"Super C++ refers to advanced C++ programming techniques that enhance coding efficiency and leverage the full power of the C++ language."
Here's a simple code snippet demonstrating a feature of C++ called classes and objects:
#include <iostream>
using namespace std;
class SuperClass {
public:
void displayMessage() {
cout << "Hello from SuperClass!" << endl;
}
};
int main() {
SuperClass obj;
obj.displayMessage();
return 0;
}
Understanding C++ Super Features
What Makes C++ a "Super" Language?
C++ stands out as a "super" language due to its robust architecture and flexibility, which allows developers to write high-performance applications. It provides the developer with control over system resources, which is crucial for performance-sensitive applications.
-
Robustness: C++ features strong type checking, enabling error detection at compile-time rather than runtime. This robustness is essential in preventing runtime errors and ensuring reliability in applications.
-
Performance: C++ is designed to achieve high performance through low-level memory manipulation. Programs written in C++ can run significantly faster than those written in higher-level languages, making it suitable for resource-constrained environments.
-
Flexibility: The language's support for multiple programming paradigms—procedural, object-oriented, and generic programming—allows developers to choose the most suitable approach for their application.
Advanced C++ Concepts
Object-Oriented Programming (OOP)
At the core of C++'s design is object-oriented programming (OOP), which promotes modularity and code reuse. Familiarizing yourself with the following principles is crucial for mastering super C++.
-
Encapsulation: This principle encapsulates data within classes, restricting access to internal object states through public interfaces. This prevents unauthorized access and misuse of data.
-
Inheritance: Inheritance allows classes to derive properties and behaviors from other classes, promoting code reuse and establishing a hierarchical relationship between classes.
-
Polymorphism: C++ supports polymorphism, which lets you invoke derived class methods through base class pointers, enabling flexible and dynamic code.
Example: A simple class hierarchy using animals:
class Animal {
public:
virtual void sound() {
std::cout << "Animal sound" << std::endl;
}
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Bark" << std::endl;
}
};
Standard Template Library (STL)
The Standard Template Library (STL) greatly enhances developer productivity by providing pre-built data structures and algorithms.
- Core Components: The STL consists of containers (such as vectors and maps), algorithms (like sort and search), and iterators that facilitate traversal of data structures.
Example: Using `std::vector` and `std::sort`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {4, 2, 5, 1, 3};
std::sort(nums.begin(), nums.end());
for (int num : nums) {
std::cout << num << " ";
}
return 0;
}
C++11/14/17/20 Features
The evolution of C++ through its various versions has introduced powerful features that enhance the language's capabilities.
- Lambda Functions: C++11 introduced lambda expressions, allowing for easier and more readable anonymous function definitions.
auto add = [](int a, int b) { return a + b; };
std::cout << add(3, 4); // Outputs 7
- Smart Pointers: Instead of manual memory management, modern C++ encourages the use of smart pointers, which automatically handle memory deallocation.
#include <memory>
void smartPointerExample() {
std::unique_ptr<int> ptr = std::make_unique<int>(5);
std::cout << *ptr; // Outputs 5
}
- std::optional and std::variant: These types help manage values that may not always be present, leading to clearer and safer code.
