The `popen` function in C++ allows you to execute a command in a new process and read from or write to its standard input or output, enabling inter-process communication.
#include <cstdio>
#include <iostream>
int main() {
FILE *pipe = popen("ls -l", "r");
if (!pipe) {
std::cerr << "Failed to open pipe!" << std::endl;
return 1;
}
char buffer[128];
while (fgets(buffer, sizeof(buffer), pipe) != nullptr) {
std::cout << buffer;
}
pclose(pipe);
return 0;
}
Understanding the Basics of popen
What Does popen Do?
The `popen` function in C++ is an essential utility that allows developers to execute shell commands and interact with their outputs directly within a C++ program. This powerful function creates a pipe between the calling program and a process specified by the command string, enabling you to either read from or write to the command's input/output streams. The primary advantage of using `popen` is the convenience it provides for executing and handling subprocesses without needing to manage multiple threads or complex inter-process communication.
Syntax of popen
The general syntax for using `popen` is as follows:
FILE *popen(const char *command, const char *mode);
-
Parameters:
- `command`: This is a string representing the shell command you wish to execute.
- `mode`: This specifies the direction of the pipe. It can be either:
- `"r"`: To read output from the command.
- `"w"`: To write input to the command.
-
Return Value: The function returns a pointer to a `FILE` stream that you can use to read from or write to the command. If `popen` fails, it returns a `nullptr`.
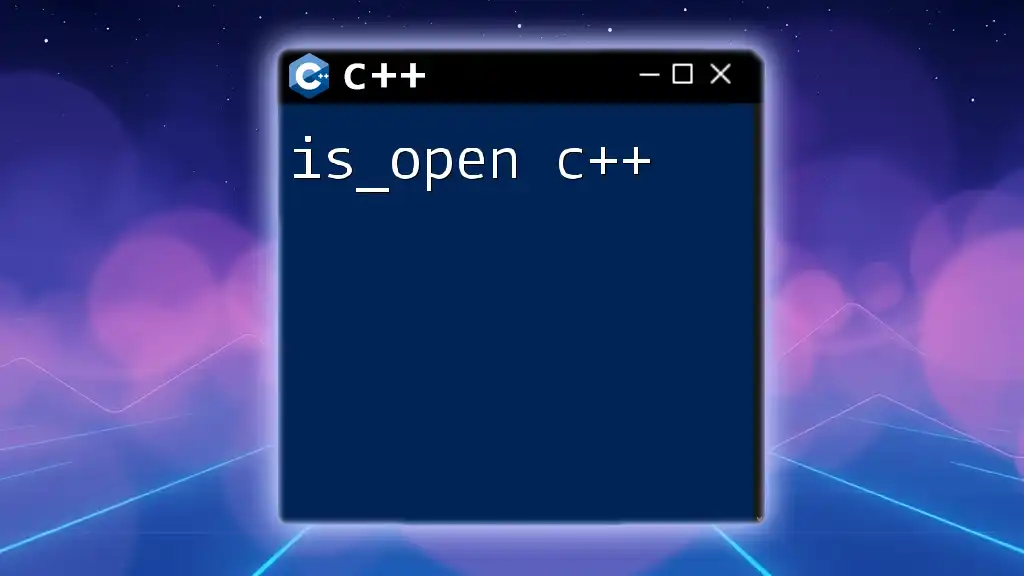
Modes of Operation
Reading from a Command
Using `popen` to read the output from a shell command is straightforward. Here's an example that demonstrates how to list the contents of the current directory:
#include <iostream>
#include <cstdio>
int main() {
FILE *fp = popen("ls", "r");
if (fp == nullptr) {
std::cerr << "Failed to run command\n";
return 1;
}
// Read output
char buffer[128];
while (fgets(buffer, sizeof(buffer), fp) != nullptr) {
std::cout << buffer;
}
pclose(fp);
return 0;
}
In this example, `popen` runs the `ls` command to list directory contents. The `fgets` function reads the command's output line by line and prints it to the console. Ensure you close the pipe with `pclose(fp)` to free resources.
Writing to a Command
Conversely, you can use `popen` to send data to a shell command. For instance, if you want to sort a list of fruits, you can write them to the `sort` command like this:
#include <iostream>
#include <cstdio>
int main() {
FILE *fp = popen("sort", "w");
if (fp == nullptr) {
std::cerr << "Failed to run command\n";
return 1;
}
// Write data to the command
fprintf(fp, "banana\napple\norange\n");
pclose(fp);
return 0;
}
In the above code, you're writing three fruit names to the standard input of the `sort` command. The command will then process them accordingly. It's a simple yet effective way of piping data through commands quickly.
