In C++, inputs can be obtained from the user using the `cin` object along with the extraction operator (`>>`) for efficient data entry.
#include <iostream>
int main() {
int number;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "You entered: " << number << std::endl;
return 0;
}
Understanding User Input in C++
The concept of user input in C++ is fundamental for creating interactive applications. It allows programs to receive data from users, making the applications dynamic and responsive. By effectively handling user input, C++ programs can perform a variety of tasks based on the information provided.
What is User Input in C++?
User input refers to any data that is entered into a program during its execution. This can include numbers, text, and other forms of data that the program uses to function. Common scenarios where user input is essential include forms, command-line utilities, games, and simulations.
Basic Input Methods in C++
C++ provides several mechanisms for receiving input. The most commonly used method is via the `cin` object, which stands for "character input". This object is part of the iostream library, which must be included at the start of any C++ program that requires input or output operations.
When using `cin`, data is read from the standard input stream. The following is a simple example that illustrates how to read an integer from the user:
#include <iostream>
using namespace std;
int main() {
int a;
cout << "Enter a number: ";
cin >> a;
cout << "You entered: " << a << endl;
return 0;
}
In this code snippet, we first declare an integer variable `a`. The program prompts the user to enter a number, which is stored in `a` when the user provides input. Finally, the inputted value is printed back to the console.
Using `cin` to Get Input
The syntax for using `cin` is straightforward: use the insertion operator (`>>`) to direct the input from `cin` into a variable. Here’s how it can handle multiple variables:
#include <iostream>
using namespace std;
int main() {
int a;
float b;
string c;
cout << "Enter an integer, a float, and a string: ";
cin >> a >> b >> c;
cout << "You entered: " << a << ", " << b << ", " << c << endl;
return 0;
}
In this example, `cin` can take various types of input—all we need to do is declare the variables we want to populate and separate them by spaces when entering data. However, keep in mind that `cin` stops reading input when it encounters whitespace.
Handling Strings with `getline`
While `cin` works well for most data types, it can struggle with strings, especially those that include spaces. In such cases, it's better to use the `getline` function, which reads an entire line of input, including any spaces.
Here is an example:
#include <iostream>
#include <string>
using namespace std;
int main() {
string name;
cout << "Enter your name: ";
getline(cin, name);
cout << "Hello, " << name << "!" << endl;
return 0;
}
Using `getline`, the user can enter their full name (even with spaces), which is then stored in the `name` variable. This approach ensures that no part of the input is lost due to whitespace.
Validating User Input
Validating user input is crucial in preventing errors and ensuring that the data received is both appropriate and safe to use. An example of input validation is visible in the following code, which ensures that the user enters a positive integer:
#include <iostream>
using namespace std;
#include <limits>
int main() {
int num;
cout << "Enter a positive integer: ";
while (!(cin >> num) || num <= 0) {
cout << "Invalid input. Please enter a positive integer: ";
cin.clear(); // clear the error state
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // ignore the invalid input
}
cout << "You entered: " << num << endl;
return 0;
}
In this example, the program enters a loop if the input is invalid. The `cin.clear()` method resets the state of `cin`, and `cin.ignore()` discards the invalid input from the buffer. This ensures the program doesn't get stuck on erroneous input.
Best Practices for Handling User Input
To enhance the effectiveness of user input handling in C++, consider the following tips:
-
Be Clear in Prompting the User: Ensure that prompts are easy to understand, guiding the user about what is expected.
-
Handle Different Types of Input Smoothly: Utilize appropriate input methods based on the data type, using `cin` for numbers and `getline` for strings.
-
Always Validate: Ensure that any input received is validated against potential errors and mishaps, maintaining the integrity of your application.
Common Mistakes to Avoid
When dealing with inputs in C++, some common pitfalls can lead to issues:
-
Forgetting to Validate Input: Skipping input validation can lead to unexpected behavior, crashes, or security vulnerabilities in the application.
-
Issues with Buffer Overflows and Security: Always sanitize inputs to protect against buffer overflow attacks, especially when dealing with strings or user-generated content.
-
Misunderstanding Data Types: Ensure a clear understanding of the data types you are working with; mixing types can lead to errors.
