In C++, the `normal_distribution` class from the `<random>` header generates numbers following a normal (Gaussian) distribution, allowing you to specify the mean and standard deviation.
Here's a simple code snippet demonstrating its use:
#include <iostream>
#include <random>
int main() {
std::default_random_engine generator;
std::normal_distribution<double> distribution(0.0, 1.0); // mean = 0, stddev = 1
for (int i = 0; i < 10; ++i) {
std::cout << distribution(generator) << std::endl; // generate 10 random numbers
}
return 0;
}
Understanding Normal Distribution
Normal distribution is a statistical concept that describes how the values of a variable are distributed. It is characterized by its bell-shaped curve, where most of the observations cluster around the central peak and probabilities for values further away from the mean taper off symmetrically in both directions. This distribution is essential in various fields, such as psychology, finance, and quality control, because many natural phenomena can be modeled with it.
Key Characteristics of Normal Distribution
A few key characteristics define normal distribution:
-
Mean, Median, and Mode: In a perfectly normal distribution, these three measures of central tendency are identical, located at the center of the curve.
-
Standard Deviation and Variance: The shape of the normal distribution curve is determined by these two properties. A small standard deviation results in a steeper curve, while a larger standard deviation flattens the curve.
-
68-95-99.7 Rule: This empirical rule illustrates how data is spread out within one, two, or three standard deviations from the mean. Approximately 68% of the data will fall within one standard deviation, 95% within two, and 99.7% within three.

C++ and Normal Distribution
C++ offers several features to deal with random number generation and statistical distributions. At the center of this functionality is the `<random>` library, which provides various generators and distribution classes to create random samples.
What is `<random>` in C++?
The `<random>` library is a standard part of C++11 and later versions, designed to generate random numbers with various properties. It allows developers to create random distributions, including uniform, normal, and Poisson, ensuring that the random numbers generated can mimic many real-life scenarios or statistical requirements.
Normal Distribution in C++
In C++, the most utilized distribution for normal data is `std::normal_distribution`. This class is part of the `<random>` library and is used to represent normal distribution.
Here's the syntax to create a normal distribution object:
std::normal_distribution<T> normal_distribution(mean, standard_deviation);
- mean: The average value around which the data points or random samples are centered.
- standard_deviation: This determines how spread out the values are from the mean.
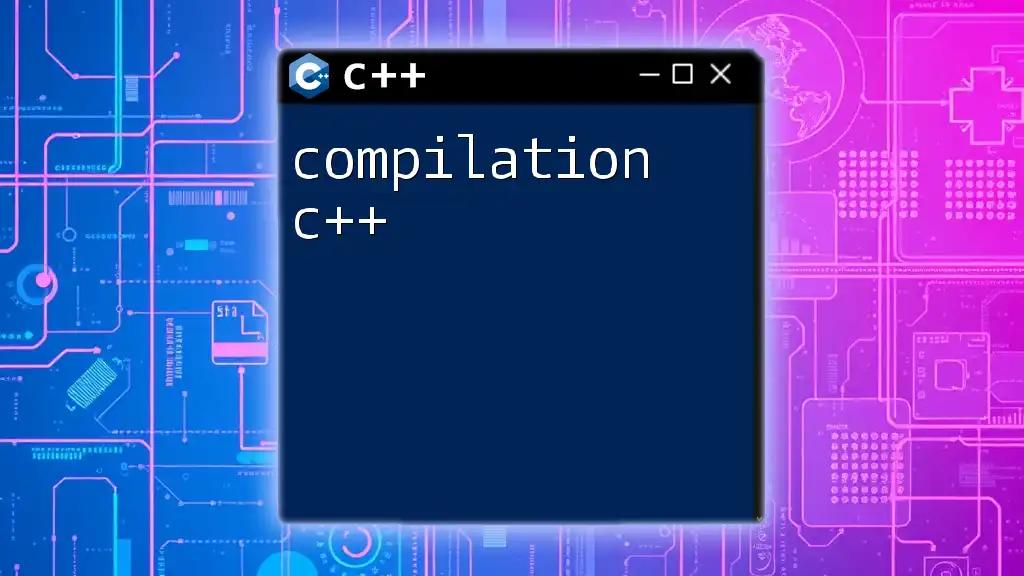
How to Use `std::normal_distribution` in C++
Setting Up Your Environment
To use normal distribution in your C++ programs, make sure to include the necessary headers:
#include <iostream>
#include <random>
Creating a Normal Distribution Object
Here's an example of how to create a normal distribution object using C++:
#include <iostream>
#include <random>
int main() {
// Obtain a random number from hardware
std::random_device rd;
// Seed the generator
std::mt19937 gen(rd());
// Normal distribution with mean 0 and standard deviation 1
std::normal_distribution<> distr(0, 1);
// Generate and print random normal numbers
for(int n = 0; n < 10; ++n) {
std::cout << distr(gen) << ' ';
}
}
In this snippet, we first seed the random number generator using `std::random_device`. The `std::normal_distribution` object is then created with a specified mean and standard deviation. In this case, we set the mean to 0 and the standard deviation to 1, representing the standard normal distribution. The loop generates and prints ten random samples from this distribution.
