"Playground C++" refers to an interactive environment where users can write, compile, and run C++ code snippets quickly to experiment, learn, and test programming concepts.
Here’s a simple example of a C++ program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Playground C++
What is Playground C++?
Playground C++ refers to an online or local environment where users can write, compile, and execute C++ code snippets in an interactive manner. These platforms provide a convenient way for learners and professionals to engage with the language without the need for an extensive setup.
Importance in Learning C++: The Playground allows new programmers to experiment with code, identify errors, and observe output instantaneously. This real-time feedback enhances the learning experience and builds confidence as users see the results of their code right away.
Key Benefits of Using Playground C++:
- Accessibility: Users can access code playgrounds from any device with internet connectivity.
- Ease of Use: No installation or configuration is required to start coding.
- Instant Feedback: Immediate execution of code helps in grasping concepts quickly.
Why Use Online Playground?
Using an online playground for developing in C++ streamlines the coding process and offers unique advantages:
Accessibility and Convenience: Online playgrounds can be accessed from anywhere, allowing learners to practice C++ on-the-go. This can be particularly useful for students or working professionals looking to improve their coding skills in their spare time.
Real-Time Feedback on Code: The ability to execute code immediately allows users to visualize outcomes and troubleshoot errors on the spot. This is especially beneficial for beginners who may struggle with debugging.
Examples of Popular Online Playgrounds: Notable platforms that provide a C++ playground include:
- Repl.it: A collaborative environment supporting numerous programming languages, including C++.
- Ideone: An online compiler and debugging tool for C++ and many other languages.
- CompileHero: A simple tool for compiling and executing C++ code snippets.
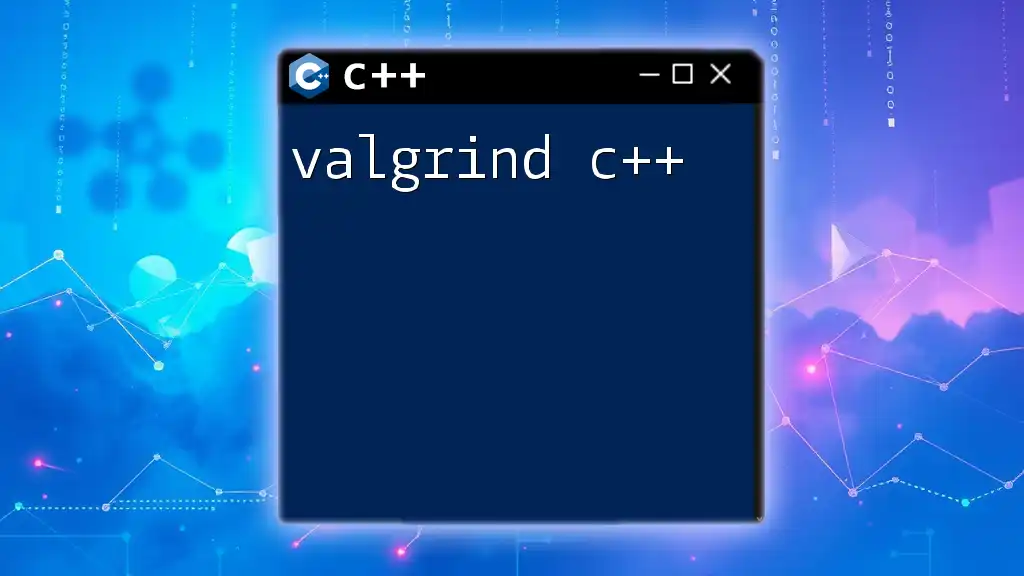
Getting Started with Playground C++
Setting Up Your Environment
How to Access a C++ Playground:
To get started, you can navigate to one of the popular online playgrounds mentioned above. Here's a simple step-by-step guide to accessing a C++ playground:
- Open your preferred web browser.
- Type the URL of your chosen playground (e.g., `https://repl.it/languages/cpp`).
- Create a free account if necessary, or use it as a guest.
Types of C++ Playgrounds Available:
- Browser-based Playgrounds: Tools like Repl.it and Ideone allow you to write and compile C++ directly in your browser.
