In C++, `nullptr` is a null pointer constant introduced in C++11, representing a pointer that points to no object or function, and it provides a type-safe way to indicate a null pointer compared to the traditional `NULL`.
Here's a simple code snippet demonstrating its use:
#include <iostream>
int main() {
int* ptr = nullptr; // Initialize a null pointer
if (ptr == nullptr) {
std::cout << "The pointer is null." << std::endl;
}
return 0;
}
What is nullptr in C++?
`nullptr` is a keyword introduced in C++11 that represents a null pointer constant. It is designed to make C++ clearer and safer by providing a type-safe way to express a null pointer. Prior to `nullptr`, the values used for null pointers, like `NULL` and `0`, could lead to ambiguity and errors, especially in overloaded function scenarios. Unlike `NULL`, which is typically defined as `0` or `((void*)0)`, `nullptr` has a specific type of `std::nullptr_t`. This distinction prevents unwanted implicit conversions and enhances code readability.
The importance of `nullptr` in modern C++ cannot be overstated. It significantly reduces the chances of bugs caused by pointer mismanagement, providing a clearer intent in the code regarding pointer usage.
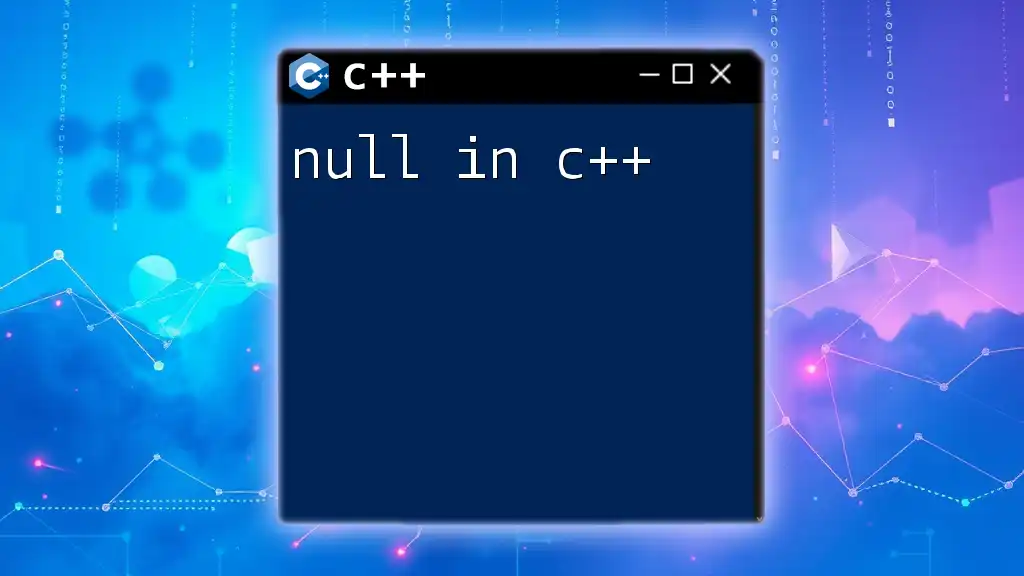
The Evolution of Null Pointers in C++
Historical Context
In earlier versions of C++, programmers commonly used `NULL` or `0` to denote null pointers. However, these approaches had their limitations. Using `NULL` can lead to confusion because it might be defined as `0`, which is also a legitimate integer value. This dual usage can create ambiguous situations, particularly in function overloads where both integer and pointer types might be expected.
Introduction of nullptr
The inclusion of `nullptr` in C++11 was a significant improvement over its predecessors. It brings the following advantages:
-
Type Safety: `nullptr` is of type `std::nullptr_t`, which cannot be implicitly converted to any integer type. This prevents errors that might arise from mistakenly treating a pointer as an integer.
-
Clarity: Using `nullptr` makes it explicit to the reader that a pointer is being referenced, enhancing the readability and maintainability of the code.
