`int64_t` in C++ is a data type defined in the `<cstdint>` header that represents a signed 64-bit integer, ensuring a consistent size across different platforms.
#include <cstdint>
#include <iostream>
int main() {
int64_t largeNumber = 9223372036854775807; // Maximum value for int64_t
std::cout << "The large number is: " << largeNumber << std::endl;
return 0;
}
What is int64_t?
`int64_t` is a data type defined in the `<cstdint>` header file in C++. It represents a signed integer that is precisely 64 bits in size. The "64" indicates the number of bits used to store the integer, allowing it to represent a wide range of values from −9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. This property makes `int64_t` incredibly useful when dealing with large values that exceed the limits of the standard integer types, such as `int` or `long`.
The primary advantage of using `int64_t` lies in its fixed-width nature, ensuring that an `int64_t` will use the same amount of memory on any platform. This feature adds a layer of reliability and portability to your programs, especially when dealing with data that may originate from various systems.
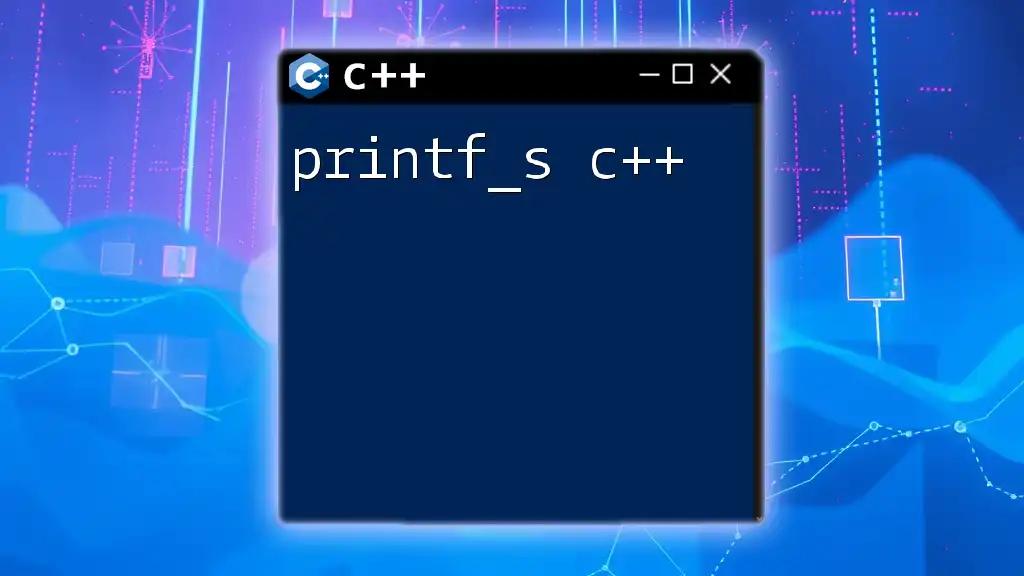
Understanding Fixed-Width Integers
What are Fixed-Width Integers?
Fixed-width integers are types that occupy a predetermined number of bits in memory. C++ provides several fixed-width integer types, including `int8_t`, `int16_t`, `int32_t`, and `int64_t`. The numeral in each identifier indicates how many bits the data type utilizes:
- `int8_t`: 8 bits
- `int16_t`: 16 bits
- `int32_t`: 32 bits
- `int64_t`: 64 bits
This fixed-width feature ensures consistency when reading or writing data across different systems and architectures.
Why Use Fixed-Width Integers?
Using fixed-width integers like `int64_t` is essential for scenarios where data precision and consistency are crucial. For instance, in financial applications, you need to ensure that monetary values are accurately represented without risk of overflow or underflow. Standard integer types in C++ may vary in size based on the architecture (32-bit vs. 64-bit systems), leading to potential errors in calculations or data interpretation.
In situations where you deal with records of different types from multiple sources, fixed-width types guarantee that the integer representation remains uniform, preventing bugs related to data size incompatibility.

How to Use int64_t in C++
Including the Header
To utilize `int64_t`, you must include the `<cstdint>` header in your file. This header defines the various fixed-width integer types.
#include <cstdint>
Declaring int64_t Variables
Declaring an `int64_t` variable is straightforward. You simply specify the type followed by the variable name.
int64_t largeNumber = 9223372036854775807; // Maximum value for int64_t
This code assigns the largest possible value for an `int64_t` type to the variable `largeNumber`. Make sure that the values you use are within the defined range, or you'll run into overflow issues.

Operators and int64_t
Arithmetic Operators
When working with `int64_t`, you can utilize the same arithmetic operators as you would with other integer types:
- Addition (`+`)
- Subtraction (`-`)
- Multiplication (`*`)
- Division (`/`)
- Modulus (`%`)
Here's an example demonstrating arithmetic operations with `int64_t`:
int64_t a = 5000000000;
int64_t b = 2000000000;
int64_t sum = a + b; // 7000000000
In this snippet, the addition of two large integers results in a valid outcome without overflow, as `int64_t` is capable of handling such large values.
