In C++, you can repeat code using loops such as `for`, `while`, or `do-while`, allowing you to execute a block of statements multiple times. Here’s an example using a `for` loop to print numbers from 1 to 5:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 5; i++) {
cout << i << endl;
}
return 0;
}
Understanding Loops in C++
Loops are an essential part of programming that allow for the execution of a block of code multiple times. They are particularly valuable in scenarios where you need to repeat actions or process collections of data. Understanding how to effectively use loops is crucial for anyone looking to excel in C++ programming.
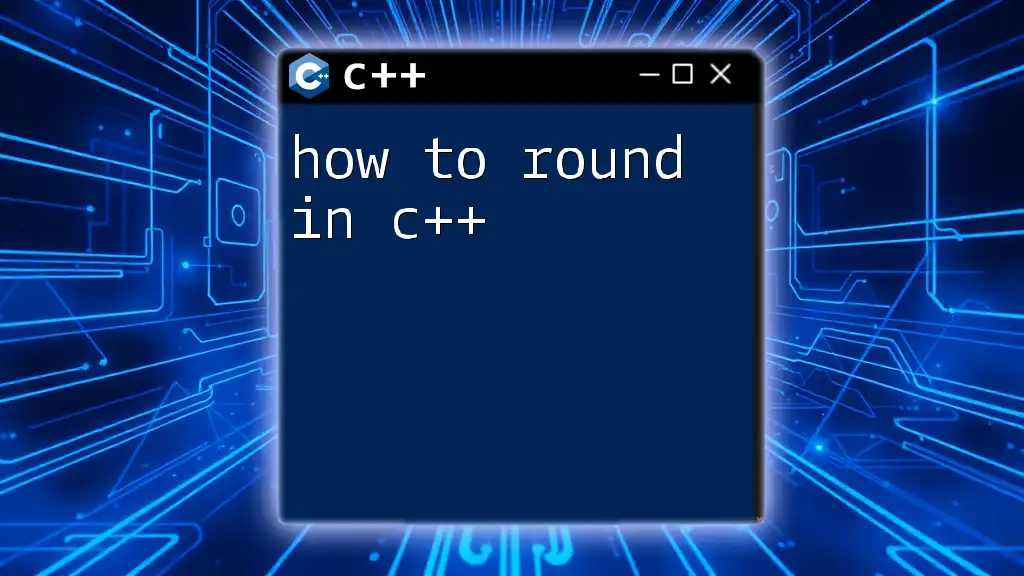
Types of Loops in C++
C++ offers several types of loops, each with its own specific use case. Mastering these loop structures will enhance code efficiency and simplify complex tasks.
For Loop
The for loop is a control structure that allows code execution to repeat a set number of times. Its syntax consists of three main parts: initialization, condition, and increment/decrement.
for(initialization; condition; increment/decrement)
{
// code to be executed
}
Use a for loop when you know in advance how many times you need to run a specific block of code. For example, if you want to print a series of numbers from 0 to 9, you can use:
for(int i = 0; i < 10; i++) {
std::cout << "Iteration: " << i << std::endl;
}
In this snippet:
- `int i = 0` initializes the counter `i`.
- The condition `i < 10` checks if `i` is less than 10.
- `i++` increments `i` by one after each iteration.
The code will output Iteration: 0 through Iteration: 9 sequentially.
While Loop
The while loop allows code execution based on a condition being true. This loop will continue to execute as long as the specified condition remains valid.
while(condition)
{
// code to be executed
}
A while loop is particularly useful when the number of iterations is not known ahead of time. Here's an example:
int i = 0;
while(i < 10) {
std::cout << "Iteration: " << i << std::endl;
i++;
}
In this example:
- The loop continues as long as `i` is less than 10.
- The counter `i` is incremented within the loop.
As a result, you will also see output from Iteration: 0 to Iteration: 9.
Do-While Loop
The do-while loop is similar to the while loop but guarantees that the block of code is executed at least once, regardless of the condition. The syntax is slightly different:
do {
// code to be executed
} while(condition);
Use this loop when you need the code to execute before checking the condition. Here's an example:
int i = 0;
do {
std::cout << "Iteration: " << i << std::endl;
i++;
} while(i < 10);
In this snippet, even if `i` starts from 0, the loop will execute and output Iteration: 0 through Iteration: 9 before the condition `i < 10` is checked again.
