POCO C++ is a collection of C++ class libraries that simplify network programming, threading, and other system tasks, making it easier to build portable and high-performance applications.
Here’s a simple code snippet demonstrating a basic HTTP server using POCO C++:
#include <Poco/Net/HTTPServer.h>
#include <Poco/Net/HTTPServerRequest.h>
#include <Poco/Net/HTTPServerResponse.h>
#include <Poco/Net/HTTPRequestHandler.h>
#include <Poco/Net/HTTPRequestHandlerFactory.h>
#include <Poco/Thread.h>
#include <Poco/Util/ServerApplication.h>
using namespace Poco::Net;
using namespace Poco::Util;
class HelloWorldRequestHandler : public HTTPRequestHandler {
public:
void handleRequest(HTTPServerRequest& request, HTTPServerResponse& response) {
response.setStatus(HTTPResponse::HTTP_OK);
response.setContentType("text/plain");
std::ostream& ostr = response.send();
ostr << "Hello World!";
}
};
class HelloWorldRequestHandlerFactory : public HTTPRequestHandlerFactory {
public:
HTTPRequestHandler* createRequestHandler(const HTTPServerRequest& request) {
return new HelloWorldRequestHandler;
}
};
class HelloWorldServerApp : public ServerApplication {
protected:
int main(const std::vector<std::string>&) {
HTTPServer server(new HelloWorldRequestHandlerFactory, ServerSocket(8080), new HTTPServerParams);
server.start();
std::cout << "Server started on port 8080." << std::endl;
waitForTerminationRequest();
server.stop();
return 0;
}
};
int main(int argc, char** argv) {
HelloWorldServerApp app;
return app.run(argc, argv);
}
What is Poco C++?
Poco C++ is an open-source, cross-platform C++ framework designed to build networked and internet-based applications efficiently. It provides a rich set of libraries that enable developers to tackle various tasks, including networking, data access, XML parsing, and multithreading, all while maintaining a high level of performance.
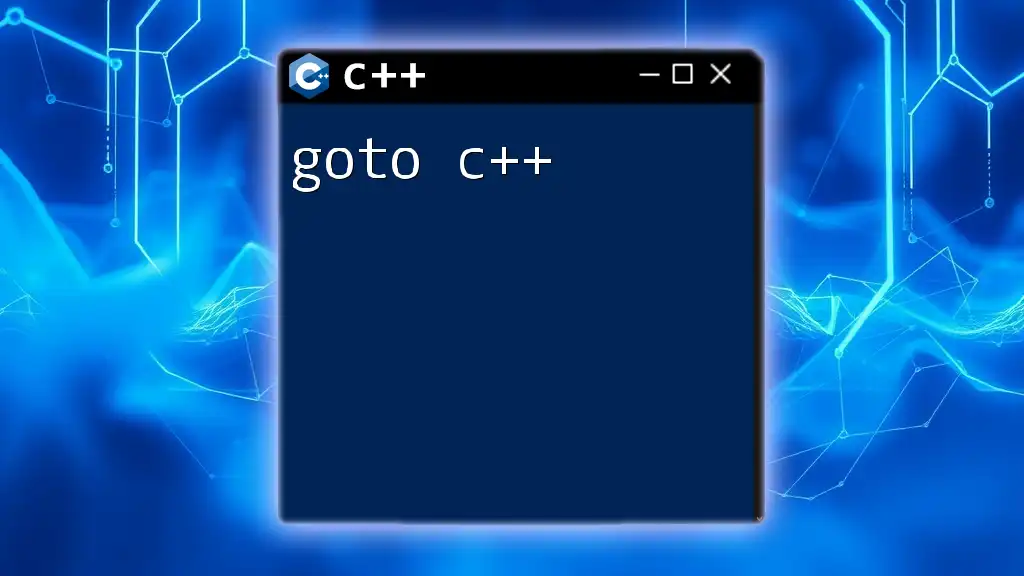
Why Use Poco C++?
Using Poco C++ offers numerous advantages, including:
- Modularity: Poco is highly modular, allowing developers to include only the necessary libraries for their projects, which minimizes bloat and enhances performance.
- Flexibility: It is suitable for a wide range of applications, from simple utilities to complex enterprise-level solutions.
