To instantiate a C++ object, you declare a variable of the object's class type and then can either use the default constructor or specify parameters if the constructor requires them. Here's a code snippet demonstrating object instantiation:
class Car {
public:
Car() {
// Default constructor
}
Car(std::string model) {
// Parameterized constructor
}
};
// Instantiating objects using both constructors
Car myCar; // Using default constructor
Car anotherCar("Toyota"); // Using parameterized constructor
What Is Instantiation in C++?
Instantiation is the process of creating an instance of a class, effectively turning a class blueprint into a usable object in memory. This process is crucial in object-oriented programming because it lies at the heart of utilizing the full features of a class, such as methods and properties.
When you instantiate a class in C++, you create an actual object based on the class definition. This allows you to work with that object through its methods and member variables, making your programming more functional and organized.

Types of Instantiation
Stack Instantiation
Stack instantiation refers to the allocation of memory on the stack for an object. Memory on the stack is automatically managed, meaning that when the function in which the object resides exits, memory is automatically reclaimed.
Example of Stack Instantiation
class MyClass {
public:
int value;
MyClass(int v) : value(v) {} // Constructor
};
void function() {
MyClass obj(10); // obj is created on the stack
// obj can be used here
// Once function exits, obj is destroyed automatically
}
In the above example, `obj` is instantiated on the stack. This is straightforward as it requires no additional memory management overhead; however, the object’s lifetime is bound to the scope in which it was created.
Heap Instantiation
In contrast, heap instantiation means that memory for an object is allocated dynamically from the heap using `new`. The memory allocated in this way must be manually managed, which means that it must also be explicitly deallocated to avoid memory leaks.
Example of Heap Instantiation
class MyClass {
public:
int value;
MyClass(int v) : value(v) {} // Constructor
};
void function() {
MyClass* obj = new MyClass(10); // obj is created on the heap
// Use obj here
delete obj; // Freeing the allocated memory
}
In this code snippet, `obj` is created on the heap using the `new` keyword. After its use, it is crucial to call `delete` to free up the memory allocated for this object, thus preventing memory leaks.
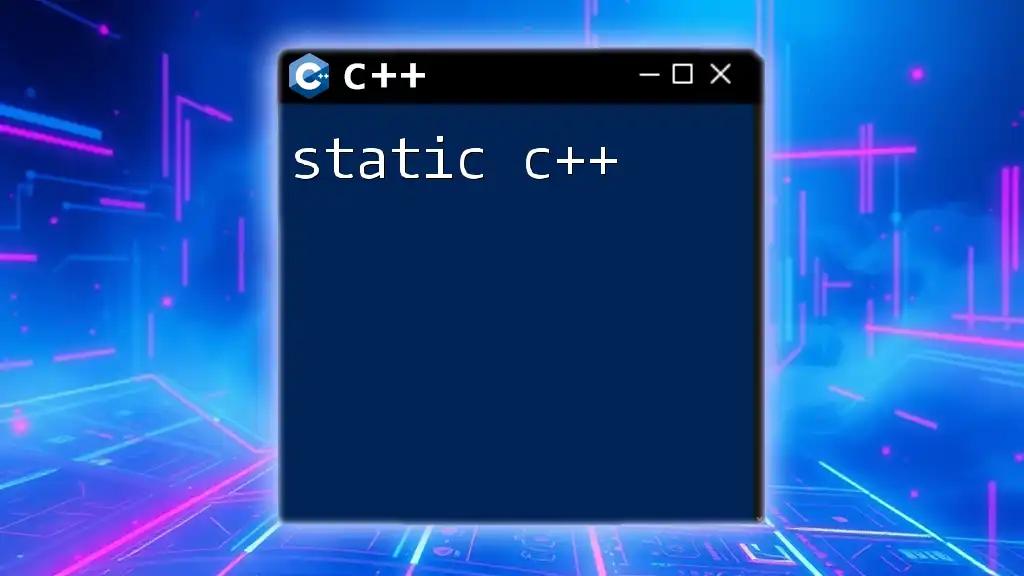
Constructors and Destructors
What Are Constructors?
Constructors are special member functions of a class that are called when an object of the class is instantiated. They enable you to initialize member variables and allocate resources when an object is created.
Constructors can be categorized into three types:
- Default Constructor: Initializes member variables with default values.
- Parameterized Constructor: Allows for initialization with specific values passed in as parameters.
- Copy Constructor: Creates a new object as a copy of an existing object.
Example of Constructors
class MyClass {
public:
int value;
MyClass() : value(0) {} // Default constructor
MyClass(int v) : value(v) {} // Parameterized constructor
MyClass(const MyClass& other) : value(other.value) {} // Copy constructor
};
In the example above, the `MyClass` provides three ways to create objects with varying initialization methods.
What Are Destructors?
Destructors are also special member functions that are called when an object goes out of scope or is explicitly deleted. They are used to perform cleanup operations, such as releasing resources or memory.
Example of Destructors
class MyClass {
public:
~MyClass() {
// Cleanup code here
}
};
The destructor in this example can help ensure any dynamically allocated resources are freed when the object is destroyed.

