The `param` keyword in C++ typically refers to parameters in function definitions, which are used to pass information into functions.
Here's a simple example demonstrating how to define a function with parameters in C++:
#include <iostream>
using namespace std;
void greet(string name) {
cout << "Hello, " << name << "!" << endl;
}
int main() {
greet("Alice");
return 0;
}
Understanding Parameters in C++
What are Parameters?
In programming, parameters are the variables used in functions to receive values from the caller. They serve as placeholders for the values that will be passed when the function is executed, allowing the same function to work with different inputs. Parameters are crucial in C++ as they help create more flexible and reusable code.
For example, consider the following simple function that takes two integers as parameters and returns their sum:
int add(int a, int b) {
return a + b;
}
In this case, `a` and `b` are parameters that allow the caller to specify the integers to be added.
Types of Parameters
Positional Parameters
Positional parameters are the most common type of parameters. They are used in the order they are defined in the function. The values supplied to the function must match the types and locations of the parameters.
Here is an example of a function with positional parameters:
void greet(const std::string& name, int age) {
std::cout << "Hello, " << name << "! You are " << age << " years old." << std::endl;
}
In this function, `name` and `age` are positional parameters. If you call the function like this:
greet("Alice", 30);
It will output: `Hello, Alice! You are 30 years old.`
Default Parameters
Default parameters allow you to provide a default value for a parameter when the function is called without that argument. This enhances the function's usability by making certain arguments optional.
Consider the following function that uses a default parameter:
void greet(const std::string& name, int age = 18) {
std::cout << "Hello, " << name << "! You are " << age << " years old." << std::endl;
}
This allows you to call `greet` with just one argument:
greet("Bob");
The output will be: `Hello, Bob! You are 18 years old.` In this case, the `age` parameter defaults to 18 when not provided.
Reference Parameters
Reference parameters allow a function to modify the original argument passed to it, rather than working with a copy. This is useful for large data structures or when you need to modify the argument in the function.
Here’s an example using reference parameters:
void increment(int& value) {
value++;
}
When you call this function:
int num = 5;
increment(num);
std::cout << num; // Outputs: 6
The original `num` is modified because it was passed by reference.
Constant Reference Parameters
Constant reference parameters provide the advantages of reference parameters while protecting the arguments from modification. This way, large objects can be passed without the overhead of copying them, ensuring they remain unchanged.
Here’s an example:
void display(const std::string& message) {
std::cout << message << std::endl;
}
Calling this function:
std::string myMessage = "Hello, World!";
display(myMessage);
In this case, `message` is passed as a constant reference, preventing any alterations to `myMessage` within the function.
Function Overloading with Parameters
Function overloading in C++ allows you to define multiple functions with the same name but different parameter types or counts. This can enhance the usability of your functions without requiring different names for similar functionalities.
Consider this example:
void display(int number) {
std::cout << "Integer: " << number << std::endl;
}
void display(double number) {
std::cout << "Double: " << number << std::endl;
}
You can call these functions with different parameter types:
display(5); // Outputs: Integer: 5
display(5.5); // Outputs: Double: 5.5
Overloading functions based on parameters enhances code clarity and function reuse.
