In C++, the `add` operation can be performed easily using the `+` operator to sum two numbers, as demonstrated in the following code snippet:
#include <iostream>
int main() {
int a = 5;
int b = 3;
int sum = a + b;
std::cout << "The sum is: " << sum << std::endl;
return 0;
}
Understanding the `add` Function
What is the `add` Function?
The `add` function in C++ serves as a fundamental tool for performing addition operations. It facilitates the combination of two or more values into a singular output, often crucial in mathematical computations and data manipulations.
Significance of the `add` Function in C++
The role of the `add` function extends beyond mere arithmetic. It becomes pivotal in various contexts, including:
- Mathematical Computations: Its practical use in algorithms that require summation of values, such as in statistical calculations.
- Data Structures: Vital for functions like adding elements to containers, including arrays and vectors.
- Code Readability: A well-defined `add` function can improve the clarity and structure of your code, making it more intuitive for others to follow.
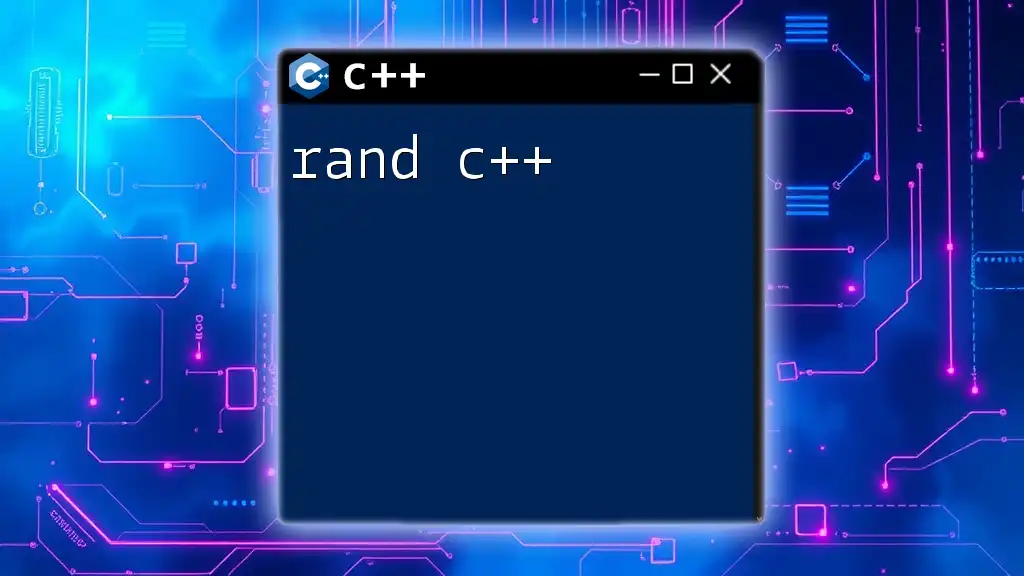
Syntax of the `add` Function
Basic Syntax
The basic syntax for creating an `add` function in C++ is straightforward. Here’s how you can define a simple function that adds two integers:
int add(int a, int b) {
return a + b;
}
This function takes two parameters, a and b, adds them, and returns the result.
Syntax Variations
Function Overloading
In C++, function overloading allows you to define multiple functions with the same name but different parameters. This is particularly useful for the `add` function, allowing it to handle different data types seamlessly:
double add(double a, double b) {
return a + b;
}
This version of `add` operates on double values, showcasing flexibility in function design.
Using Templates for Flexibility
Templates provide a powerful means of writing generalized functions. You can define an `add` function that works on any data type using templates:
template <typename T>
T add(T a, T b) {
return a + b;
}
This ensures that your function is highly flexible, able to work with different types such as integers, floats, or even custom objects, assuming they support the `+` operator.
