The `uint8_t` type in C++ is an unsigned 8-bit integer that allows you to store values ranging from 0 to 255, which is useful for handling small positive numbers efficiently.
Here's a code snippet demonstrating how to declare and use a `uint8_t` variable in C++:
#include <cstdint> // Include the header for fixed-width integer types
int main() {
uint8_t myNumber = 200; // Declare a uint8_t variable and assign a value
myNumber += 55; // Perform an addition operation
return 0;
}
Understanding `uint8` in C++
What is `uint8`?
`uint8`, which stands for "unsigned 8-bit integer," is a data type defined in C++ that allows for the storage of non-negative integer values ranging from `0` to `255`. The `uint8` type is particularly useful in situations where memory efficiency is critical, or when working with binary data, such as image processing or network protocols.
Importance of Unsigned Data Types
Using unsigned data types like `uint8` is beneficial for several reasons:
-
Data Representation: Since `uint8` can exclusively hold positive integers, it effectively doubles the range of values compared to a signed 8-bit integer. In contrast, a signed 8-bit integer can only represent values from `-128` to `127`.
-
Performance: Small data types such as `uint8` can improve memory allocation and cache performance. In systems with limited resources, every byte counts, and `uint8` helps keep the memory use minimal without sacrificing functionality.
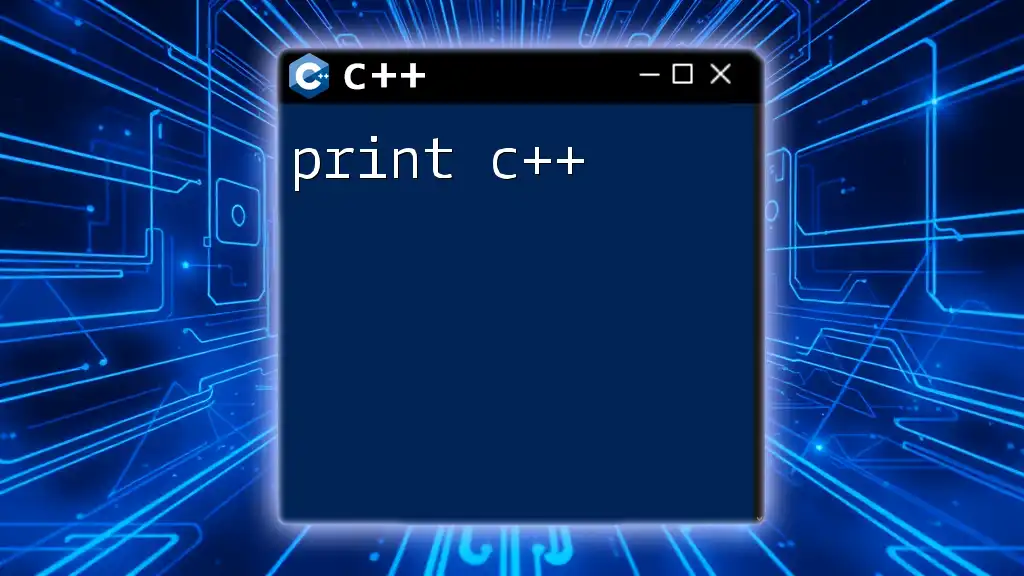
Declaring and Using `uint8` in C++
Basic Syntax for Declaring `uint8`
To use `uint8` in your C++ program, you typically include the `<cstdint>` header file, which provides fixed-width integer types, including `uint8_t`. The syntax for declaring a `uint8` variable is straightforward:
#include <cstdint>
uint8_t myVariable = 255; // Maximum value for uint8_t
In this example, `myVariable` is initialized with its maximum potential value.
Working with `uint8`
Initialization and usage of `uint8` are relatively simple. You can declare a single variable or even an array.
-
Single Value Initialization:
uint8_t myValue = 100; // A valid value
-
Array Initialization:
You can also initialize arrays to hold multiple `uint8` values:
uint8_t array[5] = {0, 1, 2, 3, 4};
This creates an array with five elements, all of which are unsigned 8-bit integers.
