To create a simple C++ GUI application using the Qt framework, follow this example to create a basic window:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.resize(200, 100);
button.show();
return app.exec();
}
Understanding GUI Basics
What is a GUI?
A Graphical User Interface (GUI) allows users to interact with software applications visually through elements like buttons, icons, and windows. Unlike a Command Line Interface (CLI), which requires text commands, a GUI is generally more user-friendly and accessible to a wider audience. It engages users by providing a more intuitive interaction model.
Why Use C++ for GUI Development?
C++ combines performance efficiency with the ability to interface at the system level, making it an excellent choice for GUI development, especially in scenarios requiring high performance, like gaming or engineering applications. Additionally, C++ has a rich set of libraries and frameworks designed specifically for creating robust GUIs, maintaining its relevance even as other languages gain popularity in the GUI space.

Choosing a GUI Framework
Overview of Popular C++ GUI Libraries
When embarking on learning how to make a C++ GUI, selecting the right framework is vital. Here are some of the most popular choices:
-
Qt
- Description: Qt is a powerful, cross-platform framework that's widely adopted for GUI applications. It provides a wide range of modules for application development.
- Key Features: Native look-and-feel, powerful graphics capabilities, and extensive documentation.
- Examples of Applications: Adobe Photoshop Elements, VirtualBox.
-
wxWidgets
- Description: This library enables developers to create applications that can compile on different platforms with a single codebase.
- Key Features: Native widgets, support for multiple platforms.
- Use Cases: Cross-platform applications with native UI.
-
FLTK (Fast, Light Toolkit)
- Description: A lightweight, cross-platform GUI toolkit that focuses on speed and simplicity.
- Advantages: Small footprint and easy to integrate into existing applications.
-
Dear ImGui
- Description: A bloat-free graphical user interface library known for its ability to create fast, real-time interfaces.
- Ideal Use Cases: Game development and applications requiring real-time data visualization.
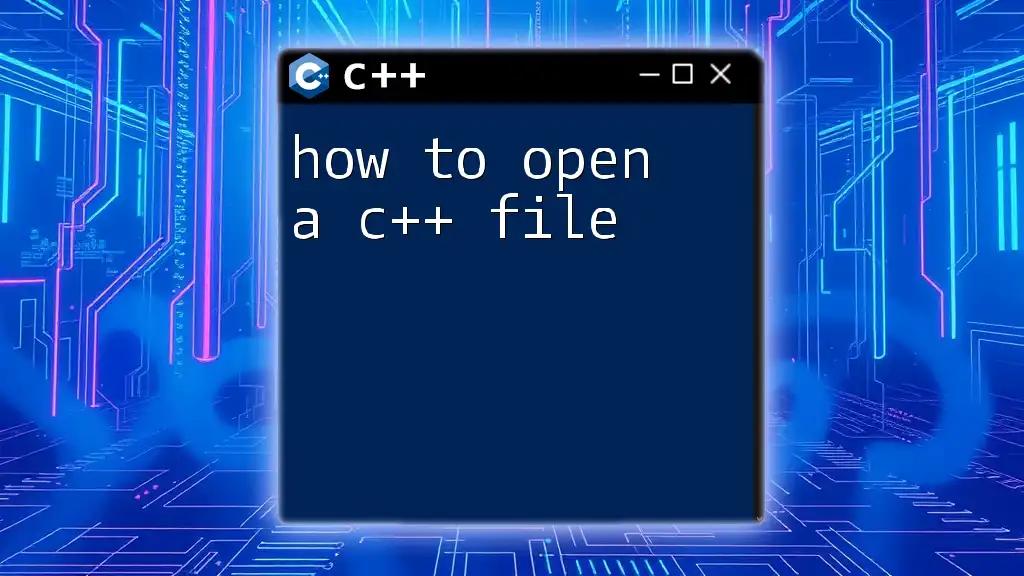
Setting Up Your Development Environment
Prerequisites
Before you dive into how to make a C++ GUI, you need to ensure you have the right tools. This includes a C++ compiler like GCC or MSVC and CMake for managing your projects.
Installing Qt
To facilitate learning C++ GUI development, you might choose Qt. Follow these steps to install it:
- Download the Qt Installer from the official site.
- Run the installer and choose the latest version of Qt, complete with Qt Creator (the integrated development environment).
- Optionally, set up your Environment Variables to include Qt binaries for easier command-line access.
Creating Your First GUI Project
Now that your environment is set up, create a project with the following structure:
- `src/`: Your source files
- `include/`: Header files
- `resources/`: Images or icons used in your application
In Qt Creator, start a new project and select "Qt Widgets Application". Follow the setup wizard to create your application.
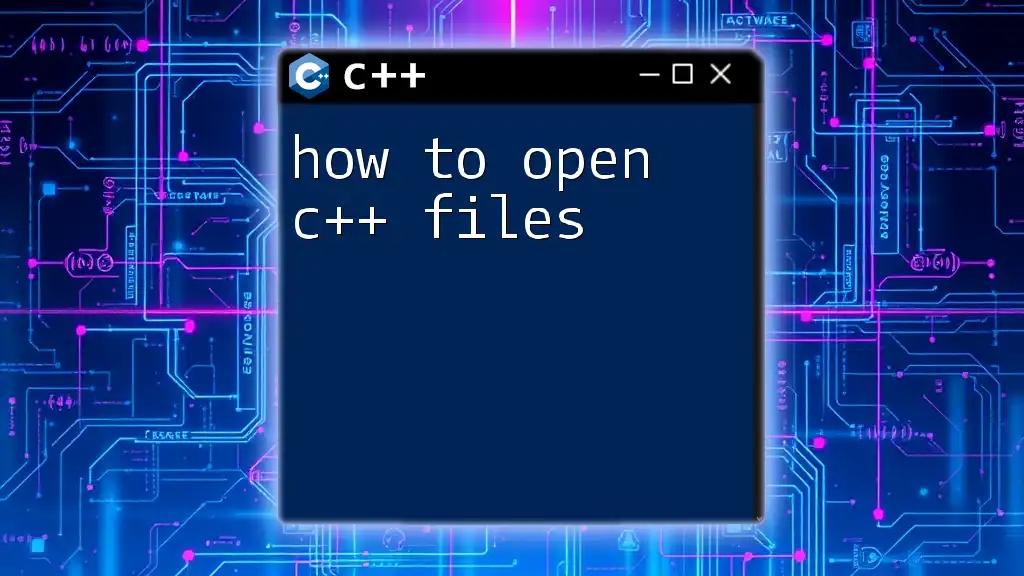
Building Your First C++ GUI
Designing the Interface
GUI design is about layout and usability. Tools like Qt Designer let you drag and drop widgets onto the interface, allowing you to visualize your application before coding it.
Adding Widgets
Widgets are the building blocks of a GUI, representing each interactive element. Common examples are buttons, labels, and text inputs.
Example: Creating a Basic Window
A great way to begin how to make a C++ GUI is by creating a basic window. Use the following code snippet:
#include <QApplication>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication a(argc, argv);
QWidget window;
window.resize(320, 240);
window.setWindowTitle("Hello C++ GUI");
window.show();
return a.exec();
}
In the code above:
- `QApplication` initializes the application, taking command-line arguments.
- `QWidget` is a standalone window where we set its size and title before displaying it.

