In C++, the `make_pair` function creates a pair of two values, simplifying the process of storing related data in a single object.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <utility> // for std::make_pair
int main() {
auto myPair = std::make_pair(10, "Hello");
std::cout << "First: " << myPair.first << ", Second: " << myPair.second << std::endl;
return 0;
}
What is `make_pair`?
`make_pair` is a function in C++ that simplifies the creation of a `std::pair` object, which is a simple container for storing two heterogeneous objects as a single unit. This feature is particularly important because it enhances type safety and allows developers to group related data without the need for defining a custom class.
Brief Overview of Pairs in C++
A pair is a data structure consisting of two data items or objects, known as the first and second members. Unlike tuples or arrays, which can store multiple items of the same type, pairs allow for heterogeneous data types, meaning you can store different types in the same structure. Pairs are commonly used in C++ to represent relationships or key-value pairs, making them invaluable for various programming tasks.

How to Use `make_pair` in C++
Syntax of `make_pair`
The syntax for `make_pair` is straightforward and follows this general structure:
std::pair<Type1, Type2> make_pair(const Type1& first, const Type2& second);
This allows the compiler to deduce the types for the `std::pair` automatically based on the arguments provided.
Creating Simple Pairs
One of the simplest uses of `make_pair` is to create a pair of two related values. Here’s a basic example:
#include <iostream>
#include <utility> // For std::make_pair
int main() {
auto myPair = std::make_pair(10, "ten");
std::cout << "First: " << myPair.first << ", Second: " << myPair.second << std::endl;
return 0;
}
In this example, we create a pair with an integer and a string. The `first` member holds the integer value, while the `second` member holds the string, demonstrating the utility of `make_pair` for grouping different data types together.
Using `make_pair` with Different Data Types
`make_pair` can handle a wide variety of data types seamlessly. Here’s another example showcasing its versatility:
#include <iostream>
#include <utility>
int main() {
auto pair1 = std::make_pair(42, 3.14);
auto pair2 = std::make_pair("Hello", false);
std::cout << pair1.first << ", " << pair1.second << std::endl;
std::cout << pair2.first << ", " << pair2.second << std::endl;
return 0;
}
In this snippet, `pair1` combines an integer with a double, while `pair2` combines a string with a boolean. The type inference provided by using `auto` allows for simpler code with less boilerplate.

Real-World Applications of `make_pair`
Use Case: Storing Key-Value Pairs
One common application of `make_pair` is in associative structures like maps. Here, it helps simplify the insertion of key-value pairs. Consider the following example:
#include <iostream>
#include <map>
#include <utility>
int main() {
std::map<int, std::string> myMap;
myMap.insert(std::make_pair(1, "One"));
myMap.insert(std::make_pair(2, "Two"));
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
In this code snippet, we create a map to associate integers with strings. By using `make_pair`, we can easily insert these relationships without manually creating a `std::pair` object.
Use Case: Function Return Types
Another powerful feature of `make_pair` is its ability to return multiple values from a function. This can be done with ease, improving code clarity. Here’s an example:
#include <iostream>
#include <utility>
std::pair<int, double> compute() {
return std::make_pair(5, 3.14);
}
int main() {
auto result = compute();
std::cout << "Integer: " << result.first << ", Double: " << result.second << std::endl;
return 0;
}
In this function, we return a pair consisting of an integer and a double. This approach allows for efficient data retrieval by encapsulating related values together, significantly enhancing modular programming.
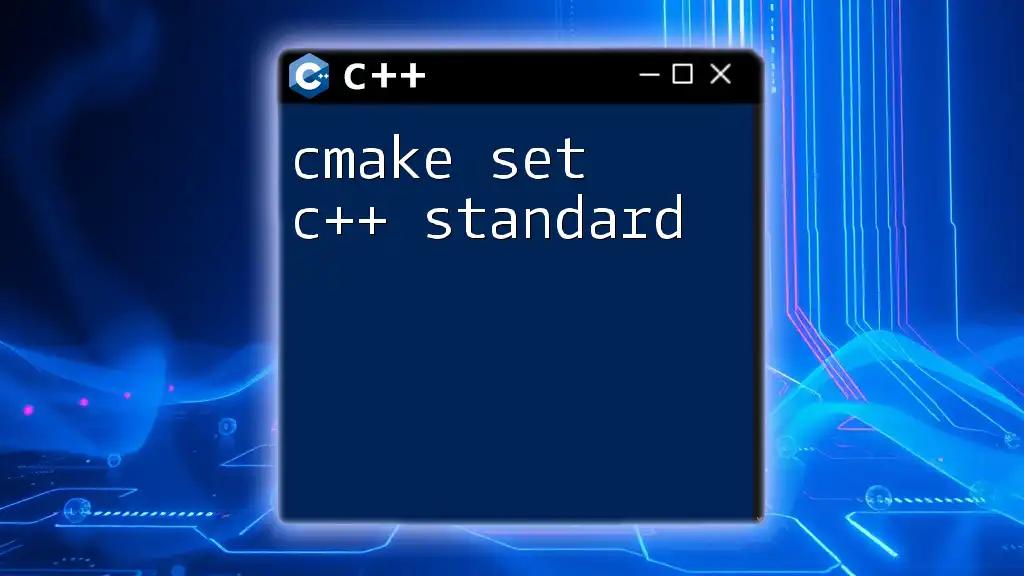
Best Practices When Using `make_pair`
Using `auto` Keyword
One of the best practices when working with `make_pair` is to utilize the `auto` keyword. This makes your code cleaner and more maintainable by reducing redundancy and potential type mismatches. For example:
auto myIntStringPair = std::make_pair(1, "One");
Not only does this save you from explicitly specifying types, but it also reduces the chance of errors stemming from type mismatches.