- Local IDE Platforms: Options like Visual Studio Code or Code::Blocks can also create a local playground experience with a full installation.
Exploring Interface and Key Features
Layout Overview: Once you access a playground, you will typically encounter a clean interface divided into an editor window for your code and an output console to view results.
Key Functionalities:
-
Code Editor: A space to write your C++ code, usually with syntax highlighting and error prompts.
-
Output Console: Displays the results or errors after code execution, allowing users to quickly identify issues.
-
Sharing Options: Many playgrounds offer features to share your code with others via links, facilitating collaboration.
Basic Code Execution
Writing Your First C++ Program:
A classic starting point for any beginner is the "Hello World" program. Here's how you can write and execute this simple script in Playground C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Running the Code: Steps and What to Expect:
After writing your code, simply click the "Run" button (or equivalent) to execute it. You should see the output "Hello, World!" in the output console, showcasing your successful first program.
Understanding Compilation and Execution Messages: If there are any errors, the playground will show you the actual line where the error occurred and suggest possible fixes, helping you learn to debug effectively.
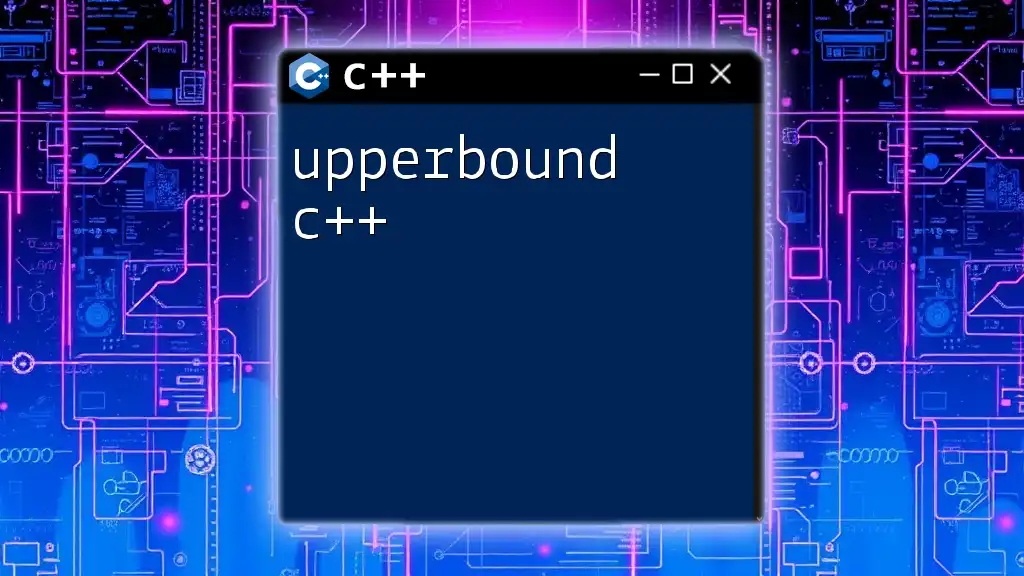
Advanced Playground Features
Customizing the Environment
Theme Selection: Light vs. Dark Mode: Most playgrounds allow users to switch between light and dark themes, which can enhance the coding experience based on personal preference.
Keyboard Shortcuts for Efficiency: Familiarizing yourself with keyboard shortcuts can drastically speed up your coding process. Common shortcuts include copy (`Ctrl+C`), paste (`Ctrl+V`), and running code (`F5`).
Utilizing Libraries and External Resources
As you get more comfortable with coding, you will want to incorporate libraries that simplify tasks:
Exploring Built-in Libraries: The standard C++ library comes with essential functionalities. For instance, using the `<vector>` library can make managing arrays easier.
Importing External Libraries: Here’s how to use the C++ Standard Template Library (STL) to manage dynamic arrays using vectors:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Debugging with Playground
Common Debugging Features: Most online playgrounds come equipped with tools that highlight syntax errors and often provide hints on how to rectify them.
Tips for Identifying Errors: Look for red underlines or error messages that indicate a compilation issue. For instance, missing semicolons are a frequent error among beginners.
