The `strncmp` function in C++ compares two strings up to a specified number of characters and returns an integer indicating their lexicographical difference.
#include <iostream>
#include <cstring>
int main() {
const char* str1 = "Hello";
const char* str2 = "Helium";
int result = strncmp(str1, str2, 3); // Compare first 3 characters
std::cout << "Comparison result: " << result << std::endl; // Outputs 0 if equal, <0 if str1 < str2, >0 if str1 > str2
return 0;
}
What is strncmp?
`strncmp` is a function in C++ that allows for the comparison of two C-style strings up to a specified number of characters. This function is particularly important when you need a controlled comparison of strings, preventing common pitfalls associated with string handling in C++.
Parameters and Return Value
The syntax for `strncmp` is as follows:
int strncmp(const char *str1, const char *str2, size_t n);
- `str1`: The first string to be compared.
- `str2`: The second string to be compared.
- `n`: The maximum number of characters to compare.
The function returns:
- A positive value if `str1` is lexicographically greater than `str2`.
- A negative value if `str1` is lexicographically less than `str2`.
- Zero if the first `n` characters of both strings are equal.

How strncmp Works
Behind the Scenes
Internally, `strncmp` compares strings character by character until either a difference is found or the specified number of characters (`n`) have been checked. Strings must be null-terminated in C++, meaning the end of the string is marked by a `'\0'` character. If the function reaches the null terminator in one or both strings before `n` characters are compared, it stops comparing further. This built-in safety mechanism helps prevent reading past the end of the string.
Return Values
Here’s how the return values are interpreted:
- Positive Value: Indicates that `str1` comes after `str2` lexicographically.
- Negative Value: Indicates that `str1` comes before `str2` lexicographically.
- Zero: Confirms that the two strings are equal in their first `n` characters.
For example:
const char *str1 = "Hello";
const char *str2 = "HelloWorld";
int result = strncmp(str1, str2, 5); // result will be 0
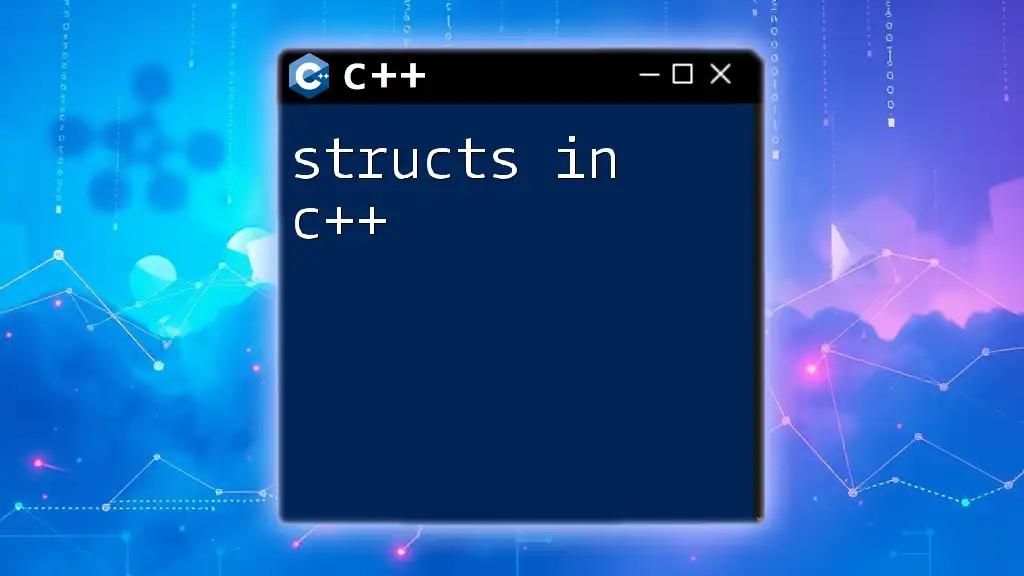
Practical Use Cases for strncmp in C++
Comparing Substrings
There are many situations where you may want to compare only part of a string instead of the entire string. Using `strncmp` allows you to specify exactly how many characters to compare, which can be especially useful in file operations or user input validation.
For instance, if you're comparing two filenames:
if (strncmp(file1, file2, 5) == 0) {
// Execute code for matching based on the first 5 characters.
