The `trim` function in C++ is commonly used to remove leading and trailing whitespace from a string, ensuring that only the core content remains.
#include <string>
#include <algorithm>
#include <cctype>
std::string trim(const std::string& str) {
auto start = str.begin();
while (start != str.end() && std::isspace(*start)) ++start;
auto end = str.end();
do { --end; } while (end != start && std::isspace(*end));
return std::string(start, end + 1);
}
What is Trimming?
Trimming a string refers to the removal of unwanted leading and trailing whitespace characters, such as spaces, tabs, or newline characters, from a string. Leading spaces appear before the first non-whitespace character, while trailing spaces appear after the last non-whitespace character. Properly trimming strings is essential for ensuring clean data, better user input management, and accurate string comparisons. By removing unnecessary whitespace, developers can streamline data processing and enhance overall application performance.
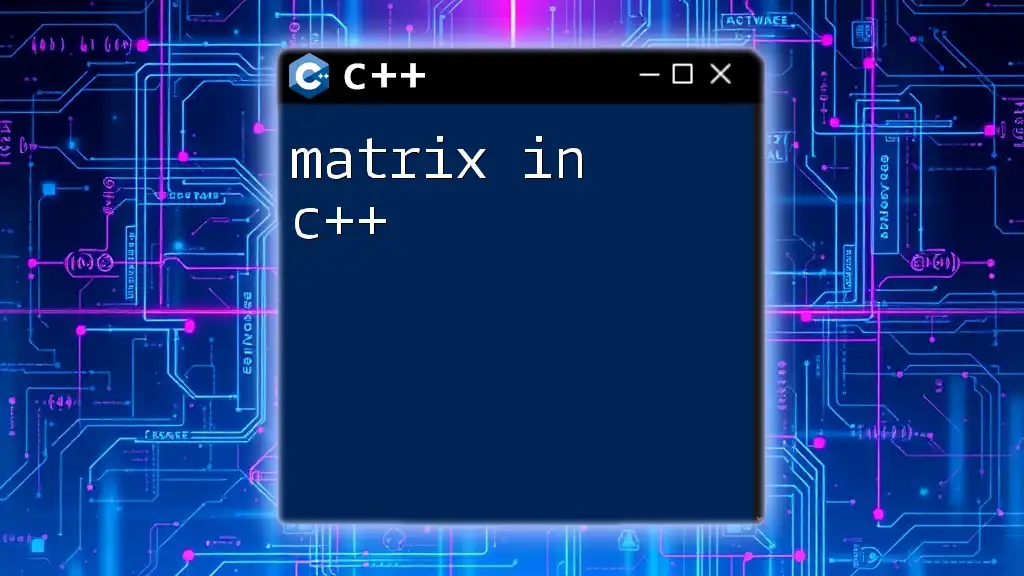
The Need for String Trim in C++
There are several scenarios where string trimming is crucial in C++ programming:
- User Input Validation: When accepting user inputs, such as names or email addresses, it's common for users to unintentionally add spaces, which can lead to unexpected errors when processing data.
- File Processing: When reading data from files, it's not uncommon to encounter extra spaces that could disrupt data integrity when parsing.
- String Comparisons: Performing comparisons between strings with leading or trailing spaces can yield false results, as they are treated as part of the string when making comparisons.
By incorporating string trimming into your C++ applications, you can prevent these issues and significantly improve the accuracy and reliability of your software.