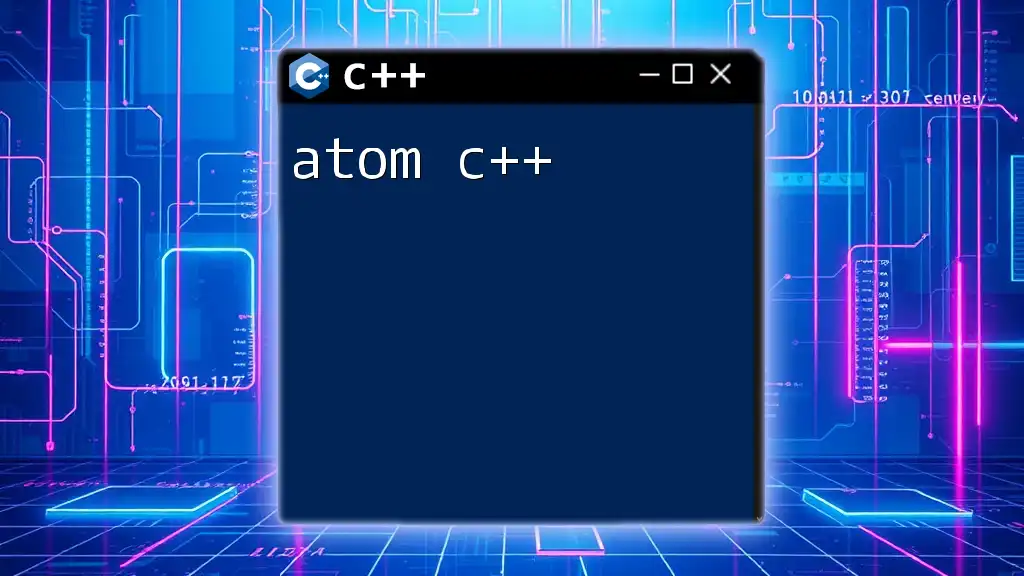
Best Practices for Using Parameters in C++
Naming Conventions
Using meaningful names for parameters enhances the readability of your code. Consistent and descriptive naming allows others (and your future self) to understand the purpose of each parameter without digging through documentation.
For example, instead of using generic names like `x` or `y`, use `radius` or `height`, as in:
float calculateArea(float radius) {
return 3.14159 * radius * radius;
}
Limiting the Number of Parameters
While it's possible to have functions with many parameters, it can make the code hard to maintain and understand. Rather than passing multiple parameters, consider using structures or classes to encapsulate related data.
For instance, instead of:
void createUser(std::string name, int age, std::string email, std::string address) {
// Implementation
}
You could define a `User` class:
class User {
public:
std::string name;
int age;
std::string email;
std::string address;
};
void createUser(const User& user) {
// Implementation
}
Documentation and Comments
Good documentation is key to maintainability. Commenting on parameter types and purposes in function headers makes your code more accessible. Use clear, concise comments to explain complex behaviors.
Example of clear documentation:
/**
* @brief Calculates the area of a circle
*
* @param radius The radius of the circle
* @return The area of the circle
*/
float calculateArea(float radius) {
return 3.14159 * radius * radius;
}
Parameter Validation Techniques
Validating parameters before processing them is crucial to ensuring the integrity of your functions. Check for valid ranges, types, and conditions.
For example:
void setAge(int age) {
if (age < 0) {
throw std::invalid_argument("Age cannot be negative");
}
// Set age
}
This ensures that the function behaves correctly, even with incorrect inputs.
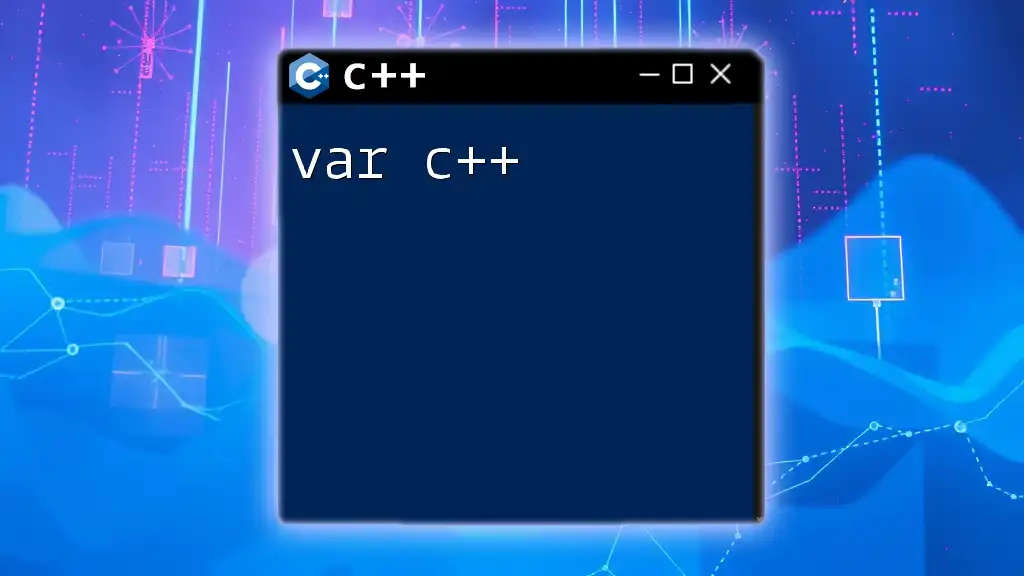
Advanced Topics
Variadic Templates
Variadic templates allow functions to accept an arbitrary number of arguments. This is particularly useful for creating flexible interfaces.
