In C++, the arrow operator (`->`) is used to access members of a class or structure through a pointer to that class or structure.
Here's an example:
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
MyClass* obj = new MyClass();
obj->display(); // Using the arrow operator to access the display function
delete obj; // Don't forget to free the allocated memory
return 0;
}
What is Arrow C++?
Arrow C++ is centered around the use of the arrow operator `->`, a pivotal feature in C++ that enables developers to work efficiently with pointers to objects. It allows for a clean and intuitive syntax when accessing members of an object through a pointer, replacing the more verbose syntax of dereferencing a pointer and then using the dot operator.
Why Use Arrow Syntax?
The primary rationale behind employing arrow syntax in C++ is to enhance conciseness and readability. C++ developers frequently deal with pointers, particularly in data structures and dynamic memory management. The arrow operator streamlines this process. For example, if you have a pointer to an object, you can access its members directly without unneeded complexity.
In real-world applications, using arrow syntax simplifies code and helps with maintenance, making it a valuable skill for any C++ programmer.
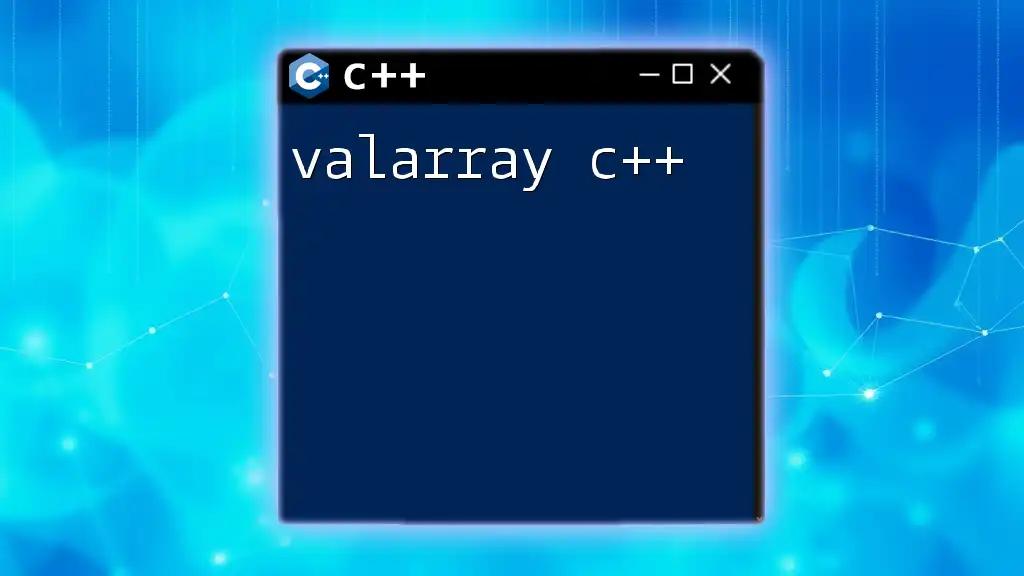
Understanding the Basics of C++ Pointer Syntax
Pointers and References Explained
Pointers are variables that store the memory address of another variable. References, on the other hand, are aliases for other variables. They provide a way to create a new name for an existing object, allowing indirect access without managing memory addresses directly.
Understanding these concepts is critical because arrow syntax primarily interacts with pointers, specifically when you're dealing with dynamic objects.
The Role of Arrow Syntax
Arrow syntax is particularly useful when accessing members of an object via a pointer. Instead of using the cumbersome notation `(*pointer).member`, the arrow operator provides a clean alternative: `pointer->member`. This improvement in syntax results in enhanced clarity and reduced errors.