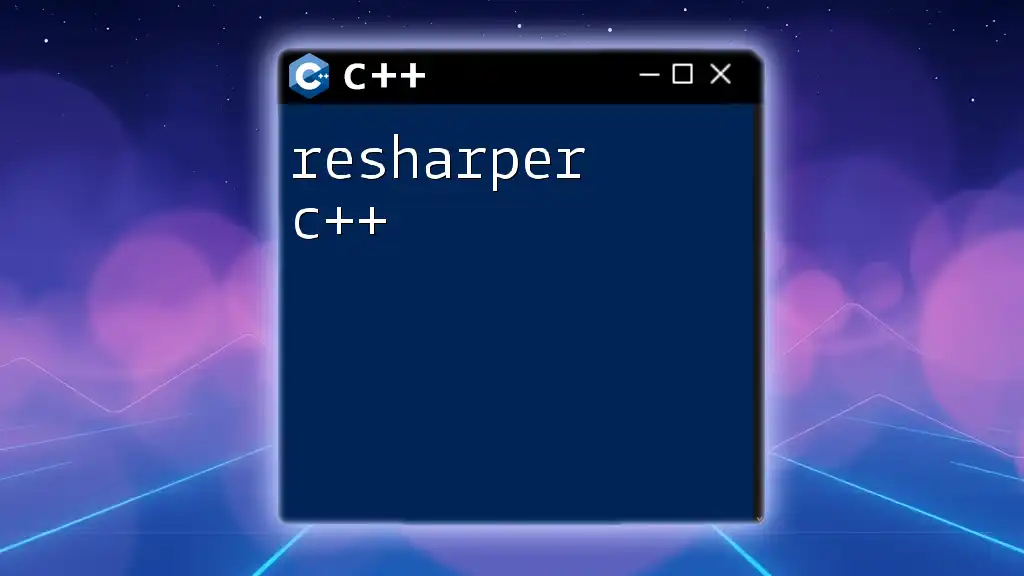
Building Blocks of Super C++
Memory Management
Memory management is a critical aspect of C++. Understanding the differences between dynamic and static memory helps prevent memory leaks and segmentation faults.
-
Dynamic vs. Static Memory: While static memory allocation occurs at compile-time and remains fixed, dynamic memory can be allocated and deallocated at runtime, offering flexibility at the cost of management complexity.
-
Manual Memory Management: With pointers, you manually allocate memory using `new` and deallocate it using `delete`.
int* array = new int[10]; // dynamic array
delete[] array; // release memory
- Automatic Memory Management: Smart pointers automate memory management, reducing the risk of leaks and dangling pointers. Incorporating these practices is essential for efficient C++ coding.
Error Handling Techniques
Exception Handling
C++ provides a structured approach to error handling through exceptions, distinguishing between normal and exceptional flow.
- Syntax: The `try`, `catch`, and `throw` keywords form the basis of exception handling. Within a `try` block, exceptions can be thrown and subsequently caught in the associated `catch` block.
try {
throw std::runtime_error("Something went wrong");
} catch (const std::runtime_error& e) {
std::cerr << e.what() << std::endl;
}
- Custom Exceptions: You can create custom exception classes to provide more context about errors.
Error Codes vs. Exceptions
While error codes are traditional methods of error handling, they often lead to code clutter and can be overlooked. Exceptions provide a clearer, more robust way to manage errors.
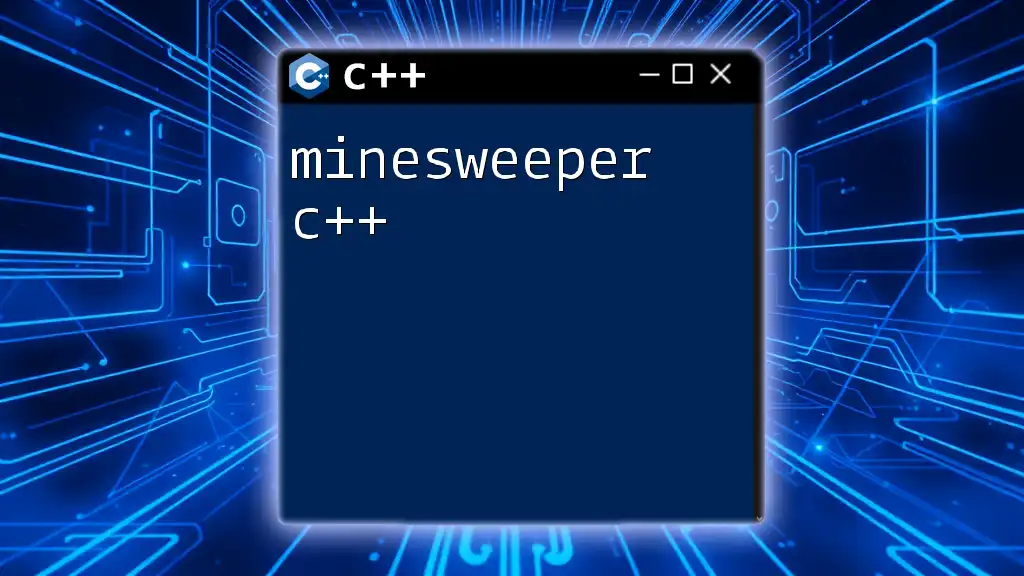
Advanced Techniques in Super C++
Multithreading in C++
C++ has made significant strides in multithreading, allowing developers to create concurrent applications effectively.
