UML (Unified Modeling Language) is a standardized modeling language that helps visualize the design of a system using diagrams, which can assist in planning and structuring C++ code effectively.
Here’s a simple C++ class example that could be represented in UML:
class Animal {
public:
Animal(const std::string& name);
void speak();
private:
std::string name;
};
What is UML?
Unified Modeling Language (UML) is a standardized modeling language used widely in software engineering to visualize the design of a system. UML provides a way to specify, visualize, develop, and document software system artifacts, making it a crucial tool in the design phase of software development.
Benefits of using UML include:
- Improved Communication: UML provides a common vocabulary for designers, developers, and stakeholders.
- Visual Representation: It helps in picturing the architecture and design of a system, facilitating better understanding.
- Early Problem Detection: By creating UML diagrams early on, potential issues can be identified and resolved before coding begins.
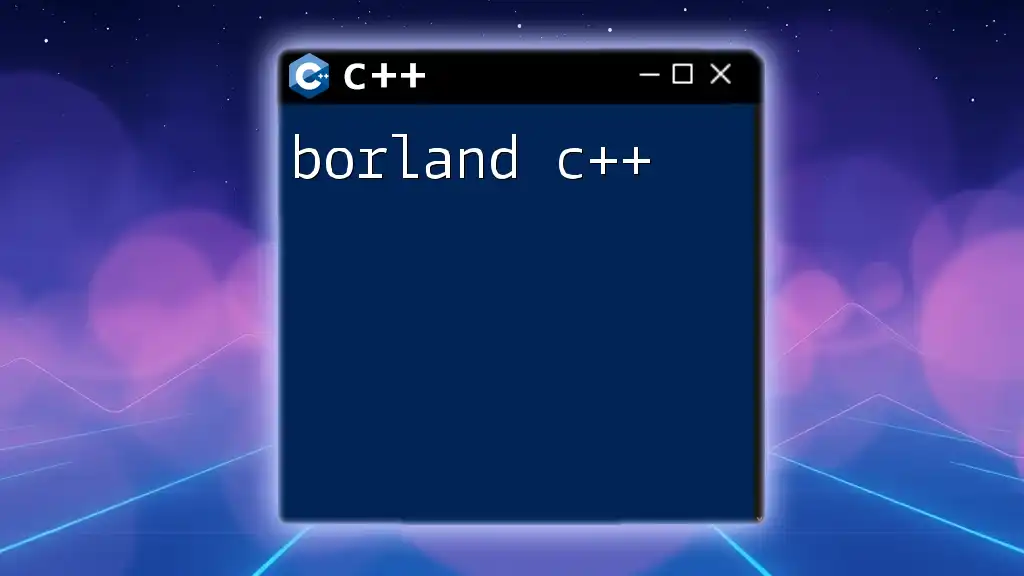
Why combine UML with C++?
Integrating UML with C++ offers several significant advantages:
- Blueprint for Development: UML diagrams serve as blueprints before developers start coding. They help in laying a clear foundation for the program structure and behavior.
- Clarity in Design: With UML, developers can visualize complex relationships and interactions within the system, leading to clearer and more organized code.
- Maintenance Ease: Well-documented UML diagrams assist in understanding existing systems, making maintenance more manageable.
