The standard deviation in C++ can be calculated using the `<numeric>` and `<cmath>` libraries to find the variance of a dataset and then take the square root.
Here's a code snippet demonstrating how to calculate the standard deviation of a vector of numbers:
#include <iostream>
#include <vector>
#include <cmath>
#include <numeric>
double standardDeviation(const std::vector<double>& data) {
double mean = std::accumulate(data.begin(), data.end(), 0.0) / data.size();
double sumSquaredDiffs = 0.0;
for (double num : data) {
sumSquaredDiffs += (num - mean) * (num - mean);
}
return std::sqrt(sumSquaredDiffs / data.size());
}
int main() {
std::vector<double> data = {10, 12, 23, 23, 16, 23, 21, 16};
std::cout << "Standard Deviation: " << standardDeviation(data) << std::endl;
return 0;
}
Understanding the Concept of Standard Deviation
What is Standard Deviation?
Standard deviation is a statistical measure that quantifies the amount of variation or dispersion in a set of data values. A low standard deviation indicates that the data points tend to be close to the mean (average) value, while a high standard deviation indicates that the data points are spread out over a wider range of values. Essentially, it provides a sense of how much individual data points differ from the mean, giving insights into the data's consistency and reliability.
Why Use Standard Deviation in C++?
In the realm of programming, especially when dealing with data analysis and statistics, understanding how to utilize standard deviation is crucial. It can help in making informed decisions based on the variability in data, whether for scientific research, financial forecasting, or quality control in manufacturing.
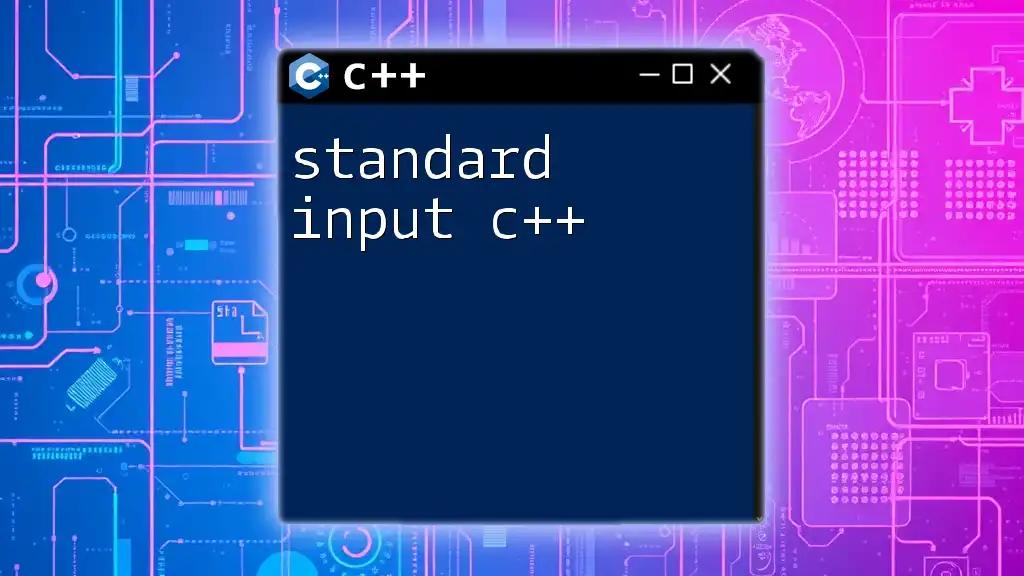
Formula for Standard Deviation
The formula for standard deviation can be a bit intimidating at first glance, but it's quite straightforward. It can be expressed as follows:
-
Population Standard Deviation:
\[ \sigma = \sqrt{\frac{1}{N}\sum_{i=1}^{N}(x_i - \mu)^2} \] -
Sample Standard Deviation:
\[ s = \sqrt{\frac{1}{n-1}\sum_{i=1}^{n}(x_i - \bar{x})^2} \]
Where:
- \( \sigma \) is the population standard deviation.
- \( s \) is the sample standard deviation.
- \( N \) is the total number of data points in the population.
- \( n \) is the number of data points in the sample.
- \( x_i \) represents each data point.
- \( \mu \) is the mean of the population.
- \( \bar{x} \) is the mean of the sample.
Understanding these formulas is essential for accurately calculating standard deviation in C++.

