In C++, the `void` keyword is used to indicate that a function does not return a value.
void myFunction() {
// This function does not return anything
std::cout << "Hello, World!" << std::endl;
}
What is Void in C++?
Definition of Void
The `void` keyword in C++ signifies that a function does not return any value. It essentially tells the compiler that the function's purpose is to execute some code or perform actions without providing output back to the caller. This kind of function is particularly useful in cases where the intent is to carry out a task rather than to calculate and return a value.
For example:
void myFunction() {
// This function does not return a value
}
Common Misconceptions
Many new programmers often confuse `void` with the absence of functionality or perceive it as an incomplete return type. However, `void` is a powerful and purposeful construct in C++. It simply distinguishes that a function is intended to perform actions without yielding a value directly as part of its signature. Understanding this distinction is crucial in capitalizing on the functionality `void` brings to your functions.
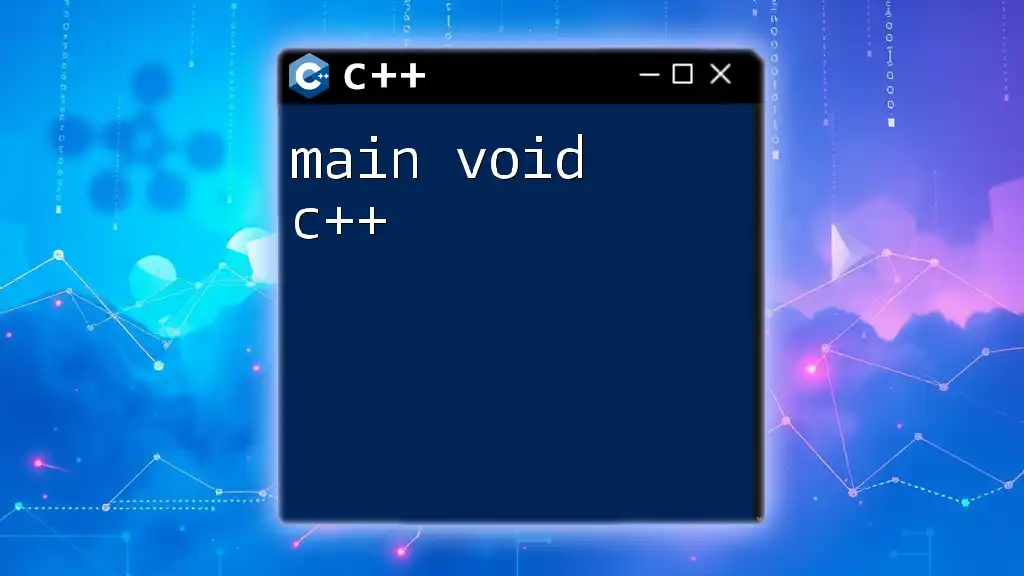
The Role of Void in C++
What Does Void Do in C++?
The void keyword plays a vital role in defining the behavior of C++ functions. It serves as an indicator that the function will execute a series of instructions but will not provide a return value. Such functions commonly handle tasks that naturally conclude without needing to provide feedback, such as outputting to the console or modifying class members.
For instance, consider the following example:
void displayMessage() {
std::cout << "Hello, World!";
}
The above function performs an action—displaying a greeting message—but does not return anything to the calling environment.
Use Cases for Void Functions
Void functions excel in scenarios where outcomes do not need to be passed back. Examples include:
- Logging: Functions may log information or errors without returning any values.
- Setup Functions: Functions that initialize parameters, settings, or objects, like configuration setups in games or applications.
- Processing User Input: Functions that handle user input directly and produce side effects, such as modifying the state of the program.
