Carbon C++ is a modern programming language designed to be a drop-in replacement for C++ while focusing on simplicity and safety, enabling developers to write efficient and reliable code.
Here's a simple code snippet demonstrating a basic "Hello, World!" program in Carbon C++:
// HelloWorld.carbon
package hello;
fn main() -> i32 {
print("Hello, World!");
return 0;
}
What is Carbon C++?
Carbon C++ is an innovative programming language that builds upon the foundations of the C++ ecosystem. Designed to address existing issues in C++ while maintaining familiarity for developers, Carbon C++ offers a pathway to modern programming practices. The project's primary aim is to create a language that is not only efficient but also facilitates better code maintainability and readability.
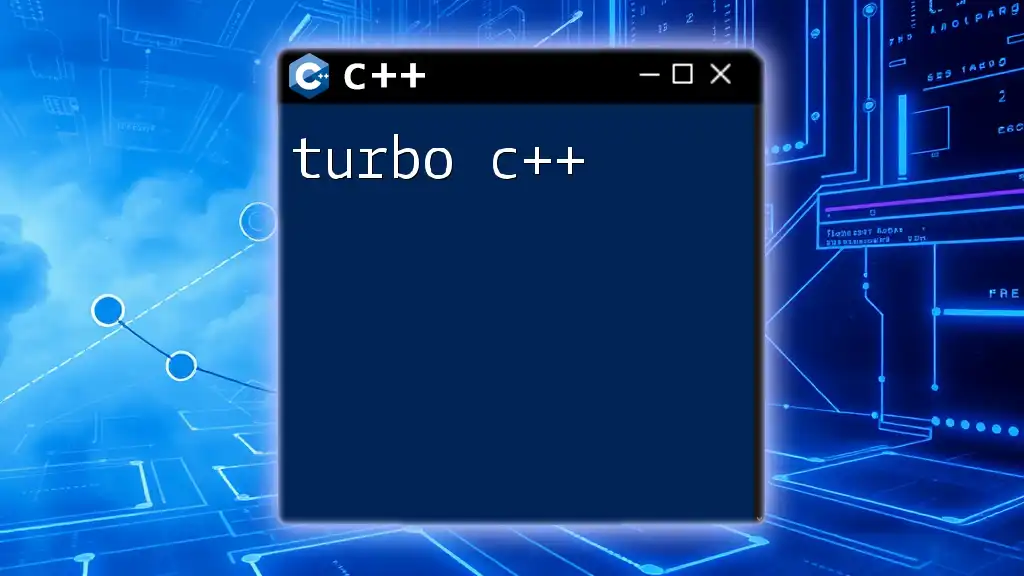
Why Carbon C++?
Despite its powerful capabilities, C++ has faced criticism related to its complex syntax and error management. Carbon C++ addresses these challenges by introducing a more modern syntax and semantics. This modern approach empowers developers to write cleaner, more understandable code, making it easier to tackle complex projects. Additionally, Carbon C++ enhances interoperability, allowing developers to utilize existing C++ codebases and libraries seamlessly.
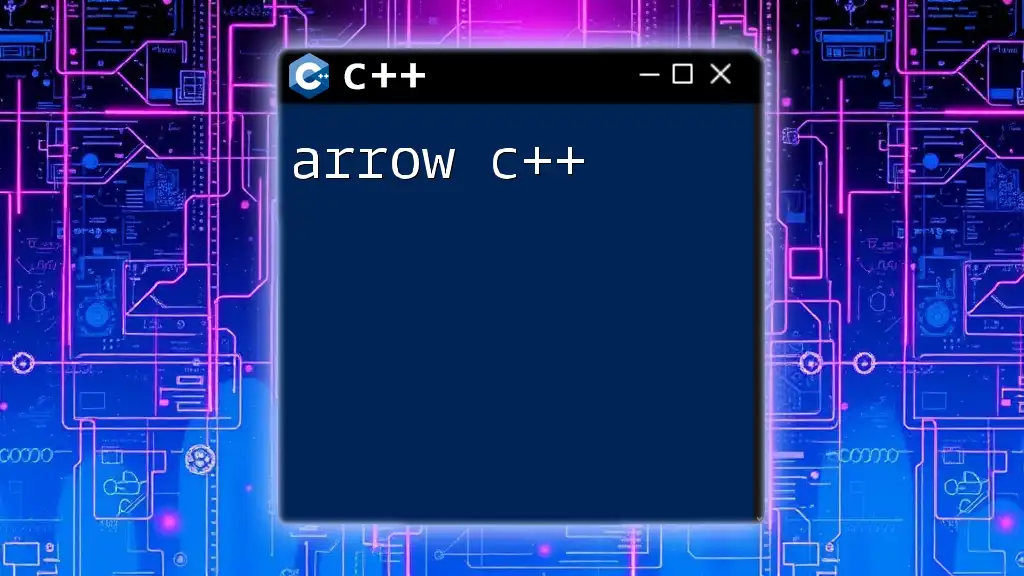
Key Features of Carbon C++
Modern Language Constructs
One of the standout features of Carbon C++ is its modern language constructs. By incorporating contemporary syntax, Carbon C++ minimizes verbosity, leading to improved code clarity. Furthermore, the language introduces enhanced error handling mechanisms, allowing developers to manage exceptions easily. This focus on simplicity translates to fewer bugs and a more robust development process.