- Documentation and Community Support: There is extensive documentation available, along with a vibrant community that contributes to its ongoing development.
In comparison to other C++ frameworks, Poco stands out for its comprehensive set of tools and libraries specifically designed for network programming, which is crucial in today’s connected world.
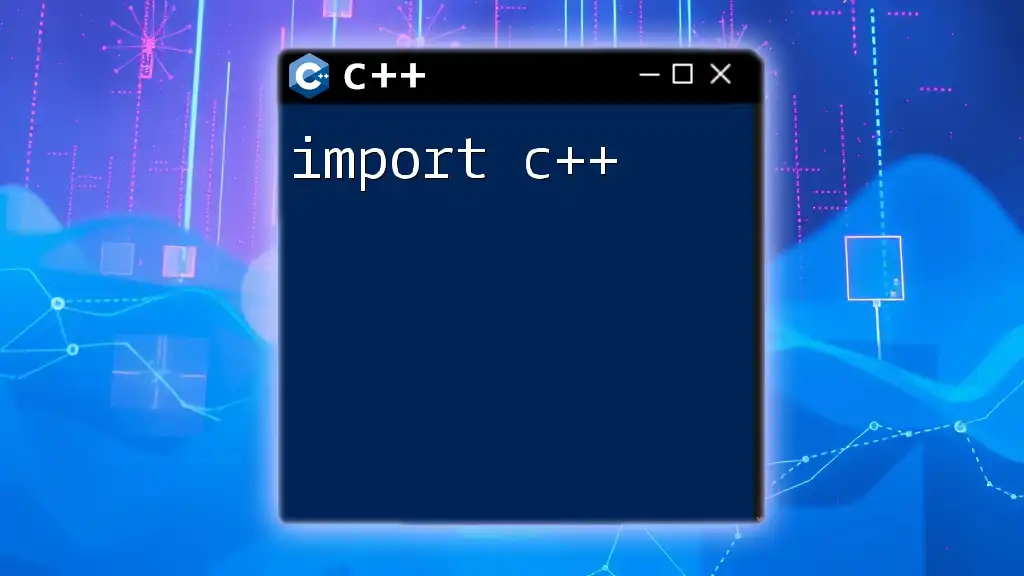
Setting Up Poco C++
System Requirements
Before installing Poco C++, ensure that your system meets the following prerequisites:
- An up-to-date C++ compiler (GCC, Clang, or MSVC)
- CMake (for building projects)
- Basic knowledge of using command-line interfaces
Installation
Installation on Windows
- Download the latest Poco C++ release from the [official repository](https://pocoproject.org).
- Unzip the package and navigate to the directory via the command line.
- Use CMake to configure and generate the build system:
cmake . cmake --build . --config Release
Installation on Linux
- Install build essentials:
sudo apt-get install build-essential
- Follow the same steps as in the Windows installation to download and build Poco.
Installation on macOS
- Use Homebrew for easy installation:
brew install poco
Verifying Installation
To verify that Poco C++ is correctly installed, you can compile a simple Hello World application using Poco libraries. If it compiles without issues, the installation was successful.