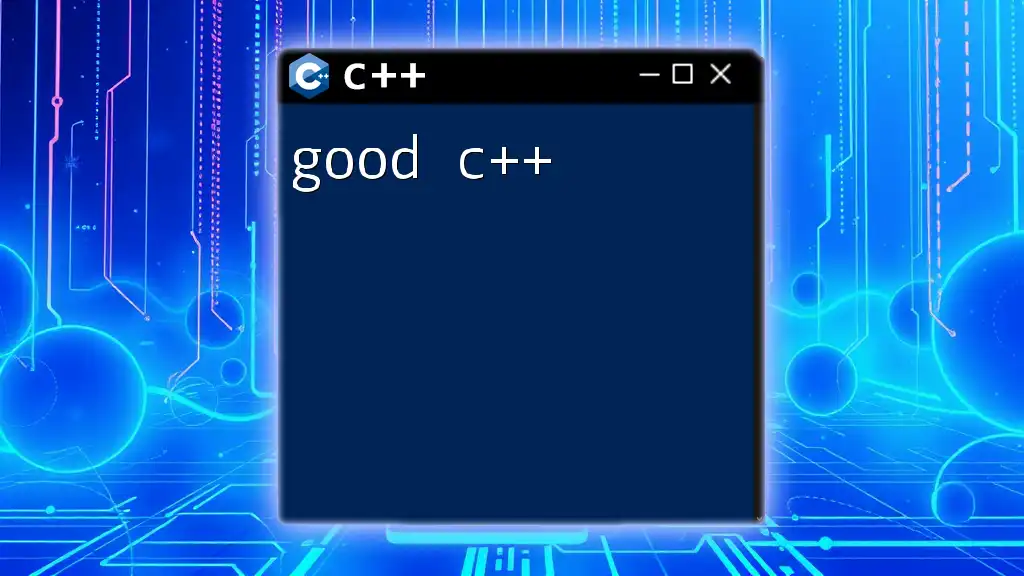
C++ Void Function: Structure and Syntax
How to Declare a Void Function
Declaring a void function is straightforward. The general syntax follows the pattern: `void functionName(parameters) { / code / }`
This pattern allows for flexibility in defining what parameters a function might require while emphasizing that it does not send a return value.
For example:
void addNumbers(int a, int b) {
std::cout << a + b;
}
In this illustration, `addNumbers` takes two integers as parameters but does not return their sum—rather, it directly prints it to the output.
C++ Void Function with Parameters
Using parameters within a void function allows for more dynamic and useful code. These parameters can be utilized to affect the behavior of the function significantly.
Here’s an example:
void printSum(int a, int b) {
std::cout << "Sum: " << a + b;
}
In this case, the `printSum` function accepts two integers and effectively outputs their sum, showcasing the flexibility of void functions to interact with runtime data without returning it.
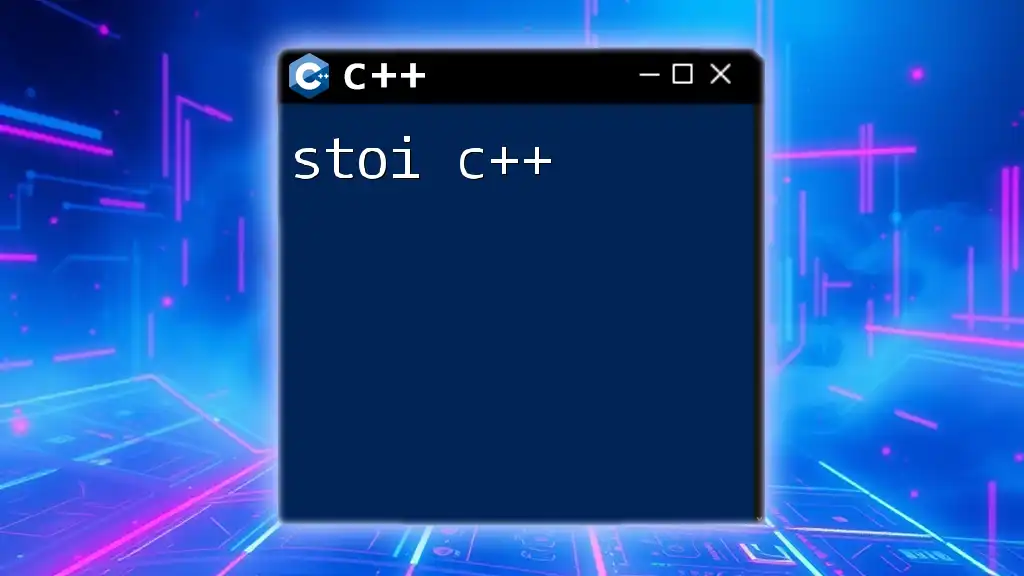
Advantages of Using Void Functions
Efficiency and Clarity
Employing void functions significantly boosts the readability and organization of code. By defining functions specifically designed to perform an action, developers can make their intentions clear, leading to less confusion when collaborating in teams or when returning to the code at a later date.