}
Ensuring Safety with strncmp
One of the main advantages of `strncmp` is its ability to compare strings safely, preventing buffer overflow errors. This is particularly helpful in user inputs or dynamic string comparisons:
const char *str1 = "Hello";
const char *str2 = "Helloo";
if (strncmp(str1, str2, 4) == 0) {
// Safely compare strings here without issues.
}
In this code, only the first four characters will be compared, mitigating any risk inherent in string size discrepancies.
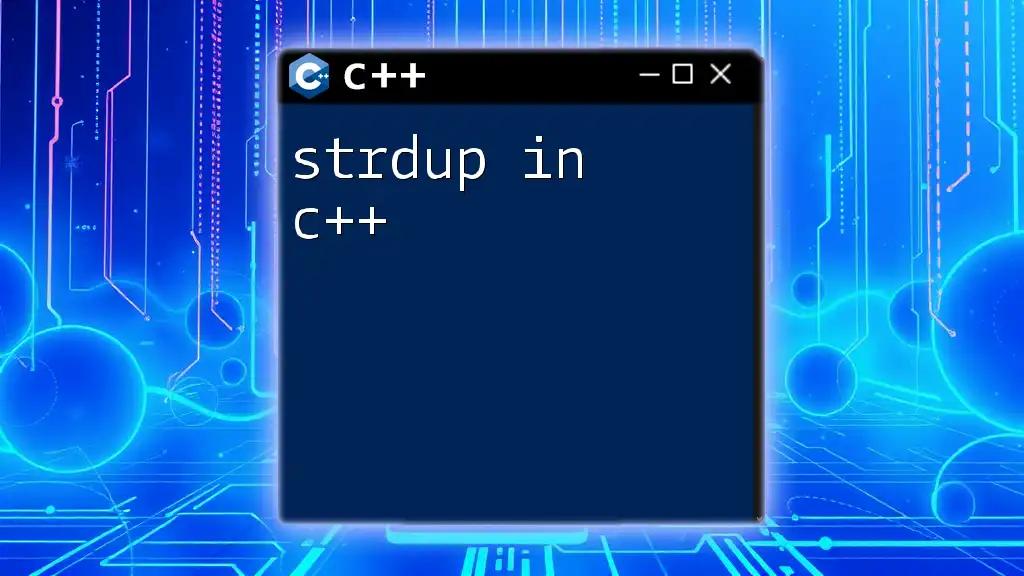
Common Mistakes When Using strncmp
While `strncmp` is a powerful tool, there are common pitfalls to avoid:
-
Ignoring string lengths: Always remember that if `n` exceeds the length of either string, `strncmp` will stop at the null terminator and will not throw an error, potentially leading to unexpected results.
-
Misunderstanding return values: It is vital to understand the implications of the return values to handle conditions correctly.
An example of incorrect usage:
if (strncmp(str1, str2, 10) == 0) {
// This might not produce the expected results.
}
If `str1` or `str2` is shorter than 10 characters, this comparison could lead to misleading conclusions about their equality.

Alternative Functions to strncmp
strcmp vs strncmp
The function `strcmp`, unlike `strncmp`, compares entire strings without a character limit. When dealing with known string lengths, opt for `strncmp` to minimize unnecessary operations. However, if you need a direct comparison without limiting the character count, `strcmp` may suit your needs better.
C++ String Class
In modern C++ programming, you can utilize `std::string`, which simplifies string handling and comparisons significantly. With `std::string`, comparison becomes straightforward with the `==` operator:
std::string strA = "Hello";
std::string strB = "Hello";
if (strA == strB) {
// Strings are equal.
}
This approach helps avoid several common issues associated with C-style strings and makes code more readable.