- std::thread: Introduced in C++11, it facilitates thread creation and management:
#include <thread>
void threadFunction() {
std::cout << "Running in a separate thread!" << std::endl;
}
int main() {
std::thread t(threadFunction);
t.join(); // Ensure the main thread waits for t to finish
}
- Synchronization: As multiple threads may access shared resources, synchronization mechanisms such as mutexes prevent data races.
#include <mutex>
std::mutex mtx;
void safeFunction() {
std::lock_guard<std::mutex> lock(mtx); // Scoped mutex lock
// Critical section
}
Template Metaprogramming
Template metaprogramming elevates the capabilities of C++ by allowing code generation and manipulation at compile time.
- Type Traits and SFINAE: These techniques enable the implementation of logic based on types, which can be powerful when creating generic libraries.
Example: A compile-time factorial calculator using templates:
template<int N>
struct Factorial {
static const int value = N * Factorial<N - 1>::value;
};
template<>
struct Factorial<0> {
static const int value = 1;
};
// Usage
std::cout << Factorial<5>::value; // Outputs 120
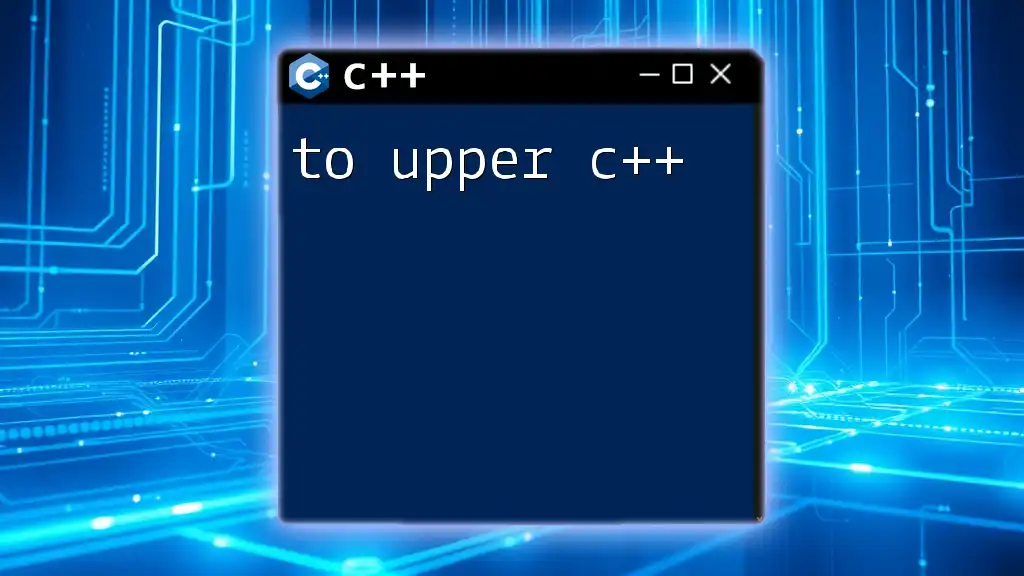
Real-World Applications of Super C++
Case Studies
Game Development
C++ is a staple in game development due to its ability to manage hardware resources effectively and deliver high-performance execution. Powerful game engines utilize advanced C++ features for rendering graphics, controlling physics, and managing memory efficiently.