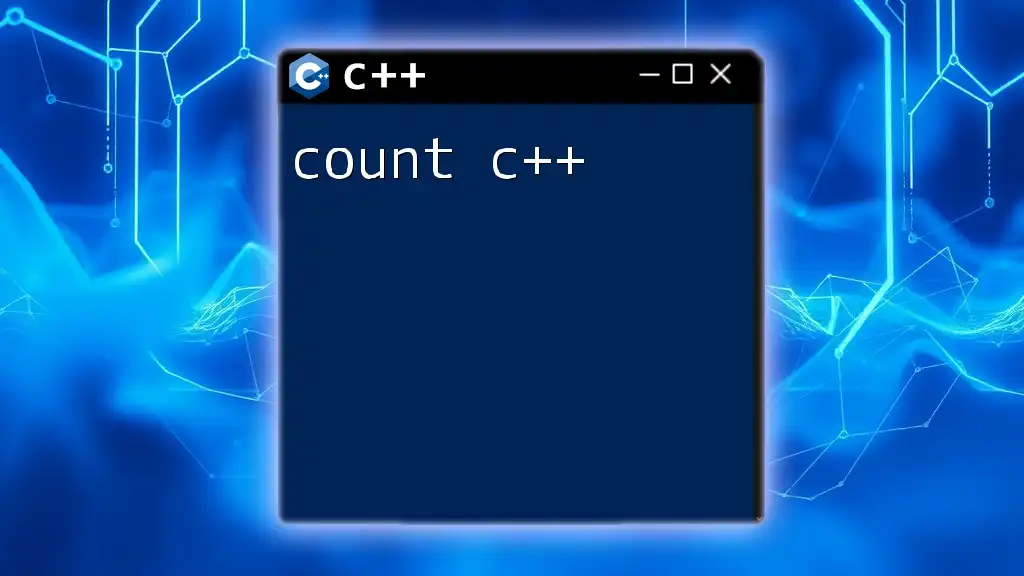
Operations with `uint8`
Basic Arithmetic Operations
Arithmetic operations with `uint8` follow the same principles as with other integer types. Basic arithmetic operations include addition, subtraction, multiplication, and division.
Here’s an example:
uint8_t a = 100;
uint8_t b = 50;
uint8_t sum = a + b; // 150
While this operation works well under normal circumstances, it's crucial to remain aware of potential overflow risks. Unsigned integers can wrap around upon reaching their maximum value, leading to unexpected results:
uint8_t overflow = 255 + 1; // Wraps around to 0
Bitwise Operations
In addition to arithmetic operations, you can also perform bitwise operations on `uint8` values, which manipulate individual bits.
Common bitwise operations include AND, OR, XOR, and NOT. Here’s how you can implement a bitwise AND:
uint8_t x = 0b11001100;
uint8_t y = 0b10101010;
uint8_t result = x & y; // Bitwise AND
In this case, `result` will hold the value of `0b10001000`, as the AND operation only retains bits where both operands are `1`.
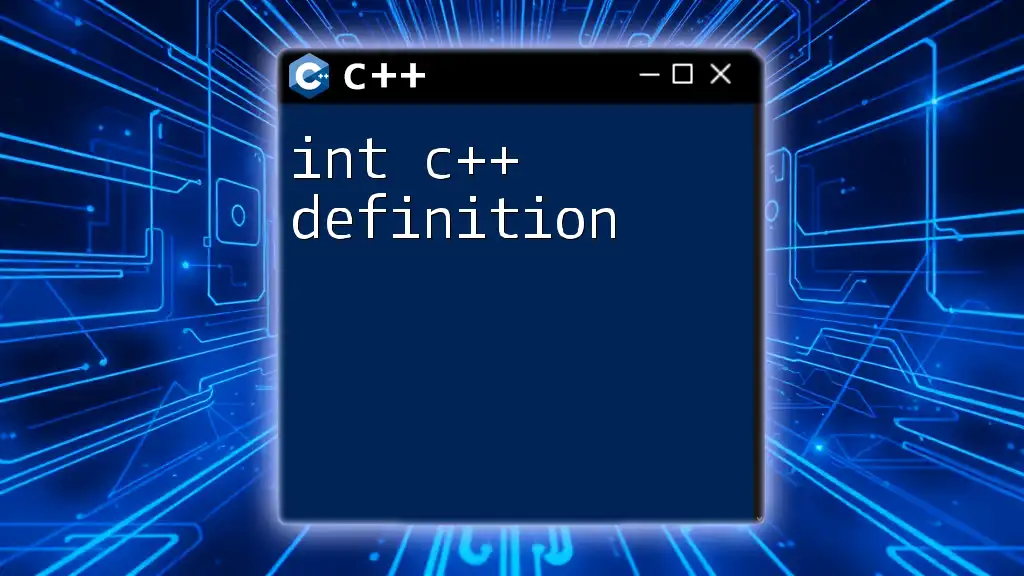
Use Cases for `uint8`
Memory Efficiency in Arrays and Data Structures
One of the notable benefits of `uint8` is its capacity to save memory. When constructing large arrays or vectors, using `uint8` can significantly reduce memory usage:
std::vector<uint8_t> dataContainer(1000); // Container for 1000 bytes
These small storage requirements are particularly advantageous in embedded systems or instances where you’re handling numerous byte-sized values, like pixels in an image.