Interoperability with Existing C++ Codebases
Carbon C++ is designed with interoperability in mind, enabling seamless integration with existing C++ applications. This means that developers can leverage established libraries without needing to rewrite substantial portions of their code. By using Carbon C++, you can maintain the benefits of existing codebases while embracing new features that enhance functionality and agility.
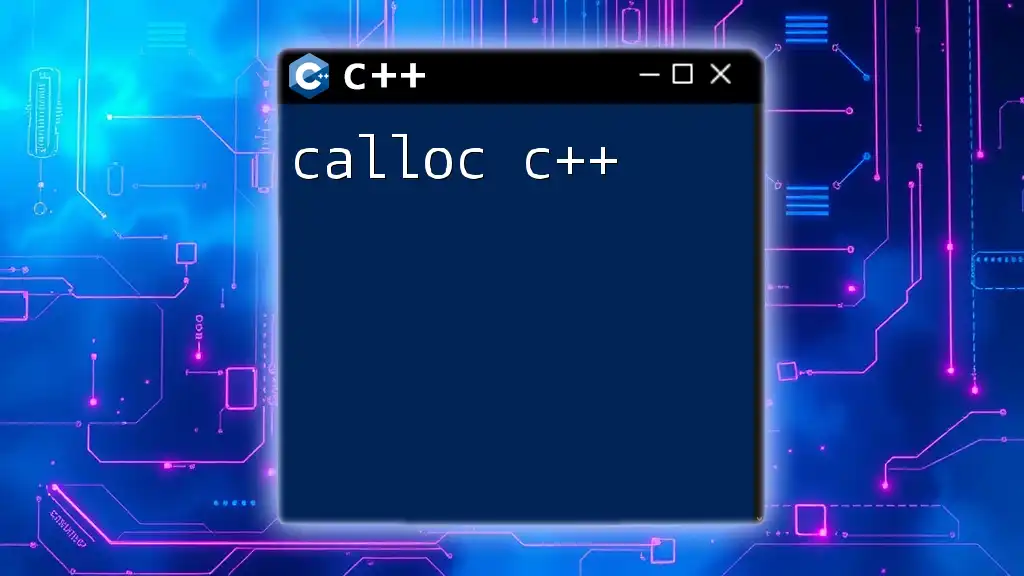
Setting Up Your Development Environment
Requirements for Carbon C++
Before diving into Carbon C++, ensure that your development environment is adequately prepared. You'll need a compatible operating system, preferably the latest version of macOS, Linux, or Windows. It's also recommended to have an up-to-date code editor or integrated development environment (IDE) that supports Carbon syntax highlighting and features.
Installation Guide
To install Carbon C++, follow these straightforward steps:
- Download the Carbon Compiler from the official source or repository.
- Extract the files to your desired location on your system.
- Set up environment variables as needed for the compiler and libraries.
- Configure your IDE appropriately to recognize Carbon-specific syntax.
For specific configuration settings, consult the official documentation, as it will provide the most accurate guidance tailored to your setup.
