`ifstream` in C++ is used to read data from files, allowing you to easily handle input file streams in your programs. Here's a simple example of how to use `ifstream` to read from a file:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("example.txt"); // Open the file
std::string line;
if (inputFile.is_open()) { // Check if the file opened successfully
while (getline(inputFile, line)) { // Read line by line
std::cout << line << std::endl; // Output the line to the console
}
inputFile.close(); // Close the file when done
} else {
std::cerr << "Unable to open file";
}
return 0;
}
Overview of File Handling in C++
File handling is a critical aspect of programming that enables applications to read from and write to files. In C++, file operations are performed using streams, which are abstractions allowing data to flow in and out of the program. The C++ Standard Library provides several classes to handle file input and output, including `ifstream`, `ofstream`, and `fstream`.
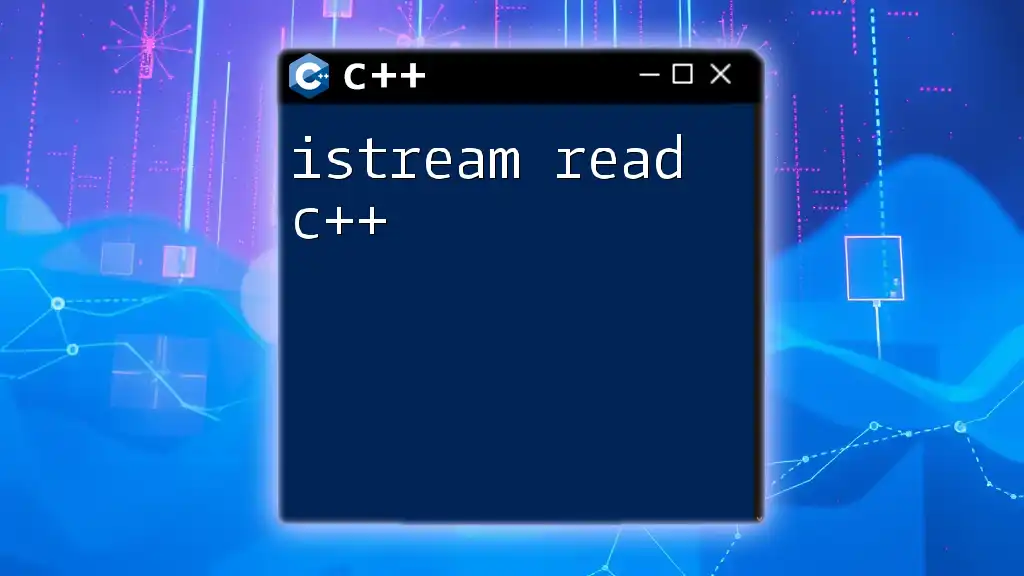
What is ifstream?
`ifstream`, short for "input file stream," is a C++ class specifically designed for reading data from files. It allows developers to read formatted and unformatted data from input files.
Key differences between `ifstream` and other stream classes:
- ifstream is used primarily for reading data from files.
- ofstream is intended for writing data to files.
- fstream can perform both reading and writing operations.
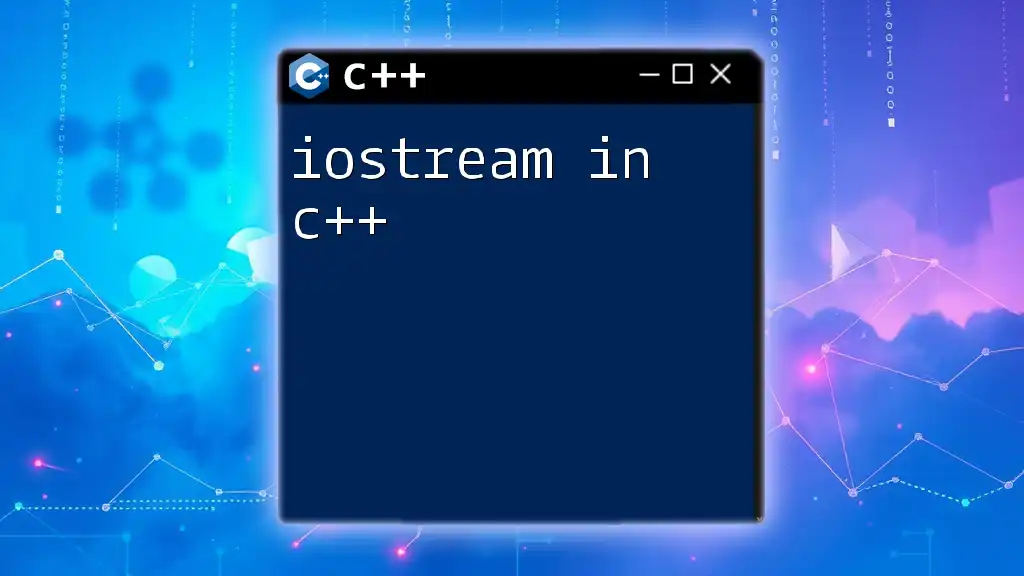
Setting Up Your Environment for Using ifstream
To begin using `ifstream` in your C++ projects, ensure you have a suitable C++ compiler and an Integrated Development Environment (IDE) set up. Many developers opt for popular IDEs like Visual Studio, Code::Blocks, or even text editors like Visual Studio Code.
