`ofstream` in C++ is a class used to create and manipulate output files, allowing you to write data to files using stream operations. Here's a simple example:
#include <fstream>
int main() {
std::ofstream outFile("example.txt"); // Create an output file stream
outFile << "Hello, World!"; // Write to the file
outFile.close(); // Close the file
return 0;
}
Understanding ofstream
`ofstream` stands for "output file stream" and is part of the C++ standard library within the `<fstream>` header. It is specifically designed for writing data to files. In the domain of file input/output (I/O), we also have `ifstream` for input file streams and `fstream` for both input and output file streams. Understanding the role of each helps in selecting the appropriate tool for your specific needs.
How `ofstream` Fits into the C++ I/O Paradigm
C++ adopts a stream-oriented approach to handle input and output operations. In this context, an output stream allows you to send data to various destinations, including files. Using `ofstream`, you can easily write data to a file with a syntax that aligns closely with standard C++ output operations, making it intuitive for C++ programmers.
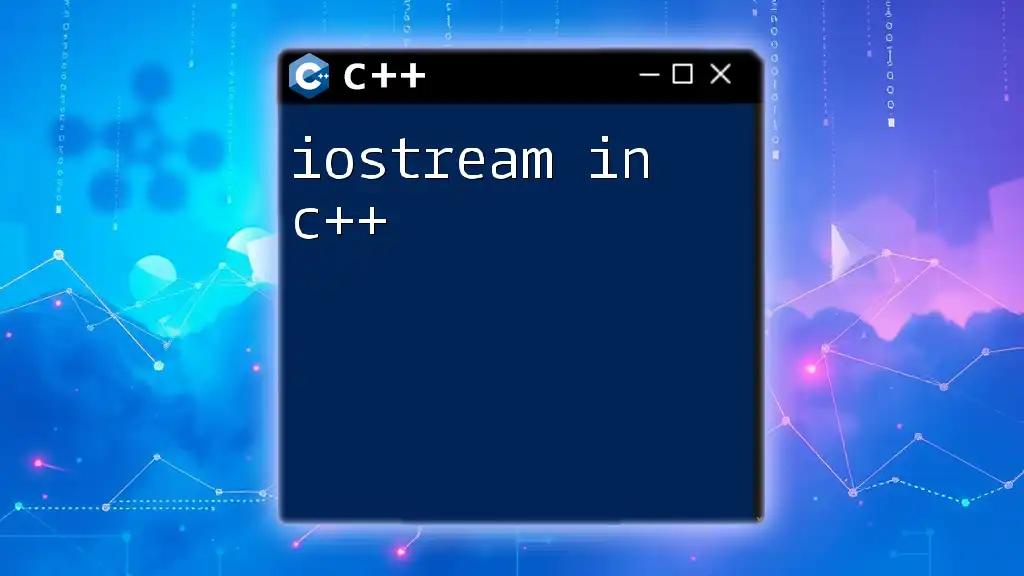
Creating and Opening a File with ofstream
Basic Syntax for ofstream
To utilize `ofstream`, you first need to include the `<fstream>` library. The basic instantiation looks like this:
#include <fstream>
std::ofstream outFile;
This line declares an ofstream object named `outFile`. However, simply declaring it does not open a file.
Opening a File
You have two primary methods for opening a file with `ofstream`: the constructor and the `open()` method.
Using the Constructor:
You can open a file directly by passing its name to the `ofstream` constructor:
std::ofstream outFile("example.txt");
Using the open() Method:
Alternatively, you can declare your `ofstream` object first and then call the `open()` method:
outFile.open("example.txt");
Error Handling:
It’s essential to check if the file opened successfully to avoid runtime errors. Here’s how you can implement error checking:
if (!outFile) {
std::cerr << "Error opening file." << std::endl;
}
This checks the state of the file stream. If it couldn't open the file, it will print an error message.