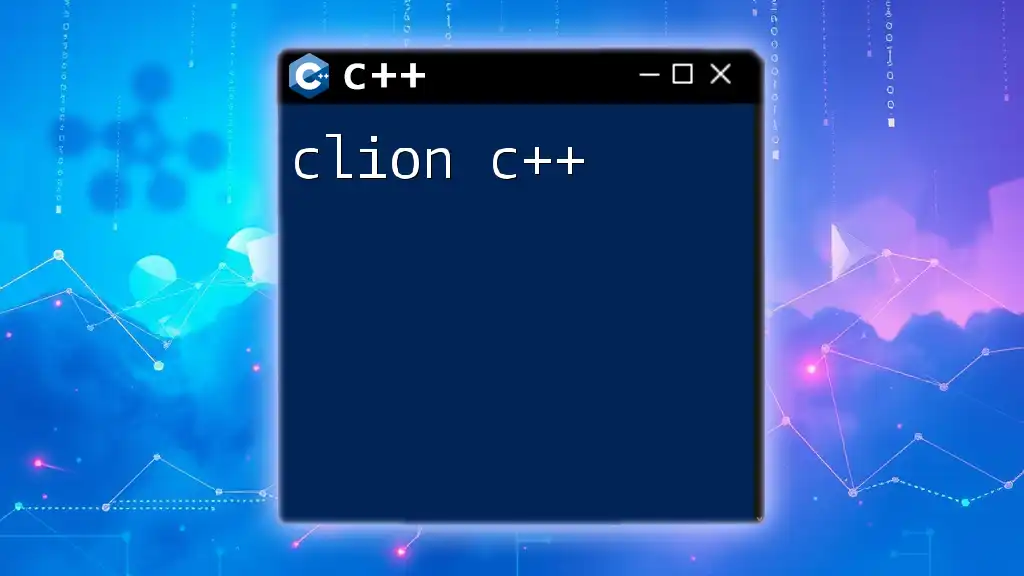
Core Syntax and Language Elements
Basic Syntax Overview
Carbon C++ offers a clean and intuitive syntax that promotes developer productivity. Here’s a brief overview of essential syntax elements:
- Variable Declarations: Variables can be declared with types that are easy to read.
var name: string = "Hello, Carbon!";
- Control Structures: Carbon C++ retains familiar control flow statements like if-else, which streamline decision-making.
// Example: Basic Control Structure
if (condition) {
// Actions when condition is true
} else {
// Actions when condition is false
}
Functions and Methods
Defining functions in Carbon C++ is straightforward, enabling developers to create reusable and modular code easily. Both regular functions and methods can be defined with clear parameter and return types.
// Example: Function Definition and Overloading
fn add(a: int, b: int) -> int {
return a + b;
}
fn add(a: float, b: float) -> float {
return a + b;
}
This approach allows developers to overload functions, providing flexibility in handling different data types.
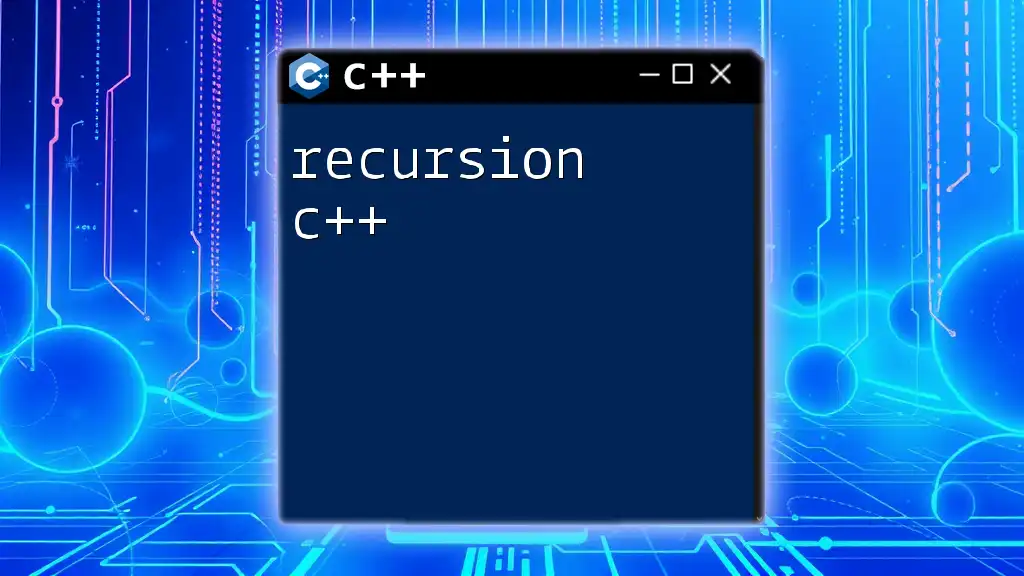
Advanced Features of Carbon C++
Memory Management in Carbon C++
One of the challenges in C++ has always been memory management. Carbon C++ introduces enhanced memory allocation strategies that alleviate some of the burdens developers typically face. While Carbon C++ allows for manual memory management, it also incorporates elements similar to garbage collection, which helps prevent memory leaks and dangling pointers.
