"Quiz C++" refers to a quick assessment tool that tests a learner's understanding of C++ programming concepts through interactive questions and code challenges.
Here's a simple C++ code snippet that implements a basic quiz question:
#include <iostream>
using namespace std;
int main() {
int answer;
cout << "What is the output of 2 + 2?\n";
cout << "1. 3\n2. 4\n3. 5\n4. 6\n";
cout << "Enter your answer (1-4): ";
cin >> answer;
if (answer == 2) {
cout << "Correct!" << endl;
} else {
cout << "Incorrect, try again." << endl;
}
return 0;
}
Understanding C++ Basics
What is C++?
C++ is a powerful general-purpose programming language that was developed as an extension of the C programming language. Created by Bjarne Stroustrup in the early 1980s, C++ has evolved significantly over the years. It incorporates object-oriented programming (OOP) features, making it versatile for a variety of applications, including systems software, application software, and game development.
Key Features of C++
C++ is known for its key features, such as:
- Object-Oriented Programming: This allows developers to create classes and objects, promoting code reuse and modularity. For example, consider the following code snippet demonstrating a simple class definition:
class Car {
public:
string brand;
int year;
void displayInfo() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
- Rich Standard Library: C++ comes with a comprehensive standard library that provides many functions, including algorithms and data structures. This library enables developers to perform complex tasks easily and efficiently.
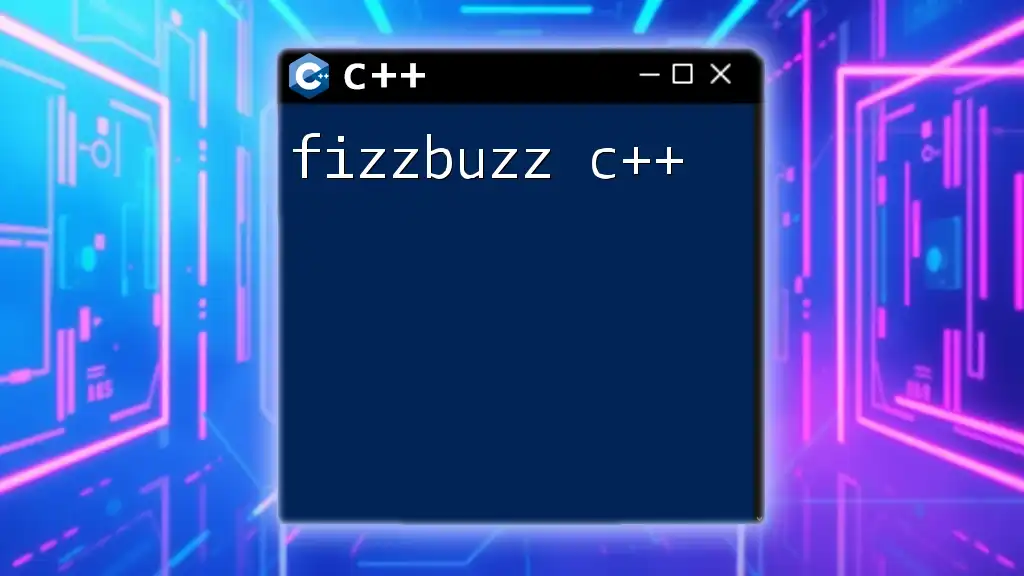
Importance of Quizzes in Learning C++
Benefits of Quizzing
Quizzes serve as an effective learning tool by engaging learners in active recall, which is crucial for retaining information. They allow for immediate feedback, helping users identify areas of weakness in their understanding. Engaging with quizzes not only enhances knowledge retention but also builds confidence in problem-solving skills.
Types of C++ Quizzes
Theoretical Quizzes
Theoretical quizzes assess understanding of concepts, definitions, and core values of C++. These quizzes typically include questions about the language’s syntax and features.
Example Question: What is polymorphism in C++?