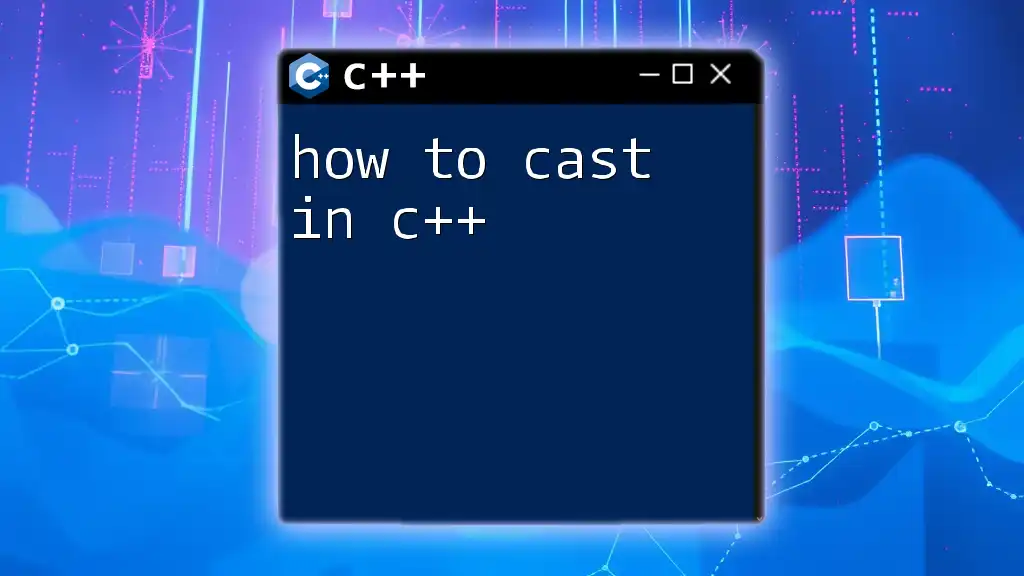
Nested Loops
Nested loops occur when one loop is placed inside another loop. This structure is useful for iterating through multi-dimensional data or performing complex operations.
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 2; j++) {
std::cout << "i: " << i << ", j: " << j << std::endl;
}
}
In this example:
- The outer loop iterates three times (`i` ranges from 0 to 2).
- The inner loop iterates through two iterations for each outer loop iteration.
The result will display combinations of `i` and `j`, such as:
i: 0, j: 0
i: 0, j: 1
i: 1, j: 0
i: 1, j: 1
i: 2, j: 0
i: 2, j: 1
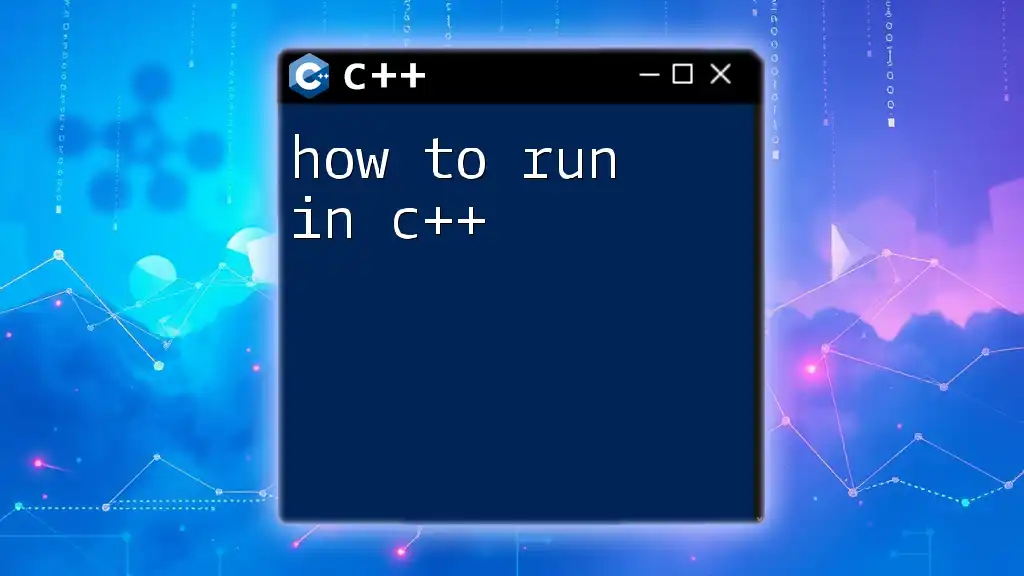
Breaking Out of Loops
Sometimes, you may need to exit a loop before its natural termination (i.e., before it completes all iterations). The break statement allows you to halt the execution of a loop instantly.
for(int i = 0; i < 10; i++) {
if(i == 5) break;
std::cout << "Iteration: " << i << std::endl;
}
In this code:
- As soon as `i` equals 5, the `break` command is executed, terminating the loop, which results in the output from Iteration: 0 to Iteration: 4 only.