Implementing Standard Deviation in C++
Setting Up Your C++ Environment
Before diving into coding, ensure you have a proper C++ development environment set up. You will primarily require the `<cmath>` library for mathematical functions and the `<vector>` library to manage dynamic arrays.
Code Snippet: Calculating the Mean
The mean is the average of all the data points and serves as the foundation for calculating standard deviation. Here’s how you can calculate it in C++:
double calculateMean(const std::vector<double>& data) {
double sum = 0.0;
for (double num : data) {
sum += num;
}
return sum / data.size();
}
Explanation: In this function, we initialize a sum variable to zero. We then iterate over each number in the data vector, accumulating the total. Finally, we return the mean by dividing the sum by the number of elements in the vector.
Code Snippet: Calculating Variance
Variance reflects how far each data point is from the mean and is crucial for standard deviation calculations. Here’s how to calculate it:
double calculateVariance(const std::vector<double>& data, double mean) {
double variance = 0.0;
for (double num : data) {
variance += (num - mean) * (num - mean);
}
return variance / (data.size() - 1); // Sample variance
}
Explanation: This function computes the variance by iterating over each number in the data vector. For each number, it computes its square difference from the mean and accumulates this value. Finally, it divides by \(n - 1\) to get the sample variance, which adjusts for the sample size.
Code Snippet: Calculating Standard Deviation
By now, you have everything needed to calculate the standard deviation, which uses both the mean and variance. Here’s a simple implementation:
double calculateStandardDeviation(const std::vector<double>& data) {
double mean = calculateMean(data);
double variance = calculateVariance(data, mean);
return std::sqrt(variance);
}
Explanation: This function first calculates the mean of the data. Then it computes the variance using that mean. Finally, it returns the square root of the variance to yield the standard deviation.
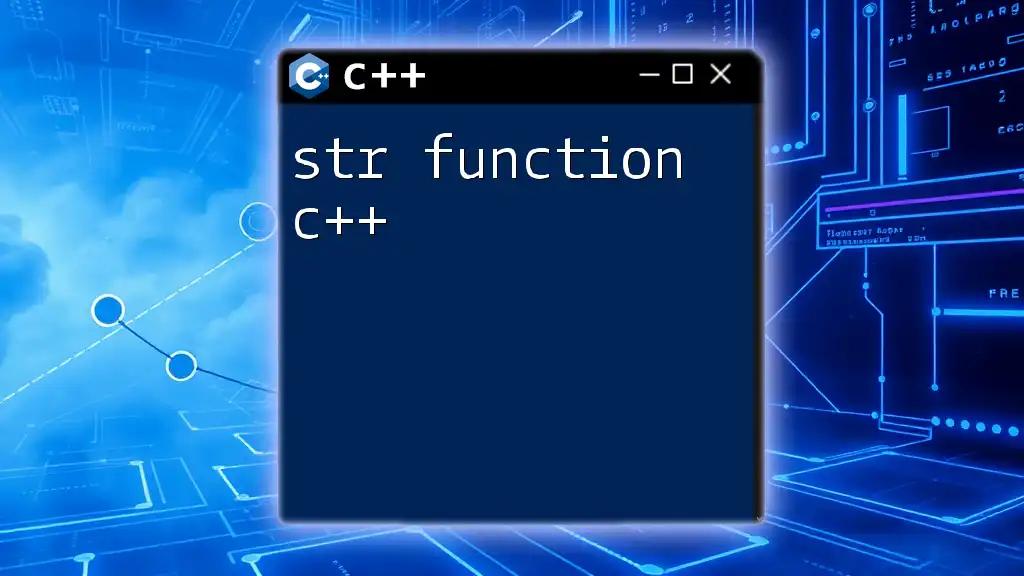
Complete Example: Putting It All Together
Now that we understand how to calculate mean, variance, and standard deviation, let’s look at a complete C++ program that ties everything together:
#include <iostream>
#include <vector>
#include <cmath>
double calculateMean(const std::vector<double>& data);
double calculateVariance(const std::vector<double>& data, double mean);
double calculateStandardDeviation(const std::vector<double>& data);
int main() {
std::vector<double> data = {10, 12, 23, 23, 16, 23, 21, 16};
double stdDev = calculateStandardDeviation(data);
std::cout << "Standard Deviation: " << stdDev << std::endl;
return 0;
}
Explanation: In this program, we include necessary libraries and define our function prototypes. Inside the `main()` function, we initialize a vector of data points. We then call the `calculateStandardDeviation()` function, and print the result. This provides a complete view of how standard deviation can be calculated and displayed in C++.

Practical Applications of Standard Deviation in C++
Data Analysis and Interpretation
Standard deviation serves as a powerful tool in data analysis, facilitating the identification of data dispersion and trends. By understanding the variability in data, analysts can assess the reliability of data-driven conclusions.
Quality Control
In manufacturing, standard deviation is frequently employed to maintain product quality. By calculating the standard deviation of measurements, companies can identify variations that exceed acceptable limits, ensuring products meet established standards.
Finance and Investment
Investors commonly use standard deviation to gauge the risk associated with a particular investment or portfolio. A higher standard deviation indicates greater volatility and risk, assisting investors in making educated decisions concerning asset allocation.

Conclusion
In summary, this guide has covered the essentials of standard deviation in C++. We explored the mathematical foundations, implemented code to calculate mean, variance, and standard deviation, and highlighted practical applications across various domains. Understanding these concepts not only enhances programming capabilities but also empowers data-driven decision-making.
As you delve deeper into statistical analysis using C++, take time to practice implementing these functions with different data sets. The more you experiment, the more confident you'll become in your ability to work with statistics in programming.