Requirements for Using ifstream
- C++ Compiler: Ensure that your development environment has a compatible C++ compiler, such as GCC or Clang.
- Project Structure: Organize your files systematically so that your program can easily access the data files you plan to read.
Including the Required Header Files
To use `ifstream`, you need to include the `<fstream>` header at the beginning of your C++ source file:
#include <fstream>
This header contains the necessary declarations for using file streams.
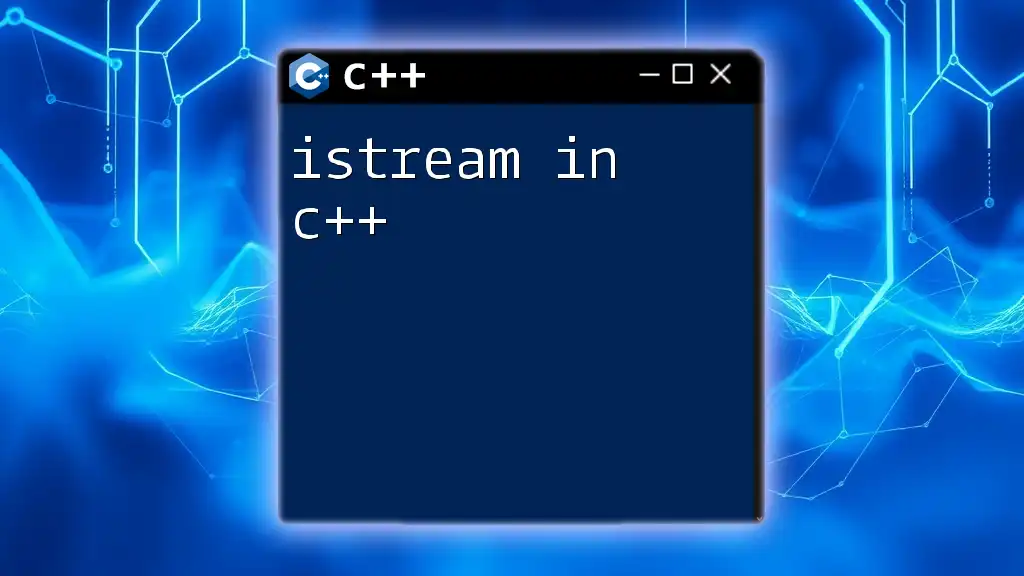
Basic Usage of ifstream
To start reading a file, you will need to create and open it using `ifstream`.
Creating and Opening Files with ifstream
The process for declaring and opening an input file is straightforward. Here's the syntax:
std::ifstream inputFile("example.txt");
Important: Always check if the file opened successfully to avoid runtime errors. You can do this with the following code snippet:
if (!inputFile) {
std::cerr << "Unable to open file" << std::endl;
return 1; // Or appropriate error handling
}
Checking if the File is Open
After attempting to open the file, it's crucial to verify that the file is accessible. Use the `if` statement to check the stream's state.