Avoiding Common Pitfalls
Despite its convenience, certain pitfalls can be encountered when using `make_pair`. One common issue involves implicit type conversions which may lead to confusion. For instance, when different data types can be implicitly converted, it might result in unintended consequences.
To avoid this, it’s often better to use explicit types when creating pairs, making your intentions clear and improving code readability.
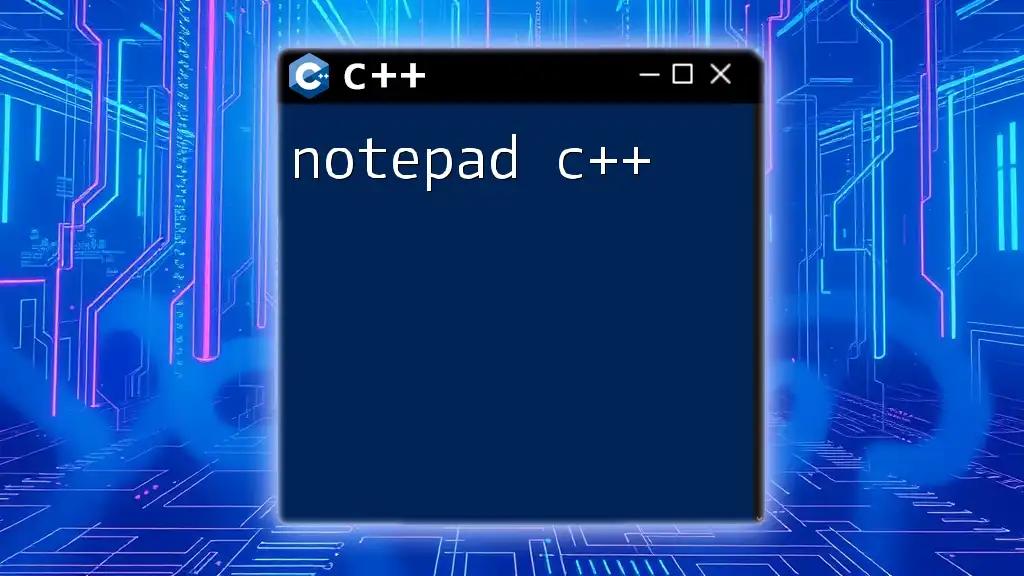
Conclusion
Recap of Key Points
In summary, `make_pair` in C++ offers a powerful and efficient way to create pairs of heterogeneous data types swiftly. Its utility in facilitating key-value pairs, returning multiple values, and enhancing type safety makes it an invaluable tool in every C++ programmer's toolkit.
When to Use `make_pair`
Utilizing `make_pair` is advantageous in scenarios involving data grouping, such as pairing related values or managing collections of unique data. It simplifies interactions with data structures and can improve function return types, leading to cleaner and more maintainable code.
Encouragement to Practice
For those looking to deepen their understanding and proficiency with C++, the best way to learn is by practicing. Experiment with `make_pair` in various contexts, and see how it can simplify your programming tasks while enhancing clarity in your code.
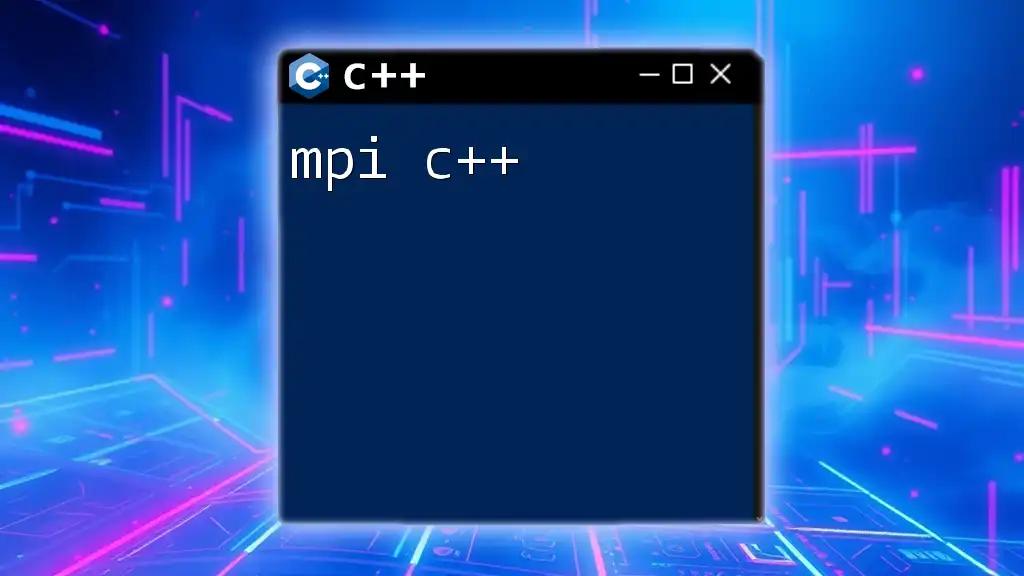
Additional Resources
References for Further Reading
- C++ Standard Library documentation for `std::pair` and `std::make_pair`.
- Tutorials on advanced C++ concepts and data structures.
Suggested Exercises
- Create a program that stores and manipulates a collection of pairs with different data types.
- Write a function that returns multiple values using `make_pair`, and utilize it in a variety of ways within your code.
Explore the utility of `make_pair` and incorporate it into your coding practices to unlock the potential of C++ programming!