Concurrency and Parallelism
Carbon C++ places a significant focus on leveraging modern computing capabilities, particularly in the realm of concurrency. With built-in support for multi-threading, developers can easily implement parallelism in their applications. The use of keywords such as `spawn` simplifies threading, allowing for smoother execution of concurrent code.
// Example: Basic Thread Creation
spawn {
// Code to run in parallel
println("Running concurrently!");
}
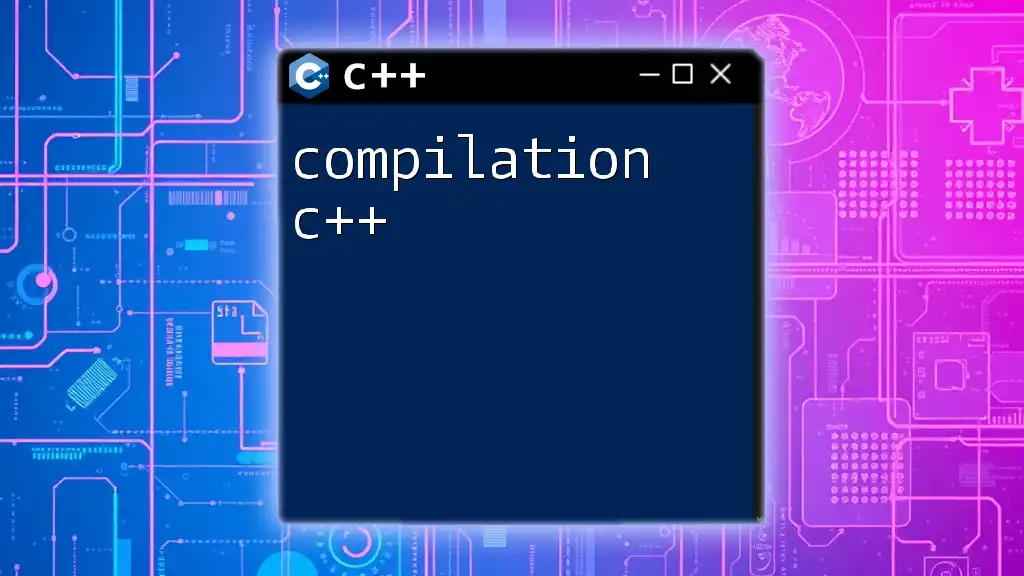
Practical Use Cases
Real-World Applications of Carbon C++
Carbon C++ showcases its capabilities across various industries, from finance to gaming. Its modern syntax and efficient performance make it suitable for projects requiring speed and accuracy. Specific case studies have demonstrated that teams adopting Carbon C++ report greater productivity and fewer bugs, leading to higher quality applications.
Carbon C++ in Game Development
Game development poses its own unique challenges, including high-performance requirements and complex systems. Carbon C++ offers tools and features that enable developers to create immersive gaming experiences without sacrificing performance. By reducing boilerplate code, Carbon C++ allows developers to focus on gameplay mechanics rather than debugging.
Applications in Systems Programming
With robust low-level capabilities, Carbon C++ is an excellent choice for systems programming. The language's performance enhancements, combined with its modern constructs, make it ideal for operating systems, drivers, and real-time applications where efficiency is paramount.
