The `set_intersection` function in C++ computes the intersection of two sorted ranges and stores the result in a specified output iterator.
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3, 4, 5};
std::vector<int> vec2 = {3, 4, 5, 6, 7};
std::vector<int> intersection;
std::set_intersection(vec1.begin(), vec1.end(),
vec2.begin(), vec2.end(),
std::back_inserter(intersection));
for (int i : intersection) {
std::cout << i << ' '; // Output: 3 4 5
}
return 0;
}
Understanding Sets in C++
What is a Set?
A set is a data structure that stores a collection of unique elements. Unlike arrays or vectors, which can contain duplicate items, sets automatically handle duplicates by ignoring them. This makes sets particularly useful in situations where you want to ensure that each element appears only once.
Advantages of Using Sets
Sets offer several advantages:
- Unique elements: No duplicates allowed, ensuring data integrity.
- Automatic sorting: Elements are stored in a sorted order.
- Efficient operations: Common operations like search, insert, and delete are performed in logarithmic time due to their underlying data structure (usually a balanced binary tree).
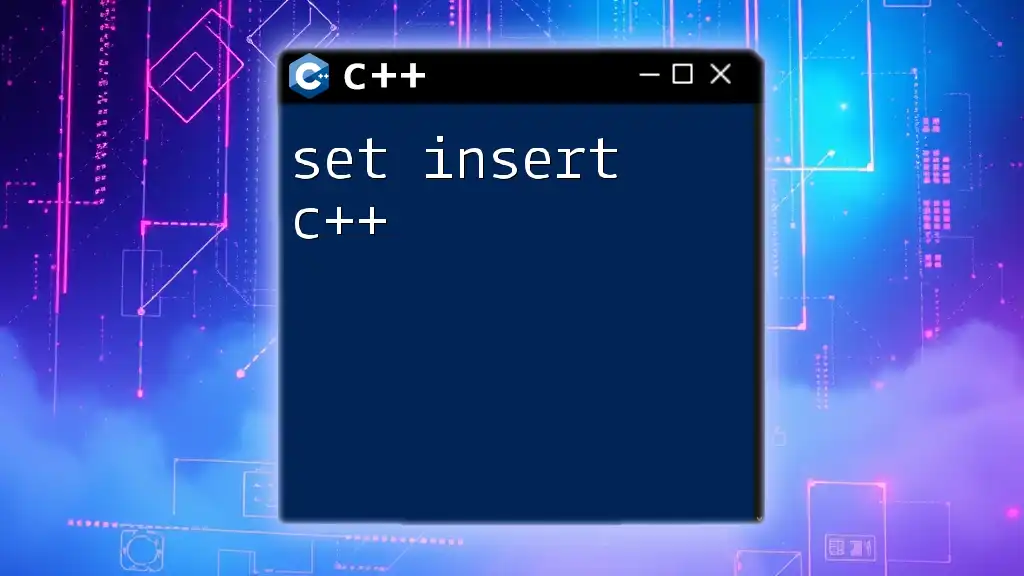
The C++ Standard Library and Sets
Overview of STL (Standard Template Library)
The Standard Template Library (STL) is a powerful set of C++ template classes and functions that provide commonly used data structures and algorithms. Sets are one of the many containers included within the STL, specifically designed for efficient handling of unique collections of elements.
Set Header File
To use sets in C++, you need to include the appropriate header file at the beginning of your code:
#include <iostream>
#include <set>
This inclusion gives access to the `std::set` and related functionalities, including `set_intersection`.