Answer: Polymorphism allows objects to be treated as instances of their parent class, enabling flexibility in code. It is a core principle of OOP in C++.
Practical Quizzes
Practical quizzes challenge users to apply their knowledge by solving coding problems.
Example Problem: Write a function to reverse a string.
Answer:
#include <iostream>
#include <string>
using namespace std;
string reverseString(const string& str) {
string reversedStr = "";
for (int i = str.length() - 1; i >= 0; --i) {
reversedStr += str[i];
}
return reversedStr;
}
In this example, the function `reverseString` takes a string as input and returns the reversed version.
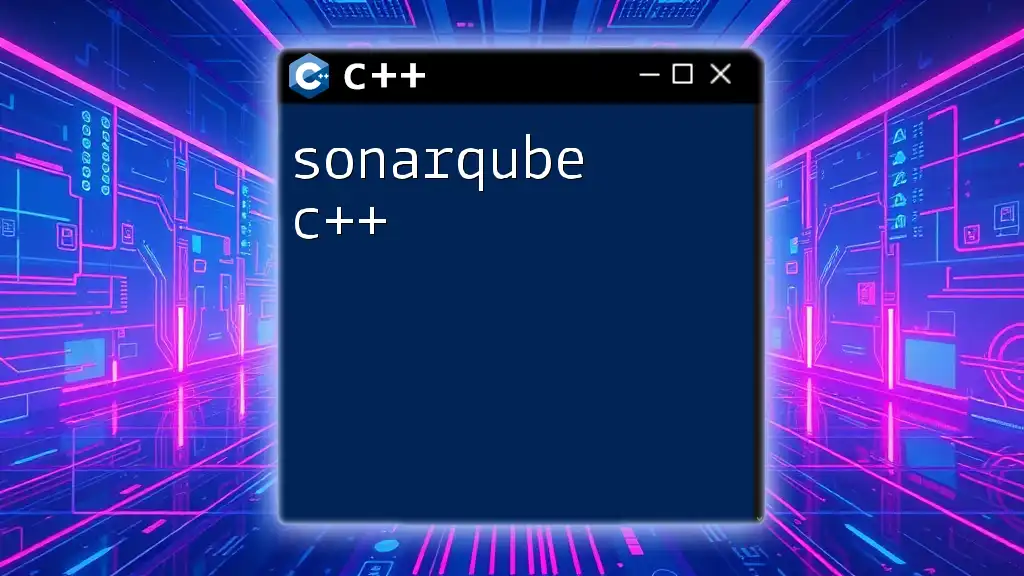
Creating Effective C++ Quizzes
Designing Questions
Writing clear and concise questions is fundamental to effective quizzes. Questions should be relevant and should vary in difficulty to accommodate learners of all levels, from beginners to advanced users.
Example Questions for C++ Quizzes
Multiple Choice Questions
Multiple choice questions can effectively test a learner's theoretical knowledge.
Example MCQ: Which of the following is the correct syntax for a for loop?
- A) `for (i = 0; i < n; i++) {...}`
- B) `for (i < n; i++) {...}`
- C) `for int i = 0; i < n; i++ {...}`
- D) `for i in range(n) {...}`
Correct Answer: A) `for (i = 0; i < n; i++) {...}`. This format is the standard syntax in C++.
True/False Questions
These questions can quickly assess understanding.
Example: C++ supports function overloading. (True/False)
Justification: This statement is true. Function overloading allows multiple functions with the same name but different parameter lists.
Code Snippet Analysis
Presenting a piece of code for analysis helps gauge logical reasoning and understanding of C++ syntax.
Example Code Snippet:
#include <iostream>
using namespace std;
void printValue(int x) {
cout << "Value: " << x << endl;
}
int main() {
printValue(42);
return 0;
}
Question: What output does this code produce? Answer: The output will be `Value: 42`.