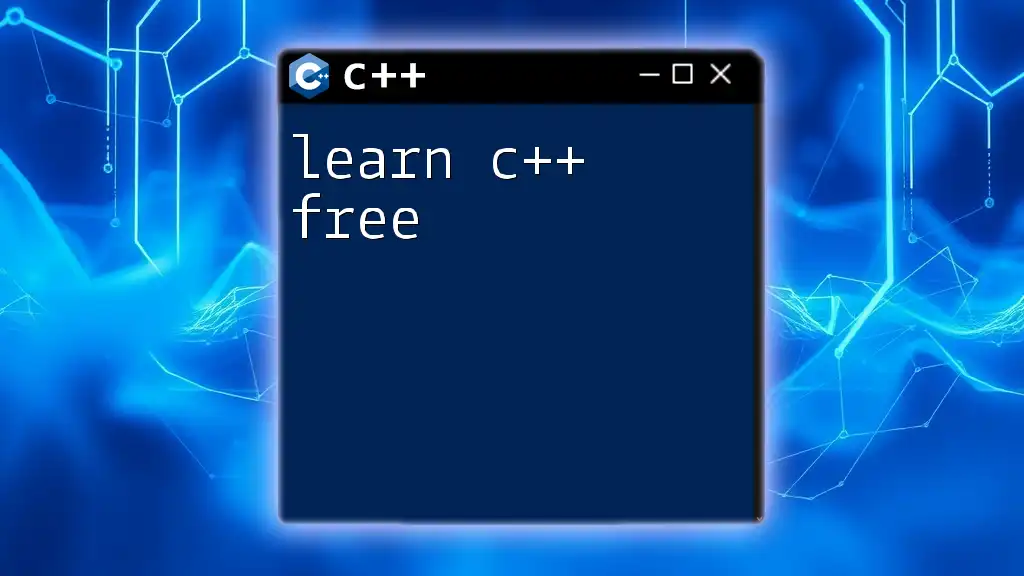
Transitioning from C++ to Carbon C++
Migrating Existing C++ Code to Carbon
Making the switch from C++ to Carbon is highly feasible thanks to the language's focus on compatibility. A well-defined migration strategy can streamline the process, allowing developers to refactor and improve existing code incrementally. Key steps include:
- Assessing current C++ code for compatibility.
- Gradually introducing Carbon features while leveraging existing logic.
- Focus on refactoring and improving error handling during the transition.
Learning Resources for Carbon C++
To facilitate your journey into Carbon C++, consider utilizing a mix of resources ranging from official documentation to community forums. Engaging in online discussions, attending webinars, and following training courses can greatly enhance your understanding of Carbon C++ capabilities.
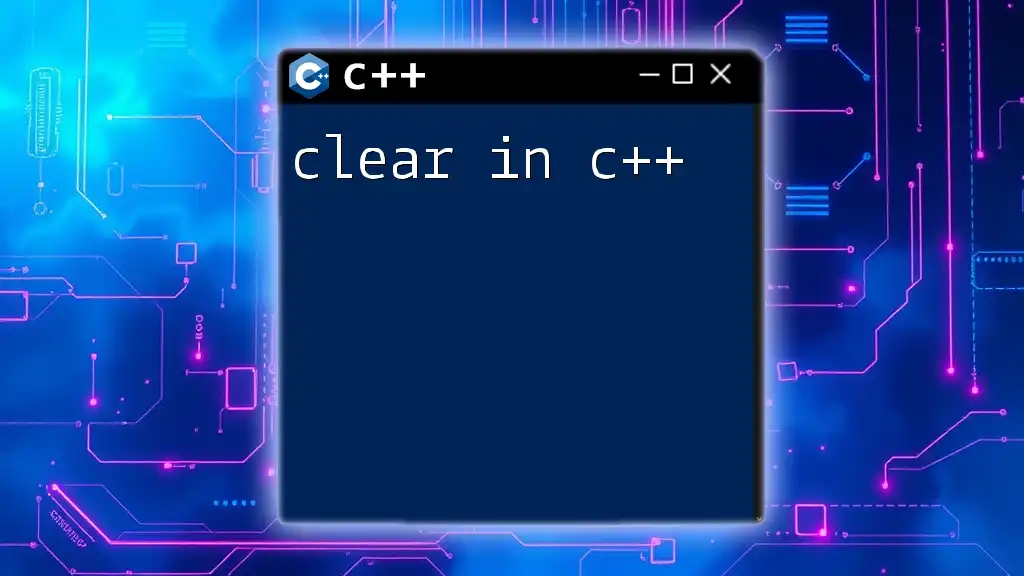
Conclusion
Future of Carbon C++
As Carbon C++ continues to evolve, the development community remains engaged, contributing to its growth and improvement. Keeping an eye on updates and participating in discussions can help you stay ahead of the curve.
Encouragement for New Users
If you are a newcomer to Carbon C++, there's no better time to dive in! With its modern syntax and efficient design, it promises to streamline your programming experience while allowing you to maintain the power and flexibility of C++. Embrace the challenges and opportunities that Carbon C++ presents, and witness the transformation in your coding journey.