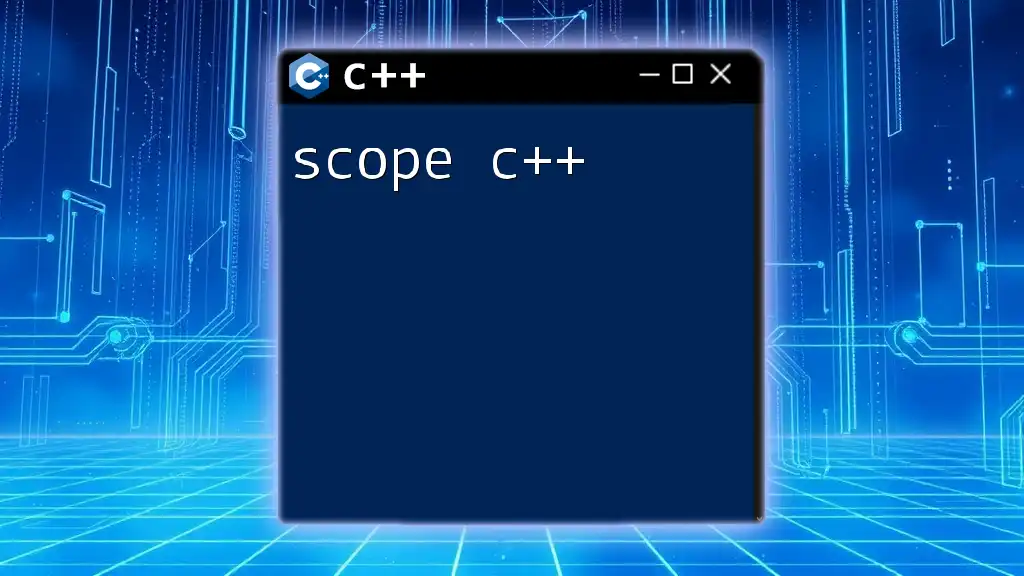
Error Handling with popen
Checking for Errors
Error handling is a crucial aspect of programming, especially when working with system calls like `popen`. Always check if the return value of `popen` is `nullptr`. If so, it indicates the command could not be executed. You should handle these situations gracefully, often by logging an error message or performing alternative actions.
When reading from or writing to the supplied stream, it's also important to check for errors during I/O operations, particularly when dealing with unexpected input or output that might affect the overall execution of your program.
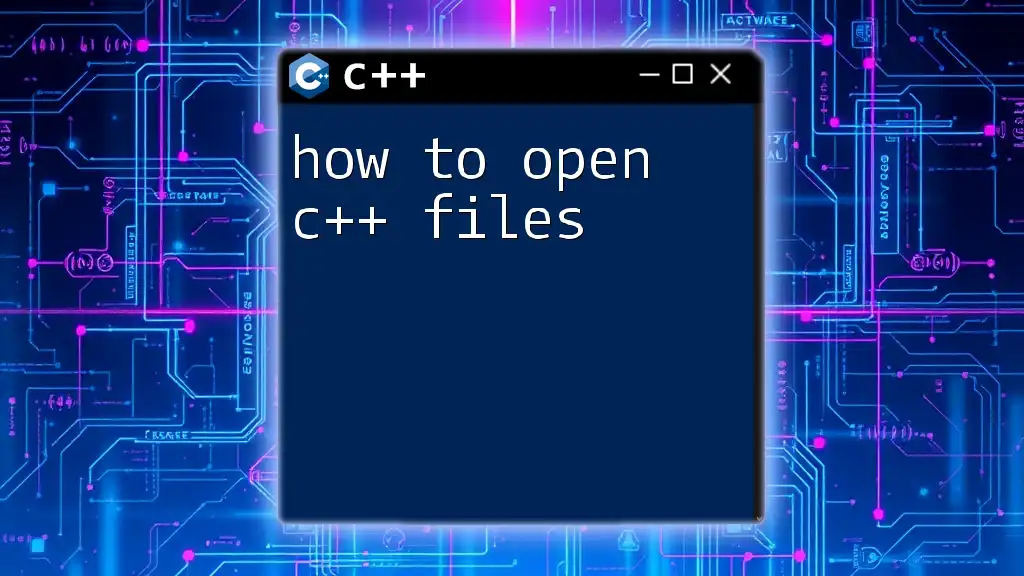
Limitations of popen
Security Risks
While `popen` is a powerful tool, it comes with security concerns. Passing user inputs directly into the command string can expose your application to command injection attacks, where an attacker can execute arbitrary commands on your system.
To prevent these security risks, always sanitize inputs, restrict command execution, and avoid constructing command strings directly from user inputs.
Performance Considerations
Performance can also be a limiting factor when using `popen`. Because it creates an entire process to run the command, `popen` can be less efficient than other methods such as using `fork` and `exec` to handle subprocesses directly. If your application frequently needs to spawn new processes, consider alternatives for better performance.