Example: Common Syntax Errors and How to Resolve Them: Suppose you forget to include a semicolon at the end of a line, like this:
std::cout << "Missing semicolon"
The playground will alert you to the error, allowing you to correct it quickly.
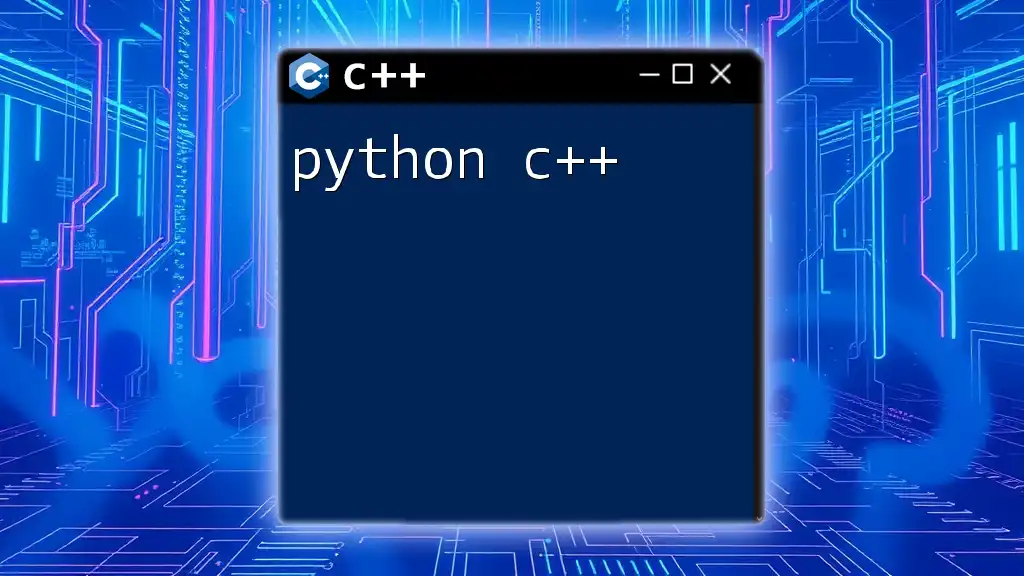
Collaborative Features
Sharing Your Code
How to Share Code Snippets: Most playgrounds offer a share feature that generates a unique URL for your code. This can be useful for collaborating with peers or seeking feedback online.
Collaborating with Others in Real-Time: Some platforms, like Repl.it, allow multiple users to edit and run code together, making group projects and learning experiences more interactive.
Example: Using Code Sharing Links: After writing code, you might click "Share," copy the link, and send it to a friend for collaboration or revisions.
Community and Resources
Engaging with the Playground Community: Many online playgrounds host communities where users can participate in forums, ask questions, and share knowledge.
Educational Resources and Tutorials Available: Platforms often have a range of tutorials and documentation designed to accelerate your learning and deepen your understanding of C++.
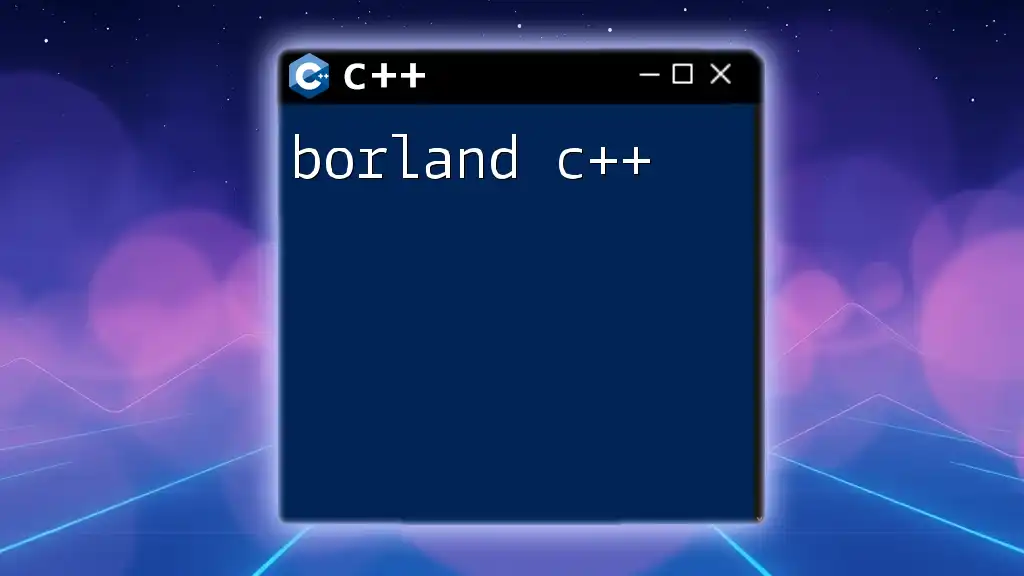
Use Cases and Applications
Learning and Practicing C++
Short Exercises and Challenges: Online playgrounds often host coding challenges and exercises tailored for various skill levels, helping users practice and reinforce learning.