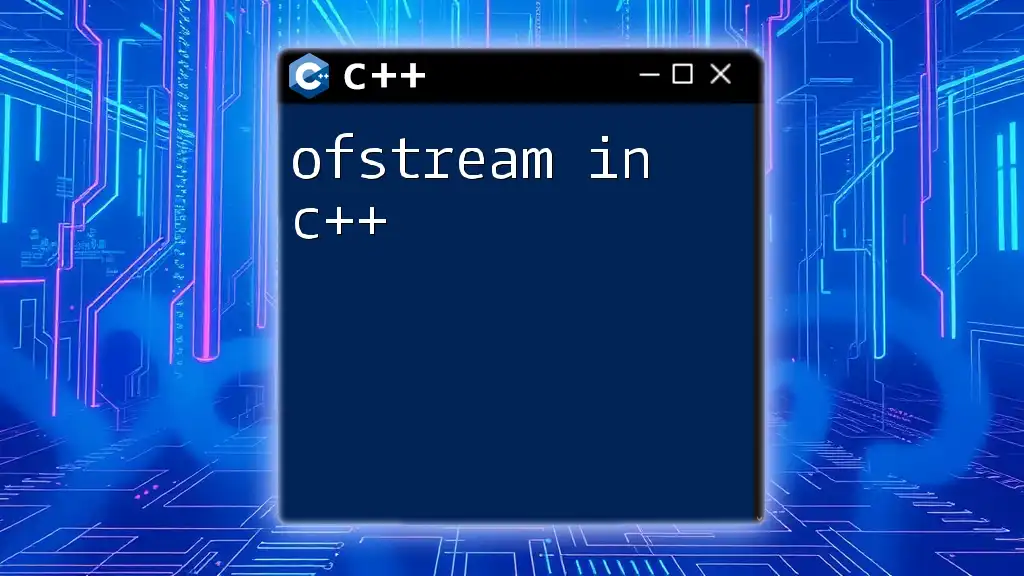
Reading Data from Files Using ifstream
Once the file is successfully opened, you can begin to read its contents.
Reading from a File with getline()
One efficient way to read lines of text is by using the `getline()` function. This function reads a full line from the input stream until it encounters a newline character.
Here’s an example demonstrating how to read multiple lines from a file:
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
In this example, each line read from the file is stored in the variable `line` and then printed to the console.
Reading Formatted Data with Operator Extraction
Using the operator extraction (`>>`) is a common method to read formatted data from a file. This operator automatically separates the input based on whitespace.
Here's how you can read an integer from a file:
int number;
inputFile >> number;
std::cout << "Number read: " << number << std::endl;
This code reads an integer from the opened file and outputs it.
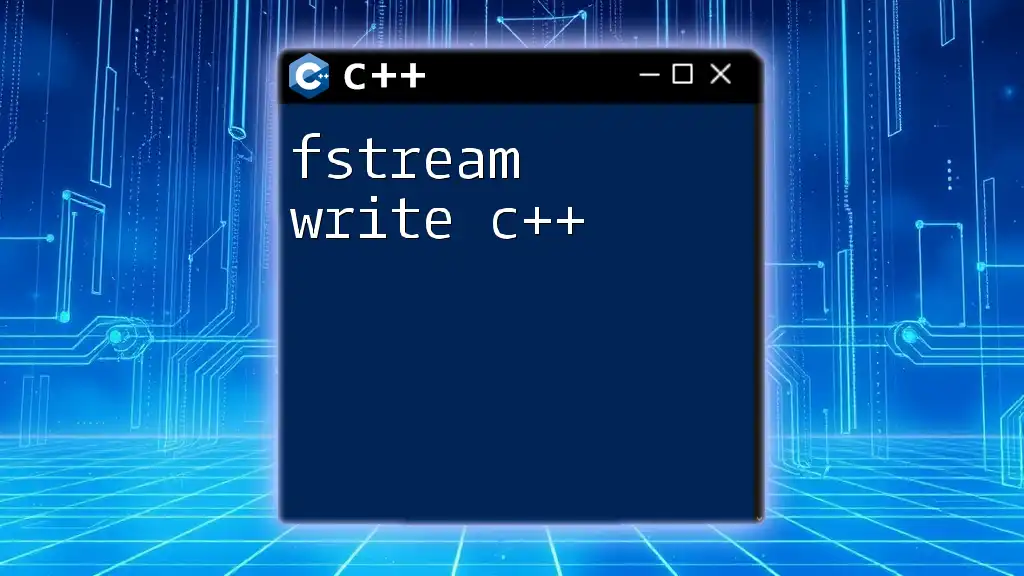
Handling Different Data Types
With `ifstream`, you can read various data types effectively. This flexibility allows the program to handle different kinds of data as needed.
Reading Various Data Types from Files
Whether you need to read integers, doubles, or strings, `ifstream` can accommodate all. Below are snippets for each data type.