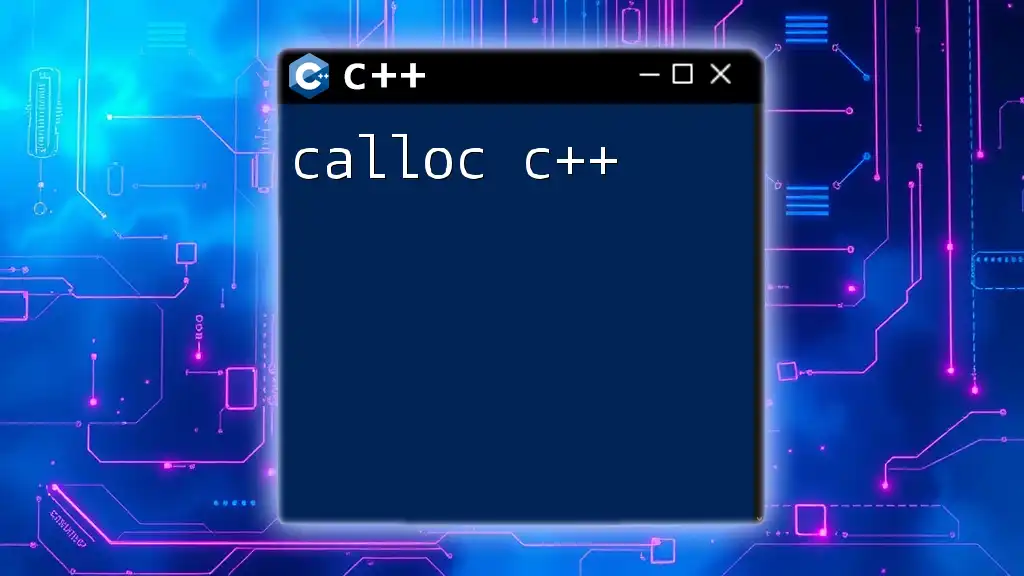
Core Features of Poco C++
Modular Architecture
Poco’s architecture is highly modular, allowing developers to include only the libraries they need for their applications. Some of the most used modules include:
- Foundation: Basic utility classes for file handling, date/time management, etc.
- Net: Features for networking, including HTTP, SMTP, FTP, etc.
- XML: Classes for parsing and generating XML documents.
Foundation Library
The Foundation Library serves as the backbone of Poco C++, providing essential features for application development.
Overview of the Foundation Library
The Foundation library encapsulates basic functionalities like data types, collections, file systems, and more. The focus on performance and simplicity makes it an ideal starting point for any Poco C++ application.
Basic Examples with Foundation
-
Creating Strings:
#include <Poco/String.h> Poco::String str("Hello, Poco!"); std::cout << str << std::endl;
-
File Operations:
#include <Poco/File.h> Poco::File file("example.txt"); file.createFile();
-
Date and Time Handling:
#include <Poco/DateTime.h> Poco::DateTime now; std::cout << now.timestamp().epochTime() << std::endl;
Networking with Poco
Overview of Network Programming with Poco
Networking is a core feature of Poco C++, making it easy to create connected applications. The Net module provides classes for web protocols, making it straightforward to interact with online services.
Creating a Simple HTTP Client
A basic HTTP client can be established using `Poco::Net::HTTPClientSession`. Here’s a sample implementation:
#include <Poco/Net/HTTPClientSession.h>
#include <Poco/Net/HTTPRequest.h>
#include <Poco/Net/HTTPResponse.h>
#include <iostream>
int main() {
Poco::Net::HTTPClientSession session("www.example.com");
Poco::Net::HTTPRequest req(Poco::Net::HTTPRequest::HTTP_GET, "/");
session.sendRequest(req);
Poco::Net::HTTPResponse res;
session.receiveResponse(res);
std::cout << "Response Status: " << res.getStatus() << std::endl;
return 0;
}
Building a Web Server
Poco also allows the quick building of web servers. The following example demonstrates a simple HTTP server setup:
#include <Poco/Net/HTTPServer.h>
#include <Poco/Net/HTTPRequestHandler.h>
#include <Poco/Net/HTTPRequestHandlerFactory.h>
#include <Poco/Net/HTTPServerParams.h>
#include <Poco/Util/ServerApplication.h>
class RequestHandler : public Poco::Net::HTTPRequestHandler {
public:
void handleRequest(Poco::Net::HTTPServerRequest& request, Poco::Net::HTTPServerResponse& response) {
response.setStatus(Poco::Net::HTTPResponse::HTTP_OK);
response.setContentType("text/html");
std::ostream& out = response.send();
out << "<h1>Hello, World!</h1>";
}
};
class RequestHandlerFactory : public Poco::Net::HTTPRequestHandlerFactory {
public:
Poco::Net::HTTPRequestHandler* createRequestHandler(const Poco::Net::HTTPServerRequest& request) {
return new RequestHandler;
}
};
class WebServerApp : public Poco::Util::ServerApplication {
protected:
int main(const std::vector<std::string>& args) {
Poco::Net::HTTPServer server(new RequestHandlerFactory(), Poco::Net::ServerSocket(8080), new Poco::Net::HTTPServerParams);
server.start();
waitForTerminationRequest(); // Wait for CTRL-C or kill
server.stop();
return 0;
}
};
POCO_APP_MAIN(WebServerApp)
XML Parsing with Poco
Poco XML Library Overview
Handling XML is critical in many applications, especially in terms of configuration files and data interchange. Poco provides robust support for parsing and creating XML documents.
