The `noexcept` specifier in C++ indicates that a function does not throw exceptions, allowing for performance optimizations and stronger exception safety guarantees.
#include <iostream>
void safeFunction() noexcept {
// This function guarantees not to throw exceptions
std::cout << "This function will not throw exceptions.\n";
}
What is noexcept in C++?
`noexcept` is a specifier in C++ that indicates whether a function is allowed to throw exceptions or not. By declaring a function with `noexcept`, you inform the compiler and developers that this function will never throw exceptions during its execution. This feature was introduced in C++11 and has become a vital part of the language, allowing for better performance optimization by the compiler.
Using `noexcept` has significant implications for both performance and the intended behavior of your functions. By clearly stating the exception guarantee, you ensure that certain operations can be performed safely without worrying about unexpected exceptions.

Why Use noexcept?
Improving Performance
One of the primary reasons to utilize `noexcept` is to enhance the performance of your code. When the compiler knows that a function is `noexcept`, it can optimize the generated code more effectively. For example, standard library containers may optimize operations like move semantics when using `noexcept`:
#include <iostream>
#include <vector>
struct NoThrow {
NoThrow() = default;
NoThrow(NoThrow&&) noexcept { std::cout << "Moved NoThrow\n"; }
NoThrow(const NoThrow&) = delete; // Prevent copying
};
int main() {
std::vector<NoThrow> vec;
vec.push_back(NoThrow()); // Can move because of noexcept
}
In this example, if `NoThrow` had a throw-specification, the vector might not use move semantics, leading to unnecessary performance overhead. Thus, declaring it `noexcept` allows for safer and faster code.
Making Intentions Clear
The `noexcept` specifier also provides clarity regarding the function's behavior. This explicit directive informs other developers that they don't need to handle exceptions when calling such functions, which can improve code readability and maintainability.
As an example, consider the following function declaration:
void processData() noexcept {
// Implementation
}
With `noexcept`, anyone reading the code understands that this function is guaranteed not to throw exceptions, which simplifies the error handling strategy.

How to Use noexcept
Defining a Function with noexcept
To define a function as `noexcept`, you can simply append the `noexcept` keyword to the function's signature. Here’s how you do it:
void safeFunction() noexcept {
// Implementation that guarantees no exceptions will be thrown
}
This declaration assures the users of your code that this function will execute without throwing exceptions under any circumstance.
Conditional noexcept
C++ allows for conditional use of `noexcept`, which provides flexibility in situations where the exception behavior depends on the function's input types. This is particularly useful when templating and when dealing with types that may or may not throw exceptions.
Here’s the syntax:
template<typename T>
void conditionalFunction(T param) noexcept(std::is_nothrow_move_constructible<T>::value) {
// Implementation dependent on whether T is noexcept move constructible
}
Example of Conditional noexcept
Consider a scenario where a function’s safety relies on the type being passed. If we have a class `Movable` which is not noexcept-constructible, we can use the conditional `noexcept` to handle the scenarios:
#include <type_traits>
struct PossiblyThrow {
// Constructor that might throw
PossiblyThrow() {}
};
template<typename T>
void process(T obj) noexcept(std::is_nothrow_move_constructible<T>::value) {
// Implementation
}
In this example, if `T` is known to be `noexcept`, the function guarantees not to throw, enhancing safety and performance characteristics.