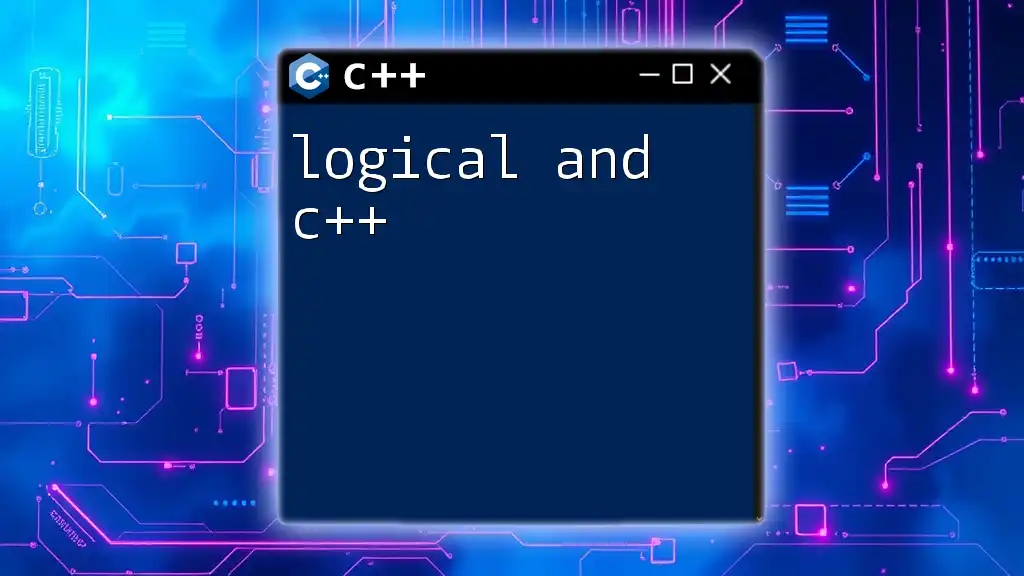
Understanding UML Components
Key UML Diagrams
Class Diagram
A class diagram is a static representation that describes the structure of a system by showing the system's classes, their attributes, methods, and the relationships among objects. For instance, a library management system's class diagram might include classes like `Book`, `Member`, and `Library`.
class Book {
public:
string title;
string author;
void borrow();
};
class Member {
public:
string name;
void registerMember();
};
class Library {
public:
void addBook(Book book);
};
Sequence Diagram
A sequence diagram illustrates how objects interact in a particular scenario of a use case. It shows the order in which messages are exchanged between objects.
For a C++ application, a sequence diagram might show the following:
- `Member` requests to borrow a `Book`
- `Library` checks availability
- if available, `Library` allows `Member` to borrow
Member -> Library: Borrow Book
Library -> Member: Check Availability
Library -> Member: Borrow Successful
Use Case Diagram
A use case diagram depicts the various interactions between users (actors) and a system. For an online shopping system, it might look like this:
Actors:
- Customer
- Admin
Use Cases:
- Search Products
- Add to Cart
- Make Payment
This helps in defining the functionalities provided by the system.
Activity Diagram
An activity diagram represents the flow of control or data in a system. An example might be the steps involved in a user logging into a system.
Start
|
Enter Credentials
|
[Validate Credentials]
/ \
True False
| |
Access Retry
|
End
UML Notation
Basic UML Symbols
Common UML symbols include:
- Classes represented as rectangles with three compartments: name, attributes, and methods.
- Interfaces shown as circles or rectangles with a «interface» stereotype.
- Associations depicted with lines connecting classes with optional multiplicity indicators.
Relationships in UML
Understanding the relationships between UML elements is vital. The main types include:
- Inheritance: Indicates a parent-child relationship. In C++ it is represented using the `:` operator.
class Animal {
public:
void eat();
};
class Dog : public Animal { // Inheritance
public:
void bark();
};
- Association: A general connection between classes, such as a `Member` having a relationship with `Book`.
class Member {
Book* borrowedBook; // Association
};
- Aggregation: Represents a whole-part relationship, suggesting that the lifetime of the part is independent of the whole.
- Composition: A stronger form of aggregation where the part cannot exist independently of the whole.
