Creating a simple C++ game can be as easy as implementing a text-based number guessing game, where the player has to guess a randomly generated number within a specified range.
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0)));
int secretNumber = rand() % 100 + 1;
int guess = 0;
std::cout << "Guess the number (between 1 and 100): ";
while (guess != secretNumber) {
std::cin >> guess;
if (guess > secretNumber)
std::cout << "Too high! Try again: ";
else if (guess < secretNumber)
std::cout << "Too low! Try again: ";
else
std::cout << "Congratulations! You guessed it!" << std::endl;
}
return 0;
}
Getting Started with C++
Setting Up Your Development Environment
To successfully make a C++ game, you first need to set up your development environment. Here’s how to get started:
-
Recommended IDEs: Choosing an Integrated Development Environment (IDE) that suits your workflow is vital. Some popular choices include:
- Visual Studio: This is a powerful IDE that offers advanced debugging features.
- Code::Blocks: A lightweight IDE that is easy to use for beginners.
-
Installing Necessary Compilers: Download and install a C++ compiler such as GCC or use the one bundled with Visual Studio. This is essential for compiling your C++ code.
-
Project Structure: Organize your files from the beginning. A simple structure might include folders for:
- Source Files (`src`)
- Header Files (`include`)
- Assets (`assets`, for graphics and sound)
Basics of C++ for Game Development
Understanding the fundamentals of C++ is crucial. Start with:
-
Data Types and Variables: This includes integers, floats, booleans, and strings. Understanding how to use these appropriately will help manage the game state.
-
Control Structures: Familiarize yourself with if statements, loops, and switch cases. These structures will allow you to control your game flow effectively.
-
Object-Oriented Programming (OOP): Learn core OOP concepts such as:
- Classes: These are blueprints for objects; for instance, you might have a Player class that defines player behavior and attributes.
- Inheritance: This allows you to create a new class based on an existing class, enabling code reuse.
- Polymorphism: Achieve different behaviors in subclasses.

Game Design Fundamentals
Conceptualizing Your Game
Before coding, you should conceptualize your game. This involves:
-
Choosing a Game Genre: Assess what type of game you want to create. Genres include platformers, puzzle games, and shooters. Each genre comes with its own set of mechanics and design challenges.
-
Sketching the Game Concept: Visualize your game mechanics, storyline, and characters. Documentation can include:
- Basic gameplay mechanics (e.g., jumping, shooting)
- Storylines and character arcs
- Game world layout
Creating Game Assets
Assets bring your game to life. You’ll need graphics for characters and environments and sound for effects and background music. Consider:
-
Graphics Creation Tools: Software like Blender for 3D graphics and GIMP for 2D art can be significant in creating tailored assets.
-
Sourcing Assets: You can also find free or paid assets online. Sites like OpenGameArt and FreeSound can be invaluable for this.
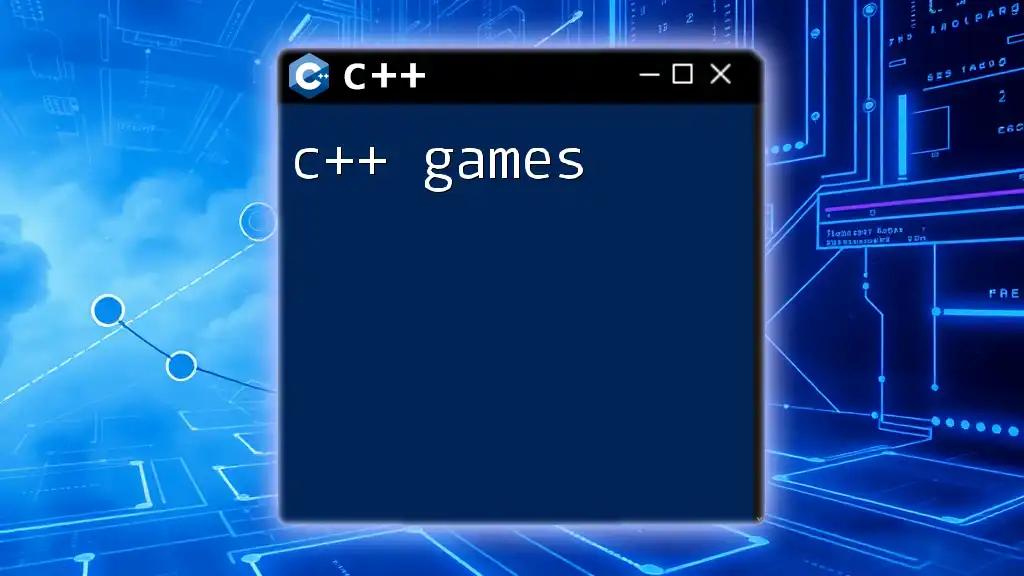
Building Your Game
Setting Up Your Game Loop
The game loop is a core architecture in game development. It governs how your game processes input, updates the game state, and renders frames. Here’s a basic structure you can use:
while (gameRunning) {
processInput();
updateGame();
render();
}
- processInput(): This function will listen for player inputs and adjustments.
- updateGame(): Update game logic, including character movements and collision checks.
- render(): The call to draw your game objects on the screen.
Implementing Game Mechanics
Collision Detection is a vital part of any game. You might want to implement bounding box collision. Here’s a simple function:
bool checkCollision(GameObject a, GameObject b) {
return (a.x < b.x + b.width &&
a.x + a.width > b.x &&
a.y < b.y + b.height &&
a.y + a.height > b.y);
}
This function helps determine if two objects are colliding based on their dimensions.
Player Controls and Movement Mechanics are also critical. Ensure you capture and process user input effectively. A basic movement example might look like this:
if (keyPressed("RIGHT")) {
player.x += player.speed;
}
Graphics and Rendering
Selecting the right graphics library is a necessity. Libraries such as SFML and SDL provide robust functionality for rendering. By utilizing SFML, you can create simple render calls like this:
window.draw(sprite);
This line of code would render your graphical sprite onto the game window.