For reading a `double` value:
double value;
inputFile >> value;
std::cout << "Value read: " << value << std::endl;
In a similar manner, strings can be read using the extraction operator:
std::string name;
inputFile >> name;
std::cout << "Name read: " << name << std::endl;
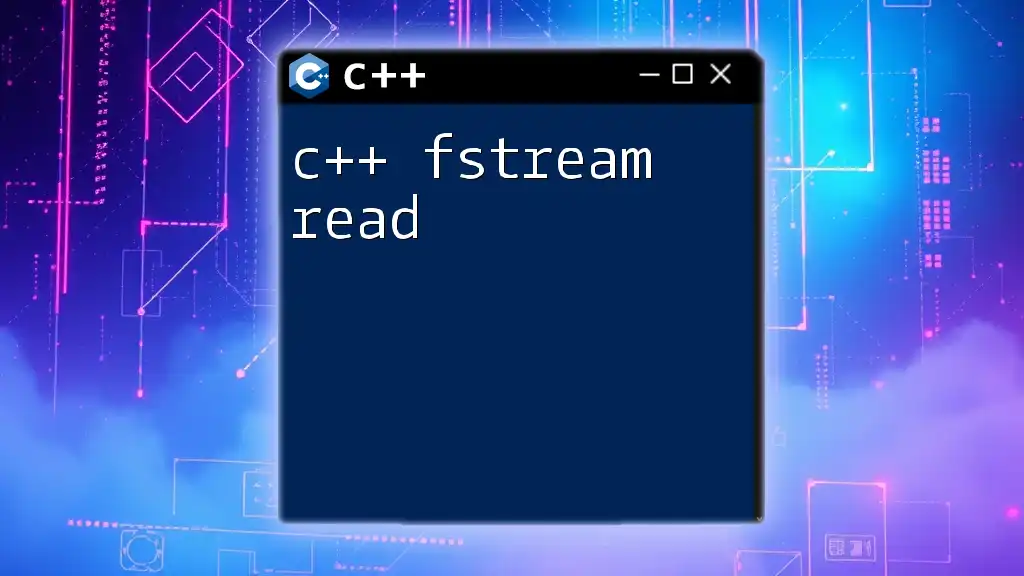
Error Handling with ifstream
File operations can often lead to errors due to various reasons such as missing files or incorrect formats. Proper error checking is essential for robust application behavior.
Best Practices for Error Checking
Implement error handling by using the `fail()` or `eof()` methods. The `fail()` method checks if the last input operation was unsuccessful—helpful for parsing errors.
Here’s a basic example of error handling:
if (inputFile.fail()) {
std::cerr << "Error reading data." << std::endl;
}
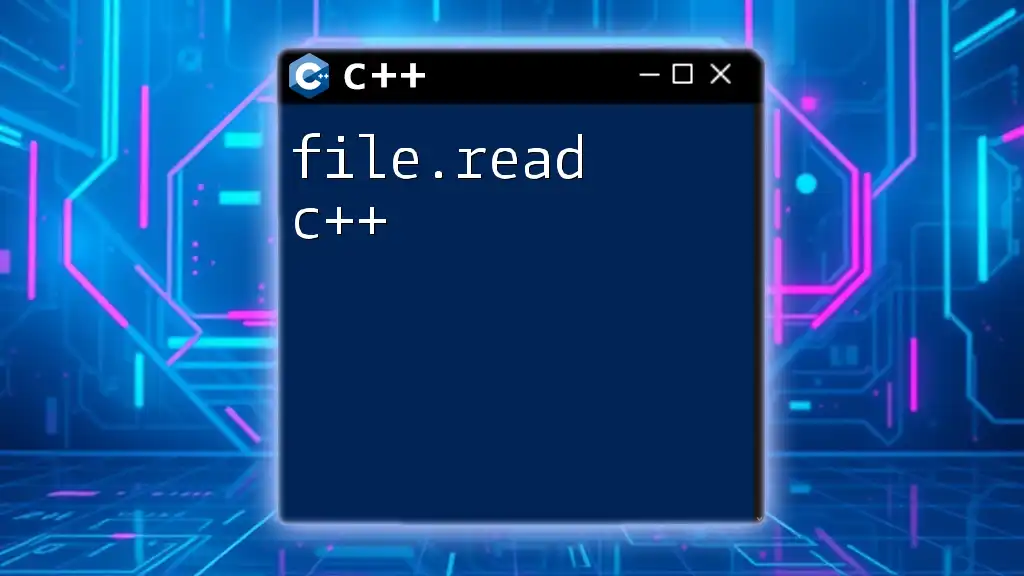
Closing the ifstream
Once you've completed all read operations, it’s essential to properly close your `ifstream` object. This action releases the resources associated with the file.