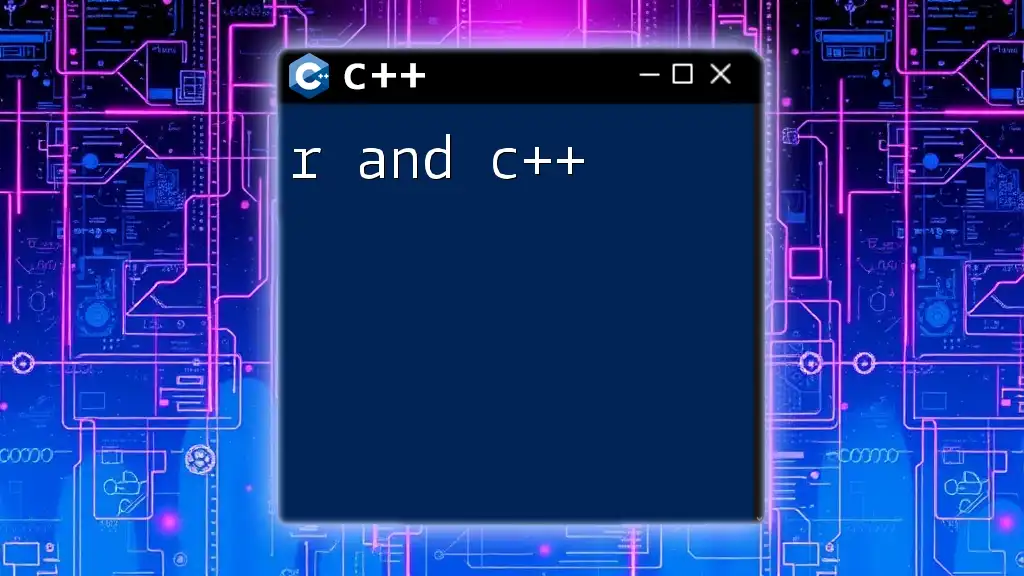
Translating UML Diagrams to C++
Class Implementation
Mapping Classes and Attributes
When transitioning from UML class diagrams to C++ class definitions, it's essential to ensure that attributes and methods translate directly.
For instance, from a UML class diagram showing a `Book` with a `title` and `author`, it can be implemented as follows:
class Book {
public:
std::string title; // Attribute
std::string author; // Attribute
void borrow() { // Method
// Implementation for borrowing
}
};
Implementing Methods
UML operations need to be translated into C++ methods. For example, a `borrow` method in UML corresponds to:
void Book::borrow() {
// Implementation logic for borrowing the book
}
Handling Relationships
Inheritance in C++
Inheritance depicted in UML can be implemented using C++’s inheritance feature. Below is a simple example showing how to inherit properties and behaviors from a base class.
class Vehicle {
public:
void start();
};
class Car : public Vehicle { // Inherits Vehicle
public:
void drive();
};
Composition vs. Aggregation
To illustrate composition and aggregation, consider the following C++ snippets:
Composition Example:
class Engine { /* details */ };
class Car {
Engine engine; // Part of Car
};
Aggregation Example:
class Driver { /* details */ };
class Car {
Driver* driver; // Driver can exist independent of Car
};
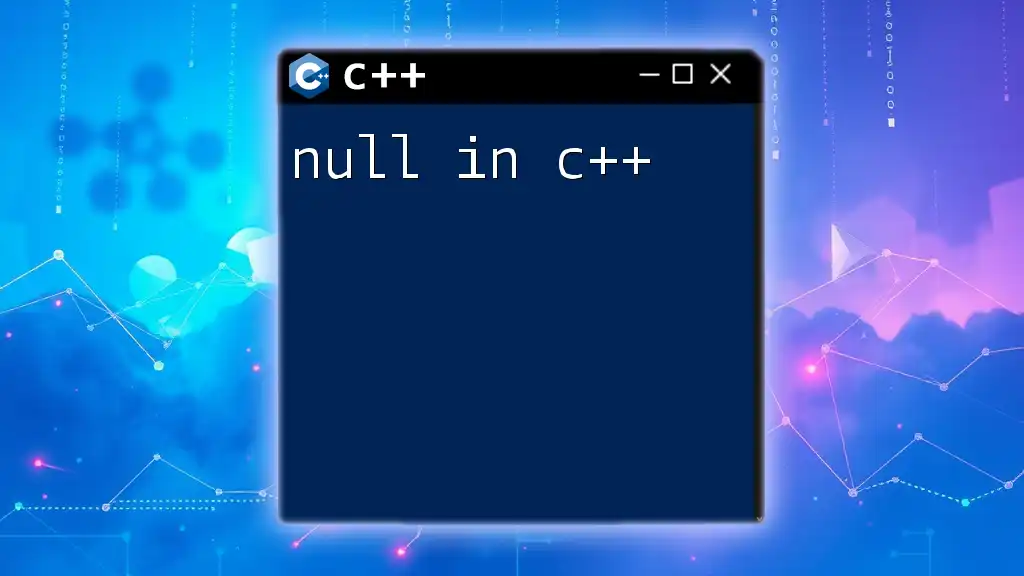
Advanced UML Techniques for C++
Design Patterns in UML
Common Design Patterns
Design patterns are reusable solutions to common problems in software design. Key design patterns include:
- Singleton: Ensures a class has only one instance.