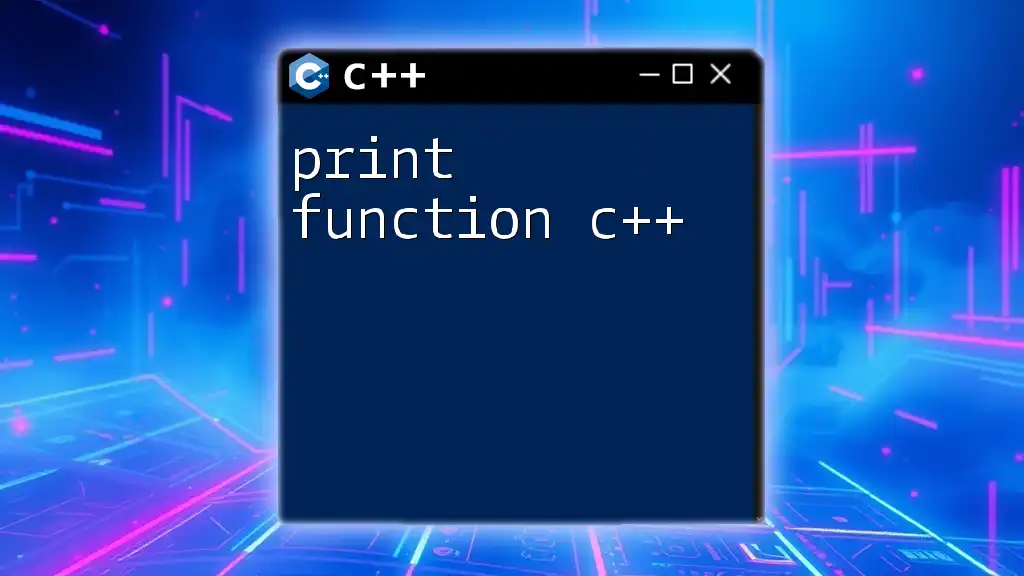
What is `set_intersection`?
Definition
The `set_intersection` function is a part of the STL algorithms that computes the intersection of two sorted ranges. In simpler terms, it identifies the elements that are common to both sets. Understanding this function is critical in scenarios where you need to analyze overlapping data.
Syntax
The syntax of the `set_intersection` function is as follows:
std::set_intersection(first1, last1, first2, last2, d_first);
The parameters work as follows:
- `first1`, `last1`: The range of the first set.
- `first2`, `last2`: The range of the second set.
- `d_first`: The beginning of the destination range where the intersection will be stored.
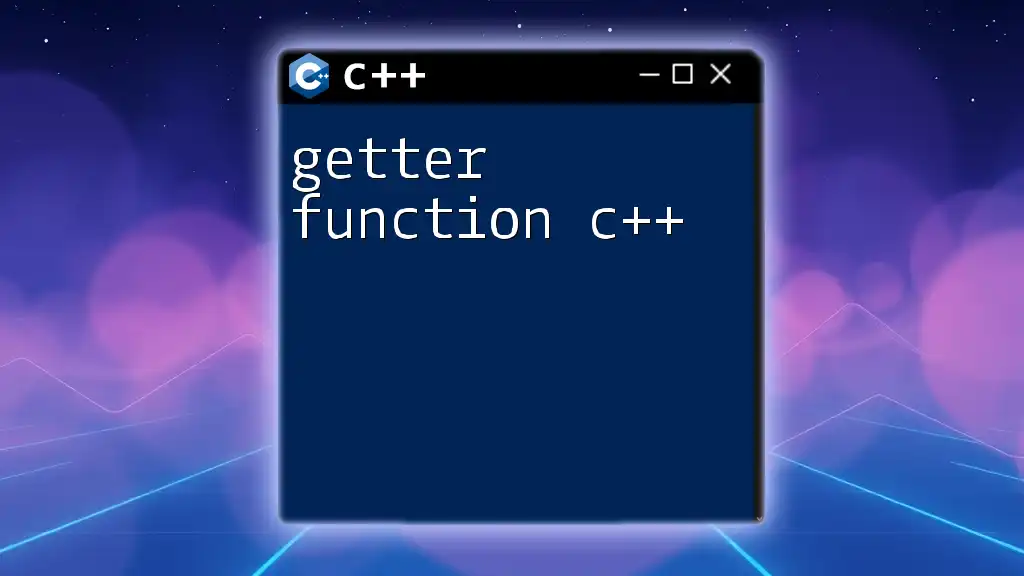
How to Use `set_intersection` in C++
Example: Basic Set Intersection
To perform a basic set intersection, you first need to create two sets. Here’s a simple example:
std::set<int> set1 = {1, 2, 3, 4, 5};
std::set<int> set2 = {3, 4, 5, 6, 7};
In this case, we have two sets containing integer values.
