To create a simple table in C++, you can use nested loops to display rows and columns of data in the console.
#include <iostream>
int main() {
std::cout << "Table:\n";
for (int row = 1; row <= 5; row++) {
for (int col = 1; col <= 5; col++) {
std::cout << row * col << "\t"; // Multiply row and column index
}
std::cout << "\n"; // Move to the next line after each row
}
return 0;
}
Understanding Tables in C++
What is a Table?
In programming, a table serves as a structured way to organize and manage data. Think of it as a grid where data is stored in rows and columns. In C++, tables can be represented using various data structures, most commonly arrays, structures, and classes.
Data Structures for Tables
Arrays
An array is a linear data structure that can hold multiple values of the same type. In the context of tables, multi-dimensional arrays are often used to represent grids. For instance, a 2D array can represent a table with rows and columns.
Example: 2D Array Declaration
int table[3][4]; // A table with 3 rows and 4 columns
Structures
A structure (struct) is a user-defined data type that allows you to combine different data types into a single unit. This is beneficial for organizing related data and can represent each row in a table clearly.
Example: Struct Definition
struct Student {
int id;
std::string name;
float grade;
};
Choosing the Right Data Structure
When deciding on the appropriate data structure for your table, consider the type and complexity of your data. Arrays are suitable for simple, homogeneous data. Structures are ideal for representing more complex, heterogeneous information, while classes allow for encapsulation and functionality.
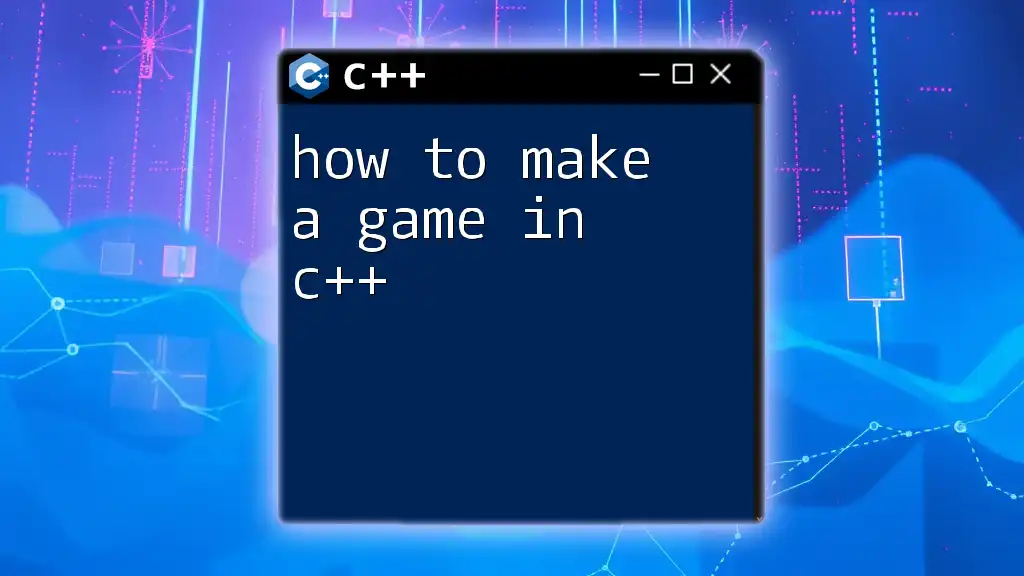
Creating a Simple Table Using Arrays
Step 1: Declaring and Initializing the Array
The first step in creating a table using arrays is to declare and initialize a 2D array. This array will hold your data in a structured format.
Example: Declare and Initialize a 2D Array
int table[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
Step 2: Accessing and Modifying Table Data
It's essential to understand how to access and modify the data within your table.
Accessing Elements: You can retrieve elements from the table using their indices.
Example:
std::cout << "Element at (1, 2): " << table[1][2]; // Output: 7
Modifying Elements: You can change the value of a specific element by directly indexing it.
Example:
table[1][2] = 99; // Change the value from 7 to 99
Step 3: Displaying the Table
To present your table data to the user, it's useful to create a function that prints out the entire array.