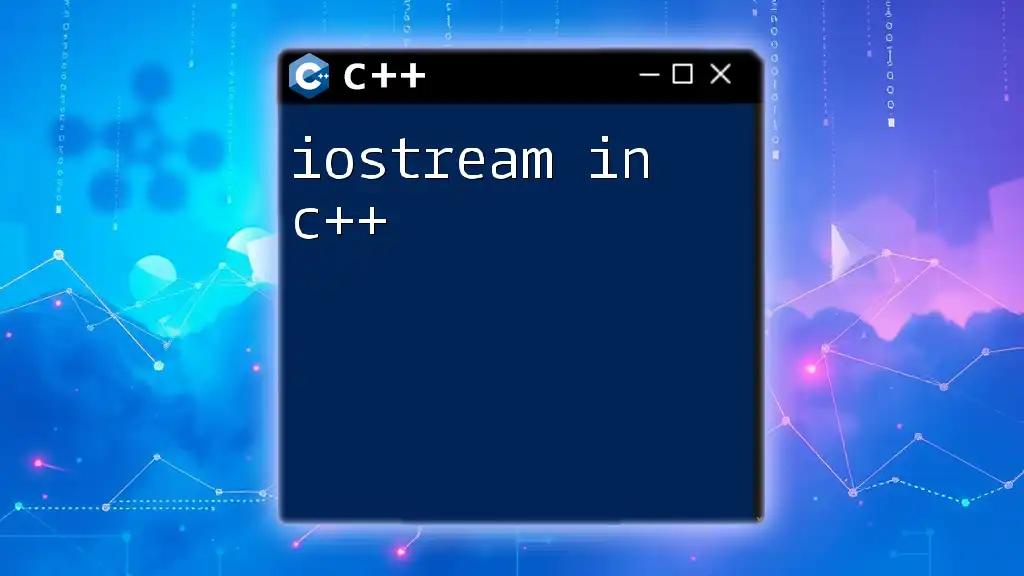
Performance Considerations
When dealing with large datasets or frequent string comparisons, performance can become a critical factor. Using `strncmp` is generally efficient, but be mindful that comparing strings character by character can lead to increased complexity depending on the string sizes and the number of comparisons.
If performance matters, consider the lengths of strings being compared. When comparing a small substring versus a long string, `strncmp` often performs better by limiting the number of comparisons.
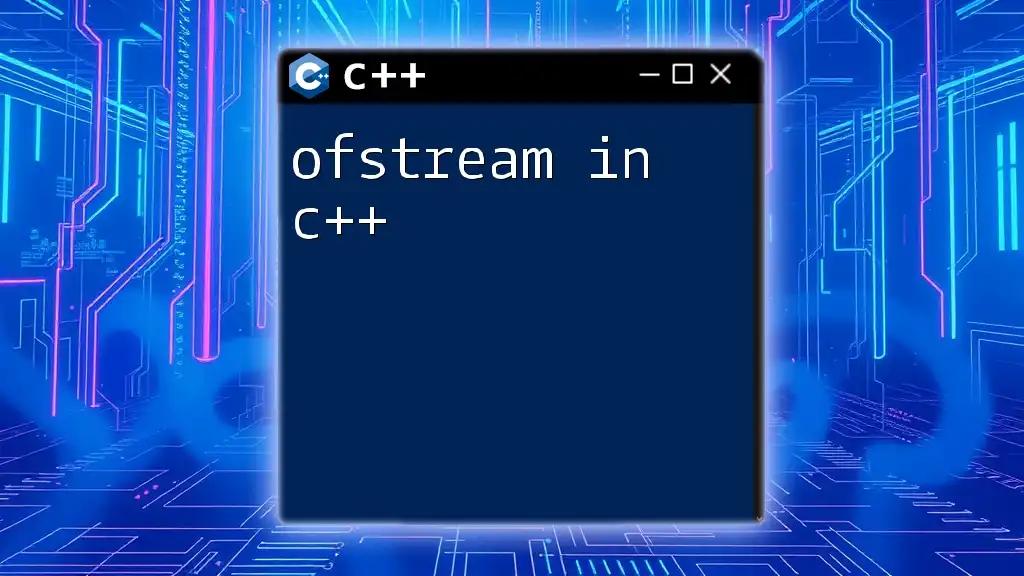
Conclusion
In summary, `strncmp in C++` is an invaluable function for safely comparing portions of C-style strings. Its parameters allow for controlled comparisons, making it useful for various applications ranging from file parsing to user input validation. By understanding its functionality and ensuring correct usage, you can leverage this function to write efficient and safe C++ code.
