`istream` in C++ is a class that enables input stream functionality, allowing the program to read data from various sources, such as the keyboard or files.
Here’s an example of using `istream` to read an integer from standard input:
#include <iostream>
int main() {
int number;
std::cout << "Enter an integer: ";
std::cin >> number; // Using istream to read input
std::cout << "You entered: " << number << std::endl;
return 0;
}
Understanding Streams in C++
What Are Streams?
In C++, streams are abstract representations of input/output (I/O) devices. Streams allow data to flow seamlessly between the program and the external environment, such as keyboards or files. Understanding the distinction between input streams (for receiving data) and output streams (for sending data) is crucial for effective data handling in C++.
The Role of istream
The `istream` class, part of the C++ Standard Library, specifically handles input operations. It provides a powerful interface for reading data from various sources, including user input or files. By mastering `istream`, programmers can enhance their ability to accept and process user data, which is essential for interactive applications.
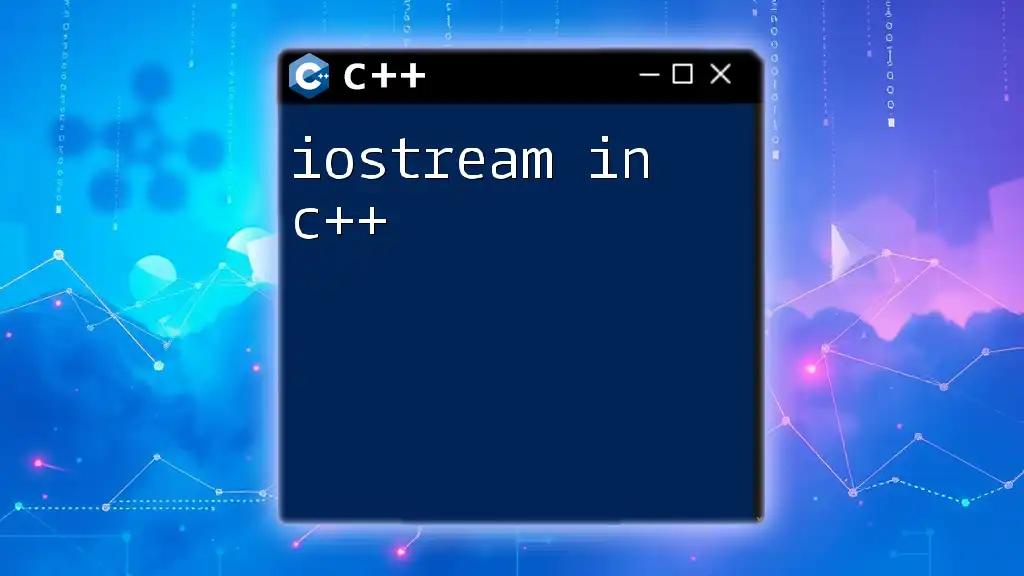
Getting Started With istream
Including the Required Headers
To utilize `istream`, you must include the `<iostream>` header. This file contains definitions for the I/O stream objects, including `cin`, which is the standard input stream.
#include <iostream>
Basic Usage of istream
An `istream` object can be created directly as part of standard input operations, typically through the `cin` instance.
std::istream& input = std::cin;
Commonly, you will use `std::cin` directly for input operations, allowing users to provide data during the runtime of the program. `istream` is crucial for functions that require user input or require reading from files.