Function to Print the Table:
void printTable(int arr[3][4]) {
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 4; j++) {
std::cout << arr[i][j] << " ";
}
std::cout << std::endl;
}
}
Call this function to display your table after populating it.
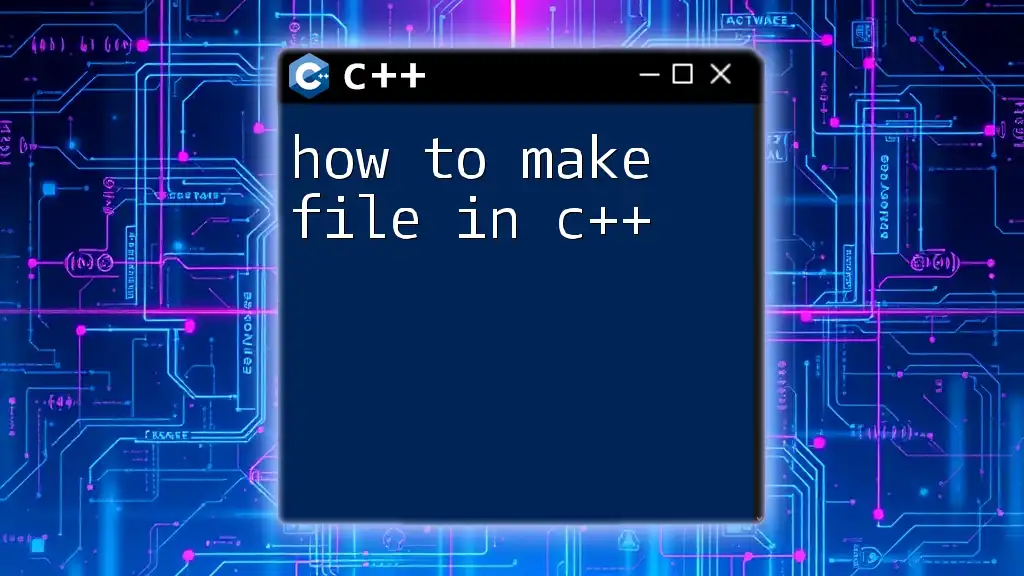
Creating a Table Using Structures
Step 1: Defining the Structure
Defining a structure is the first step to creating a table that can hold complex data types. Structures are particularly useful when you want to group different attributes of an entity.
Step 2: Creating an Array of Structures
Once you have defined a structure, you can create an array of that structure to represent a table.
Example:
Student table[3]; // Declare an array of Student structures
Step 3: Initializing the Array of Structures
You can initialize each individual structure in the array with specific values:
table[0] = {1, "Alice", 85.5};
table[1] = {2, "Bob", 90.0};
table[2] = {3, "Charlie", 88.0};
Step 4: Displaying the Table of Structures
It’s important to provide a way to display the data within your structures.
Function to Print Student Details:
void printStudents(Student students[], int size) {
for (int i = 0; i < size; i++) {
std::cout << "ID: " << students[i].id
<< ", Name: " << students[i].name
<< ", Grade: " << students[i].grade << std::endl;
}
}
You would call this function to display the student table after initializing it.