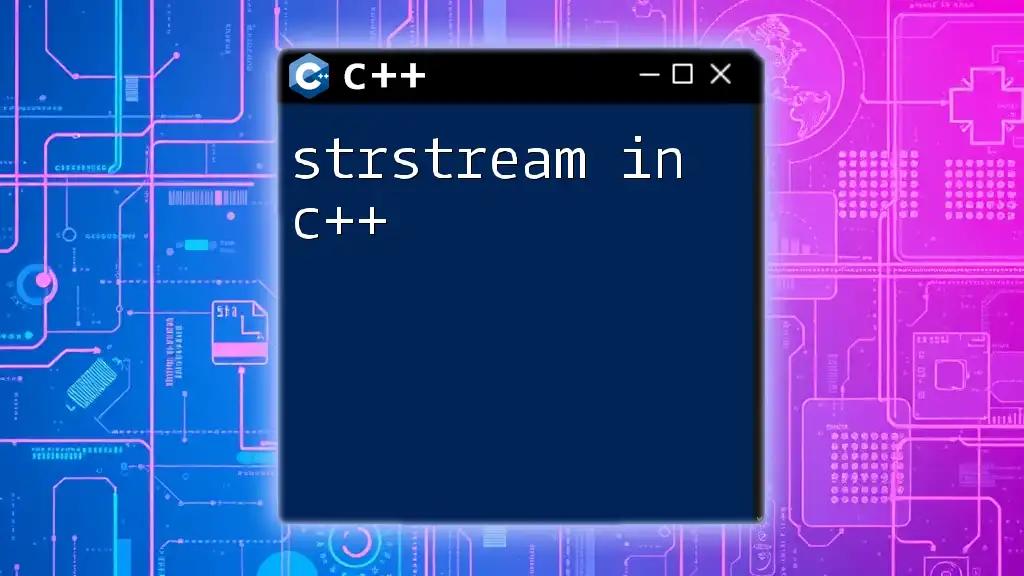
Additional Resources
For further reading, visit the official C++ documentation on `strncmp` and explore additional string handling tutorials and books for a more comprehensive understanding of string manipulation in C++.
Examples Section
Here you can expand with more diverse examples showcasing different scenarios where `strncmp` proves useful, ensuring clarity and practical comprehension for your readers.
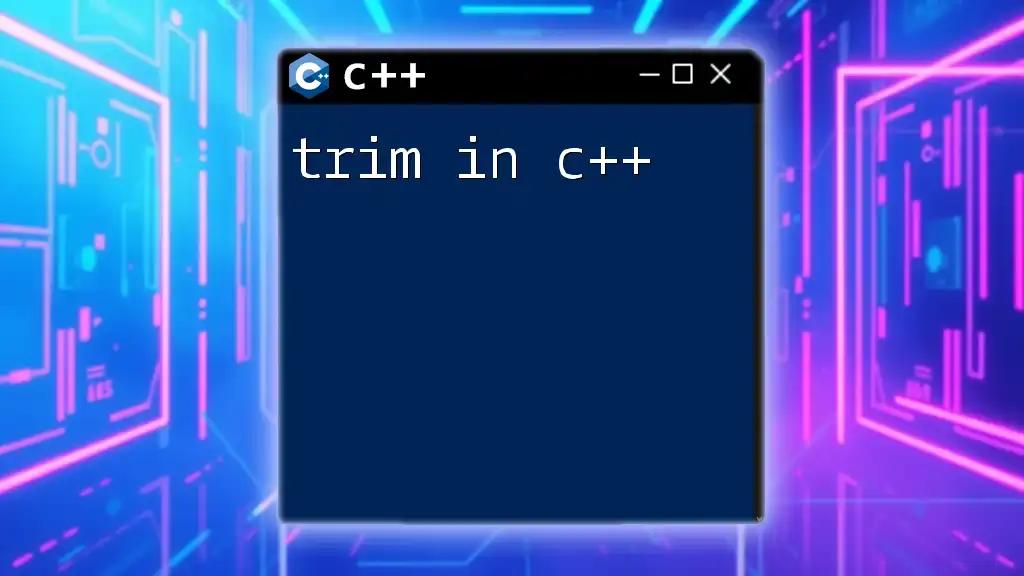
FAQs about strncmp in C++
If you have questions or misconceptions regarding `strncmp`, consider checking out some frequently asked questions. Addressing these queries can deepen understanding and clear up potential misunderstandings.
References
For more advanced studies, consult the C++ standards on string manipulation and explore reliable resources that delve into the nuances of string operations in-depth.