Communication Protocols
In fields like low-level communication protocols, every byte counts. Using `uint8` allows developers to define messages efficiently within bandwidth limitations. Consider the following function:
void sendByte(uint8_t data) {
// code to send data over a serial interface
}
In this example, `sendByte` allows you to transmit a byte of data, ensuring efficient memory use during communication.
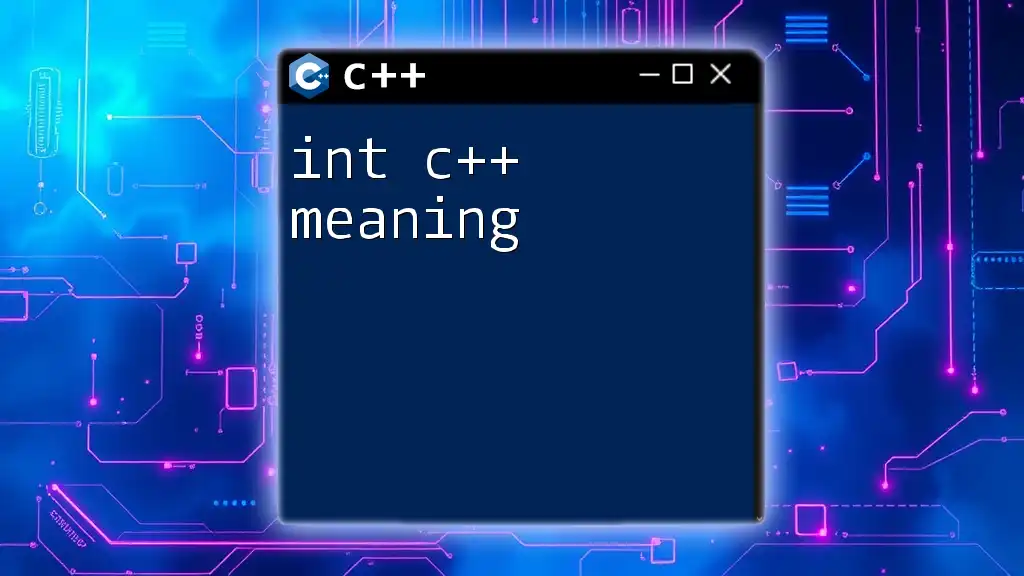
Common Pitfalls when Using `uint8`
Soft Limitations
While `uint8` is user-friendly, developers must practice caution. The primary concern is overflow. Exceeding the maximum capacity will result in unpredictable behavior, which can lead to bugs that are challenging to debug.
For instance:
uint8_t overflowExample = 255 + 1; // May lead to unexpected results: wrapped to 0
Type Conversions
Implicit type conversion can also introduce errors. When mixing `uint8` with larger integer types, you may unintentionally lose data. Consider the following code:
int largerValue = 300;
uint8_t smallValue = largerValue; // Loss of data; smallValue will hold undefined behavior.
This situation can result in incorrect calculations or operations, so careful management of types is essential.