Steps to Perform Set Intersection
Now, let's execute the intersection of the two sets. You will need an output container to store the result:
std::vector<int> result;
std::set_intersection(set1.begin(), set1.end(), set2.begin(), set2.end(),
std::back_inserter(result));
This code calls `set_intersection` and inserts the result into the `result` vector.
Output of the Intersection
To display the resulting intersection, you can iterate through the `result` vector:
for (int i : result) {
std::cout << i << " ";
}
// Output: 3 4 5
This output confirms that the intersection of `set1` and `set2` includes the elements 3, 4, and 5.
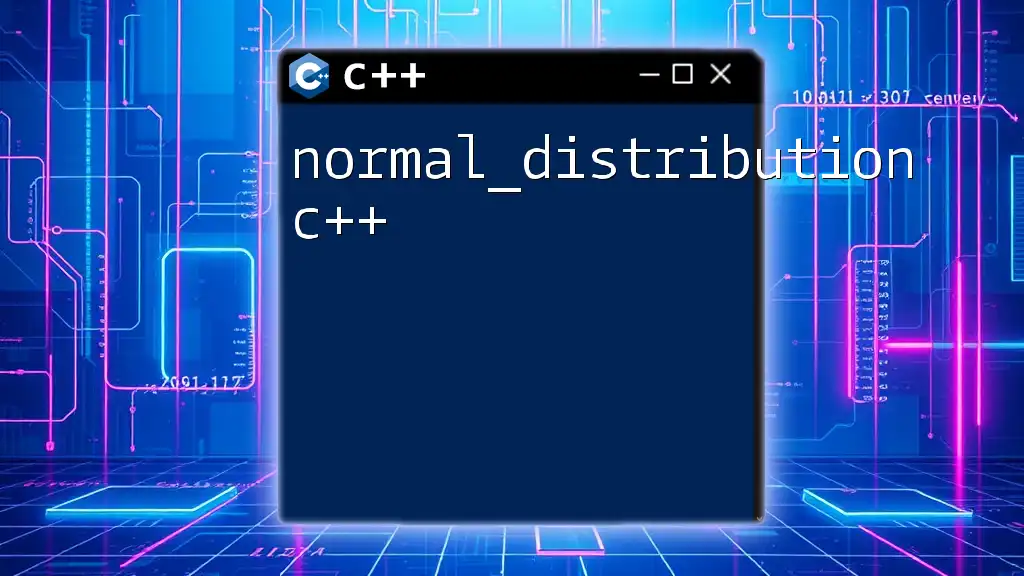
Detailed Breakdown of Parameters
Input Parameters
The input parameters (`first1`, `last1`, `first2`, `last2`) specify the ranges of the sets on which the intersection will be performed. Using iterators, you can define where the elements begin and end. This allows for flexibility in the operation.
Output Parameters
The output parameter `d_first` determines where the results will be stored. It’s crucial to ensure that this destination is of an adequate type (like a vector or another set) to capture the intersection results.
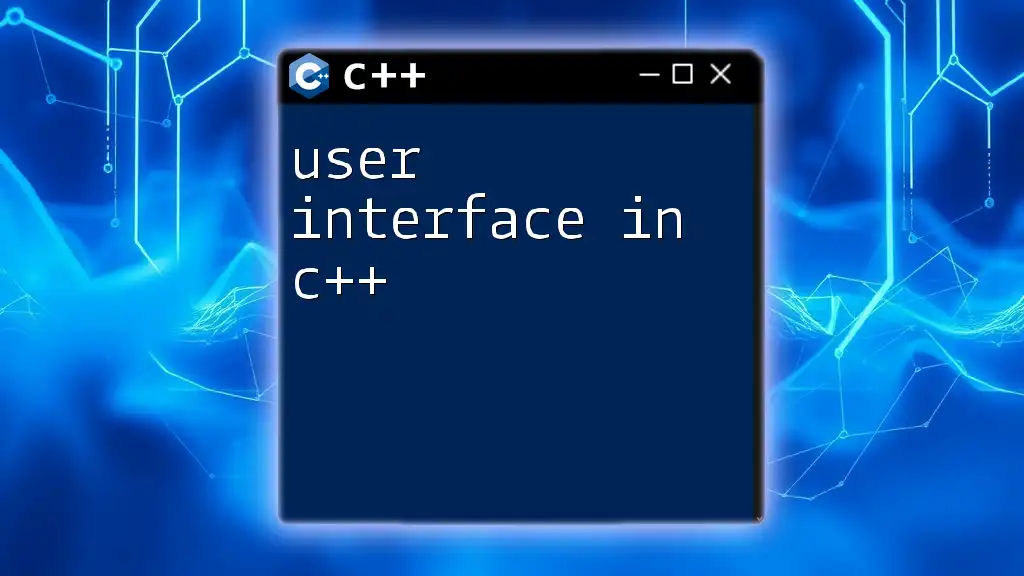
Practical Applications of `set_intersection`
Use Cases
The `set_intersection c++` function has numerous real-world applications:
- Data analysis: It can be used to identify common values in datasets, such as overlapping products in inventory systems.