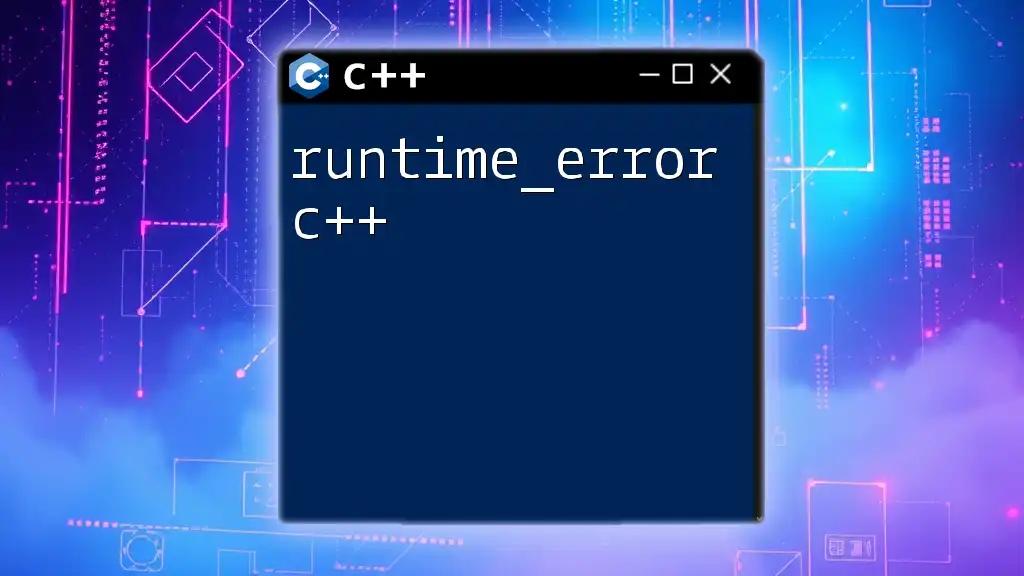
The Arrow Operator `->` in Detail
What is the Arrow Operator?
The arrow operator `->` is used to access members of a class or struct through a pointer. It significantly simplifies the syntax compared to the dot operator, which necessitates dereferencing the pointer first.
Using the Arrow Operator with Pointers
Let's look into a basic example that illustrates how the arrow operator works with class instances:
class MyClass {
public:
int value;
MyClass(int val) : value(val) {}
};
int main() {
MyClass *obj = new MyClass(10);
std::cout << obj->value; // Output: 10
delete obj;
return 0;
}
In this code snippet, we create an instance of `MyClass` dynamically and utilize the arrow operator to access the `value` member.
Arrow Operator with Function Pointers
The arrow operator is also beneficial when dealing with function pointers. Consider the following example:
void printValue(int val) {
std::cout << "Value: " << val << std::endl;
}
int main() {
void (*funcPtr)(int) = printValue;
funcPtr(20); // Output: Value: 20
return 0;
}
Here, we declare a function pointer and call the function using this pointer, showcasing another application of pointers and thus indirectly utilizing the arrow syntax.
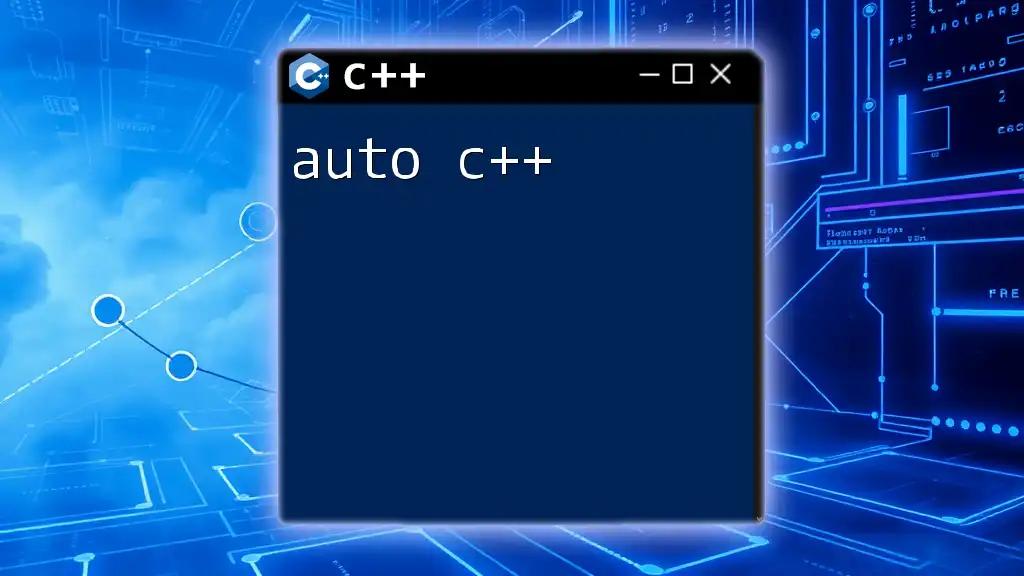
Advanced Usage of Arrow Syntax
Arrow Syntax with Data Structures
Arrow syntax shines especially when used with data structures, such as linked lists or binary trees. Consider the example of a linked list:
struct Node {
int data;
Node* next;
};
void printList(Node* head) {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
}
In this example, the `printList` function iterates over a linked list using the arrow operator to access each node’s `data` and `next` pointers.