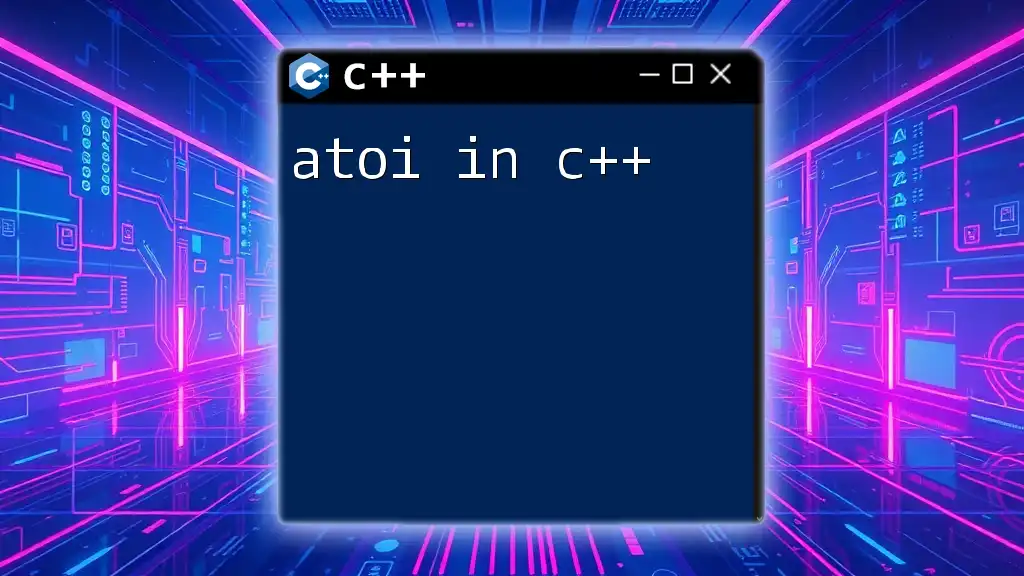
Built-in String Functions in C++
C++ offers a powerful `std::string` class that enables various string manipulations. Understanding the built-in functions available helps in working efficiently with strings. Here’s a quick overview:
- Constructor: To create a string object.
- Length: To find the size of the string with `str.length()`.
- Append: To concatenate strings using `str1 + str2`.
Here’s a simple example of creating and manipulating a string with `std::string`:
std::string example = " Hello World! ";
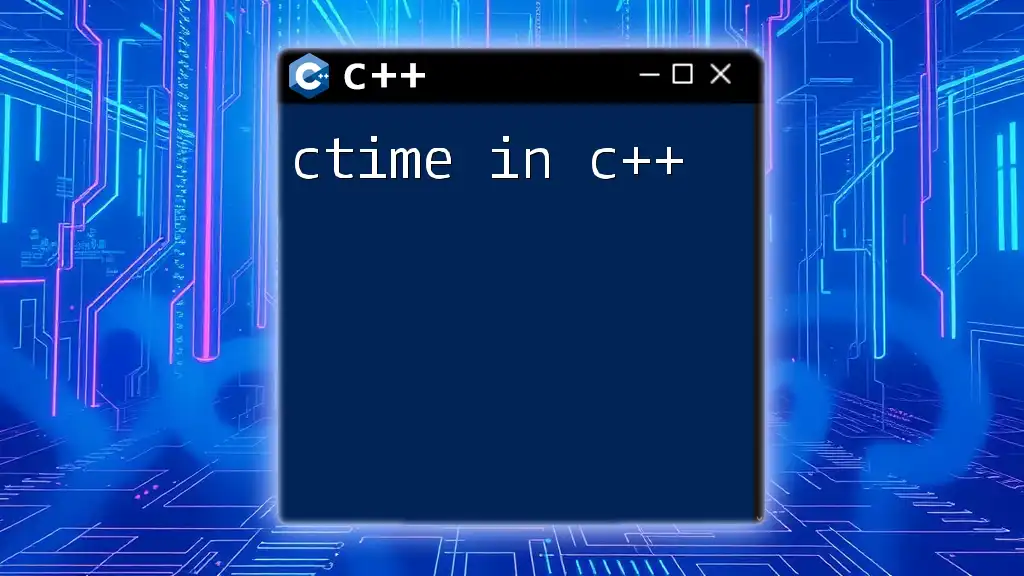
Implementing a C++ Trim Function
While C++ does not provide a built-in function specifically for trimming strings, you can easily create your own. Below is a step-by-step guide to implement trimming functionality.
Trimming Leading Spaces
Leading spaces can be particularly problematic, especially when processing user input. To remove these spaces, create the following function:
std::string trim_left(const std::string &str) {
size_t start = str.find_first_not_of(" \t\n");
return (start == std::string::npos) ? "" : str.substr(start);
}
In this code:
- We use `find_first_not_of` to locate the first character that is not a whitespace.