Continuing to the Next Iteration
The continue statement is another essential tool that tells the loop to skip the current iteration and proceed to the next one. This can be used to filter out unwanted values effectively.
for(int i = 0; i < 10; i++) {
if(i % 2 == 0) continue; // Skip even numbers
std::cout << "Odd number: " << i << std::endl;
}
In this example:
- When `i` is even, `continue` prevents the execution of the subsequent code (`std::cout`), thereby printing only odd numbers. The output will be:
Odd number: 1
Odd number: 3
Odd number: 5
Odd number: 7
Odd number: 9
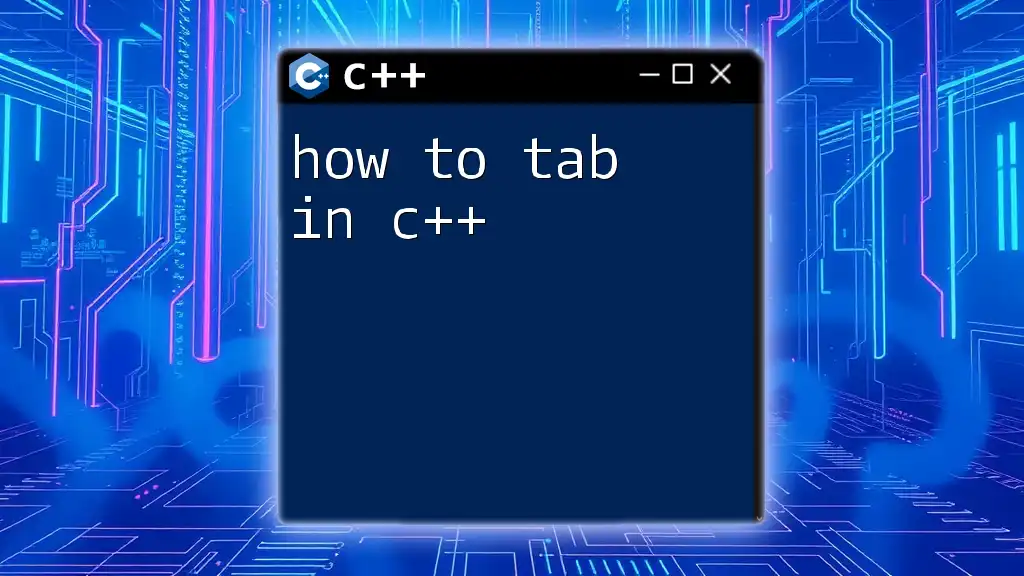
Tips for Writing Efficient Loops
Efficiency in loop writing is crucial. Here are some best practices to consider:
- Avoid Infinite Loops: Ensure that the loop has a well-defined exit condition to prevent it from running indefinitely, which can crash your program.
- Minimize Work inside Loops: Move any calculations or function calls that do not need to be inside the loop outside of it. This can significantly improve performance, especially in large data sets.
- Clear Intent: Always write loops that are easy to read and understand. Clear coding practices can save time during debugging and maintenance.
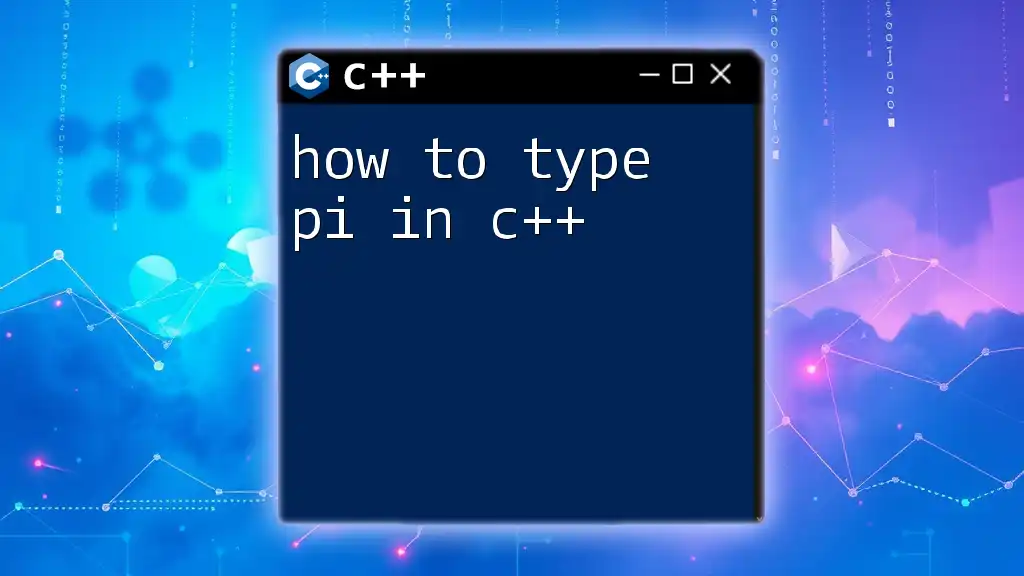
Conclusion
In this guide, we explored how to repeat in C++ using various loop constructs, including for loops, while loops, and do-while loops. By mastering these tools, you can increase the efficiency and simplicity of your code, ultimately making you a more effective programmer. Practicing these concepts will help you solidify your understanding and utilize C++ loops to their full potential.
Additional Resources
For further learning, be sure to check the official C++ documentation, which provides in-depth explanations and additional examples. Engaging with community forums can also be beneficial, as they offer a platform for asking questions and sharing programming knowledge.