Parsing XML Documents
To parse an XML document, you can use the following approach:
#include <Poco/XML/DOMParser.h>
#include <Poco/XML/Document.h>
#include <Poco/XML/Node.h>
#include <iostream>
int main() {
Poco::XML::DOMParser parser;
Poco::XML::Document* pDoc = parser.parse("sample.xml");
Poco::XML::NodeList* pList = pDoc->getElementsByTagName("exampleTag");
for (size_t i = 0; i < pList->length(); ++i) {
std::cout << pList->item(i)->nodeName() << std::endl;
}
return 0;
}
Generating XML Documents
Creating XML documents from scratch can be accomplished as shown here:
#include <Poco/XML/XMLWriter.h>
#include <Poco/DOM/Document.h>
#include <Poco/DOM/Element.h>
#include <Poco/DOM/DOMFormatter.h>
#include <iostream>
int main() {
Poco::XML::Document doc;
Poco::XML::Element* pRoot = doc.createElement("root");
Poco::XML::Element* pChild = doc.createElement("child");
pChild->setTextContent("This is a child element.");
pRoot->appendChild(pChild);
Poco::XML::DOMFormatter formatter(std::cout);
doc.write(formatter);
return 0;
}
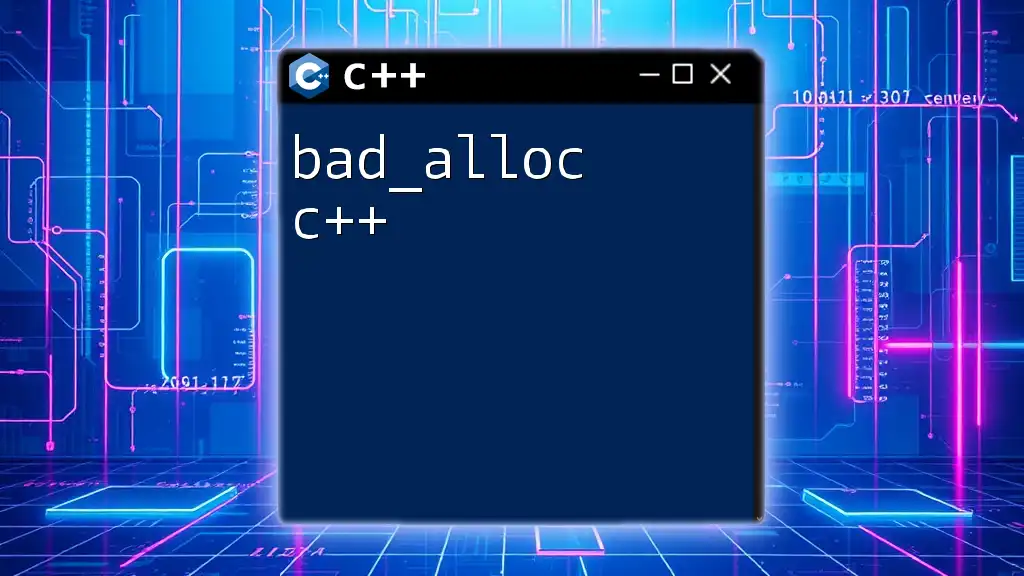
Advanced Features of Poco C++
Data Access and Persistence
Poco C++ excels in database connectivity through its Data module, which simplifies interactions with various databases by providing an ORM (Object-Relational Mapping) layer.
Using Poco with SQLite
Here’s an example of connecting to an SQLite database and executing commands:
#include <Poco/Data/Session.h>
#include <Poco/Data/SQLite/Connector.h>
int main() {
Poco::Data::SQLite::Connector::registerConnector(); // Register SQLite connector
Poco::Data::Session session("SQLite:sample.db");
session << "CREATE TABLE IF NOT EXISTS person (id INTEGER PRIMARY KEY, name TEXT)", Poco::Data::Keywords::now;
session << "INSERT INTO person (name) VALUES ('John Doe')", Poco::Data::Keywords::now;
return 0;
}
Exception Handling
Poco C++ also features a robust exception handling mechanism.
