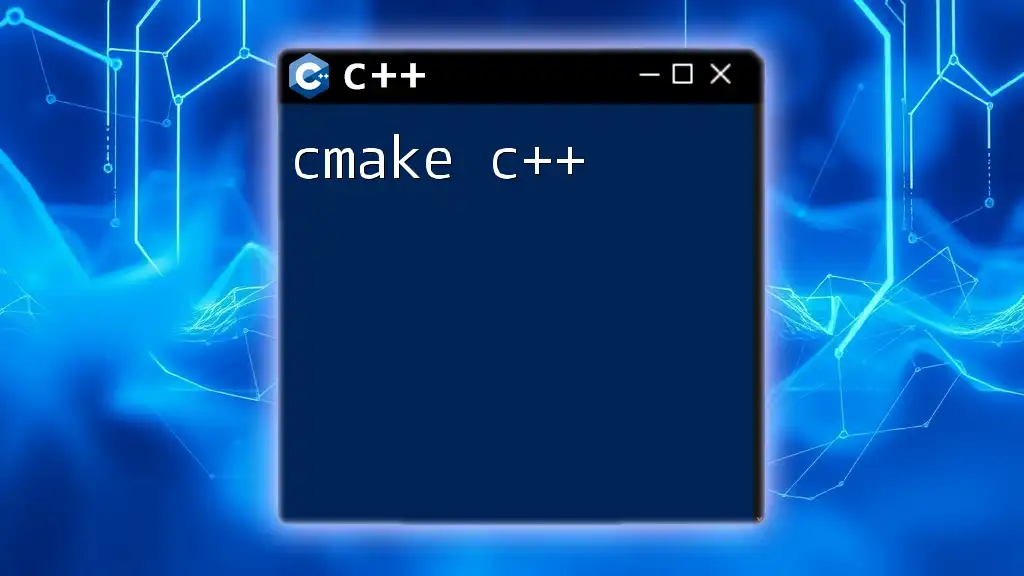
Advanced CMake Features
CMake Functions and Macros
As your project grows, writing reusable code becomes more important. CMake allows you to create functions and macros, which can help you in this regard. Here’s a simple function:
function(my_function arg)
message("${arg} is passed to the function")
endfunction()
Using CMake with Multiple Targets
If your project contains multiple executables or libraries, you can configure each one separately in the same CMakeLists.txt file. This scalability is one of CMake's key advantages.
CMake Testing with CTest
Incorporating tests into your development workflow can be a decisive factor in maintaining code quality. CMake integrates well with CTest, a testing tool that comes with it. To enable testing, add:
enable_testing()
add_test(NAME MyTest COMMAND hello)
This will allow you to run tests easily and automate quality checks.
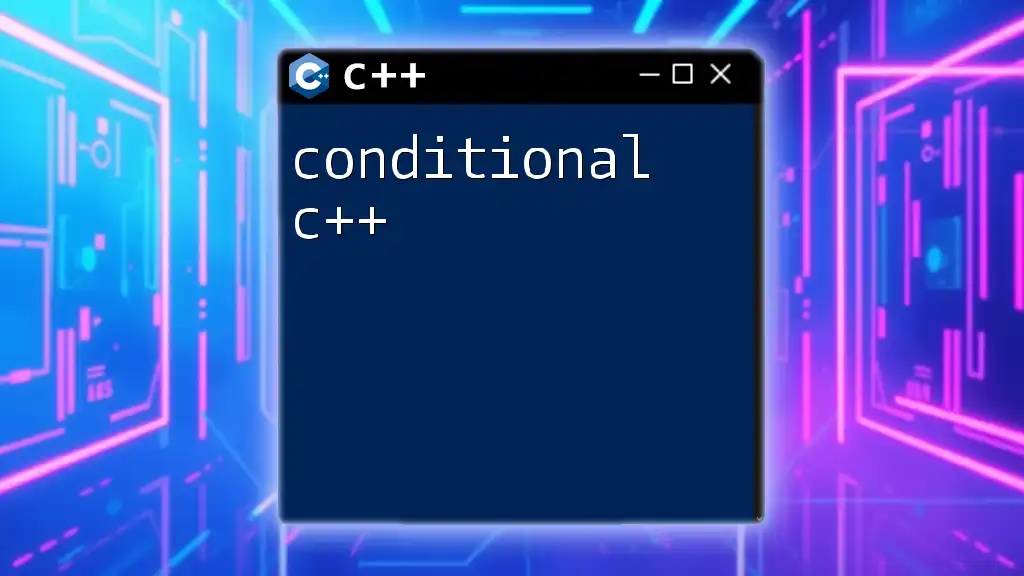
Building Your Project
Running CMake to Generate Build Files
To build your project using CMake, you need to run the configuration first. Open your terminal and execute the following commands:
mkdir build
cd build
cmake ..
This creates a build directory, navigates into it, and prepares the project for compilation.