Comparison Operators
`int64_t` supports all standard comparison operators, enabling effective comparisons of values:
- Equal to (`==`)
- Not equal to (`!=`)
- Less than (`<`)
- Greater than (`>`)
- Less than or equal to (`<=`)
- Greater than or equal to (`>=`)
For example:
if (a > b) {
// Do something if a is greater than b
}
Using `int64_t` in comparisons gives you confidence that your conditions will evaluate correctly without unexpected behavior due to type differences.
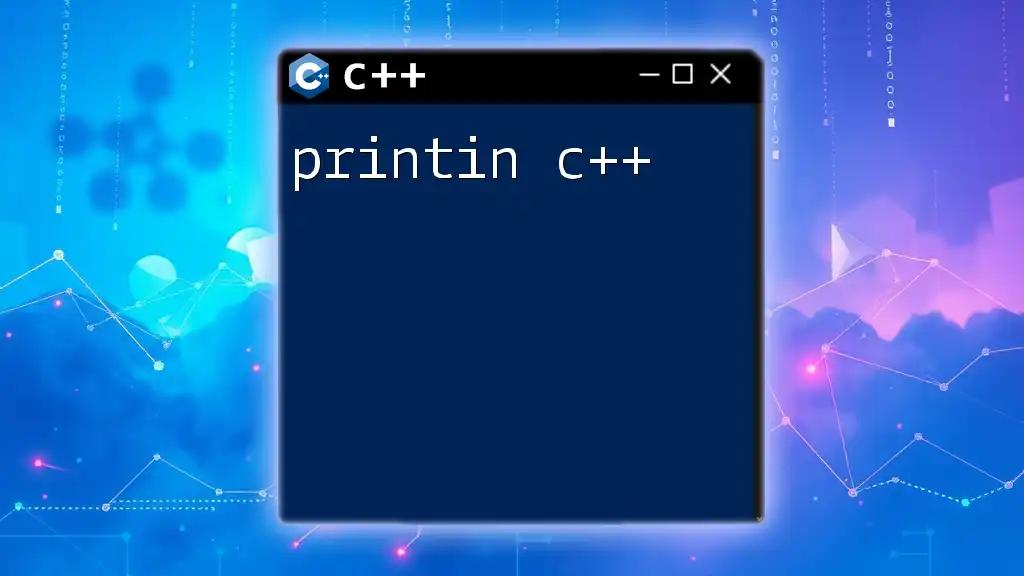
Practical Use Cases of int64_t
Applications in Data Processing
`int64_t` is especially popular in applications that involve large data sets, such as databases and financial software. For instance, when representing currency in a robust financial application, you might use `int64_t` to avoid floating-point precision errors:
int64_t price = 199990000; // Represents $199,990.00 in cents
This approach ensures that price calculations are precise, as working with integers eliminates issues that arise with rounding in floating-point arithmetic.
Benchmarking and Performance
In high-performance computing contexts, using the correct data type is critical for efficiency. Since `int64_t` is precisely 64 bits, operations on this type can be optimized in assembly, leading to better performance, especially when handling large numerical calculations.

Common Pitfalls and Best Practices
Overflow and Underflow Risks
While `int64_t` can handle large numbers, you must still be cautious of overflow and underflow, especially during arithmetic operations. An overflow occurs when you try to store a value larger than what can be represented:
int64_t a = INT64_MAX; // 9223372036854775807
int64_t b = 1;
int64_t result = a + b; // This will overflow
This will lead to unexpected behavior, so it's essential to implement checks to handle such scenarios:
if (a > INT64_MAX - b) {
// Handle overflow case
}
Performance Considerations
When using `int64_t`, consider how it affects memory usage and performance within your application. Although it provides the ability to handle larger values, it may introduce additional overhead when compared to smaller data types. Benchmarking can help determine the best choice for your specific use case.
Using int64_t with Other Data Types
You can often find yourself needing to integrate `int64_t` with floating-point types, which can result in casting issues. For example:
int64_t bigValue = 98765432101234;
double d = static_cast<double>(bigValue);
While this conversion ensures that `bigValue` can be used within floating-point calculations, pay attention to possible loss of precision, especially if the number you're converting exceeds the range of floating-point types.