Systems Programming
Operating systems and embedded systems often leverage C++ for its performance and control capabilities. Its low-level access to memory and system resources makes it an excellent fit for writing drivers and application frameworks.
Open-Source Projects
Exploring notable open-source projects such as Chromium, Unreal Engine, or Blender can provide insights into how super C++ techniques are applied on a large scale. These projects exemplify the language's performance and flexibility in real-world applications.
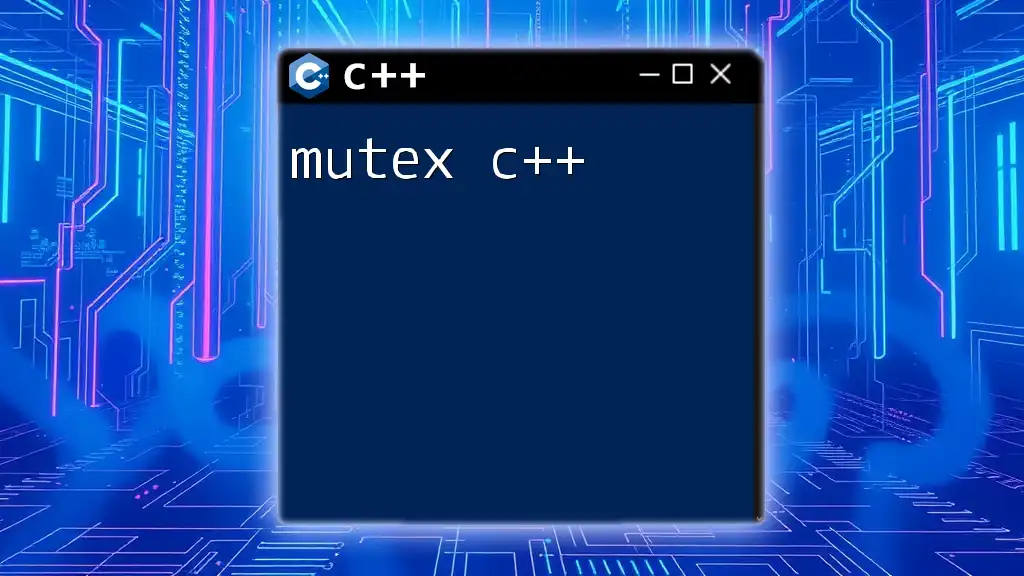
Conclusion
Super C++ encompasses an array of advanced concepts, techniques, and features that empower developers to craft efficient and reliable applications. By mastering these components, you will significantly enhance your capabilities and performance as a programmer. Continuous practice, exploration of real-world applications, and engagement with the programming community will further strengthen your skills and understanding of this powerful language.