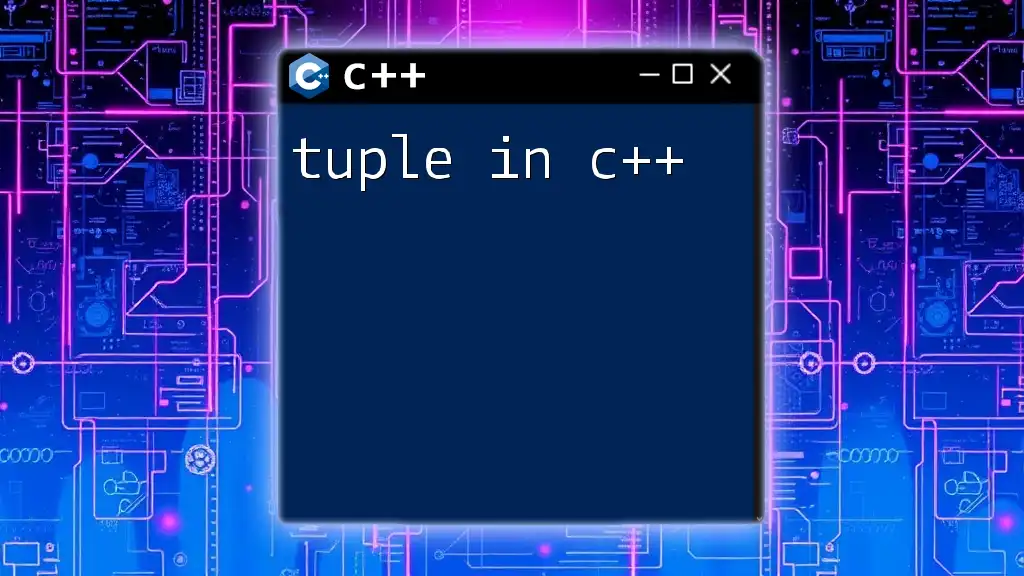
Syntax and Usage of nullptr
Declaring and using `nullptr` is straightforward. To declare a pointer and initialize it to null, you simply write:
int* ptr = nullptr;
Examples of Initializing Pointers with nullptr
Here is an illustrative example of how to correctly use `nullptr`:
void function(int* p) {
if (p == nullptr) {
// Handle null pointer case
}
}
int main() {
int* p1 = nullptr; // Correctly initializes pointer to null
function(p1); // Function called with nullptr
}
This code demonstrates the use of `nullptr` in a real function, clearly indicating that `p1` is not currently pointing to a valid memory address.
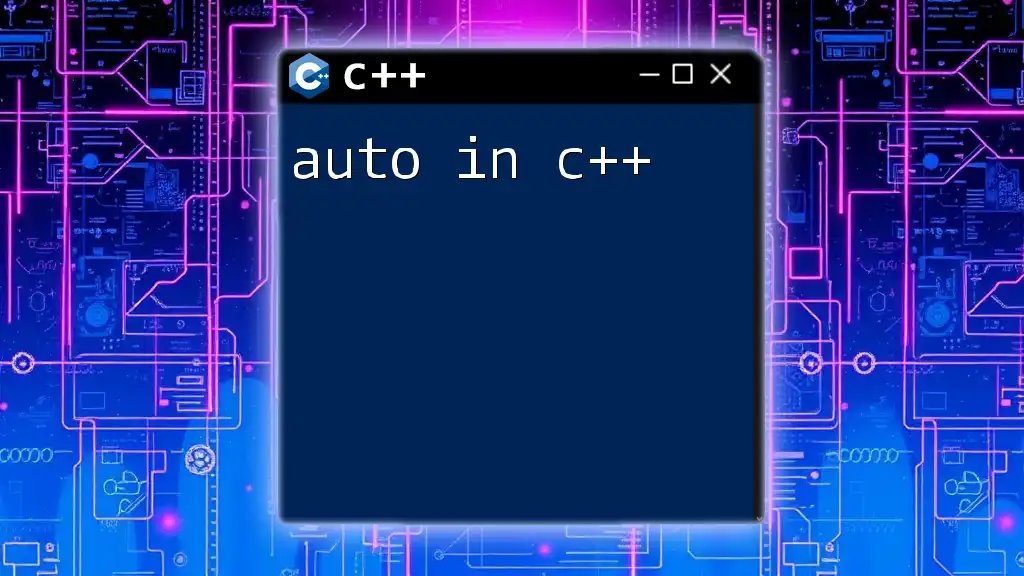
nullptr vs NULL and 0
Differences Between nullptr, NULL, and 0
Understanding the differences is crucial for effective programming in C++. Here’s a breakdown:
-
Type Safety: As previously mentioned, `nullptr` provides type safety. `NULL` and `0` can be interpreted as integers, which leads to ambiguity in overload resolution.
-
Performance Implications: Using `nullptr` can help the compiler resolve the correct overloaded version of a function, potentially improving compile-time optimization.