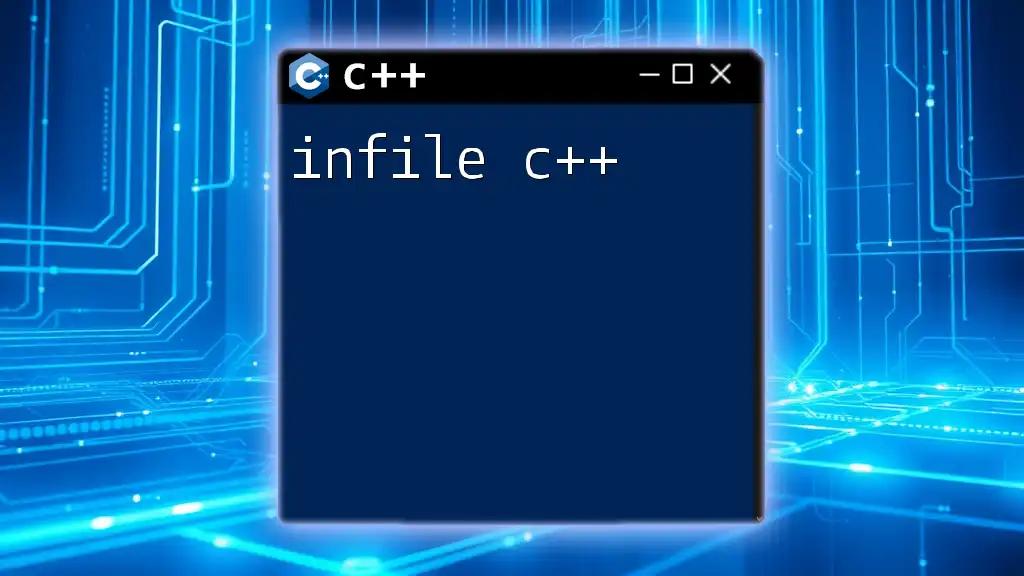
Conclusion
Understanding how to effectively use `int64_t` in C++ is crucial for developers working with applications that require managing large numbers accurately. By leveraging fixed-width integers, you can ensure portability and maintain precise calculations across different platforms. As you continue to develop, remember to keep an eye on potential pitfalls like overflow and underflow, and always select the appropriate data type for your specific requirements. With practice, you'll become proficient in wielding `int64_t` to create robust, efficient software solutions.
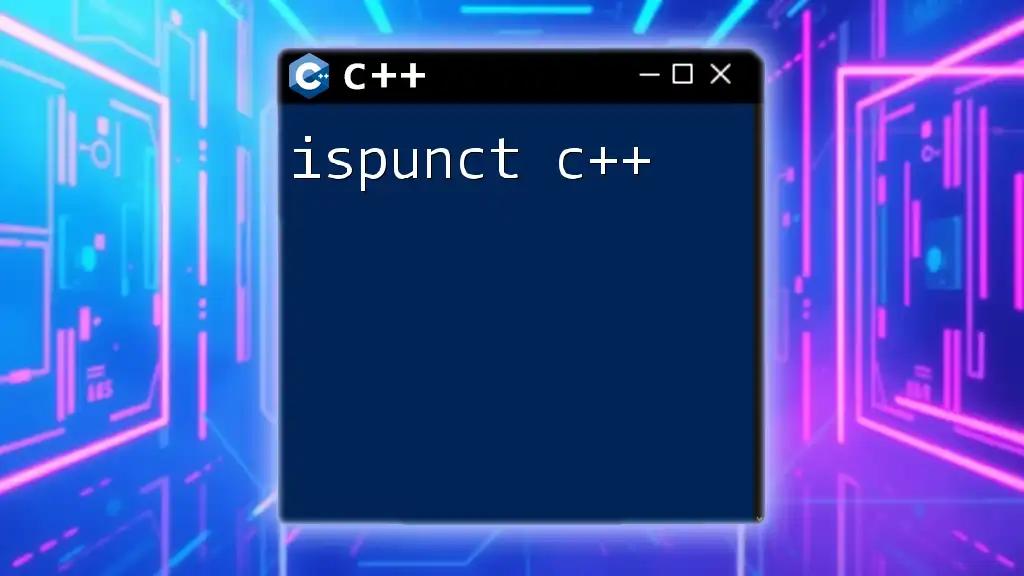
Additional Resources
For more in-depth knowledge, it's recommended to check the official C++ documentation and explore resources such as specialized books and online courses tailored to advanced C++ programming.

FAQs
What is the range of int64_t?
The range of `int64_t` extends from −9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
How does int64_t compare to long long?
In C++, `long long` typically holds at least 64 bits, meaning it often behaves similarly to `int64_t`, though depending on the platform, `long long` may not always provide the same guarantees.
Can int64_t be used in a multi-threaded environment?
Yes, `int64_t` is thread-safe like other basic data types, but remember to manage synchronization properly if multiple threads modify the same variable.
What issues should I be aware of when using int64_t on different compilers?
While compilers generally adhere to standard definitions, inconsistencies may arise with how `int64_t` interacts with other data types and operations, specifically in older compilers. Always consult the compiler documentation to understand any specific guidelines or differences.