Example: Simple Programming Challenges with Solutions: For instance, you may be challenged to write a program that counts the number of vowels in a string, which you can test immediately in the playground.
Real-world Applications: Developers often use playgrounds for rapid prototyping of ideas or for algorithm testing before integrating code into larger projects.
Competitions and Hackathons
Participating in Online Coding Competitions: Many coding competitions leverage playgrounds for participants to write and submit code, allowing for speedy evaluation.
Benefits of Using Playground During Hackathons: With the ability to experiment and iterate quickly, playgrounds serve as ideal environments for developing and refining ideas on the fly.
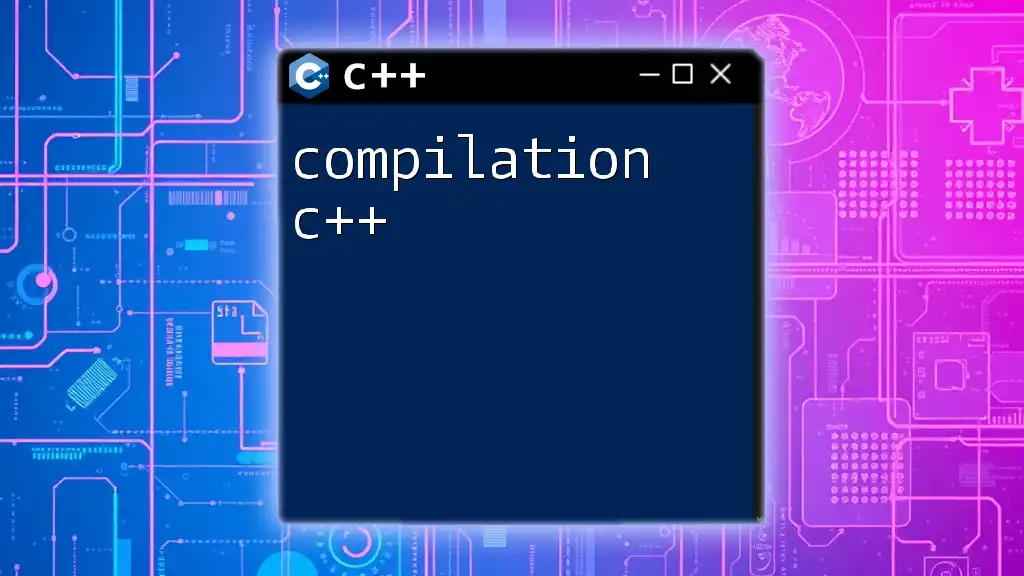
Conclusion
Future of C++ Development in Online Playgrounds
As technology continues to evolve, online C++ playgrounds are becoming increasingly sophisticated, incorporating features such as integrated AI tools for code suggestions and enhancements. These advancements signify the growing role of these platforms in the educational journey of new developers.
Final Thoughts
In summary, Playground C++ presents a unique opportunity for learners and professionals alike to explore C++, enhancing their coding skills through immediate application and feedback. Whether you are starting your coding journey or looking to refine existing knowledge, now is the perfect time to dive into Playground C++ and unleash your potential in programming.