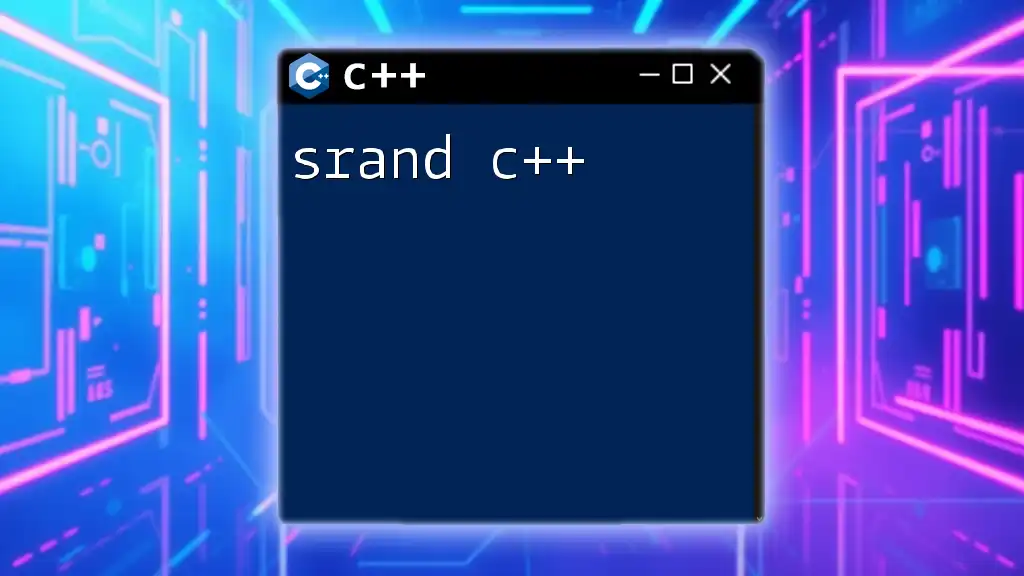
Implementing the `add` Function
Basic Implementation
Implementing the basic `add` function is simple. Below is a complete example, including a basic `main` function to demonstrate its use:
#include <iostream>
using namespace std;
int add(int a, int b) {
return a + b; // Add two integers
}
int main() {
cout << "Sum: " << add(3, 5); // Outputs: Sum: 8
return 0;
}
This code snippet clearly shows how to declare and utilize the `add` function, which outputs the sum of 3 and 5.
Implementing `add` in Real-World Applications
Using `add` in Data Structures
The `add` function can be effectively utilized to manipulate data structures. For example, consider how you can add elements to a vector:
#include <iostream>
#include <vector>
using namespace std;
void addToVector(vector<int>& vec, int value) {
vec.push_back(value);
}
int main() {
vector<int> myVector;
addToVector(myVector, 10);
cout << "Vector size: " << myVector.size(); // Outputs: Vector size: 1
return 0;
}
In this example, the `addToVector` function demonstrates how the `add` concept can be applied to dynamically manage a collection of integers.
Using `add` Function with Classes
Creating a class that implements an `add` function encapsulates behavior and state. Here’s an example:
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
};
int main() {
Calculator calc;
cout << "Sum: " << calc.add(4, 6); // Outputs: Sum: 10
return 0;
}
This example showcases how object-oriented programming principles can be applied, enabling organization of functionalities related to calculations.