Lambda Functions and Arrow Syntax
With modern C++ features, such as lambda functions, arrow syntax can still play a role. Here’s a concise example:
auto lambda = [](int x) { return x * 2; };
std::cout << lambda(5); // Output: 10
While we do not explicitly use the arrow operator here, the concepts of pointers and references still underpin the effective use of lambda functions, showcasing the broader impact of arrow syntax in C++ programming.
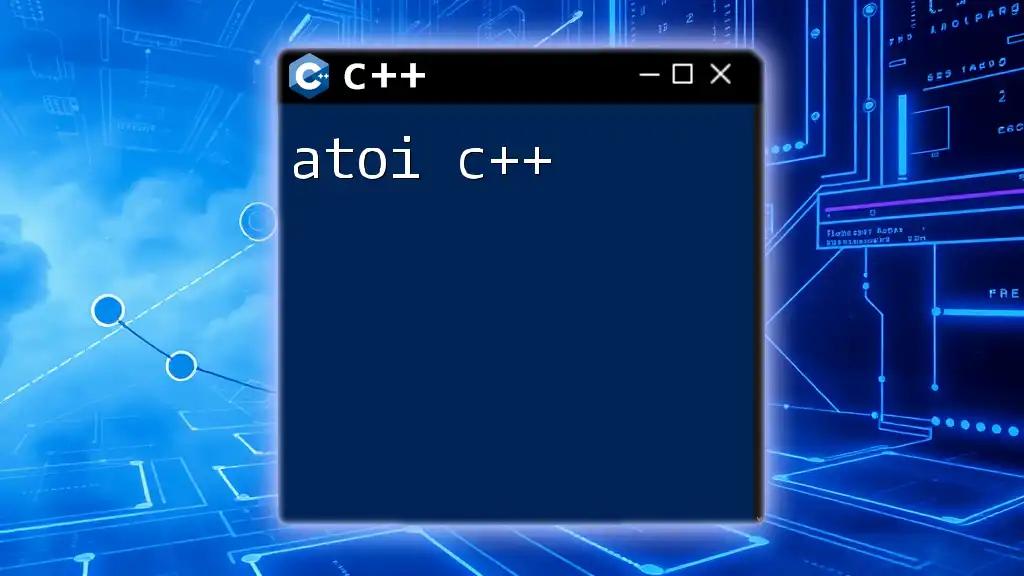
Common Mistakes with Arrow Syntax
Misusing Arrow Operator
One common mistake involves misapplying the arrow operator to non-pointer types. It's essential to remember that the arrow operator is intended for pointers; using it on non-pointer variables will lead to compilation errors.
Dereferencing Issues
Understanding when to dereference pointers is crucial. Many developers struggle with cases where they might incorrectly combine pointer dereferencing with arrow operator usage. A solid grip on how and when to apply these concepts will save you from runtime errors and undefined behaviors.