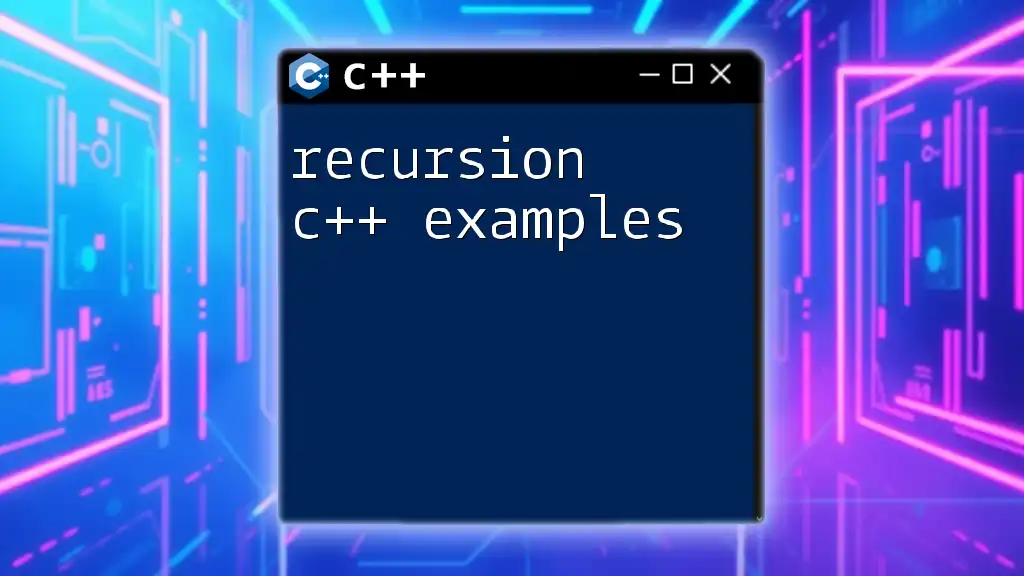
Additional Resources
- Official Documentation: A comprehensive guide to all features and syntax.
- Community Forums and Support: Engage with fellow developers and seek help.
- Books and Online Courses on Carbon C++: Structured learning paths will enhance your proficiency.
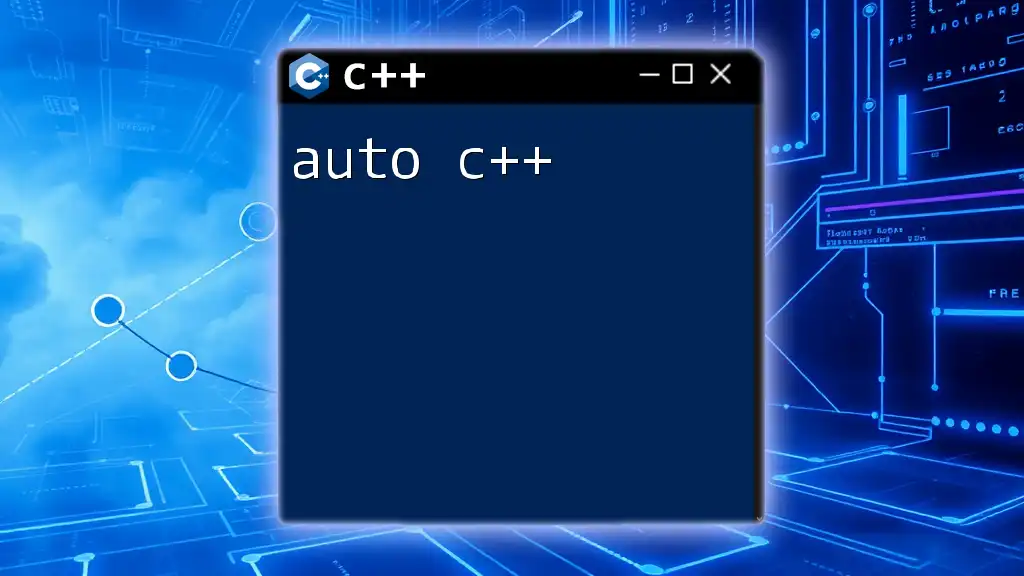
FAQs
What is the primary focus of Carbon C++?
The core focus of Carbon C++ is to modernize the programming experience while enhancing maintainability and performance, specifically addressing C++’s existing limitations.
Can I use Carbon C++ with my existing C++ projects?
Absolutely! Carbon C++ is designed to be interoperable with existing C++ codebases, allowing you to enhance your projects without needing a complete rewrite.
How does Carbon C++ differ from other programming languages?
Carbon C++ retains much of the performance and flexibility of C++ while introducing modern constructs that make development easier and more intuitive, addressing challenges that many developers face in traditional C++.