Building the Project
After generating the necessary build files, you can compile your project. Depending on your setup, use commands like:
make # On Unix-like systems
or
cmake --build . # Cross-platform command
This will create the executable based on your specified source files.
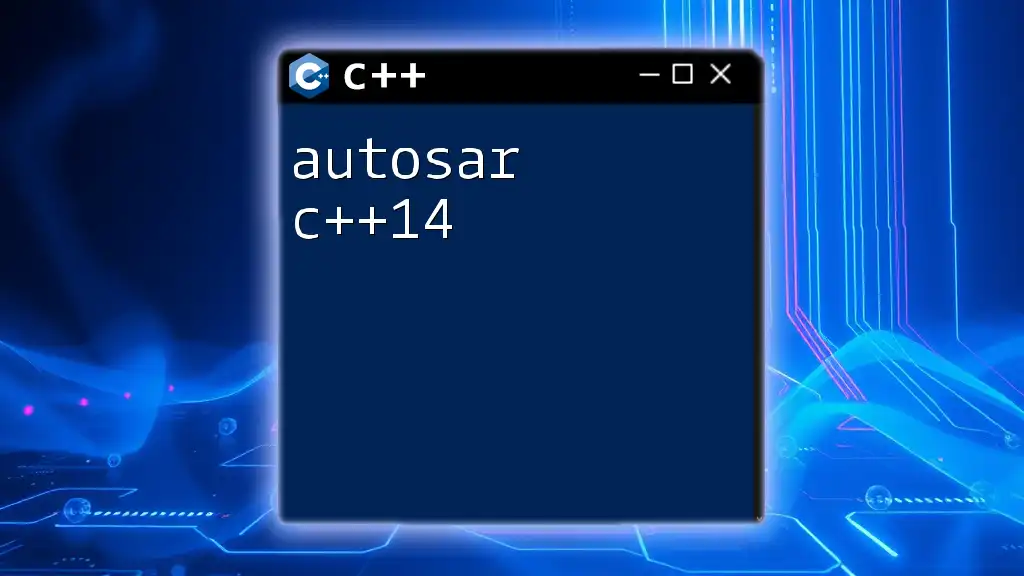
Conclusion
In summary, this CMake tutorial for C++ has equipped you with the foundational knowledge to get started with CMake, from installation to building and linking libraries. You now have the tools to manage your C++ projects more effectively, paving the way for seamless cross-platform development.
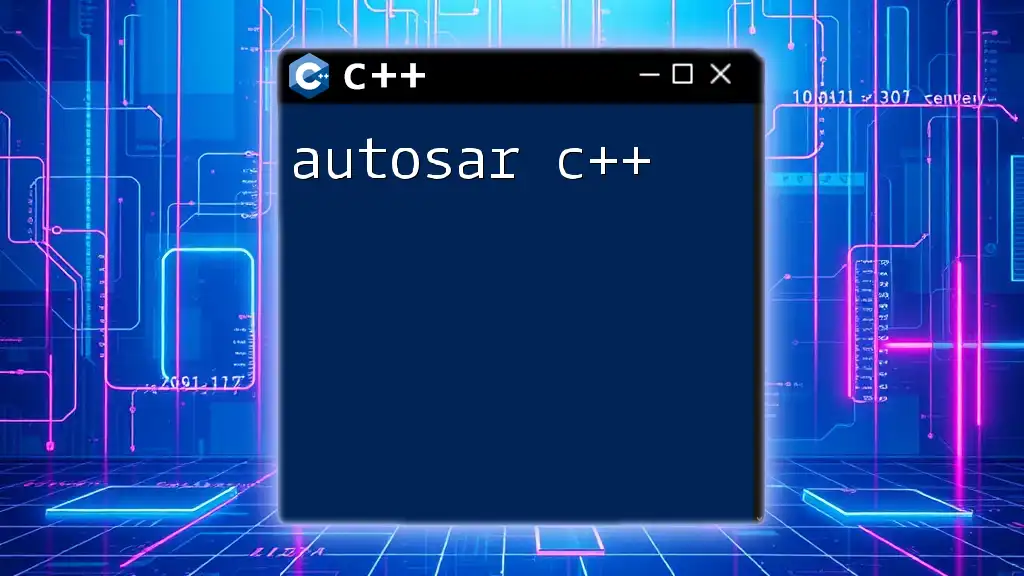
Frequently Asked Questions
Common CMake Issues and Resolutions
One of the common pitfalls in using CMake includes configuration errors. Ensure your CMakeLists.txt files are free of syntax errors and that libraries are correctly linked to avoid frustration.
Tips for Effective CMake Usage
To maximize the effectiveness of CMake, maintain clean and well-structured CMakeLists.txt files. Keep your variables organized and use comments to clarify complex configurations. Embrace modular design principles and watch your project improve in maintainability and scalability.