- If no non-whitespace character is found, an empty string is returned. Otherwise, we return the substring starting from the first non-whitespace character.
Trimming Trailing Spaces
Similarly, trailing spaces can disrupt string processing. Here’s how to trim them using a function:
std::string trim_right(const std::string &str) {
size_t end = str.find_last_not_of(" \t\n");
return (end == std::string::npos) ? "" : str.substr(0, end + 1);
}
This process mirrors the trimming of leading spaces, but we use `find_last_not_of` to find the last non-whitespace character and return the substring leading up to that character.
Trimming Both Leading and Trailing Spaces
Combining both functions, you can create a comprehensive trim function that trims spaces from both ends:
std::string trim(const std::string &str) {
return trim_right(trim_left(str));
}
This function provides a complete solution to trim whitespace from any string.
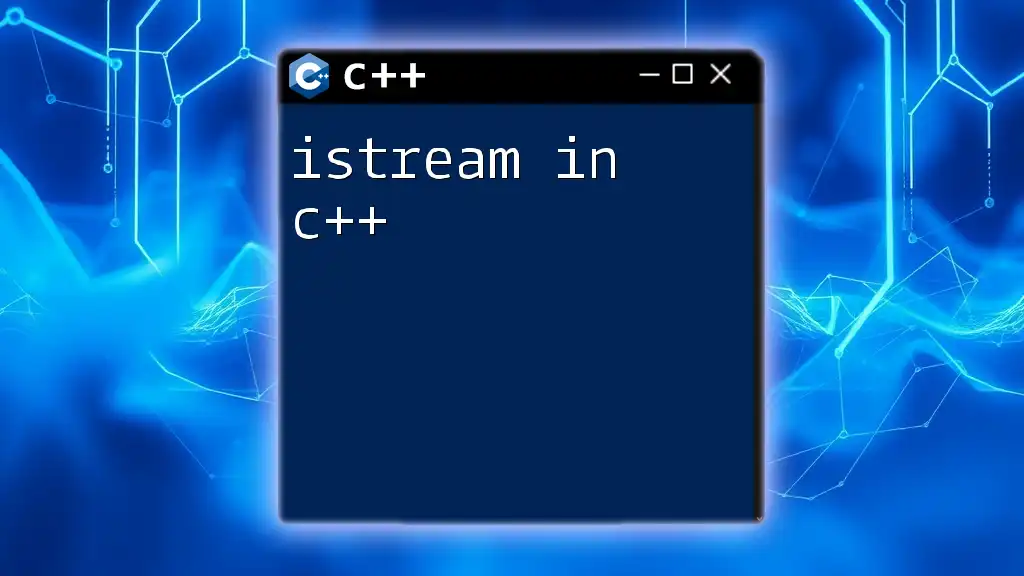
Using Third-Party Libraries for Trimming
While creating your own trim function is straightforward, you can also utilize third-party libraries designed for more extensive string manipulation. One such popular library is Boost.
By leveraging Boost's functionality, you can eliminate the need to write your own trim function. Here’s an example using Boost's `trim` function:
#include <boost/algorithm/string.hpp>
std::string example = " Hello Boost! ";
boost::algorithm::trim(example);
By incorporating Boost, you gain access to a variety of string manipulation tools, increasing your efficiency and potentially reducing errors in your custom implementations.