To close the stream, use the following method:
inputFile.close();
This step ensures that all file resources are freed, preventing potential memory leaks.
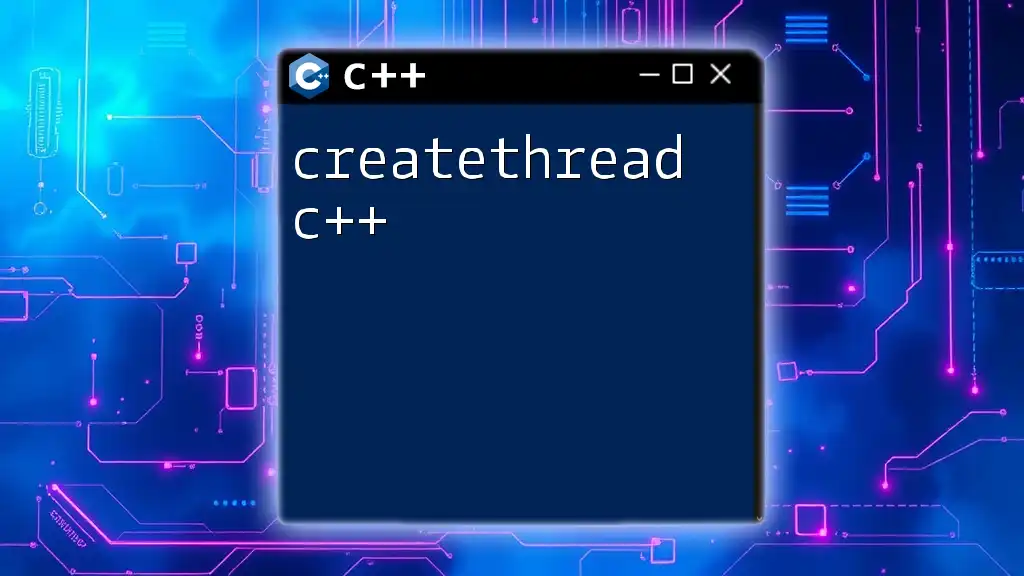
Common Issues and Troubleshooting
While working with `ifstream`, you may run into issues. Understanding typical mistakes and their resolutions can make your programming experience smoother.
Debugging Tips for ifstream Errors
- File Not Found: Ensure the specified file path is correct. You can also use absolute paths to avoid confusion.
- Incorrect Data Types: Ensure that the data types in the file match those expected in your code. Read operations may fail if there's a mismatch.
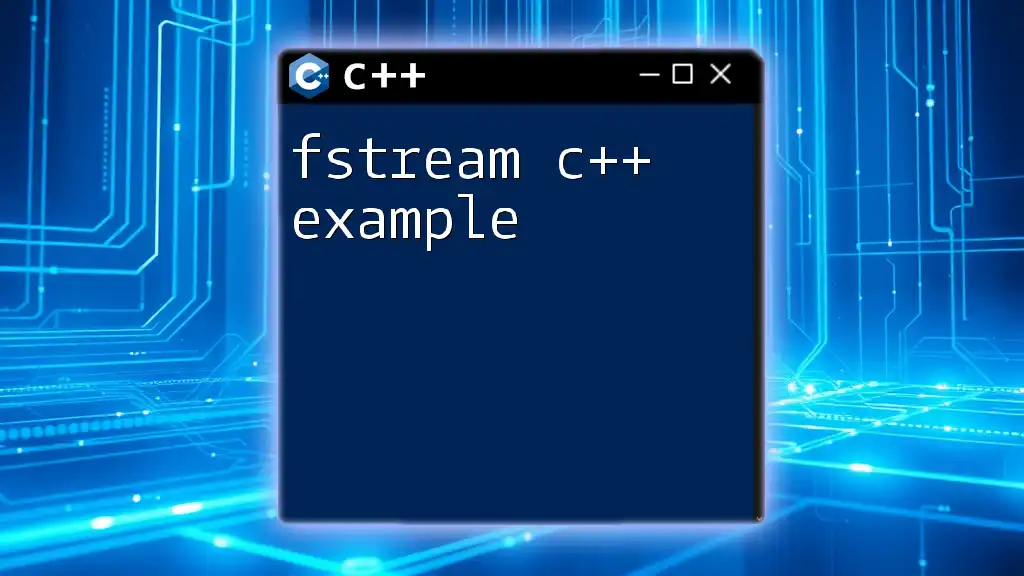
Conclusion
In this comprehensive guide, we've explored how to effectively use `ifstream` for reading files in C++. From creating file streams, reading various data types, to implementing error handling strategies, you now have a solid foundation to work with file input streams in your applications.