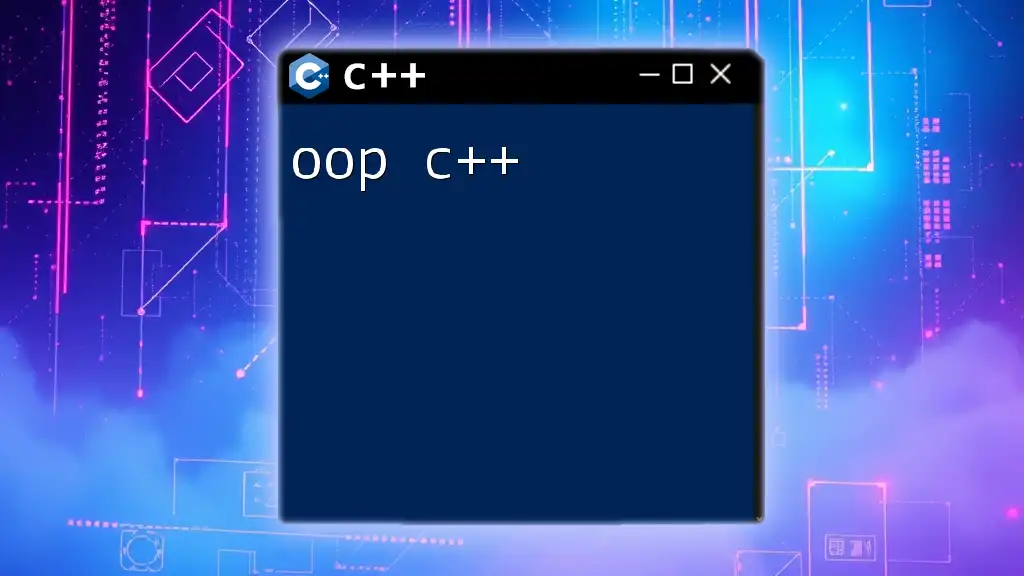
Practical Applications of popen in C++
System Administration Tasks
One of the primary use cases for `popen` is in crafting automation scripts for system administration tasks. For example, you can use `popen` to monitor system resources such as CPU usage through commands like `top` or `vmstat`. This ability makes it easy to gather and log system metrics programmatically.
Data Processing
`popen` is also a powerful tool in data processing. You can leverage it to create pipelines between standard Unix commands. For instance, you might want to use `popen` to retrieve the output of a command, process it, and then pass it to another command for further analysis.
Example of Piping Data Between Commands
Here’s an example where we use `popen` to find and count the number of `.cpp` files in a directory:
#include <iostream>
#include <cstdio>
int main() {
FILE *fp = popen("ls *.cpp | wc -l", "r");
if (fp == nullptr) {
std::cerr << "Failed to run command\n";
return 1;
}
char buffer[128];
fgets(buffer, sizeof(buffer), fp);
std::cout << "Number of .cpp files: " << buffer;
pclose(fp);
return 0;
}
In this example, the command counts the number of `.cpp` files in the current directory using a pipe. The output is read and printed, demonstrating how to combine multiple commands efficiently.
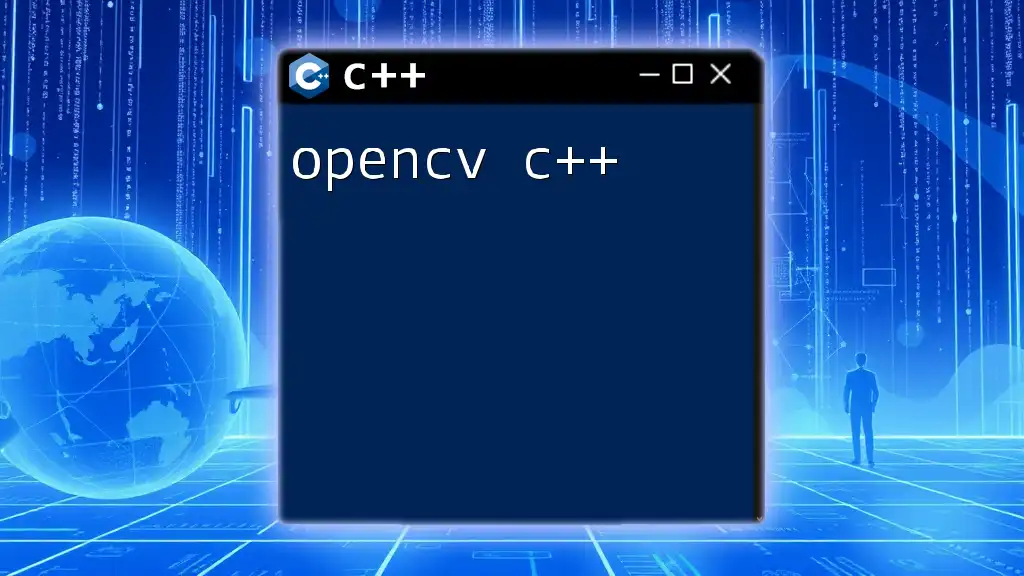
Conclusion
In this article, we explored the `popen` function in C++, understanding its purpose, applications, and limitations. This powerful command execution function allows for seamless interaction with shell commands, enabling developers to enhance their applications with external command capabilities. However, it's crucial to manage security and performance issues diligently to make the most out of its advantages. By practicing with various examples, you'll gain greater confidence in using `popen` effectively in your C++ projects.