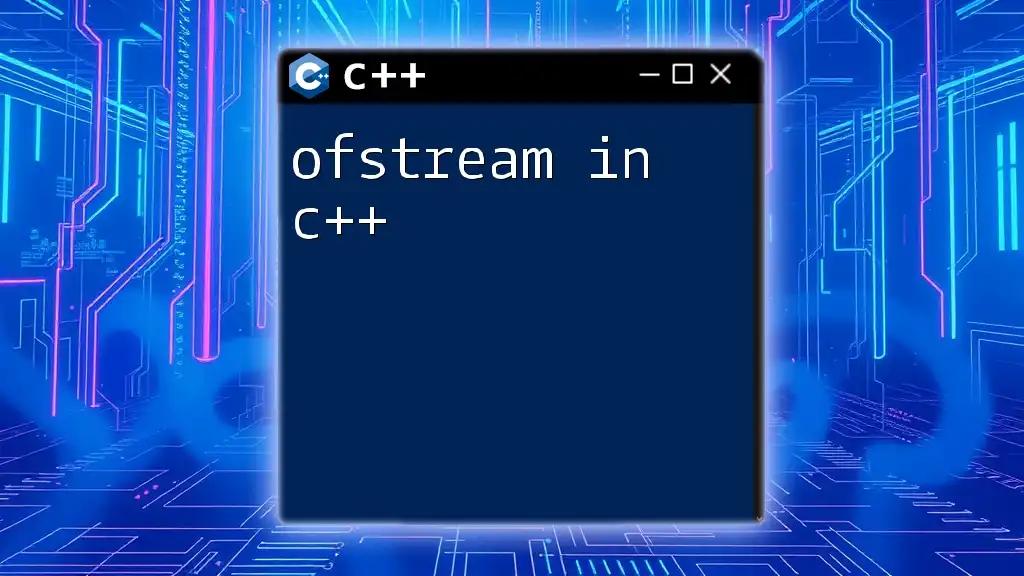
Common Forms of Input With istream
Reading from Standard Input
Reading data from standard input is a common use of `istream`. You typically use the `>>` operator to extract data from `cin`.
int number;
std::cout << "Enter a number: ";
std::cin >> number;
In this example, a prompt guides the user to enter a number, which is then stored in the variable `number`. The ease of use of `istream` makes it user-friendly, encouraging efficient interaction.
Reading from Files Using istream
To read from files, `ifstream` (input file stream) inherits from `istream`. This allows you to leverage `istream` capabilities while reading data from files.
#include <fstream>
std::ifstream file("data.txt");
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
}
In this example, we open a file named `data.txt` for reading, check if the file is successfully opened, and read it line by line utilizing `getline()`, another essential `istream` function.
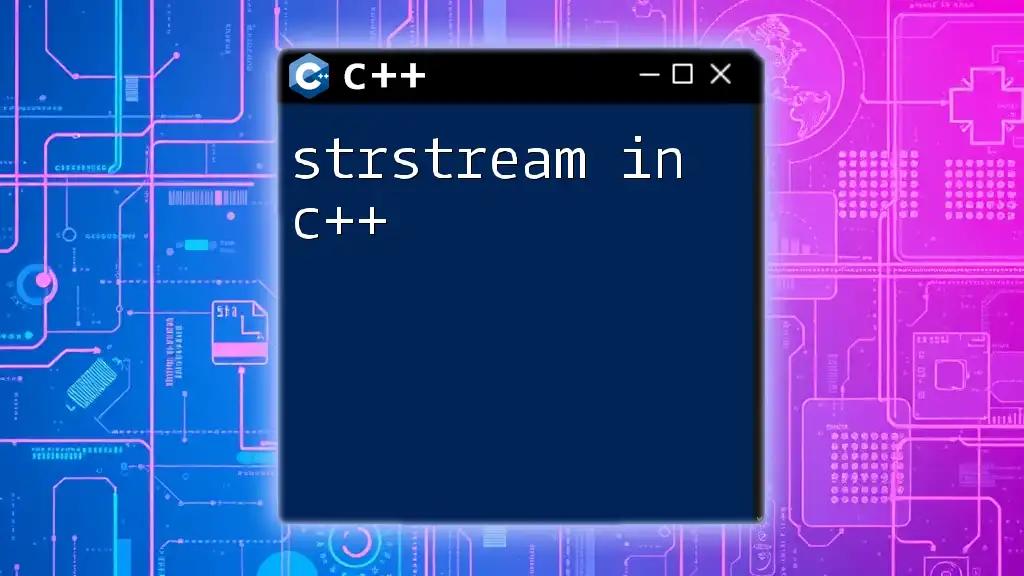
Key Functions and Methods of istream
Fundamental Input Methods in istream
`istream` provides several methods for extracting data. The most common methods include:
-
`getline()`: Reads a line of text including spaces, stopping at a newline character.
std::string input; std::cout << "Enter a line: "; std::getline(std::cin, input);
-
`>>` Operator: Commonly used for formatted input, suitable for reading fundamental data types.
double value; std::cout << "Enter a decimal number: "; std::cin >> value;
-
`read()`: Reads a specified number of characters into a buffer.
char buffer[10]; std::cin.read(buffer, sizeof(buffer));
Choosing between these methods generally depends on the type of data being read and the desired format.
