The `rand()` function in C++ is used to generate pseudo-random numbers, typically requiring the `cstdlib` header for access and `srand()` for seeding the random number generator.
Here’s a simple example:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed the random number generator
int randomNumber = std::rand() % 100; // Generate a random number between 0 and 99
std::cout << "Random Number: " << randomNumber << std::endl;
return 0;
}
Understanding rand in C++
The `rand()` function in C++ is a library function defined in `<cstdlib>`, which generates pseudo-random numbers. The basic syntax of the `rand()` function is straightforward, but understanding its functionality requires a deeper look.
The `rand()` function returns an integer value ranging from 0 to RAND_MAX, which is a constant defined in the `<cstdlib>` header. The value of RAND_MAX can vary by system but is typically at least 32767. It’s important to note that the numbers generated are not truly random; they are pseudo-random, meaning they are generated using a deterministic algorithm.
Random Number Generation Concepts
Random number generation (RNG) in programming typically involves algorithms that produce sequences of numbers that approximate the properties of random numbers. The `rand()` function utilizes a pseudo-random number generator (PRNG), which requires an initial value known as a seed.
The seed establishes the starting point for the number generation process. By default, the system clock can serve as a seed, but without setting it, the `rand()` function will produce the same sequence of numbers every time your program runs. This makes it crucial to seed the random number generator properly to achieve unpredictable results.
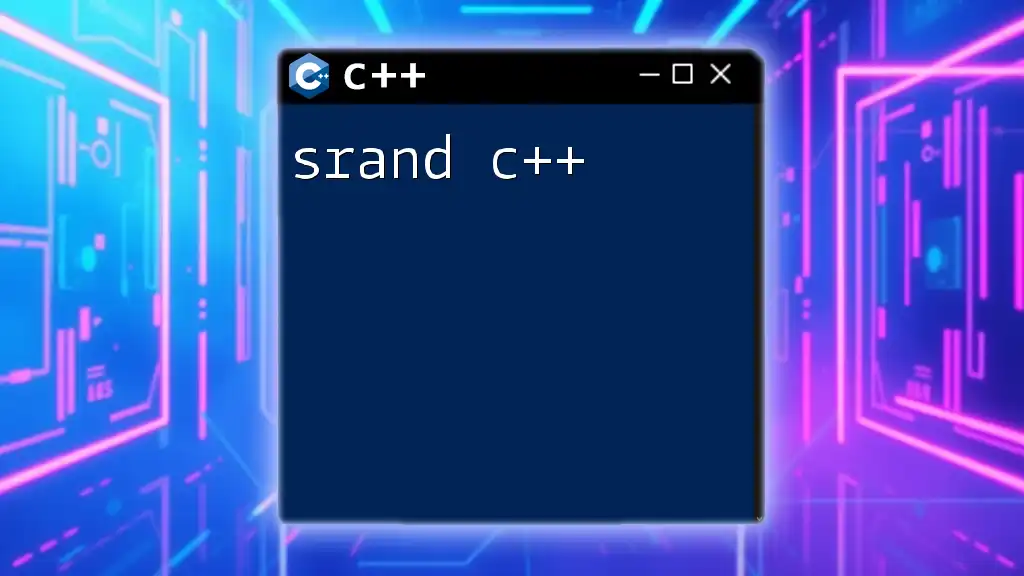
Using rand cpp Functionality
To use the `rand()` function in your program, you must include the `<cstdlib>` header. Here’s a simple way to generate a random number:
#include <iostream>
#include <cstdlib>
int main() {
std::cout << "Random number: " << rand() << std::endl;
return 0;
}
When you run this code, it will print a random number each time, but unless you seed the generator, it will repeat the same sequence on subsequent runs.
Example: Generating a Simple Random Number
To make the output more varied, you need to seed the generator with the current time. Here’s an updated example:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed with current time
std::cout << "Random number: " << rand() << std::endl;
return 0;
}
By seeding with `std::time(0)`, you ensure that the random number generator produces a different sequence of random numbers each time you run your program.