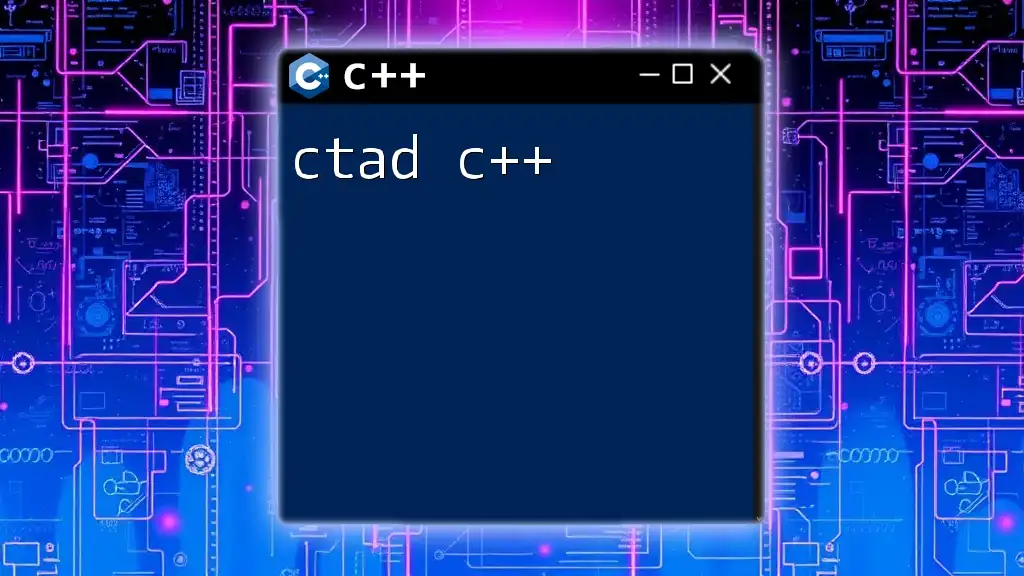
Best Practices for Using the `add` Function
Consistency in Naming Conventions
When you create functions like `add`, employing consistent naming conventions enhances code clarity. Choose descriptive names that provide immediate context about what the function does. For instance, if the purpose of the function is to add two integers, naming it `addIntegers` could be beneficial in distinguishing it from other forms of addition.
Performance Considerations
In high-performance applications, consider implementing inline functions where appropriate. This can reduce the overhead associated with function calls and improve execution speed.
Error Handling
It's crucial to address potential errors in your function. For example, an `add` function could overflow if it exceeds the limits of the data type. Implementing basic error handling can safeguard against such issues:
int add(int a, int b) {
if (a > INT_MAX - b) {
throw overflow_error("Integer overflow in addition");
}
return a + b;
}
This example includes a check for overflow, elevating the robustness of the function.
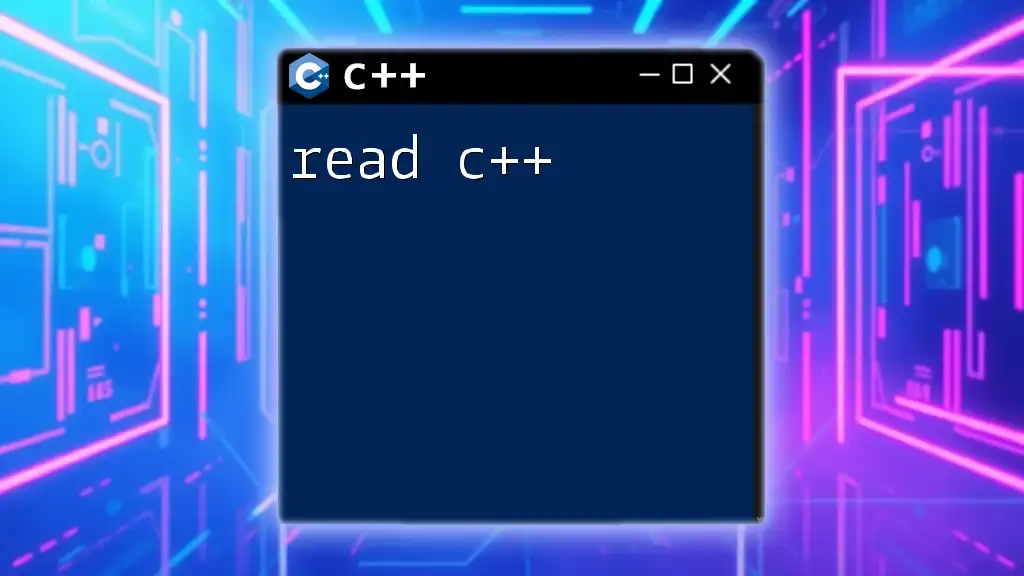
Conclusion
In summary, mastering the `add` function in C++ is essential for both beginners and seasoned programmers. It serves as a fundamental building block, applicable in various contexts, from basic arithmetic to more complex data structures. By implementing good practices and employing its versatility through overloading and templates, you can significantly enhance your coding proficiency in C++. Continue exploring the myriad possibilities C++ offers, and utilize functions like `add` to streamline your programming experience.