When to Use Void Functions
Choosing to use void functions is particularly beneficial in situations where return values add no discernable value. For example:
- When executing a series of output statements
- When you want to effect changes within objects or the program state without needing feedback
- In event callbacks, where the event handling itself suffices
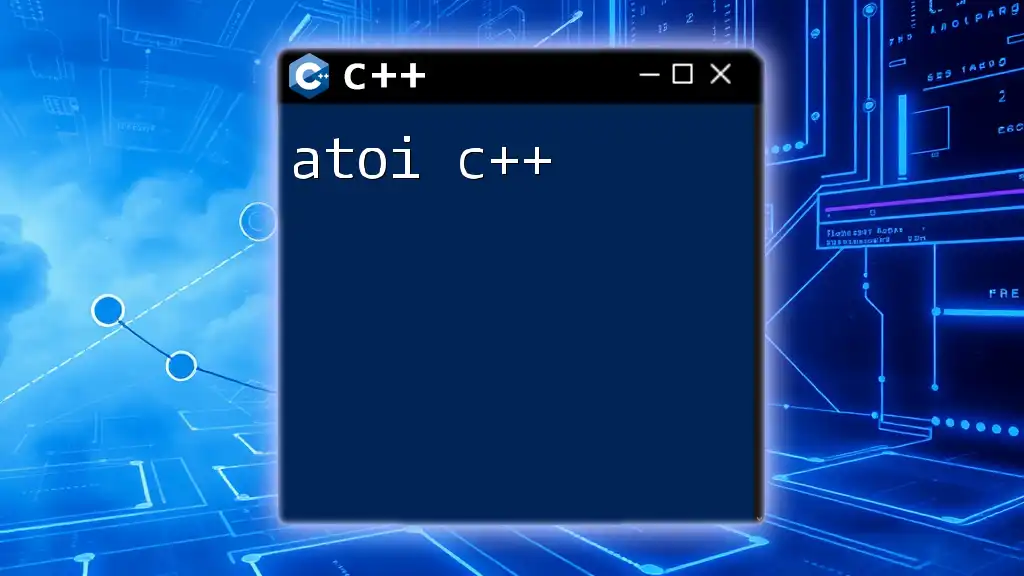
Void in C++: An Example Case Study
Implementing a Simple Application
Creating a simple C++ program allows us to see `void` functions in action. Below is an example demonstrating the use of a void function to greet a user:
void greetUser(std::string name) {
std::cout << "Welcome, " << name << "!";
}
int main() {
greetUser("Alice");
return 0;
}
In this example, the `greetUser` function takes a string parameter (the user's name) and outputs a welcoming message. The absence of a return type underscores the function's role purely as an action rather than a calculative process.
Explanation of the Example
As we can see from the code, the `greetUser` function focuses on performing a specific task: greeting the user. The absence of a return statement confirms that it excutes its purpose without needing to send any data back to its invoking context. This is a straightforward illustration of how void functions can streamline programming tasks while keeping the code clean and purposeful.