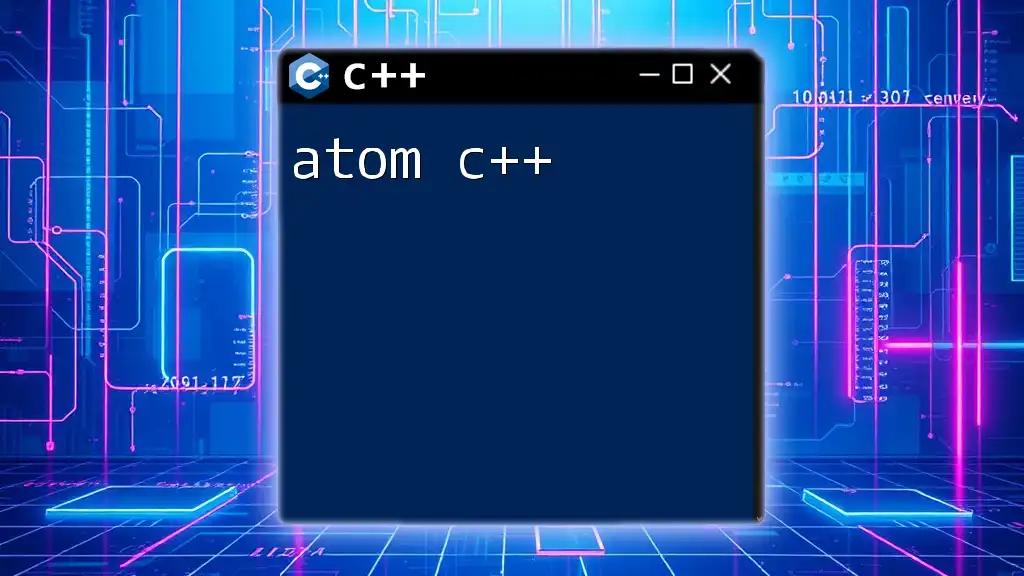
Best Practices for Using Arrow C++
Keeping Code Clean and Readable
When using arrow syntax, maintaining a clean and readable codebase is vital. Names for pointers should be intuitive, and code should be structured in a way that logically hints at the relationships between objects. This practice makes it easier for others—and yourself—to read and maintain the code in the future.
Performance Considerations
Using pointers and the arrow operator can lead to performance benefits, especially in cases where object copying is expensive. By working directly with pointers, you avoid unnecessary duplication, improving your program's efficiency.
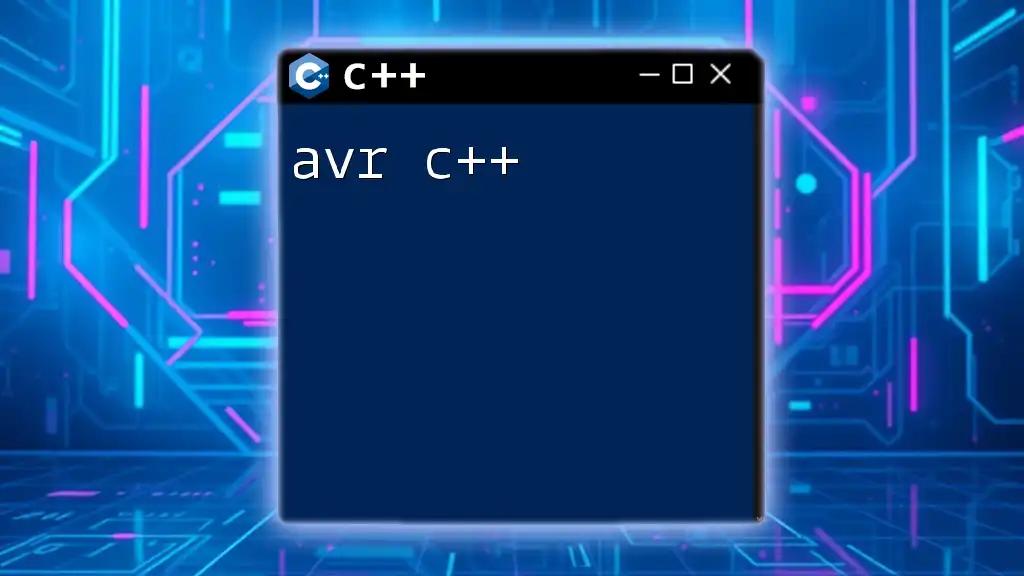
Real-World Applications of Arrow C++
Popular Use Cases
Arrow C++ techniques are prevalent in various domains, from game development to machine learning and software engineering. They help in building data structures like linked lists, trees, and heaps—essential components in many algorithms.
Case Studies
Several companies effectively utilize C++ and arrow syntax. For instance, game engines rely heavily on pointers to manage complex object relationships and maximize performance due to the real-time nature of games. Such applications illustrate the practical advantages of mastering Arrow C++.