-
Use Cases: Use `nullptr` in modern codebases and C++11 onward. Prefer using `NULL` or `0` only in legacy code that hasn't transitioned to C++11.
Practical Examples
To further illustrate:
int* p1 = NULL; // Legacy C-style. Can cause confusion.
int* p2 = 0; // Ambiguous: could mean a null pointer or integer.
int* p3 = nullptr; // Clear and type-safe.
In the example, `p3`, initialized with `nullptr`, is easy to identify as a null pointer, clearly conveying intent.
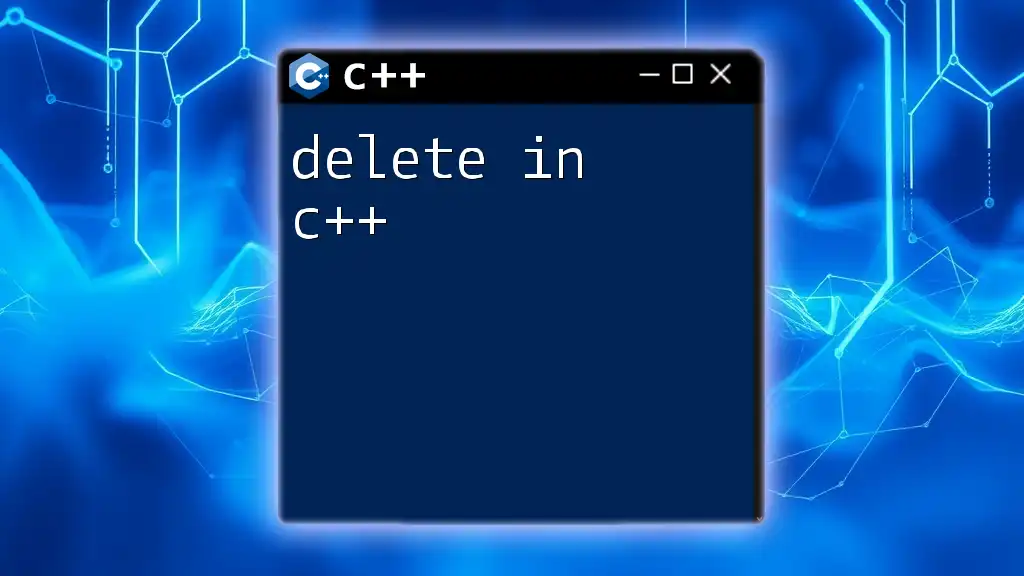
Common Mistakes When Using nullptr
Despite its advantages, developers can still make errors in using `nullptr`. Some common mistakes include:
-
Misconceptions about nullptr: Some programmers may incorrectly treat `nullptr` as equivalent to a zero value in expressions involving numbers.
-
Incorrect Comparisons: Comparing `nullptr` with integer types can lead to subtle bugs, as the implications of such comparisons can be misleading.
-
Assigning nullptr to Non-pointer Types: Using `nullptr` where an integer or function type is expected can result in compiler errors, as `nullptr` is specifically for pointer context.
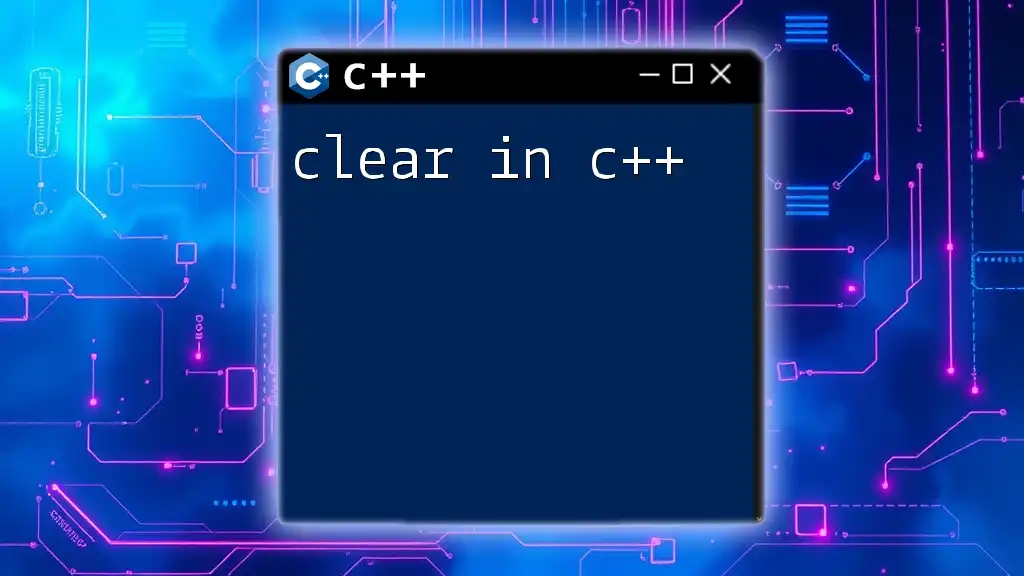
Best Practices for Using nullptr in C++
To maximize the safety and effectiveness of `nullptr`, consider these best practices:
-
When to Use nullptr: Always prefer `nullptr` when initializing, comparing, or working with pointers in modern C++.