Handling Different Data Types
`istream` allows for reading various data types, including integers, floating-point numbers, and strings. The mechanisms for type safety and conversions where feasible ensure robustness.
int age;
std::cout << "Enter your age: ";
std::cin >> age;
When using the `>>` operator for input, if the data type does not match the expected input (e.g., entering a letter instead of a number), `istream` sets an error state.

Error Handling with istream
Understanding State Flags
`istream` has built-in state flags, such as `eof` (end of file), `fail`, and `bad`, which provide information about the stream's current status.
- `eof`: Indicates whether the end of the input stream has been reached.
- `fail`: Set when an input operation fails due to type mismatch.
- `bad`: Indicates a serious error, usually related to reading from a device or file.
You can check these flags using the state-checking methods:
if (std::cin.fail()) {
std::cout << "Input fail!";
}
Implementing Robust Input
To ensure safe and user-friendly input, implement strategies like clear error handling with loops that prompt the user until valid data is received.
int number;
while (true) {
std::cout << "Enter a number: ";
std::cin >> number;
if (std::cin.fail()) {
std::cin.clear(); // clear the fail state
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // discard invalid input
std::cout << "Invalid input. Please enter a valid number.\n";
} else {
break; // break when input is valid
}
}
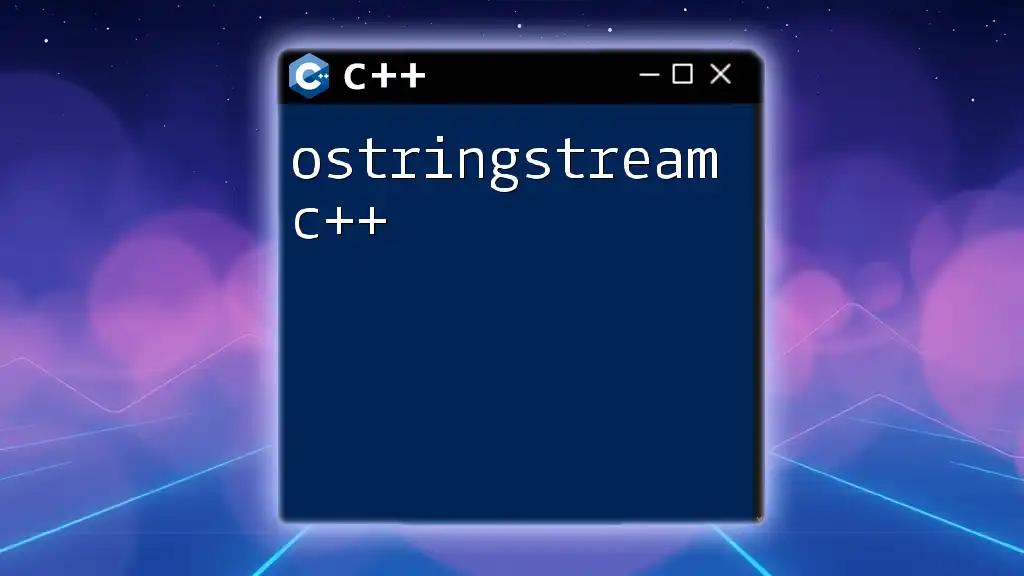
Advanced Usage of istream
Custom Input Streams
You can create custom input streams by extending `istream`. This is particularly useful for specialized input handling scenarios, such as reading from networks or custom data sources.
class MyInput : public std::istream {
public:
MyInput() : std::istream(nullptr) {
// Custom initialization
}
// Implement custom input functionalities
};
Overloading Operators with istream
Operator overloading for the `>>` operator allows you to define how objects of custom data types are read from an input stream.
class Person {
public:
std::string name;
int age;
friend std::istream& operator>>(std::istream& in, Person& p) {
in >> p.name >> p.age;
return in;
}
};
// Usage
Person person;
std::cout << "Enter name and age: ";
std::cin >> person;
This example showcases how to read an object of `Person` using `istream` capabilities.