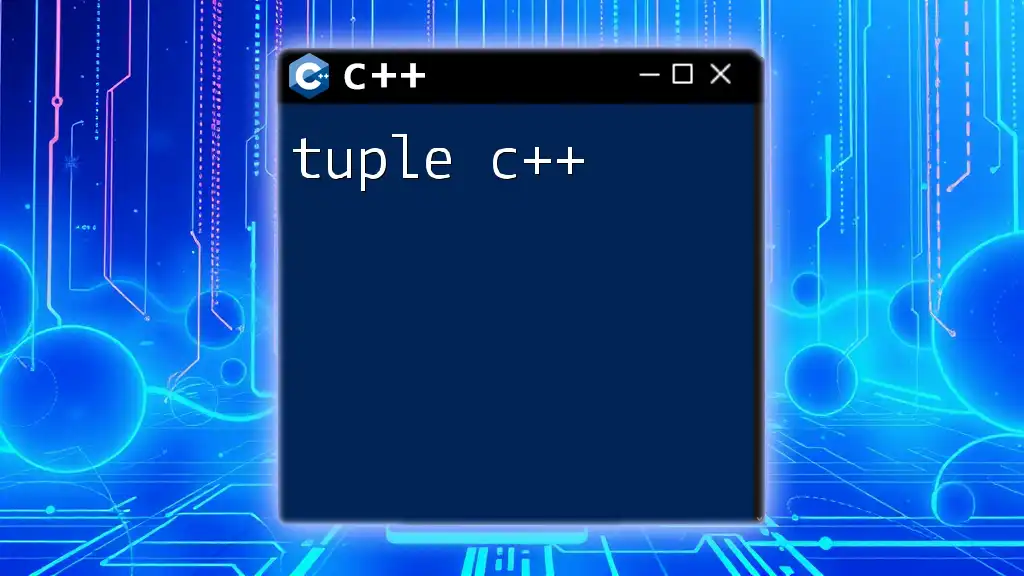
Additional Resources
Recommended Books and Courses
- "Effective Modern C++" by Scott Meyers
- "C++ Primer" by Stanley Lippman
- Online platforms like Coursera, Udemy, or freeCodeCamp offer excellent C++ programming courses.
Online Communities and Forums
Join forums and communities like Stack Overflow, Reddit’s r/Cplusplus, or the C++ section on GitHub to engage with fellow C++ enthusiasts.
Practice Projects
Consider developing projects such as a simple game engine, a file parser, or a personal finance management tool to apply the concepts learned throughout this guide.
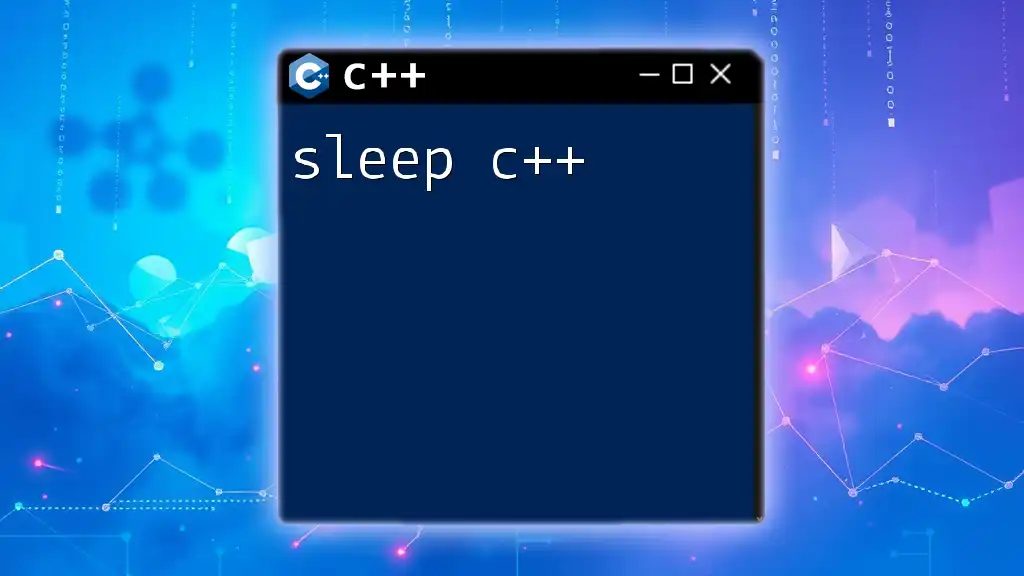
FAQs
- What is the best way to learn C++? Engaging in hands-on projects and leveraging online courses or books is highly effective.
- How do I manage memory in C++? By opting for smart pointers and understanding manual allocation techniques, you can efficiently manage memory.
- What are the advantages of multithreading in C++? Multithreading allows your programs to utilize CPU resources more effectively, leading to faster processing and improved performance.