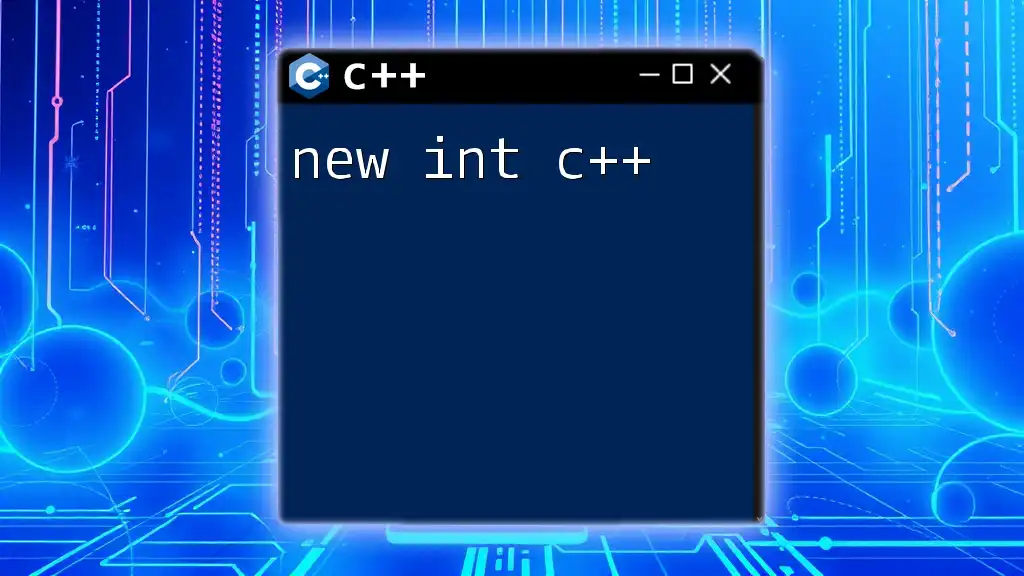
Best Practices for Using `uint8` in C++
When to Use and When to Avoid
Understanding when to use `uint8` is crucial for effective programming. Its application shines in situations where memory efficiency is paramount, such as in image processing, embedded programming, or when handling large datasets.
Conversely, if you regularly require the representation of negative values or need to deal with arithmetic that exceeds `255`, it is wise to consider larger data types.
Code Structuring Tips
To maintain clarity, strive to organize your code effectively while using `uint8`. When integrating `uint8` arrays or structures, ensure that your data is well-documented and accessible. Here's an example of a well-structured array declaration:
std::array<uint8_t, 256> colorPalette; // A palette for colors ranging from 0 to 255
Using clear array names and type definitions helps maintain readability and manageability, especially in larger codebases.
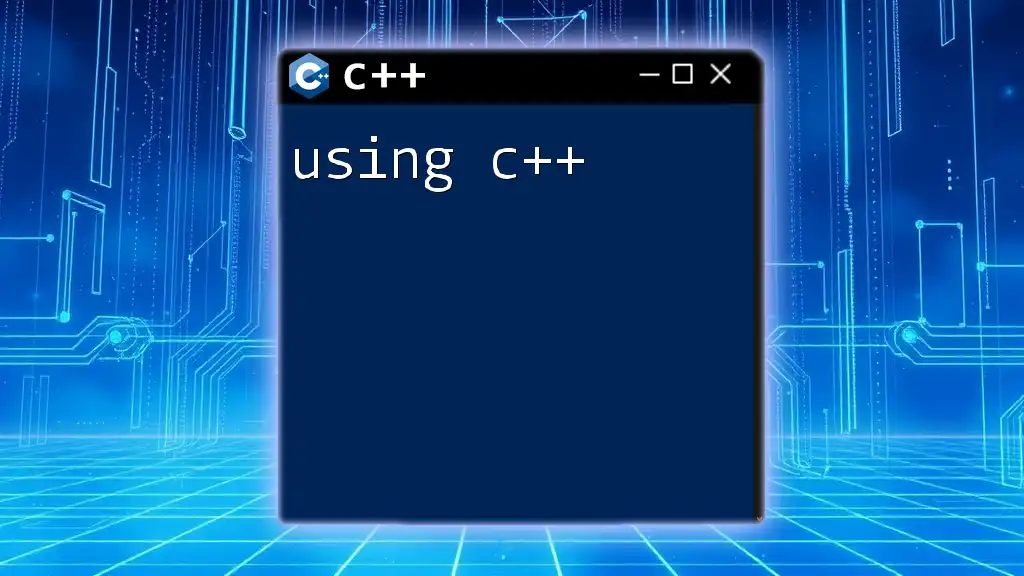
Conclusion
The `uint8` data type in C++ serves as a versatile and efficient tool for storing non-negative integers. By thoroughly understanding its attributes, limitations, and potential applications, you can utilize `uint8` to enhance your programming skills, leading to more efficient memory use and performance in your applications. Make the most of this powerful data type by experimenting with it in your projects, ensuring to adhere to best practices to mitigate any pitfalls along the way.