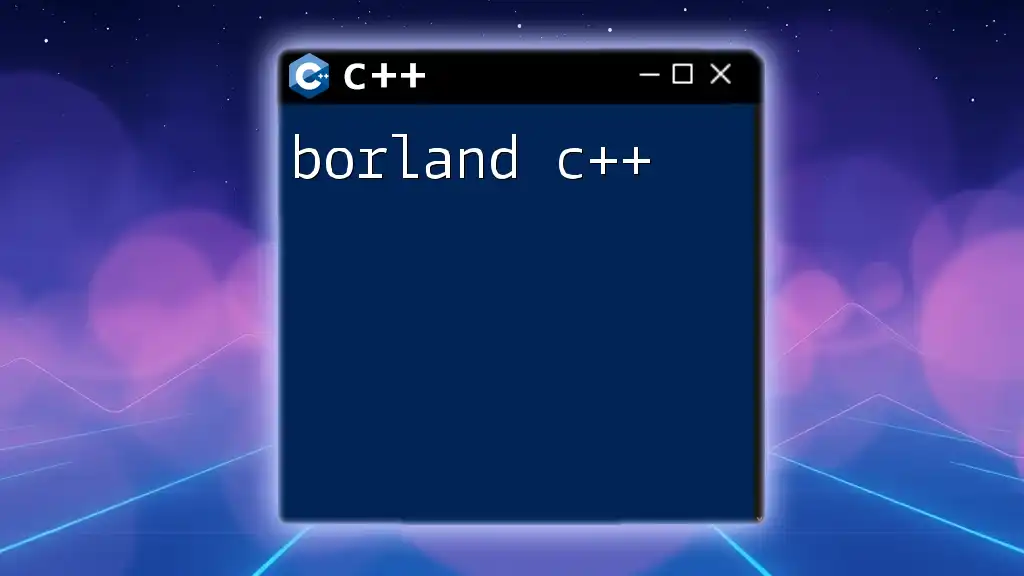
Customizing Random Number Output
Besides generating numbers, you might want to restrict the range of the numbers produced by `rand()`. To achieve this, you can use the modulo operator (`%`). This operator allows you to limit the values generated within a specific range.
Using Modulo Operator for Range
For instance, if you want to generate random numbers between 0 and 99, you can modify your code like this:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed with current time
int random_number = std::rand() % 100; // Random number between 0 and 99
std::cout << "Random number between 0 and 99: " << random_number << std::endl;
return 0;
}
In this example, using `% 100` limits the output to a value in the range of 0 to 99.
Importance of Using std::srand()
It is crucial to use `std::srand()` to set the seed value, as failing to do so will lead to predictable random numbers. Every time you run the program without seeding, the sequence of random numbers will start at the same point.
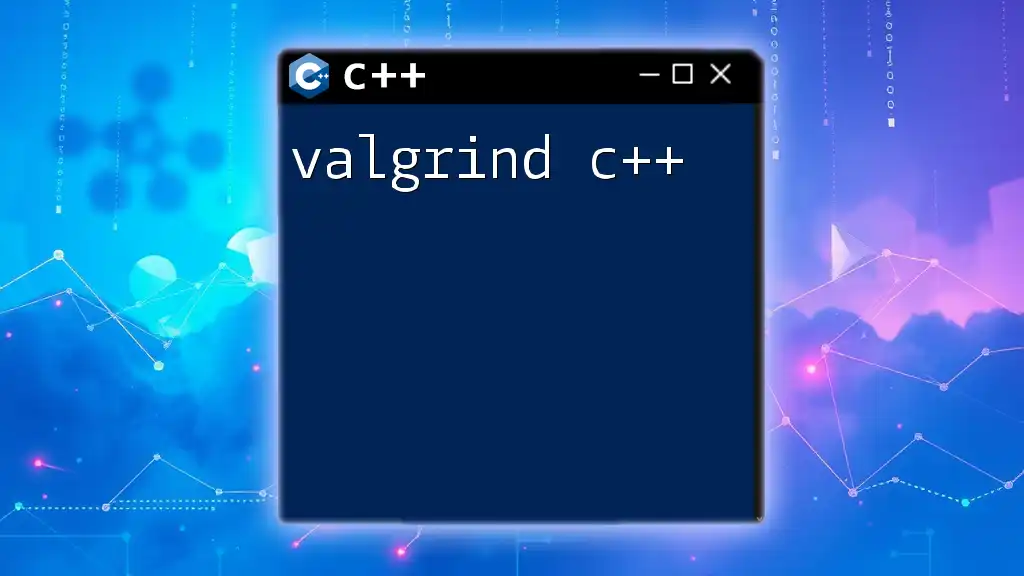
Best Practices for Using rand in C++
While `rand()` can be handy, there are some best practices to follow to avoid common pitfalls:
- Avoid Using rand() for Cryptographic Purposes: Because `rand()` generates pseudo-random numbers, it is not suitable for applications requiring strong randomness, like encryption.
- Beware of Modulo Bias: When using the modulo operator, the randomness can sometimes be biased. For example, numbers that don’t evenly divide RAND_MAX may not be uniformly distributed within the desired range.
Alternatives to rand()
For better randomness and more control, consider using the `<random>` header available in C++11 and later. This library provides modern random number generators, such as:
- `random_device`: Generates random numbers from a hardware source when available.
- `mt19937`: A Mersenne Twister engine that produces high-quality random numbers.
Example: Using random_device and mt19937
Here's a simple example using the modern C++ random library:
#include <iostream>
#include <random>
int main() {
std::random_device rd; // Obtain a random number from hardware
std::mt19937 eng(rd()); // Seed the generator with random_device
std::uniform_int_distribution<> distr(1, 100); // Define the range
std::cout << "Random number between 1 and 100: " << distr(eng) << std::endl;
return 0;
}
In this code snippet, `random_device` is used to seed `mt19937`, resulting in a more unpredictable sequence of random numbers. The `std::uniform_int_distribution` is then used to generate numbers within a specified range.