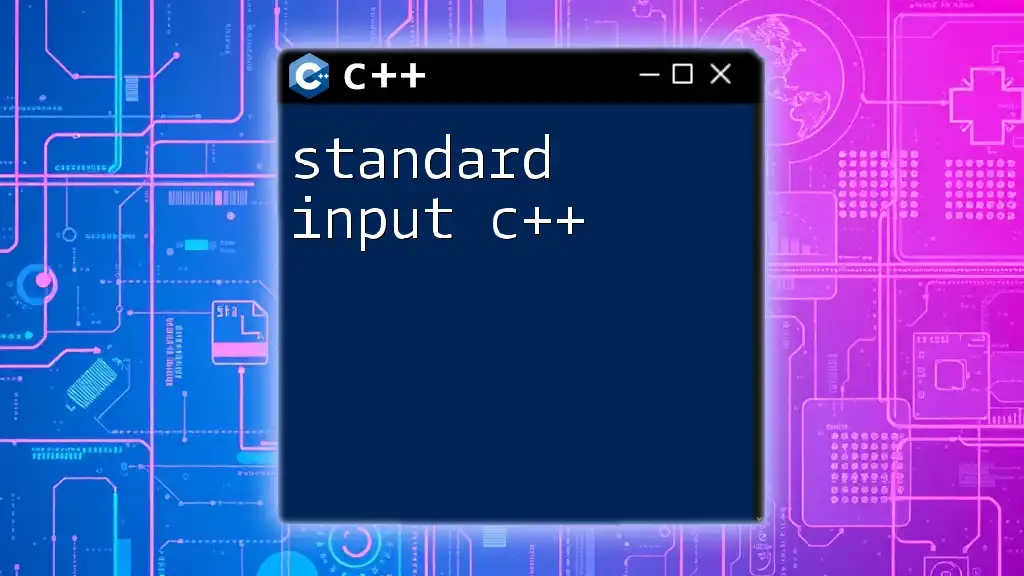
Conclusion
Understanding how to effectively manage inputs in C++ is crucial for creating competent and user-friendly applications. By mastering methods such as `cin` and `getline`, validating user inputs, and adhering to best practices, developers can enhance both the functionality and security of their C++ programs.
Preparing users for real-world applications by practicing these techniques can foster a deeper appreciation for effective programming. The keys to success are clarity, validation, and adapting input methods to their rightful uses. Happy coding!
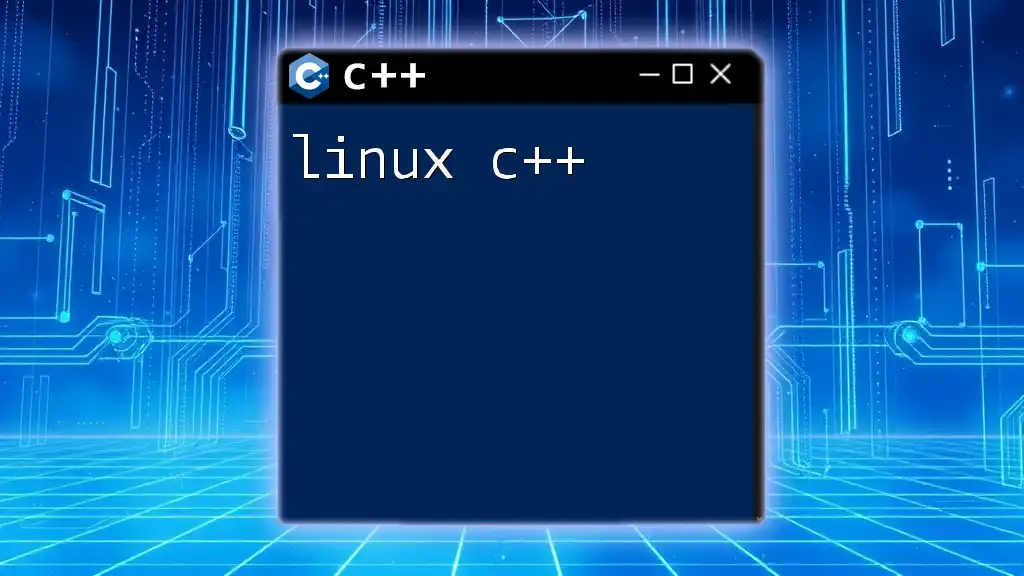
Further Reading and Resources
To deepen your understanding of user input in C++, consider exploring various online tutorials, official documentation, and community forums dedicated to C++ programming. Whether through books, video lessons, or interactive coding sites, there is much to learn and explore.