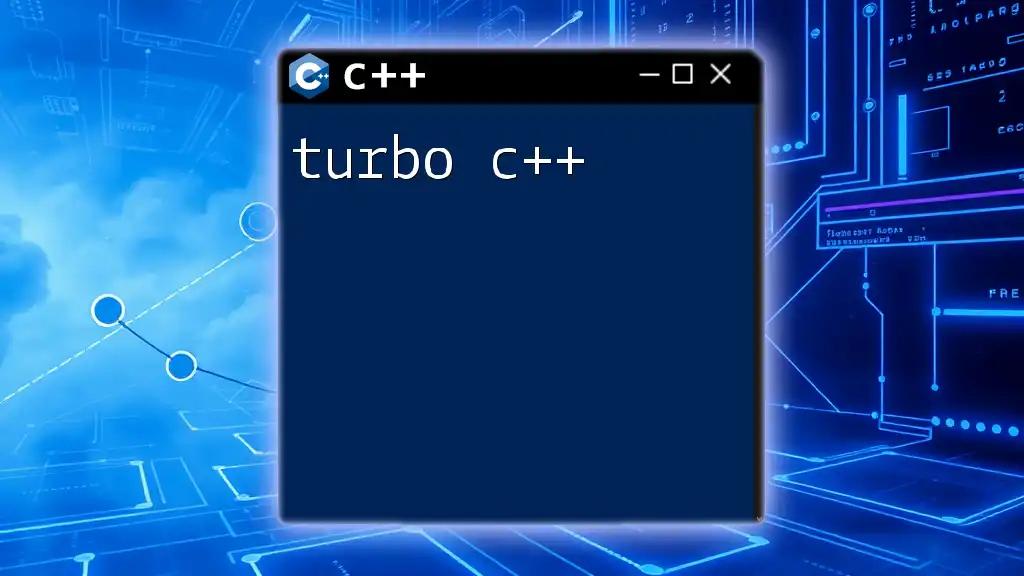
Conclusion
In summary, mastering Arrow C++ syntax will significantly enhance your C++ programming skills. From improving code readability to streamlining complex data structure manipulations, the arrow operator is a fundamental component that can greatly simplify tasks involving pointers. As you continue honing your skills, remember that regular practice is key to becoming proficient in using this powerful feature.
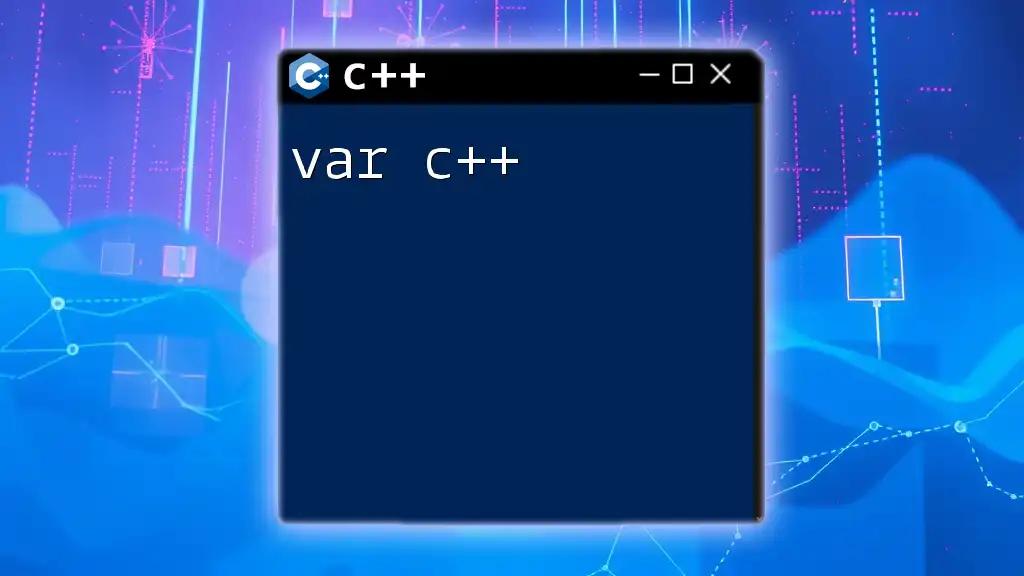
Additional Resources
For those looking to advance their understanding of arrow syntax and C++, consider exploring books and online tutorials focused on C++ best practices. Engaging in community forums also provides valuable opportunities to share knowledge and learn from other developers in the field.