Advanced Features
Adding Sound and Music
To enhance the player's experience, integrating sound is essential. Libraries such as FMOD or OpenAL can be used to implement sound and music:
soundBuffer.loadFromFile("sound.wav");
sound.setBuffer(soundBuffer);
sound.play();
This snippet loads a sound file and plays it, adding an auditory element to your gameplay.
Implementing AI and Enemies
For a richer gameplay experience, adding artificial intelligence (AI) for enemies can create challenges for players. You might use state machines to manage enemy behavior and transitions. Here’s a simple approach:
if (playerDetected) {
chasePlayer();
} else {
idle();
}
This code makes the enemy switch between idle and chase states based on player proximity.

Polishing Your Game
Debugging and Optimization
As you progress in making your C++ game, be prepared for debugging and optimization. Use tools like gdb for debugging and profilers to diagnose performance issues. Common techniques include:
-
Logging: Add logs to understand flow and debug issues. Use `std::cout` to print run-time information.
-
Optimization: Profile your game to identify bottlenecks, optimizing critical areas to enhance performance.
Testing Your Game
Testing is fundamental. Playtest your game, gather feedback from testers, and make necessary adjustments. Utilize beta testers to uncover issues you might not notice yourself.

Publishing Your Game
Creating an Installer
Once your game is polished, the next step is to package it. Creating an installer helps in distributing your game. Consider using tools like Inno Setup or NSIS, which streamline the process of creating executable installation files.
Marketing Your Game
Finally, promoting your finished product is essential for reaching your target audience. Consider using channels like Steam, itch.io, or even a personal website to publish your game. Engage with communities on social media and gaming forums to create buzz around your launch.
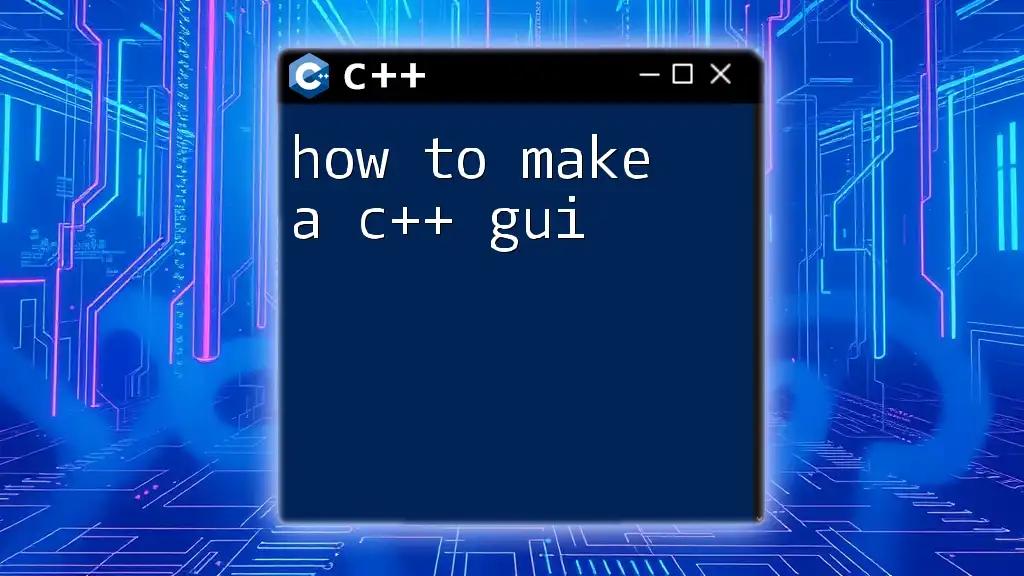
Conclusion
In this guide, we've explored what it takes to make a C++ game. From setting up your environment to conceptualizing ideas, and building and publishing your game, the journey can be challenging yet rewarding. Remember to keep learning and iterating on your game, and, most importantly, enjoy the process. There are countless resources available to support you in your ongoing education, including books, online courses, and active game development communities. Embrace your creativity, and happy coding!