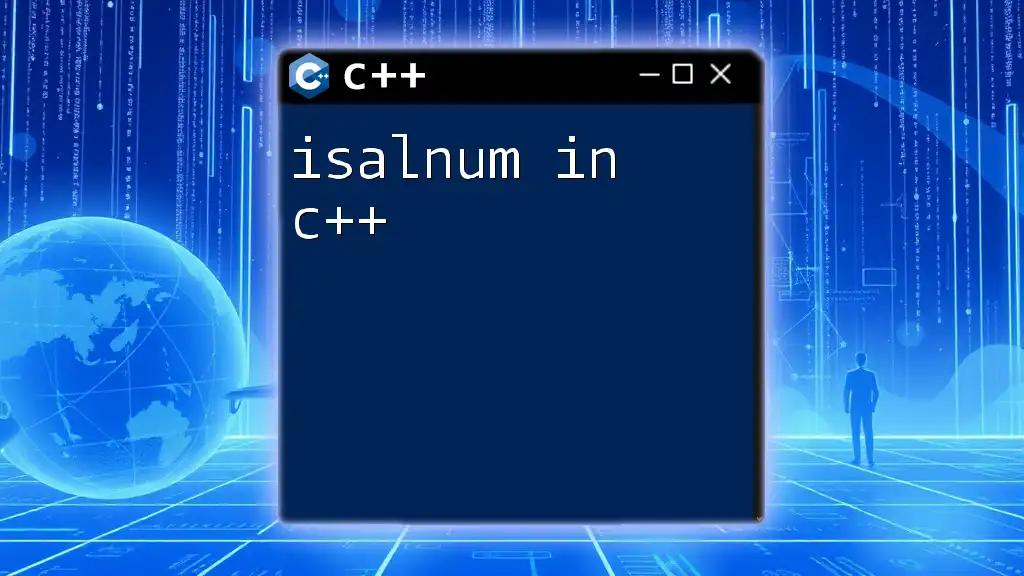
Summary
In conclusion, istream in C++ provides a robust and flexible framework for handling input from various sources. By mastering `istream`, developers can create more interactive applications that effectively gather and process user data. Using the techniques explored in this article will enhance your C++ programming skills, enabling you to develop more resilient input handling in your applications.
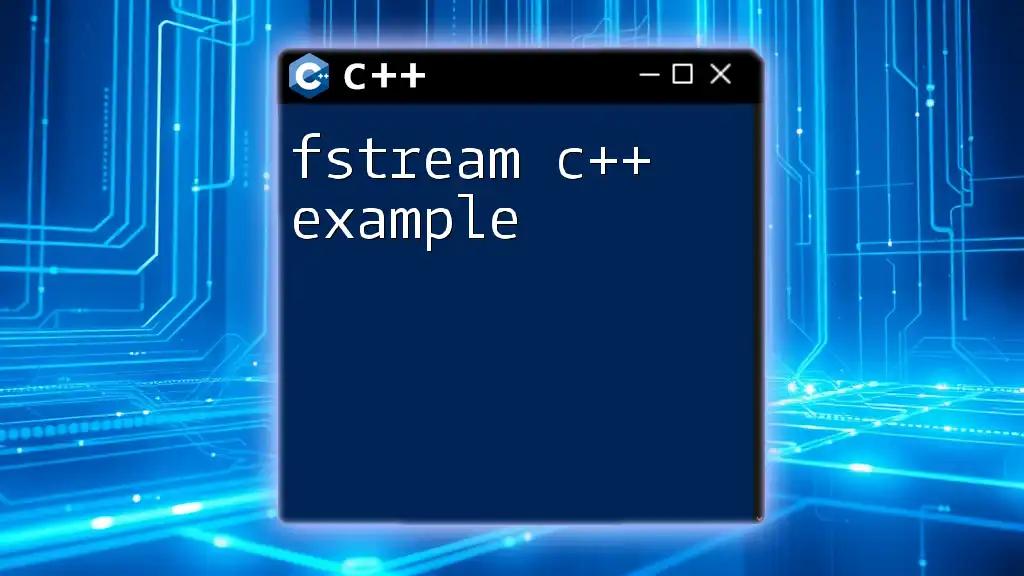
Additional Resources
For those looking to deepen their understanding further, consider exploring the official C++ documentation, relevant programming books, and additional tutorials that cover streams and input/output operations.

Conclusion
Mastering `istream in C++` is an invaluable skill for any programmer looking to handle user input efficiently and effectively. Engaging with the concepts and examples provided is an excellent way to solidify your understanding and ensure you're prepared to tackle real-world coding challenges. Don't hesitate to experiment with the examples and ask questions to enhance your learning experience!