Overview of Poco's Exception Handling Mechanism
Poco uses a tailored exception hierarchy that helps developers manage errors gracefully. This makes debugging and error tracking more straightforward.
Creating Custom Exceptions
To create a custom exception, you can extend the `Poco::Exception` class. Here's how:
#include <Poco/Exception.h>
class MyCustomException : public Poco::Exception {
public:
MyCustomException(const std::string& msg) : Poco::Exception(msg) {}
};
int main() {
try {
throw MyCustomException("This is a custom exception.");
} catch (const MyCustomException& e) {
std::cerr << "Caught exception: " << e.displayText() << std::endl;
}
return 0;
}
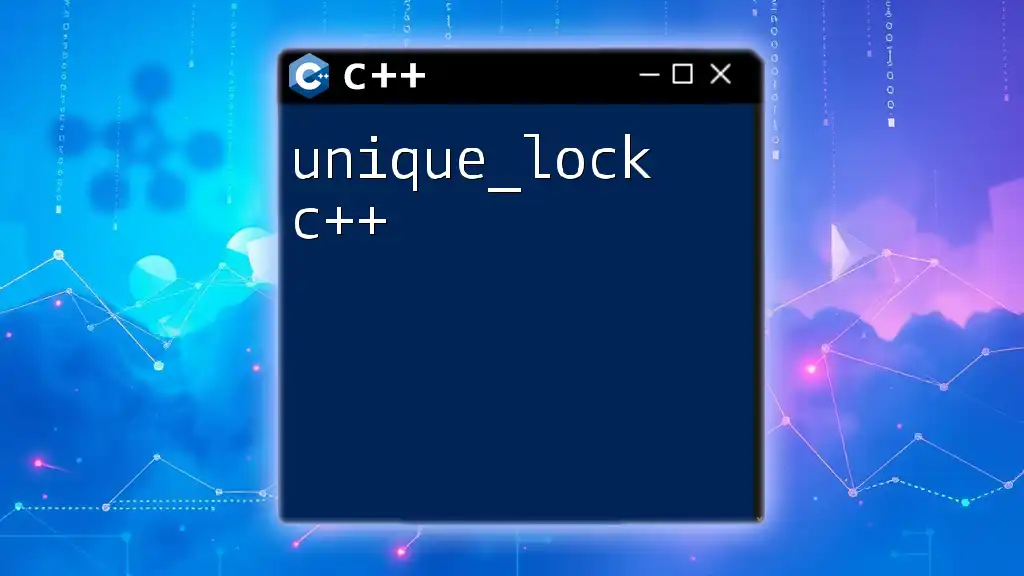
Best Practices with Poco C++
Effective Memory Management
While Poco handles memory allocation and deallocation effectively, developers should remain cautious with resource management, particularly with objects that handle files or network connections.
Concurrency and Thread Management
Poco provides extensive support for threading, allowing developers to create, manage, and synchronize threads seamlessly.
Overview of Available Threading Tools
Modules like `Poco::Thread` and `Poco::Mutex` provide powerful primitives for managing concurrency in applications.