Understanding noexcept and Exceptions
Difference between noexcept and try/catch
It's crucial to articulate the distinction between `noexcept` and traditional exception handling mechanisms like `try` and `catch`. The `noexcept` specifier does not actually catch exceptions; rather, it gives a guarantee about the function's behavior. When an exception is thrown from a `noexcept` function, the program will call `std::terminate()` instead of returning to the caller, effectively making your program immediately crash.
Here’s an example that demonstrates this behavior:
void mayThrow() noexcept {
throw std::runtime_error("This will terminate the program!");
}
int main() {
try {
mayThrow(); // Results in std::terminate being called
} catch (...) {
std::cout << "Caught exception!" << std::endl;
}
}
In this case, because `mayThrow()` is defined as `noexcept`, it results in a program termination rather than catching the exception.
Impact on Stack Unwinding
One significant consequence of using `noexcept` is how it interacts with stack unwinding. If an exception is thrown in a `noexcept` function, the stack is not unwound. Instead, the program is terminated, letting no destructors execute. This means you need to be very cautious when using `noexcept`.
For instance, if you call another function from within a `noexcept` function and that function throws, it leads to an immediate call to `std::terminate()`.
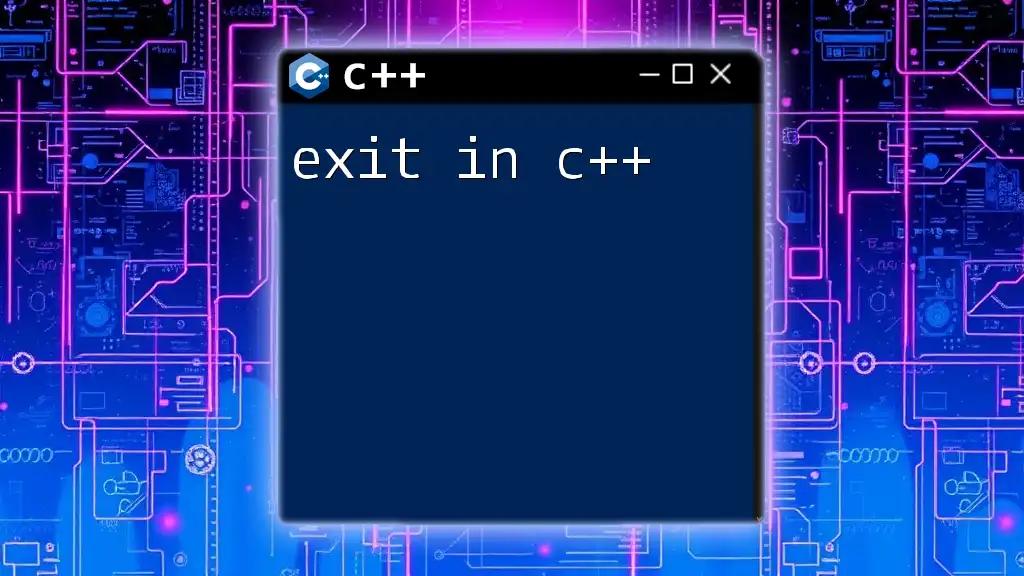
Best Practices for Using noexcept
When to Use noexcept
Use `noexcept` when:
- You believe the function will never throw exceptions.
- You want to enhance performance, especially with classes and types known for `noexcept` behavior.
- You want to clarify your code’s intent to other developers.
By using `noexcept` judiciously, you establish a reliable contract about your function’s behavior, which ultimately results in cleaner code.
Avoiding Pitfalls
Be careful not to use `noexcept` indiscriminately. If you declare a function as `noexcept` and it throws, it will result in abrupt program termination. Always ensure that the function's implementation genuinely respects the no-throw promise.
For example, consider this incorrect use of `noexcept`:
void riskyFunction() noexcept {
throw std::out_of_range("This is not allowed!"); // Violates noexcept
}
This code will not work as intended. Always verify that exceptions are not thrown from `noexcept` functions, and remember that destructors should generally not be marked `noexcept` unless they genuinely comply with the contract.

Tools and Techniques
Static Analysis
Several static analysis tools can assist you in verifying the use of `noexcept`. Tools like Clang-Tidy can inspect your code and warn you about improper use cases involving exception specifications. Emphasizing the use of these tools will help maintain clean and efficient code.
Testing noexcept Behavior
Testing functions that use `noexcept` effectively requires a careful approach. A common practice is to structure your tests such that they methodically cover paths that would potentially trigger an exception.
Here's a simple example using a testing framework to ensure that a function behaves as expected:
#include <cassert>
void testFunction() noexcept {
// Perform tests without throwing
// No exceptions should occur
}
int main() {
testFunction(); // Should complete without exceptions
return 0;
}
Make sure to integrate robust testing in your codebase, specifically for functions designated `noexcept`, ensuring that they stay true to their declaration.

Conclusion
In summary, `noexcept` in C++ is a powerful tool that can enhance your code by providing performance benefits and clearer interfaces. However, it also comes with responsibilities and potential pitfalls. Clearly stating that a function will not throw exceptions can improve your program's reliability and performance, especially when used in conjunction with types that meet these guarantees. Always practice due diligence and rigorously test your `noexcept` implementations to make the most of this language feature. With the right approach, `noexcept` can make your C++ code more robust and efficient.

Additional Resources
For those looking to deepen their understanding of exception handling in C++, the official C++ documentation is an excellent resource. It provides comprehensive insights and guidelines regarding exception specifications and the proper use of `noexcept`.