Adding Functionality to Your GUI
Handling User Input
C++ GUI frameworks typically utilize a concept called signals and slots to handle user interaction. This mechanism allows certain actions (like button clicks) to trigger specific functions in your application.
Example: Connecting a Button Click
Here's how to connect a button's click event to a function that outputs a message:
#include <QPushButton>
#include <QDebug>
// In your setup function
QPushButton *button = new QPushButton("Press Me");
QObject::connect(button, &QPushButton::clicked, [](){
qDebug("Button Pressed!");
});
This snippet demonstrates how to create a button and connect its `clicked` signal to a lambda function that logs a message. Understanding lambda functions can significantly simplify event handling.
Updating the GUI Dynamically
For maintaining a responsive GUI, the use of threads or timers is essential. A timer can periodically update GUI components, such as changing the text displayed on a label.

Advanced GUI Features
Creating Menus and Toolbars
Menus enhance the usability of applications. In Qt, creating a menu bar is straightforward.
Example: Implementing a Basic Menu Bar
QMenuBar *menuBar = new QMenuBar();
QMenu *fileMenu = menuBar->addMenu("File");
fileMenu->addAction("Exit", qApp, &QApplication::quit);
In this snippet, a menu named "File" is created, which includes an "Exit" action that quits the application when selected.
Using Dialogs
Dialog windows are vital in GUIs for user interaction. They can collect input or display messages. For instance, to create a simple message box:
QMessageBox::information(nullptr, "Message", "Hello, World!");
This displays a message box with a title and content—a fundamental feature for engaging users.
Customizing Widgets
Enhancing the aesthetics of widgets can improve user experience. Customizing colors, fonts, and styles using Qt stylesheets can give your application a unique look that matches its functionality.

Debugging and Testing Your GUI Application
Common Errors and Fixes
As with any development process, errors are inevitable. Common issues include compilation errors due to missing includes or runtime errors from poor event handling.
Debugging Practices
Using debugging tools like gdb or the Qt Creator Debugger can help track down issues efficiently. Adopting best practices, such as structuring your code logically and maintaining modular functions, aids debugging significantly.

Conclusion
Creating a C++ GUI may seem daunting at first, but with the right framework and understanding of basic concepts, it becomes an attainable goal. Start with simple projects, explore advanced features, and soon you will be creating robust applications. Don't forget to engage with the development community for support and resources as you hone your skills in how to make a C++ GUI.

Additional Resources
For further learning, consult official documentation for Qt and wxWidgets, enroll in online courses, and consider reading books dedicated to C++ and GUI programming. Each resource can equip you with the tools needed to excel in creating delightful user interfaces.