- Social networking: This function is useful for finding mutual friends or shared interests when comparing user profiles.
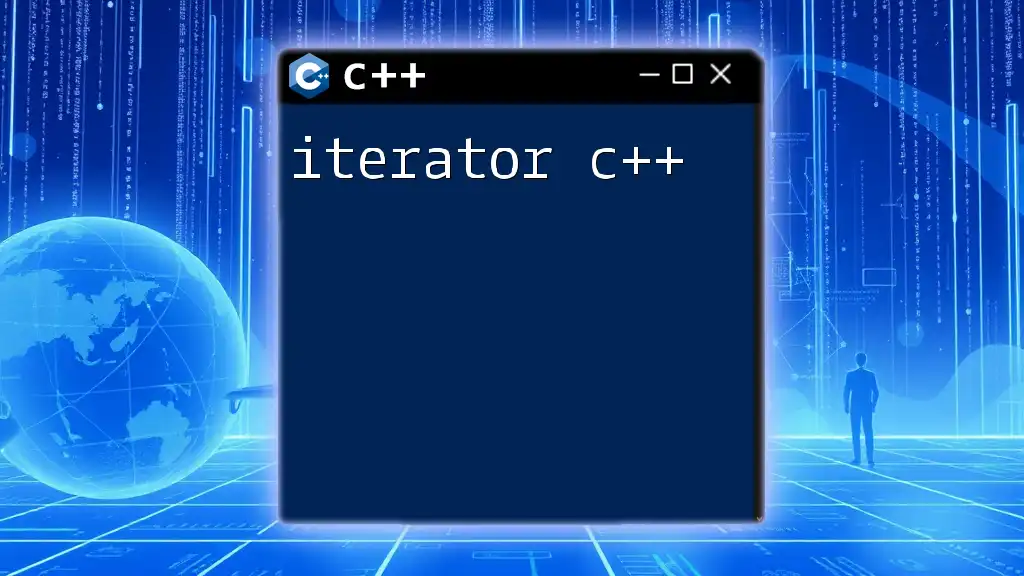
Performance Considerations
Time Complexity
The time complexity of the `set_intersection` function is O(n + m), where n and m are the sizes of the two sets. This efficiency stems from the fact that both sets must be traversed, but comparisons are done in logarithm time due to their sorted nature.
Best Practices
To optimize performance when using `set_intersection`, ensure that both sets are sorted prior to performing this operation. This guarantees that the algorithm runs efficiently without unnecessary sorting overhead.