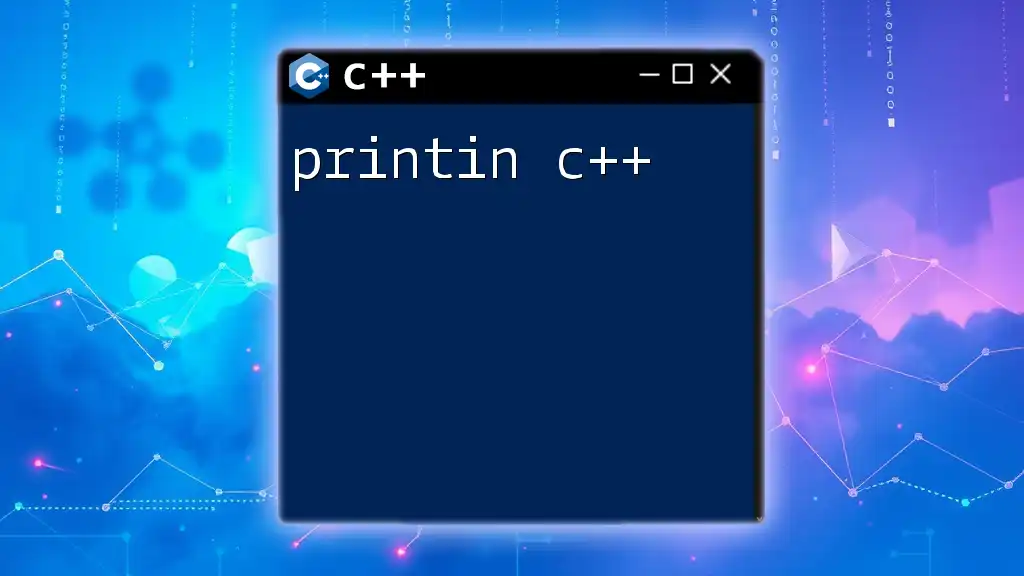
Performance Considerations
When implementing string trimming, it’s essential to consider performance, especially when handling large datasets. The complexity of your trimming functions can affect the overall performance of your application.
Factors to consider include:
- Input Size: Larger strings naturally take longer to process.
- Repeated Trimming: If string trimming is applied frequently on the same input, consider caching the results.
- Use of Libraries: Evaluate the performance implications of third-party libraries versus custom solutions. Benchmarks can help inform your decision.
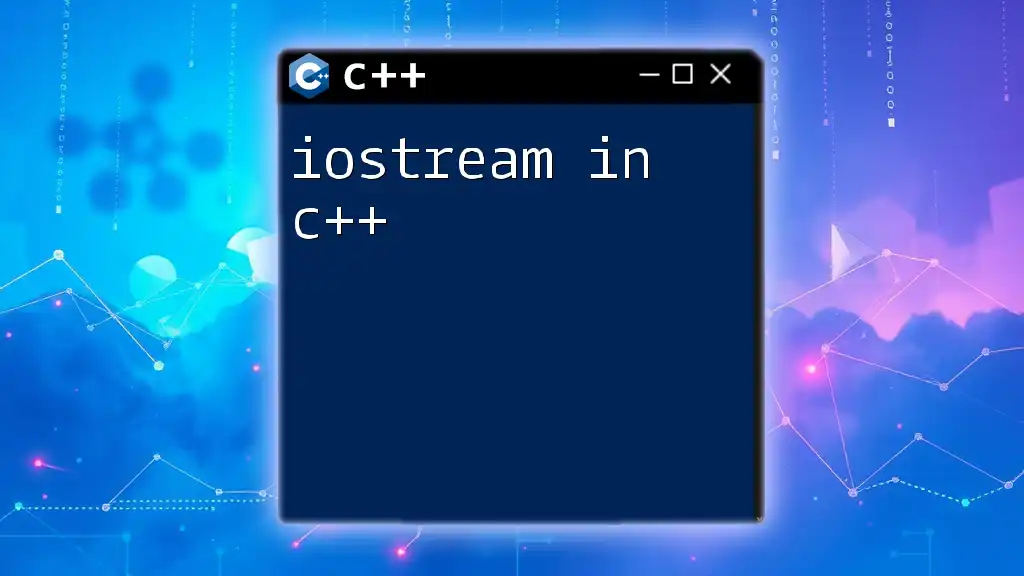
Conclusion
In C++, the importance of trimming strings cannot be overstated. Proper string trimming plays a vital role in maintaining data integrity and ensuring accurate comparisons. By mastering both custom trim functions and leveraging third-party libraries, developers can create robust applications with cleaner data management.