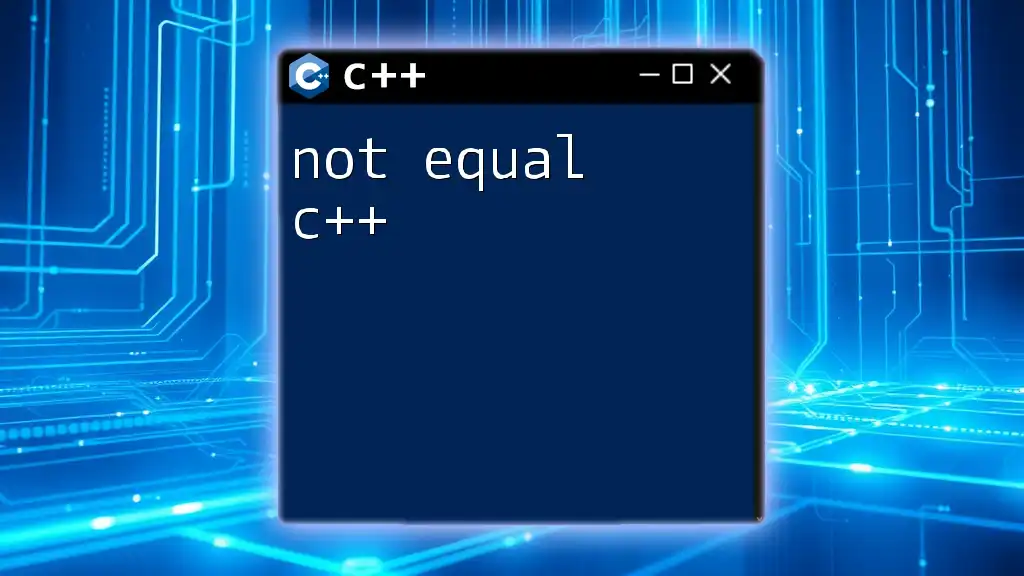
Best Resources for C++ Quizzes
Online Quiz Platforms
An array of platforms specializes in quizzes for C++. Websites like Codecademy, HackerRank, and LeetCode offer quizzes that can help assess both theoretical knowledge and practical skills. These platforms often provide features such as instant feedback, performance tracking, and community discussions.
C++ Community and Forums
Engaging with C++ forums, such as Stack Overflow and Reddit’s C++ community, not only helps in finding quizzes but also allows for discussions with peers. These platforms are excellent for sharing resources, asking questions, and discussing tricky concepts.
Books and eBooks
Several books focus on C++ and include quizzes and exercises as part of the learning process. Books like "C++ Primer" and "Effective C++" contain valuable exercises, offering readers a structured approach to mastering C++.
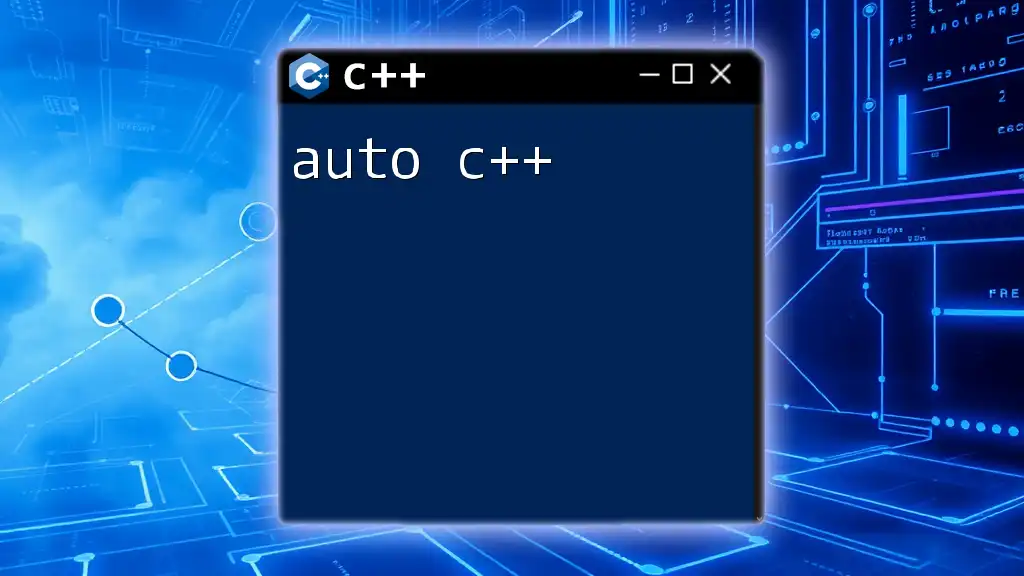
How to Set Up Your Own C++ Quiz
Defining the Objectives
Before creating a quiz, define your objectives. Are you targeting beginners or intermediate users? Do you aim to cover specific topics like memory management or templates? Identifying these factors helps tailor the quiz effectively.