Here's a simple example:
template<typename... Args>
void log(Args... args) {
(std::cout << ... << args) << std::endl; // C++17 fold expression
}
You can call this function with any number of arguments:
log("Value: ", 5, " and count: ", 3.14); // Outputs: Value: 5 and count: 3.14
Lambda Expressions as Parameters
C++ allows the use of lambda expressions as parameters, enabling you to pass functions as arguments. This can make your code more flexible and concise.
Here’s an example:
void execute(const std::function<void()>& func) {
func(); // Call the passed function
}
execute([]() {
std::cout << "Hello from a lambda!" << std::endl;
});
This flexibility can enhance the design of your functions.
Template Parameters
Template parameters enable functions to operate on generic types. This can reduce code duplication and improve maintainability.
Here's an example of a function template:
template<typename T>
T findMax(T a, T b) {
return (a > b) ? a : b;
}
You can call this function with different types:
std::cout << findMax(10, 20); // Outputs: 20
std::cout << findMax(5.5, 3.3); // Outputs: 5.5
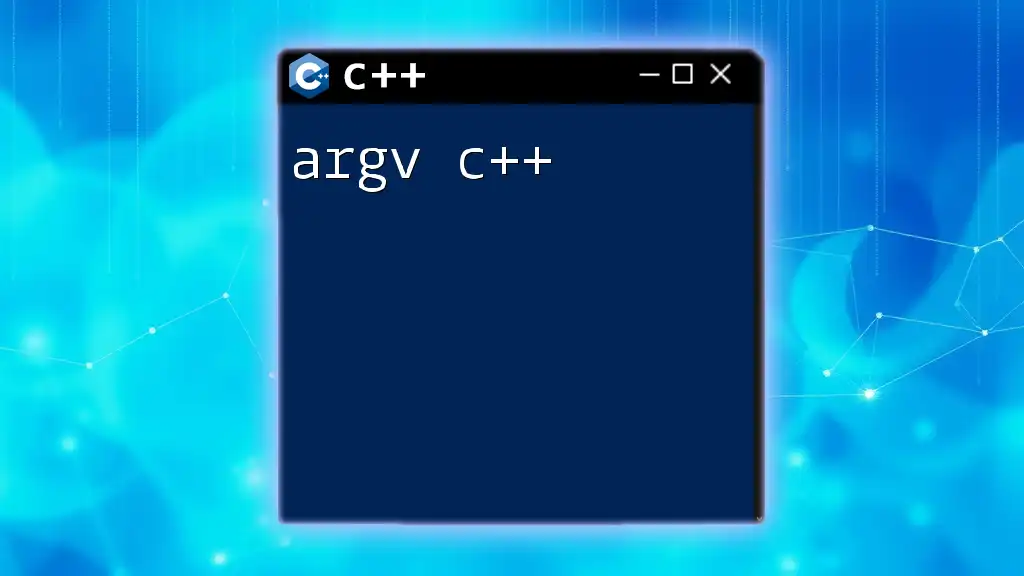
Conclusion
Understanding the use of parameters is essential for effective C++ programming. From basic positional parameters to advanced topics like variadic templates, mastering parameters allows you to write flexible, reusable, and maintainable code. Emphasizing best practices, such as clear naming conventions, parameter validation, and documentation, will further enhance the quality of your code. Experiment and practice with different types of parameters to deepen your understanding of this fundamental C++ concept.