Understanding Object Lifetimes
Understanding the lifetime of an object is crucial for effective memory management in C++. Objects created on the stack are destroyed automatically once they go out of scope, while objects created on the heap must be explicitly deleted.
Static vs Dynamic Lifetime
-
Static Lifetime: The memory is allocated when the program starts and deallocated when the program ends. Static variables live throughout the entire runtime of the program.
-
Dynamic Lifetime: The object exists until it is explicitly destroyed, allowing for more control, but also requiring more responsibility for the programmer.
Using RAII for Resource Management
RAII (Resource Acquisition Is Initialization) is a programming technique that binds the lifecycle of resources to the lifetime of objects. Using RAII, resources are allocated during object creation and deallocated when the object is destroyed, effectively managing resource usage and preventing leaks.

Common Mistakes in Instantiation
Memory Leaks
A common pitfall in C++ programming occurs when dynamically allocated memory is not properly deallocated. This is known as a memory leak.
void function() {
MyClass* obj = new MyClass(10);
// Missing delete statement may lead to a memory leak
}
In this example, if `delete obj;` is not called, memory allocated for `obj` remains allocated, wasting resources.
Object Slicing
Object slicing can occur when an object of a derived class is treated as a base class object. This can lead to the loss of derived class-specific attributes and methods.
class Base {
public:
virtual void func() { /*...*/ }
};
class Derived : public Base {
public:
void func() override { /*...*/ };
};
void function() {
Base obj = Derived(); // Slicing occurs here
}
In this code, the `Derived` object is sliced when assigned to a `Base` type, resulting in the loss of any additional functionality.
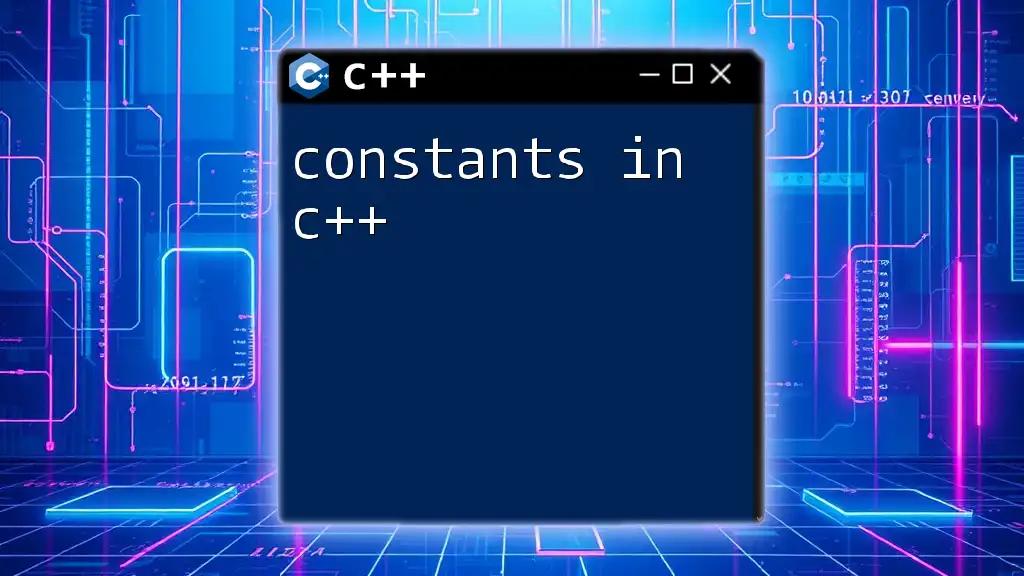
Best Practices for Instantiation
Using Smart Pointers
To prevent memory leaks and manage memory automatically, it is recommended to use smart pointers in modern C++. Smart pointers like `std::unique_ptr` and `std::shared_ptr` take ownership of dynamically allocated memory and automatically delete it when no longer needed.
Example of Smart Pointers
#include <memory>
void function() {
std::unique_ptr<MyClass> obj = std::make_unique<MyClass>(10);
// No need to manually delete; memory will be freed automatically
}
In this example, `obj` is a unique pointer that will automatically be destroyed when it goes out of scope, effectively managing memory without manual intervention.

Conclusion
Properly instantiating objects in C++ is fundamental for effective programming. By understanding the principles of stack and heap instantiation, constructors and destructors, and applying best practices like using smart pointers, developers can create robust and efficient applications. With careful management of object lifetimes and resources, programmers can leverage the full power of C++ while minimizing pitfalls associated with manual memory management. Continue to explore and practice these concepts to become proficient in instantiating objects in C++.