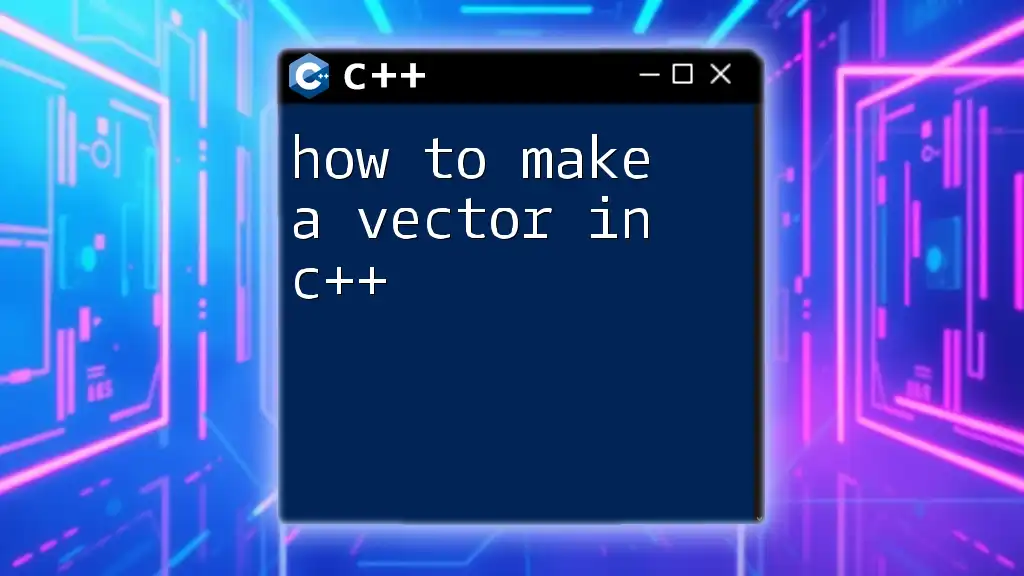
Advanced Table Creation with Classes
Step 1: Defining a Class
When working with more complex data, utilizing classes can encapsulate both data and methods, leading to better organization. This approach is especially useful when you need to manage the data actively.
Step 2: Methods for Managing Table Data
You can define methods within your class to manipulate and retrieve data from the table.
Example Code for Adding Data:
class StudentTable {
private:
std::vector<Student> students;
public:
void addStudent(int id, std::string name, float grade) {
students.push_back({id, name, grade});
}
// Additional Methods for data management...
};
Step 3: Example Usage
Using the defined class, you can create an instance and add student records dynamically:
StudentTable st;
st.addStudent(1, "Alice", 85.5);
st.addStudent(2, "Bob", 90.0);
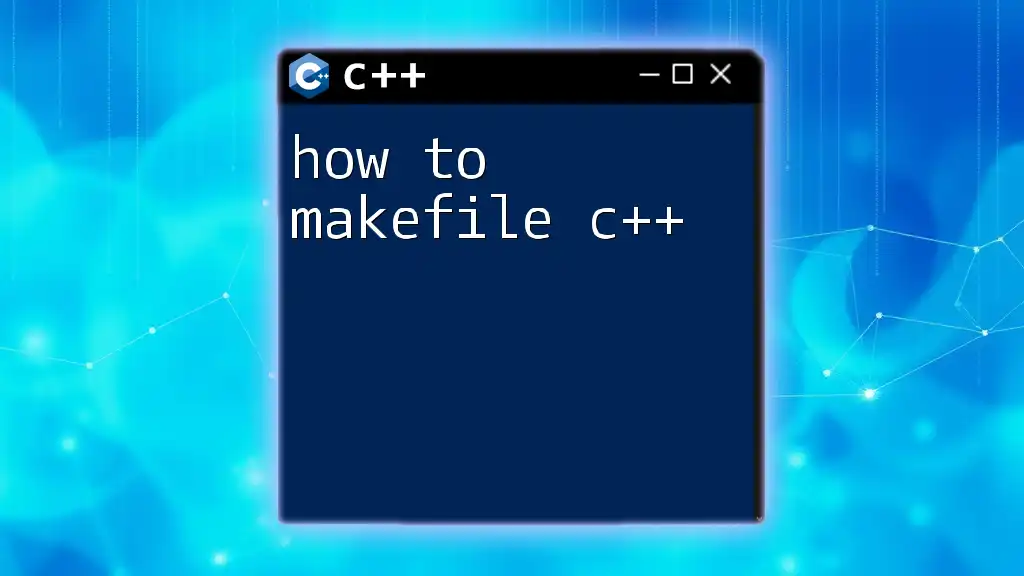
Conclusion
In this guide, we've explored various ways to create tables in C++, from using simple arrays to more complex structures and classes. By understanding how to manipulate these data structures efficiently, you can organize and manage your data effectively. Experimenting with these concepts will help you deepen your knowledge of C++ and enhance your programming skills. Start applying these techniques today to see how they can amplify your coding projects!