-
Code Clarity: Using `nullptr` enhances code readability, making it evident to future readers that you are dealing with pointers.
-
Smart Pointers Integration: When working with smart pointers like `std::unique_ptr` or `std::shared_ptr`, initializing with `nullptr` sets up a valid base state for these pointers.
std::unique_ptr<int> up(nullptr); // Initializes a unique pointer to nullptr
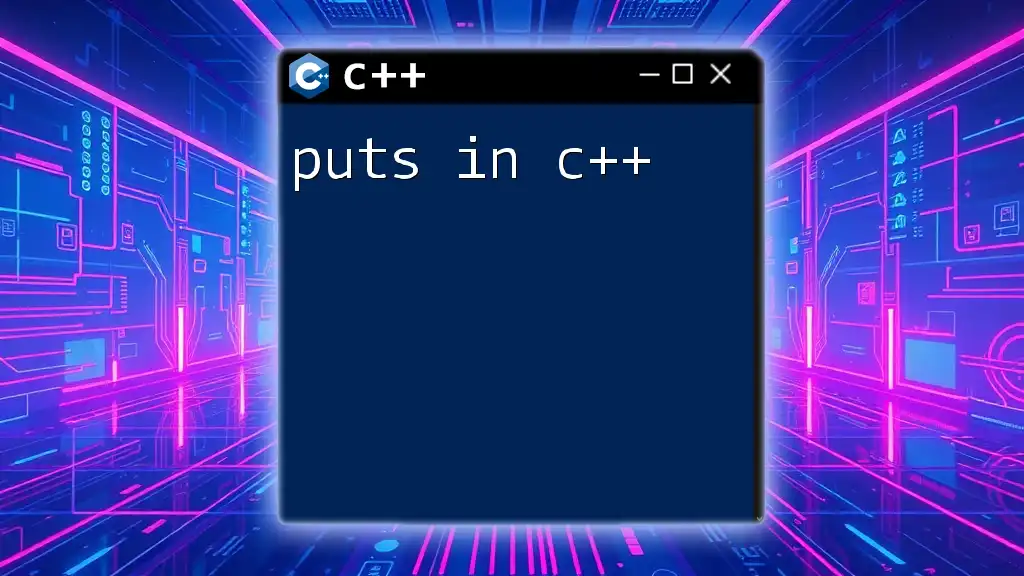
Advanced Usage of nullptr
Overloading Functions with nullptr
Understanding how `nullptr` behaves in function overloads is essential. It allows for clearer function resolution, as seen in this example:
void func(int* p);
void func(char* p);
func(nullptr); // Calls func(int*), not ambiguous
In this case, `nullptr` indicates a preference for the pointer of type `int*`, demonstrating the clarity it brings to function calls.
Template Programming and nullptr
When using templates, `nullptr` can play a crucial role in specialization, making code more robust:
template<typename T>
void process(T* ptr) {
if (ptr == nullptr) {
// Handle nullptr case
}
}
Here, `process` clearly accepts a pointer of any type, and `nullptr` allows for safe and explicit checks against null.