Generating Random Samples
The generated samples can be used for simulations, statistical tests, or analysis. It's important to note that each time you run the program, you'll receive different sequences of numbers due to the randomness incorporated in the generation process.
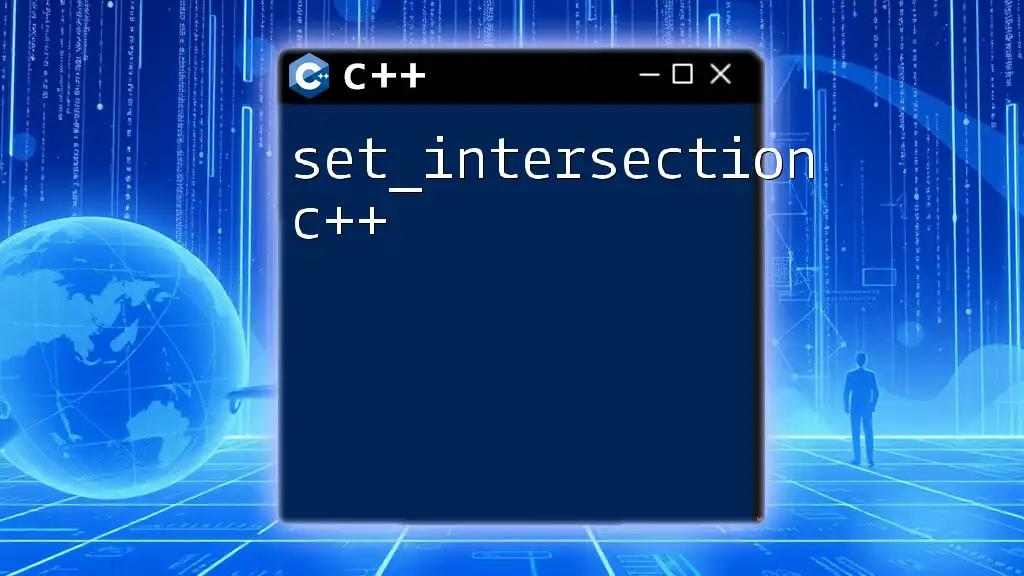
Applications of Normal Distribution in C++
Simulation of Real-World Phenomena
Normal distribution plays a vital role in simulating real-world phenomena. For instance, in finance, stock prices often exhibit behavior that can be approximated by a normal distribution due to a variety of contributing factors.
Here’s an example of simulating stock prices using normal distribution:
#include <iostream>
#include <random>
void simulateStockPrices() {
std::random_device rd; // Obtain a random number from hardware
std::mt19937 gen(rd()); // Seed the generator
// Normal distribution with mean 100 and std deviation 15
std::normal_distribution<> priceDistr(100, 15);
for(int i = 0; i < 10; ++i) {
std::cout << "Simulated stock price: $" << priceDistr(gen) << std::endl;
}
}
In this function, we simulate stock prices using a normal distribution. The generated price distribution is centered around the mean price of $100 with a standard deviation of $15. Each stock price generated can represent a possible outcome based on our parameters.
Statistical Analysis
Normal distribution is also utilized in statistical analysis. In hypothesis testing, for example, we often assume that sample means are normally distributed due to the Central Limit Theorem. This allows statisticians to conduct analyses such as computing confidence intervals, performing t-tests, or analyzing relationships between variables.
When we want to confirm that a dataset follows normality, we can use `std::normal_distribution` to assess residuals in regression analysis or check assumptions in various statistical tests.