- Factory: Defines an interface for creating an object, but lets subclasses alter the type of created objects.
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified.
For instance, a Singleton might look like this:
class Singleton {
public:
static Singleton& getInstance() {
static Singleton instance;
return instance;
}
private:
Singleton() {} // Private constructor
};
Implementing Design Patterns in C++
Drawing from UML diagrams representing design patterns, implementations in C++ often take the form of distinct classes exhibiting the pattern’s fundamental principles.
class Factory {
public:
virtual Product* createProduct() = 0;
};
class ConcreteFactory : public Factory {
public:
Product* createProduct() override {
return new ConcreteProduct();
}
};
Model-Driven Development with UML
Introduction to Model-Driven Development (MDD)
Model-Driven Development (MDD) leverages models (often UML) as the primary artifacts in the development process, emphasizing the transformation from models to code.
Tools for MDD with UML and C++
Popular tools that support MDD in UML and C++ development include:
- Rational Rose: A tool that provides UML capabilities and integrates with C++ development.
- StarUML: An open-source UML modeling tool that is highly customizable.
Code Generation from UML Models
Many modern tools can automatically generate C++ code from UML models, streamlining the development process and ensuring consistency between design and implementation.
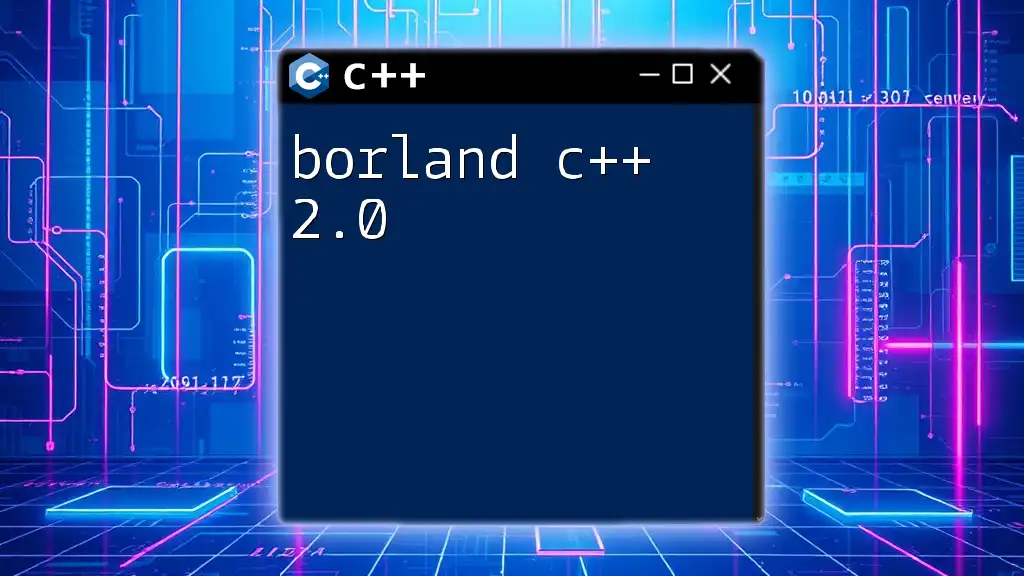
Best Practices for Using UML with C++
Effective Documentation
Maintaining Clarity
It's crucial to maintain clarity in both UML diagrams and C++ code. Well-structured and meaningful diagrams can significantly reduce misunderstandings during development.
Version Control
Just as with code, version control for UML diagrams is important. Use tools that support versioning to track changes and facilitate collaboration among team members.
Common Pitfalls and How to Avoid Them
Overcomplicating UML Diagrams
Keep UML diagrams simple, focusing on the essential elements necessary for understanding the system. Avoid cluttering diagrams with excessive details that could confuse rather than clarify.
Mismatch Between UML and C++ Code
Consistency is key. Regularly revise your UML diagrams to reflect any changes made in the C++ code to ensure that documentation accurately represents the implemented system.