Code snippet: Managing threads with `Poco::Thread`
#include <Poco/Thread.h>
#include <iostream>
class MyRunnable : public Poco::Runnable {
public:
void run() override {
std::cout << "Running in a separate thread." << std::endl;
}
};
int main() {
Poco::Thread thread;
MyRunnable runnable;
thread.start(runnable);
thread.join();
return 0;
}
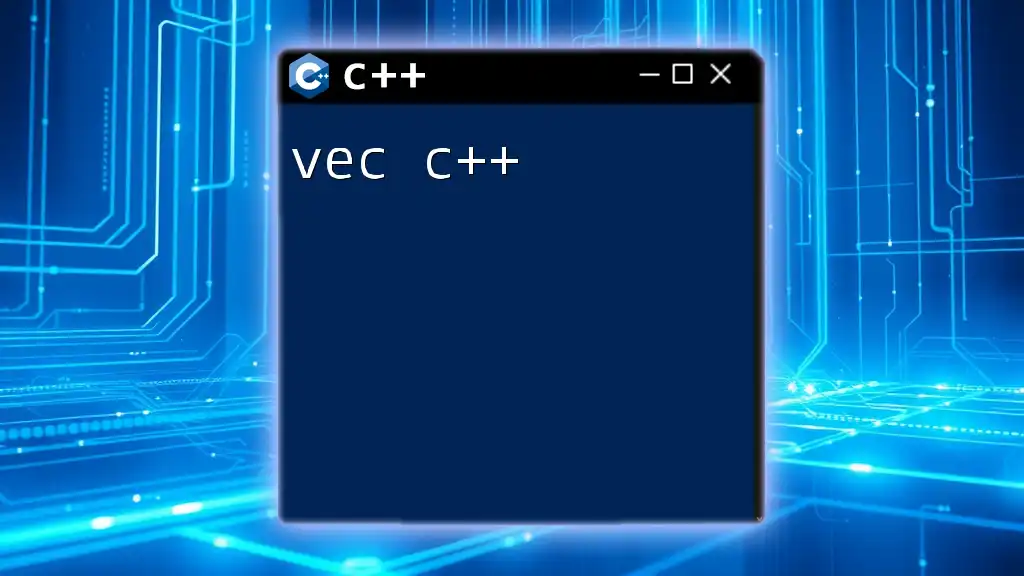
Real-World Applications of Poco C++
Case Studies
Poco C++ has been instrumental in building a variety of applications across industries. For instance, financial institutions utilize it for building secure data transaction systems, while IoT developers use it for robust device communication in smart applications.
Common Use Cases
Poco C++ is often deployed in scenarios that involve:
- Web servers for dynamic content generation.
- Networked applications to facilitate communication between distributed systems.
- Pro-caching systems that optimize data access through smart caching mechanisms.
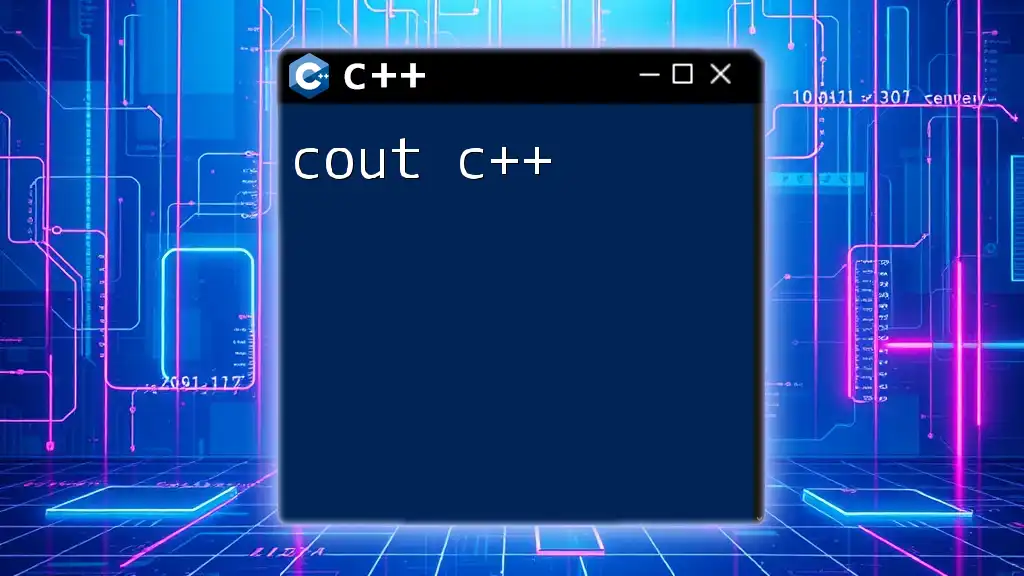
Conclusion
Poco C++ exemplifies a modern C++ framework that seamlessly integrates numerous functionalities essential for networked applications. With its expanding capabilities and community support, it is well-positioned to adapt to new technological advances.