Choosing a Quiz Format
Decide on the format of your quiz, which could be online, paper-based, timed, or untimed. Each format has its own advantages—online quizzes can offer immediate scoring, while paper quizzes may encourage deeper thought due to the lack of instant feedback.
Implementing Technology for Quizzes
Various tools are available to help you create and distribute C++ quizzes. Google Forms, Quizlet, and Kahoot are excellent options that allow for visually appealing and interactive quizzes, where you can easily input questions and track results.
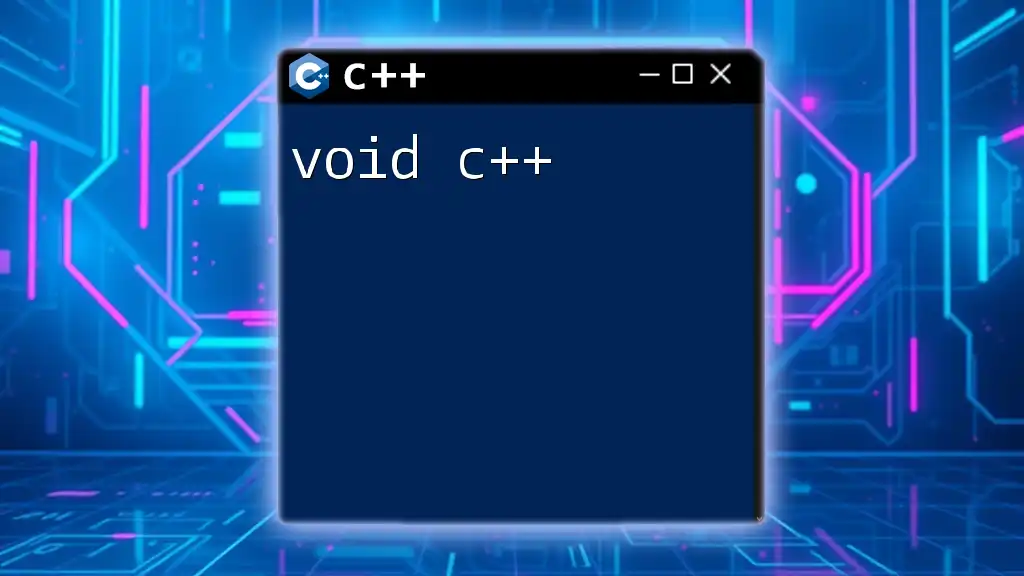
Conclusion
In summary, quizzes are a smart approach to reinforcing knowledge in C++. They challenge understanding, provide immediate feedback, and enhance retention of fundamental C++ concepts. By incorporating quizzes into your study routine, you can actively engage with the material and refine your programming skills.
Whether you create your own quizzes or take advantage of available resources, practicing through quizzes is an invaluable strategy for mastering C++. So, embark on this journey today and test your knowledge with various quizzes available to help solidify your understanding of C++.