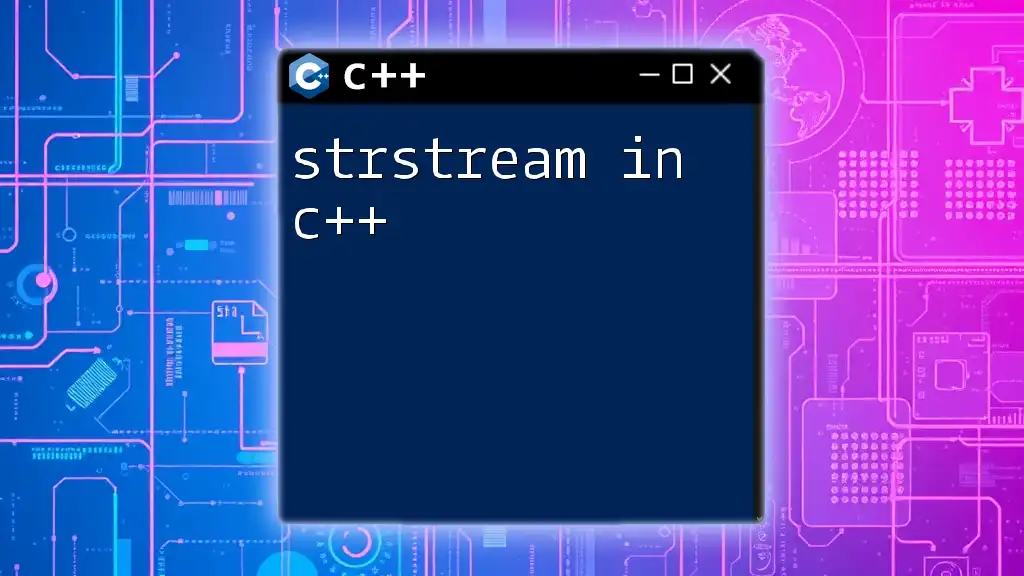
Additional Resources
For further learning about file handling in C++, consider visiting the official C++ documentation or enrolling in focused online courses. There are many resources available that delve deeper into C++ file I/O operations and best practices.
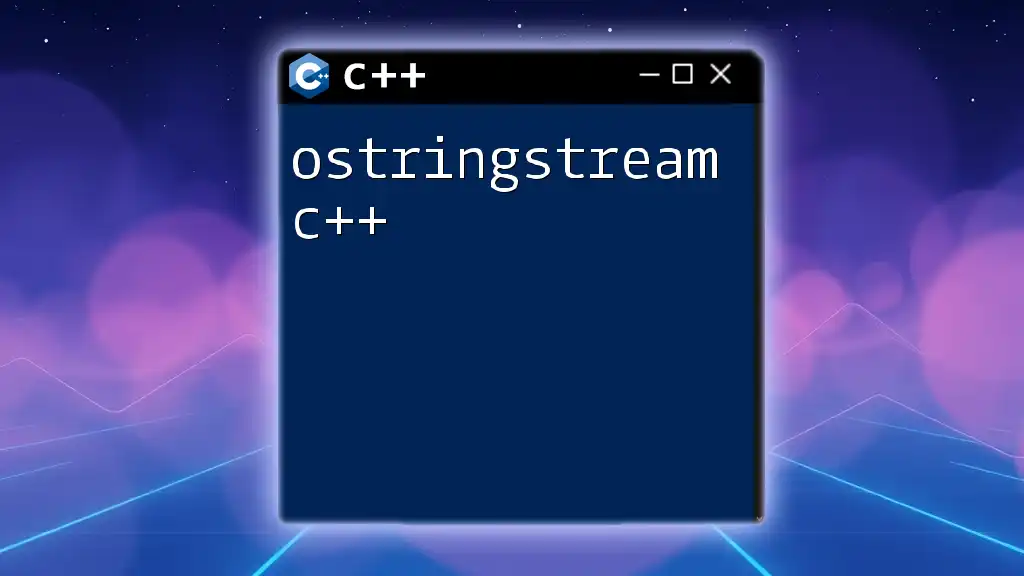
Call to Action
As you embark on your programming journey, consider joining communities where you can share knowledge, ask questions, and collaborate with fellow learners. Don’t hesitate to explore, experiment, and put your knowledge of `ifstream` to practical use!