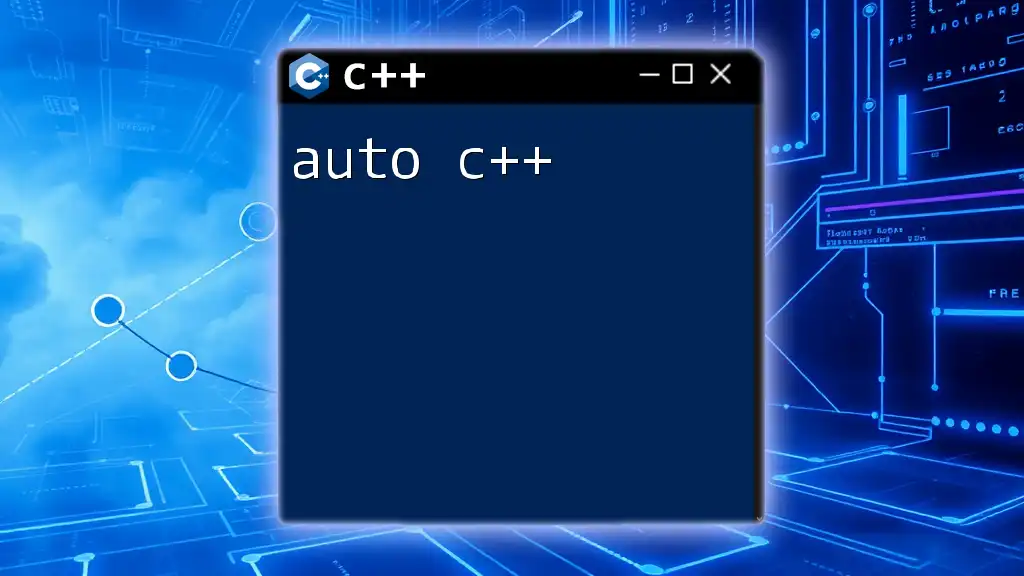
Additional Resources
For an in-depth exploration of Poco C++, consider the following resources:
- Official Documentation and Tutorials: The best source for comprehensive guidelines and best practices.
- Community Forums: Engage with other developers to share insights and troubleshooting advice.
- Books and Online Courses: Further your skills with structured learning paths tailored to Poco C++.
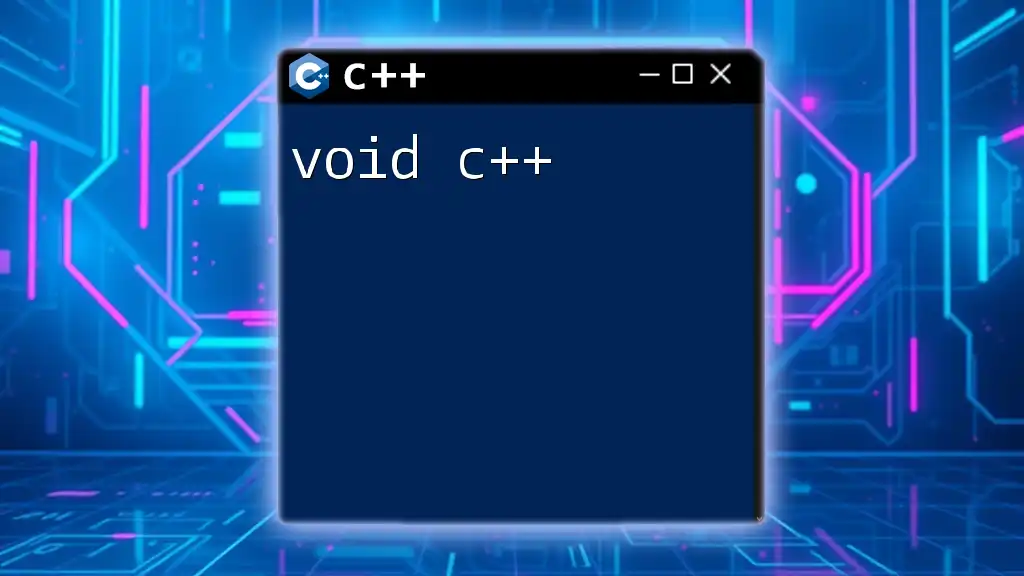
Call to Action
We encourage you to explore and practice using Poco C++. With its robust set of features, you can unlock new possibilities in your C++ development journey. If you have any questions or want to learn more, feel free to reach out to us!