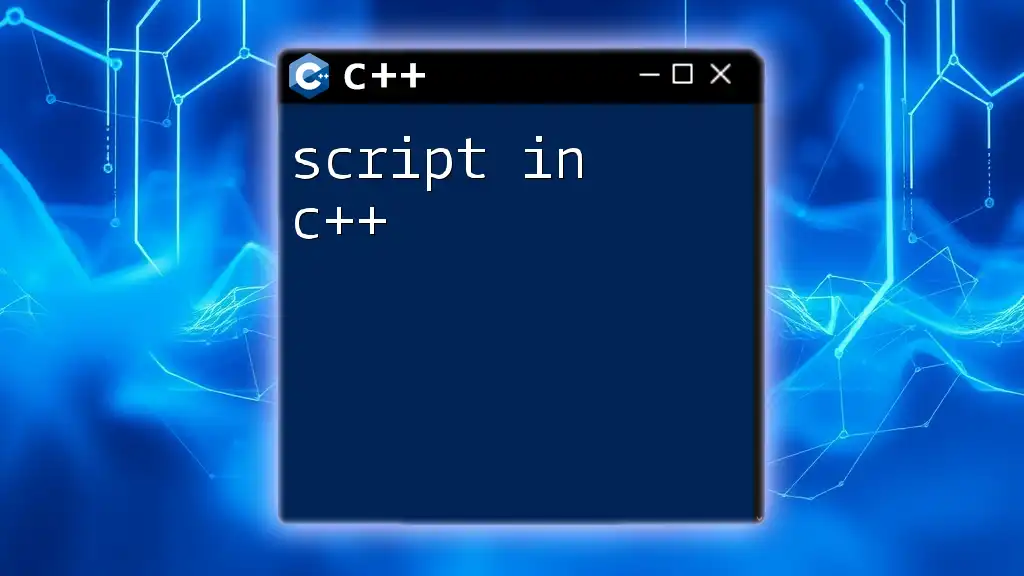
Debugging with nullptr
Debugging null pointer dereferences can be challenging. Here are some techniques to effectively manage `nullptr`-related issues:
-
Use Assertions: Incorporate assertions to validate pointer status at critical points in the code.
-
Debugging Tools: Utilize tools like Valgrind or AddressSanitizer, which help detect memory misuse, including null pointer dereferencing.
-
Build Contextual Help: Maintain documentation or comments around complex pointer logic to aid debugging and future maintenance.
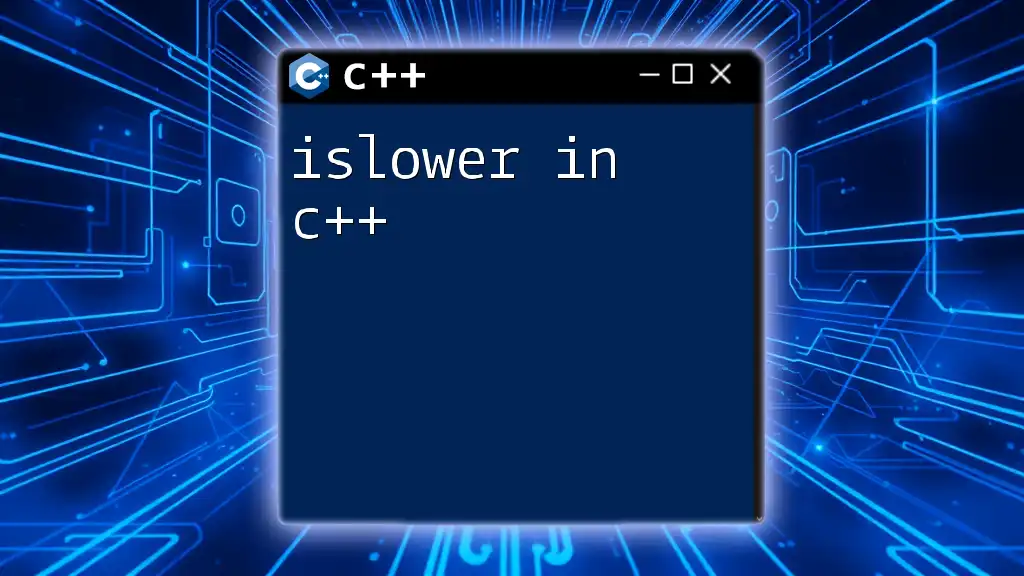
Conclusion
In summary, `nullptr in C++` is a significant advancement that enhances code clarity and type safety. By adopting `nullptr`, developers can write more maintainable and error-free code. Transitioning to `nullptr` from legacy definitions like `NULL` or `0` represents a best practice that all C++ programmers should embrace.
By understanding and implementing the principles of `nullptr` into your programming practices, you'll not only improve your code's integrity but also hone your skills as a C++ developer, leading to better coding standards in the C++ community.