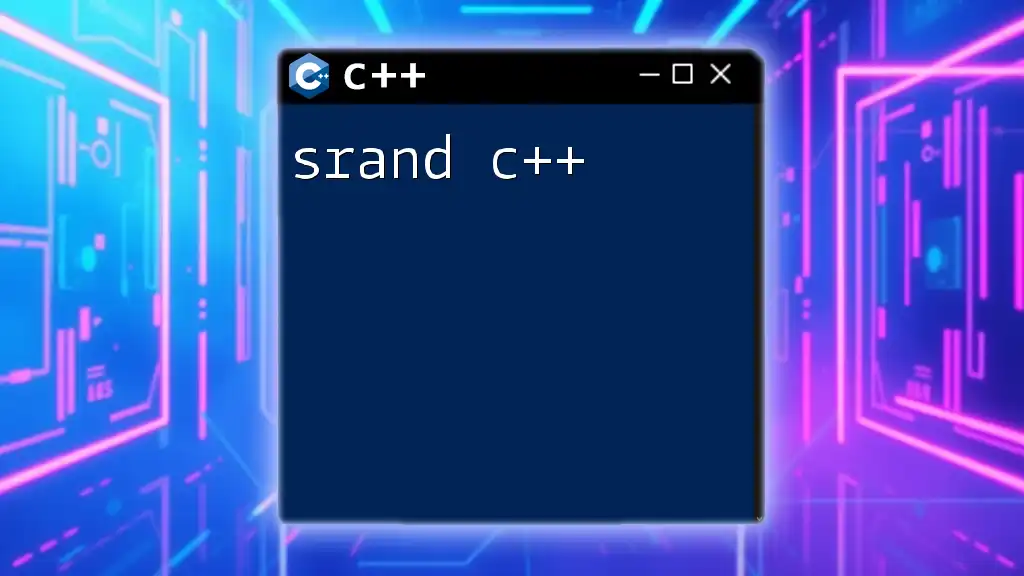
Conclusion
The effective combination of UML and C++ can significantly enhance software design and development. By understanding UML components, translating diagrams into C++ code, and maintaining good documentation practices, developers can create well-structured, scalable, and maintainable C++ applications. Start implementing UML techniques in your C++ projects, and experience the benefits of clear design and effective communication in your development process.
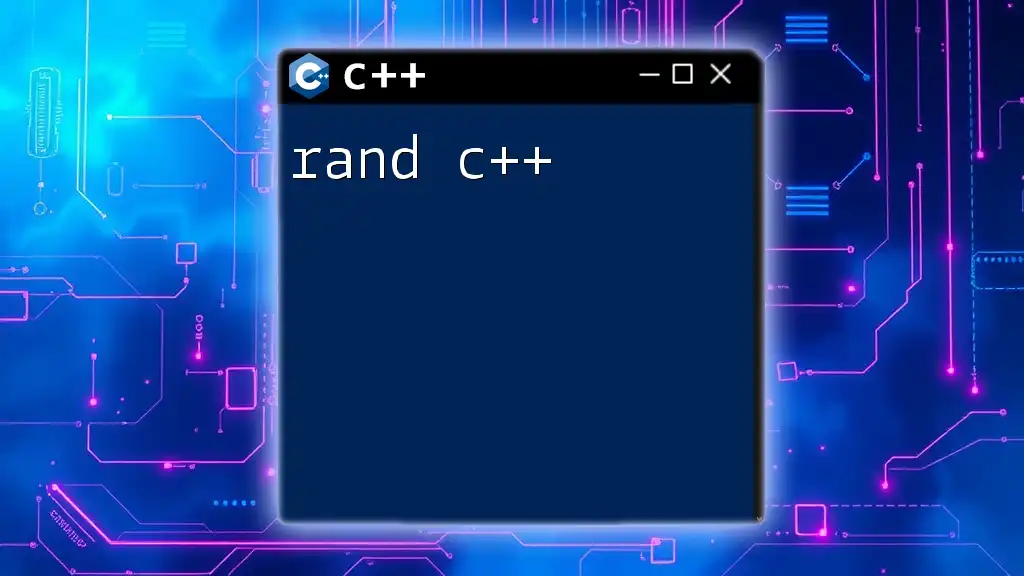
References
Books and Resources:
- "UML Distilled" by Martin Fowler
- "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides
Tools and Software:
- Rational Rose
- StarUML
- Visual Paradigm