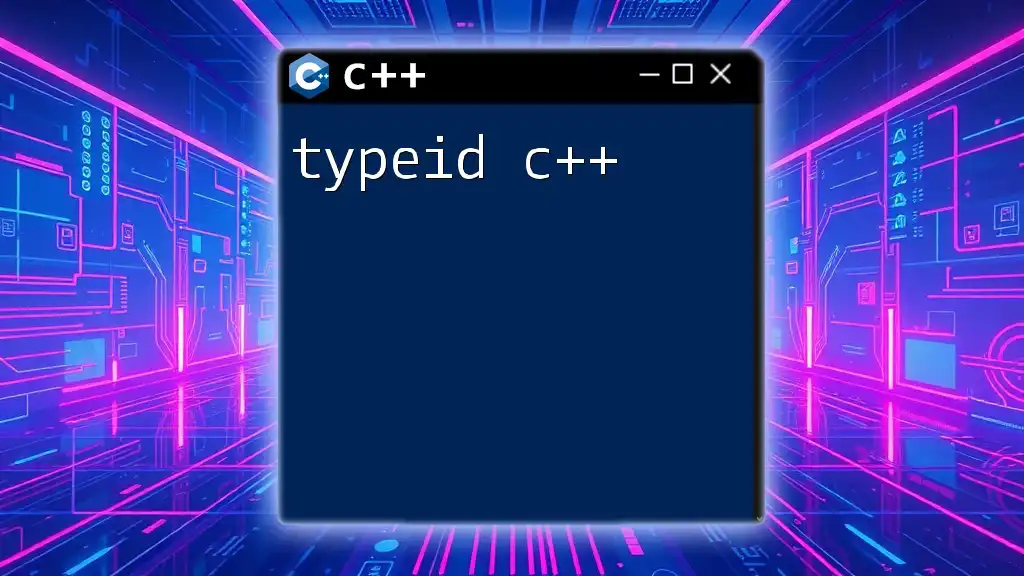
Conclusion
In summary, the `void` keyword in C++ is a crucial concept for crafting effective functions that focus on executing actions rather than returning values. By understanding its applications, advantages, and implications in code, programmers can enhance the clarity and efficiency of their applications. With practice, you will harness the power of void functions and apply them appropriately in your C++ projects.
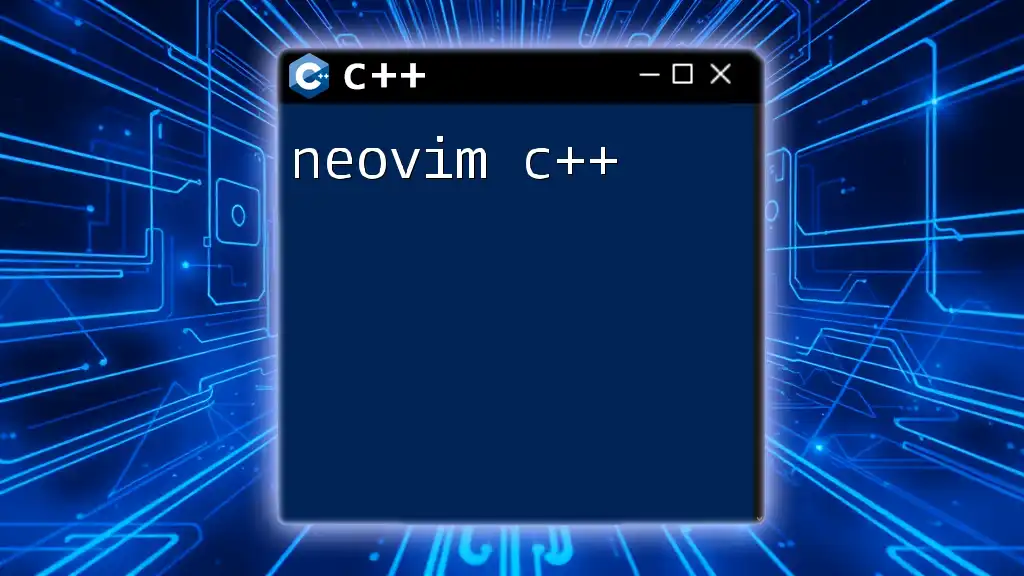
Additional Resources
For further reading, consider exploring tutorials dedicated to function implementation in C++ or reference materials that delve into advanced C++ concepts involving functions and the void type. These resources can deepen your understanding and enable experimentation with different scenarios in programming.