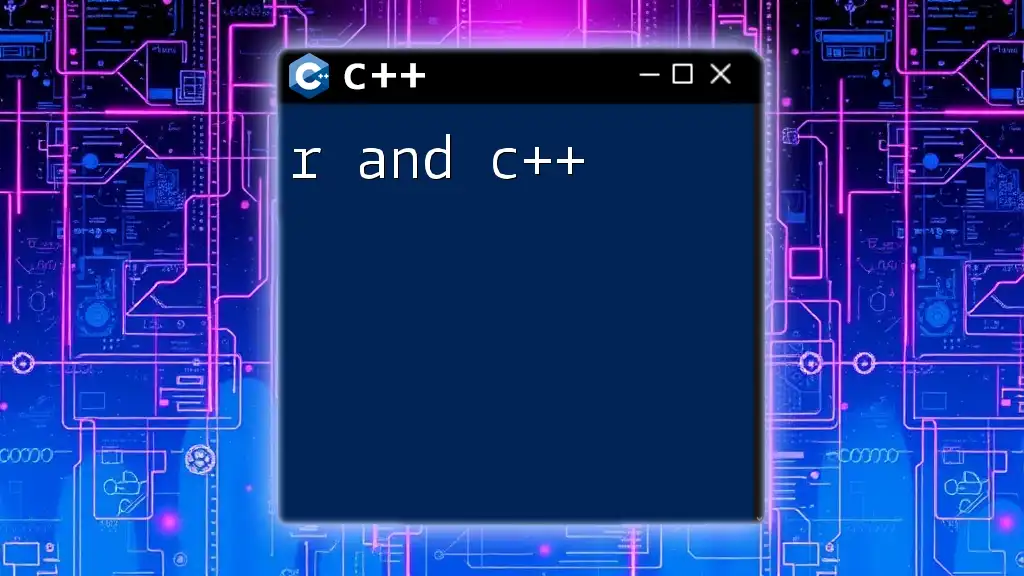
Practical Applications of Random Numbers in C++
Random numbers have a variety of applications in different fields:
- Games: Random numbers are essential for simulating dice rolls, shuffling cards, or spawning enemies in games.
- Simulations: Techniques like Monte Carlo simulations depend on randomness for modeling complex systems and forecasting outcomes.
- Cryptography: Random numbers are crucial for generating keys or initializing secure sessions in cryptographic applications.
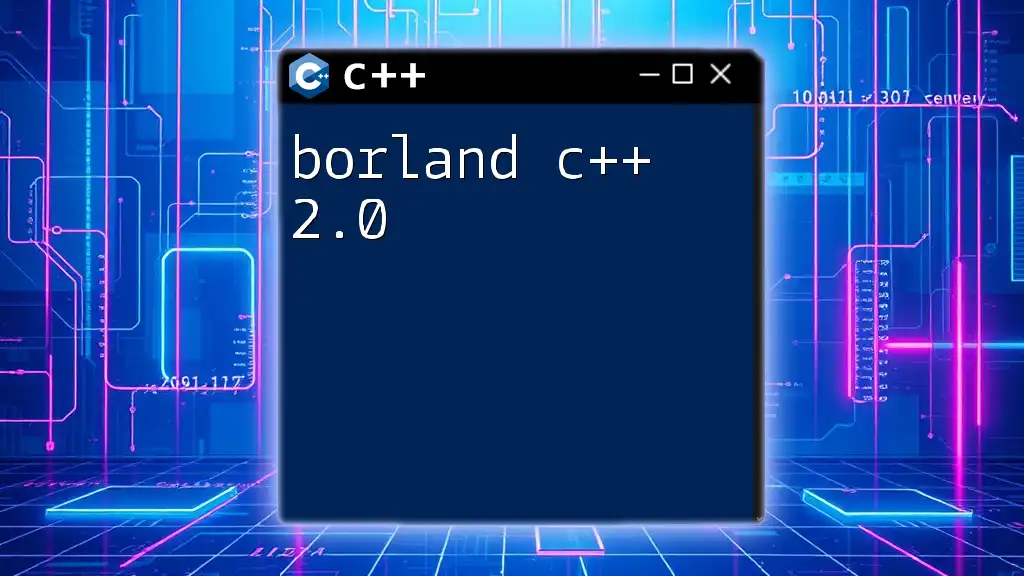
Conclusion
Understanding how to use `rand()` in C++ is fundamental for many programming scenarios, but it’s equally important to appreciate the intricacies of random number generation. By using proper seeding and alternative libraries, developers can create more varied, unpredictable, and reliable random outputs. Exploring advanced techniques and practices can enhance your capabilities in handling randomness effectively in C++.