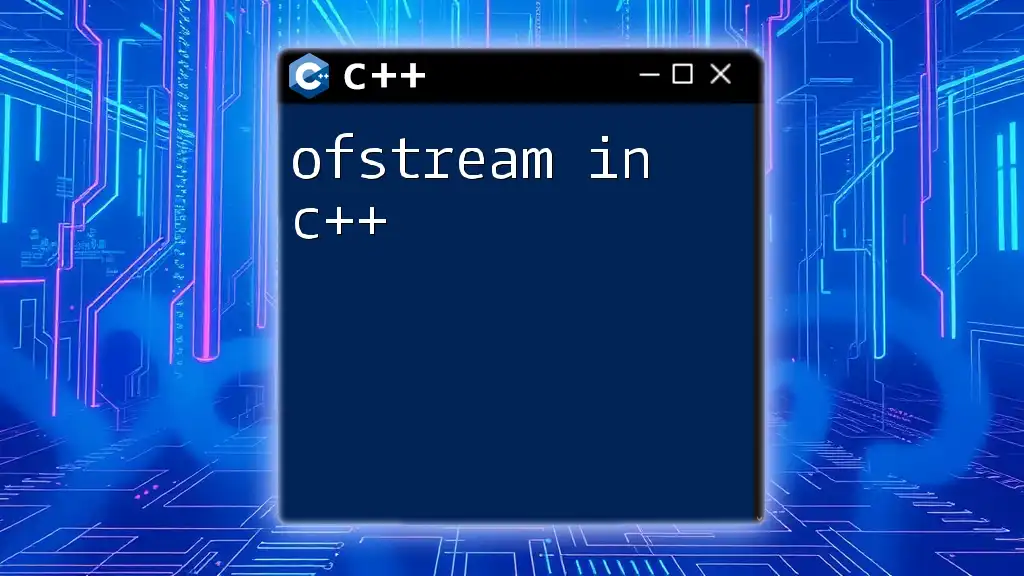
Example Use Cases
Consider a scenario where you ask for user input during runtime:
std::string user_input;
std::cout << "Enter a name: ";
std::getline(std::cin, user_input);
std::string trimmed_input = trim(user_input);
This snippet captures user input and immediately trims any leading or trailing spaces, thus ensuring that you handle the input correctly from the start.
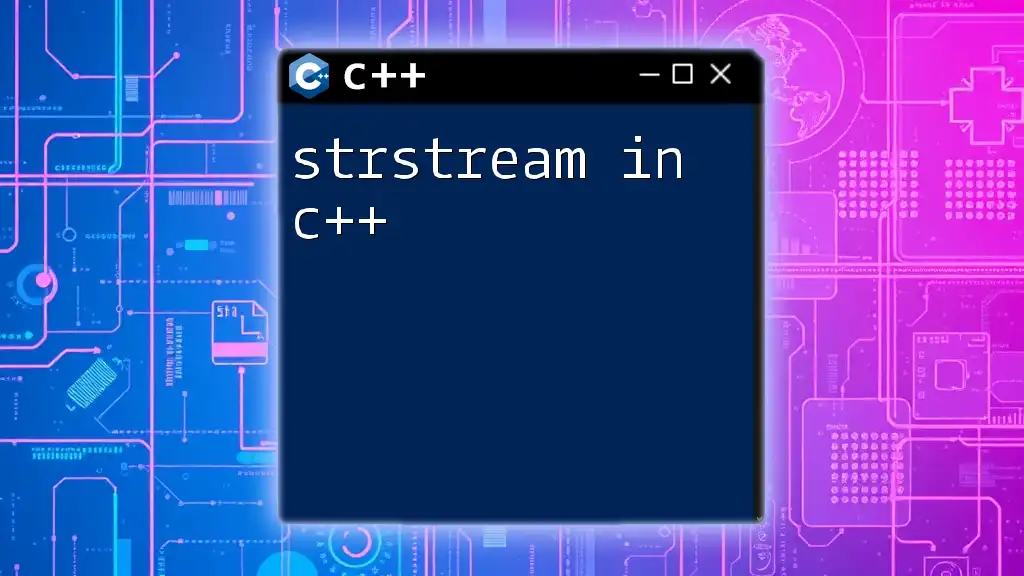
Additional Resources
Explore further readings and resources for expanded knowledge on string manipulation in C++. Access the official C++ documentation, and consider consulting the Boost libraries for advanced functionality.
By implementing string trimming in your applications, you'll cultivate cleaner data handling practices and foster user satisfaction through reliable functionality.