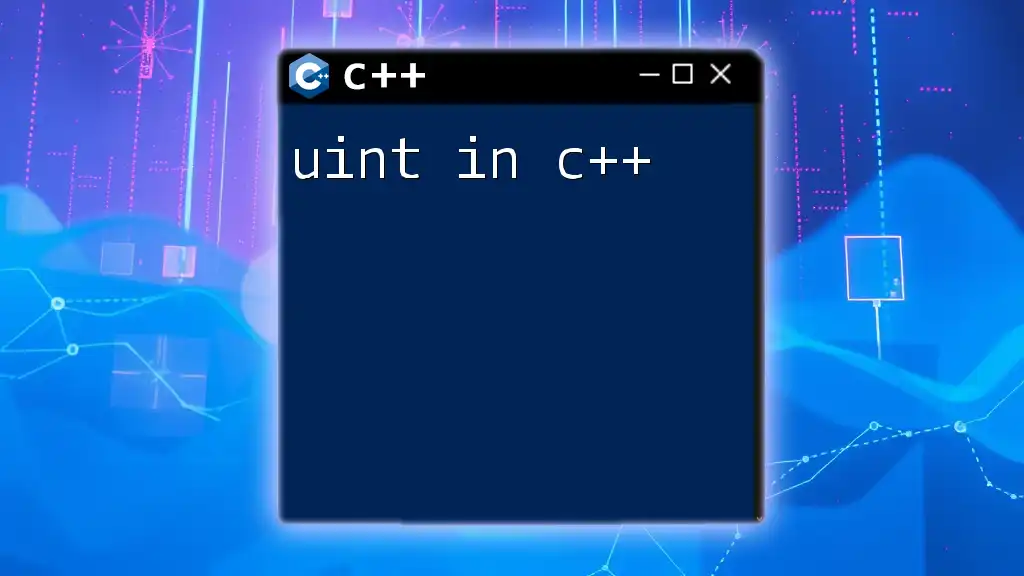
Additional Resources
For those looking to deepen their understanding of `nullptr` and general C++ programming, consider exploring the following resources:
- Recommended books such as "Effective Modern C++" by Scott Meyers.
- Online courses available on platforms like Coursera or Udemy.
- Community forums like Stack Overflow for ongoing discussions and problem-solving.
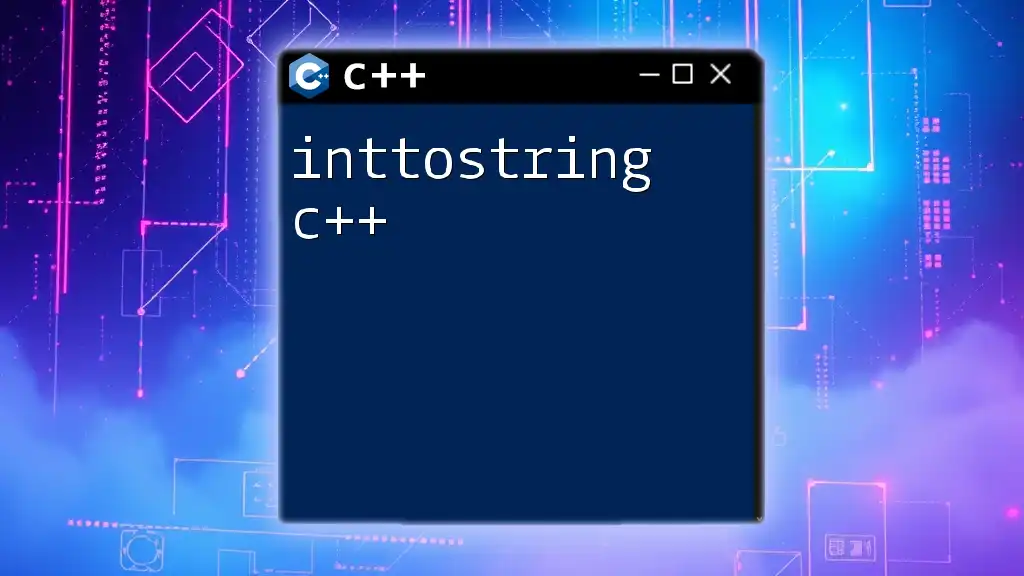
Call to Action
As you embark on your journey with C++, consider subscribing to our tutorials and workshops to enhance your skills in using `nullptr` effectively. Share your experiences and questions about `nullptr` in C++, and together, let's elevate the standards of modern C++ programming!