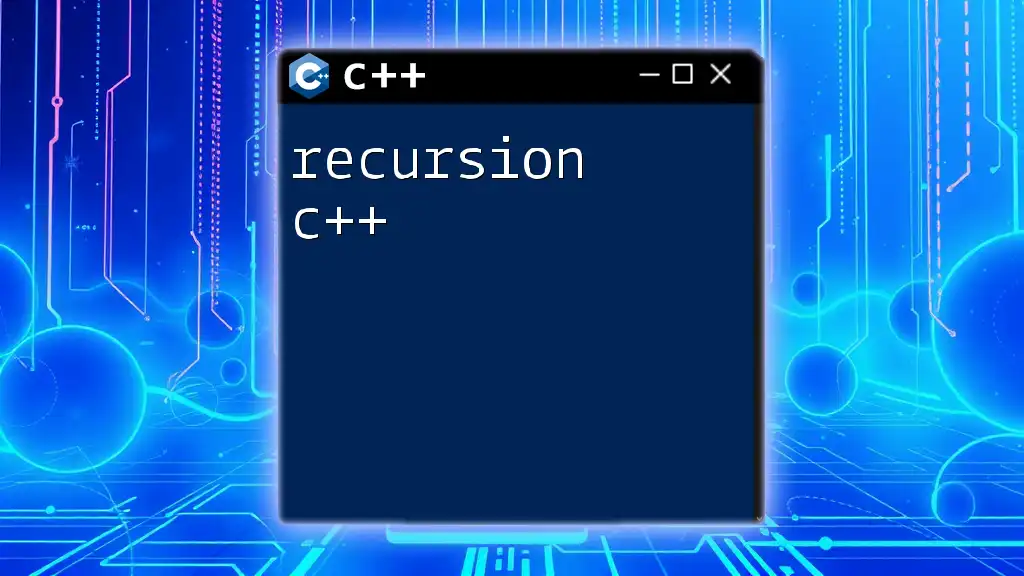
Common Errors and Troubleshooting
Common Mistakes
- Using unsorted ranges: `set_intersection` requires that both input sets be sorted. Forgetting this can lead to incorrect results.
- Misconfigured output iterator: Ensure that the output container is appropriately set up to receive the results.
Debugging Tips
If you encounter issues while using `set_intersection`, check for the following:
- Make sure that both input sets are sorted.
- Verify that the output iterator can accept the results (using `std::back_inserter`, for example).
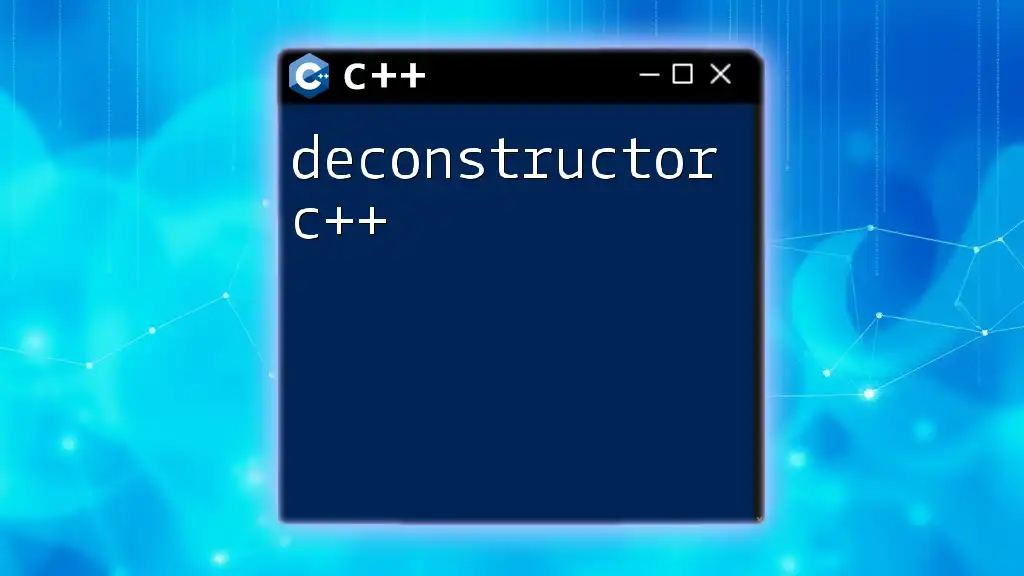
Conclusion
Incorporating `set_intersection` in your C++ projects can significantly improve your capabilities in managing and analyzing data. By understanding this function, you will be equipped to explore further C++ set operations and broaden your programming toolkit. Don’t hesitate to apply what you’ve learned about `set_intersection` and witness how it can streamline your data handling efforts.