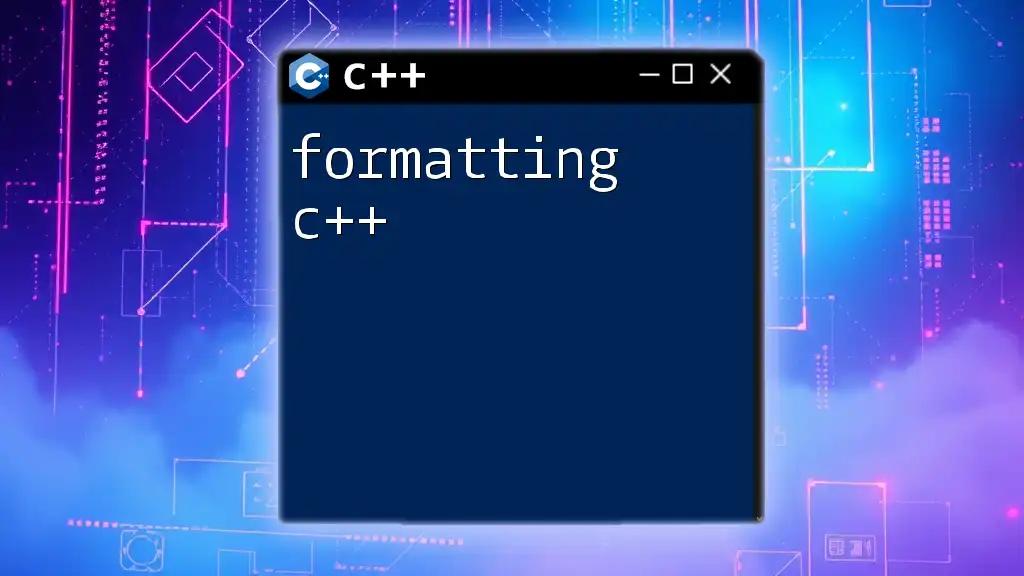
Visualizing Normal Distribution
Visual representation of normal distribution can significantly enhance understanding. Several libraries can help you plot normal distributions in C++, such as Matplotlib or other visualization tools. Here’s a pseudo-code layout of how you’d plot a normal distribution:
// Pseudo-code example for plotting
// Requires additional library like MatplotlibCPP
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
void plotNormalDistribution() {
std::vector<double> x;
std::vector<double> y;
double mean = 0;
double stddev = 1;
// Generating x and y values
for(double i = -4; i <= 4; i += 0.1) {
x.push_back(i);
y.push_back((1 / (stddev * sqrt(2 * M_PI))) * exp(-0.5 * pow((i - mean)/stddev, 2)));
}
plt::plot(x, y);
plt::title("Normal Distribution (Mean=0, Std Dev=1)");
plt::xlabel("Value");
plt::ylabel("Probability Density");
plt::show();
}
The pseudo-code demonstrates generating x and y values to represent the normal distribution curve, with mean and standard deviation defined. When implemented correctly with an appropriate library, it will plot the classic bell curve shape.
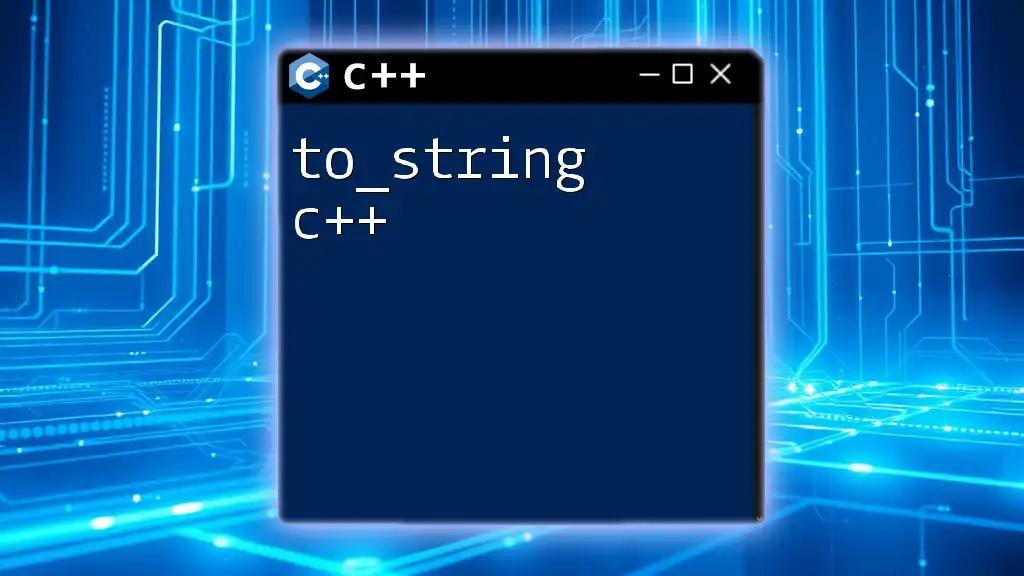
Conclusion
In this guide, you've learned that normal_distribution c++ is pivotal in statistical data generation and analysis. By leveraging C++'s `<random>` library and the `std::normal_distribution` class, you can create random samples that reflect real-world phenomena.
Whether simulating financial markets, performing statistical tests, or analyzing data distributions, understanding the concepts of normal distribution can enrich your programming toolkit. For further learning, consider engaging with books, online courses, or other resources focused on statistics and C++. Experimenting with `std::normal_distribution` and other features will solidify your understanding and improve your practical skills in C++.