Writing Data to a File
Basic Writing Operations
Once the file is open, you can start writing data. The `<<` operator is used for this purpose, similar to writing to standard output with `cout`. Here's a simple example:
outFile << "Hello, World!" << std::endl;
This line writes "Hello, World!" to the file followed by a newline character.
Writing Multiple Lines
Writing multiple lines follows the same principle. Here’s how you can do it:
outFile << "Line 1" << std::endl;
outFile << "Line 2" << std::endl;
Every call to `outFile <<` appends new data to the end of the file. It is crucial to include `std::endl` if you want to introduce new lines.
Writing Different Data Types
`ofstream` can handle various data types, such as integers, floats, and strings. For example:
int age = 30;
outFile << "Age: " << age << std::endl;
This will save "Age: 30" into the file. Using `<<`, you can combine different data types in a single write operation, further showcasing the flexibility of `ofstream`.
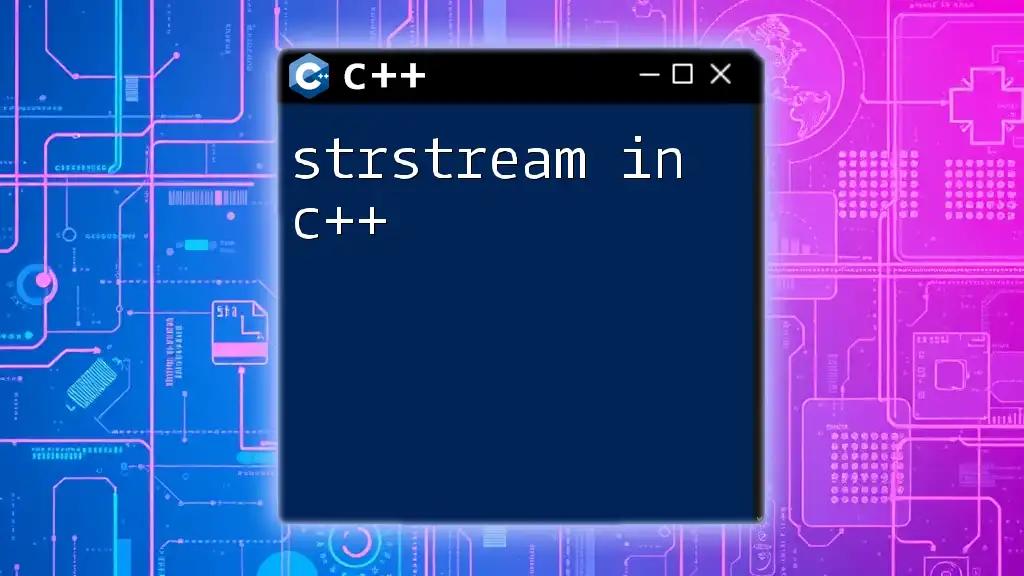
Closing the File
Importance of Closing the File
After you finish writing to the file, always close it. Neglecting to do so can lead to data loss, buffer corruption, or file locking issues. Closing a file signals the end of operations and ensures that all data written is properly saved.
Syntax for Closing an ofstream
To close an `ofstream`, you simply call the `close()` method:
outFile.close();
It’s good practice to close files as soon as you’re done using them to free up resources.

Error Handling with ofstream
Common Issues
When working with file streams, several issues can arise, such as failure to open a file or write access issues. It’s crucial to implement error handling to address these problems.
Using Exceptions
To improve robustness, you can enable exceptions for file streams. This involves utilizing try-catch blocks to catch and handle exceptions. Here’s an example:
try {
outFile << "Writing some data" << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
In this snippet, if any exception occurs during the write operation, it will be caught, and an error message will be displayed.
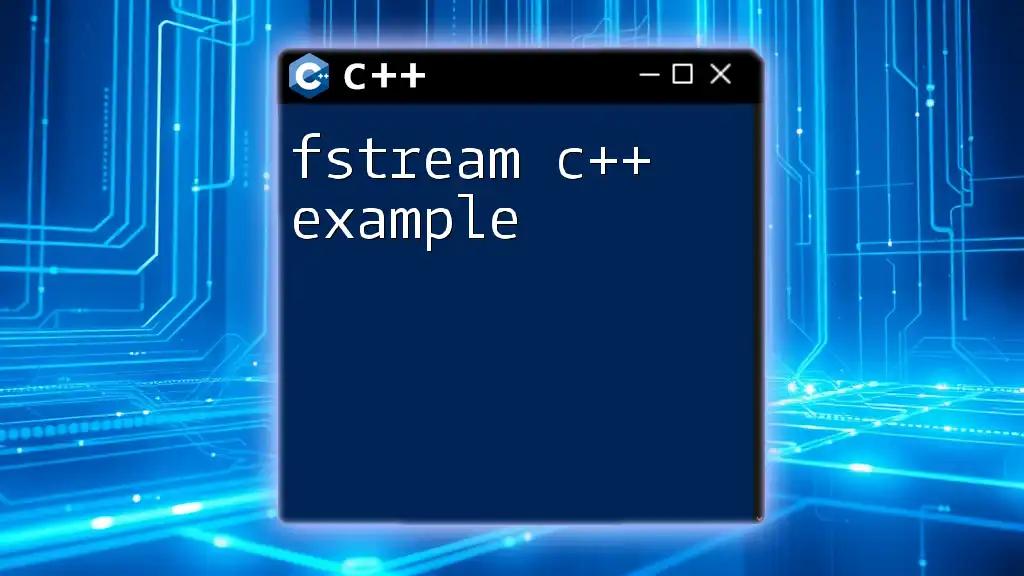
Best Practices for Using ofstream
To optimize your file handling operations with `ofstream`, consider the following best practices:
- only open files when necessary and close them immediately after use to conserve resources.
- understand the difference between `std::endl` and `'\n'`. While both produce newlines, `std::endl` also flushes the output buffer, which can slow down performance if used excessively.
- be aware of buffering considerations when writing large amounts of data. It might be beneficial to write data in chunks rather than line-by-line to enhance efficiency.

Conclusion
In this comprehensive guide, we've explored the ins and outs of `ofstream in C++`. From creating and opening files to writing and closing them, these essential commands provide the foundation for file output operations in your C++ applications. As you continue your journey in C++, mastering file handling will significantly improve your programming capabilities and teach you valuable skills that can be applied to file data management. Be sure to practice extensively and explore additional resources to deepen your understanding.