In C++, you don't use an "import" statement like in some other programming languages; instead, you utilize the `#include` directive to include the necessary libraries or headers.
Here's how you would typically include the standard input-output library:
#include <iostream>
This directive allows you to use functionalities related to input and output streams in your C++ program.
Understanding the Basics of Importing in C++
What Does Importing Mean in C++?
In C++, importing refers to the act of including external libraries and modules into your code. This allows you to leverage existing code functionalities rather than reinventing the wheel. Unlike languages like Python or Java, which use an `import` statement, C++ utilizes the `#include` directive to bring in definitions, declarations, and other files that are essential for your program to function.
Why Importing is Essential
The practice of importing brings several significant advantages:
- Code Reusability: By importing libraries, you utilize existing, tested code, which saves you time and effort.
- Modular Design: Importing helps in maintaining a clean separation between code components, making it easier to manage larger projects.
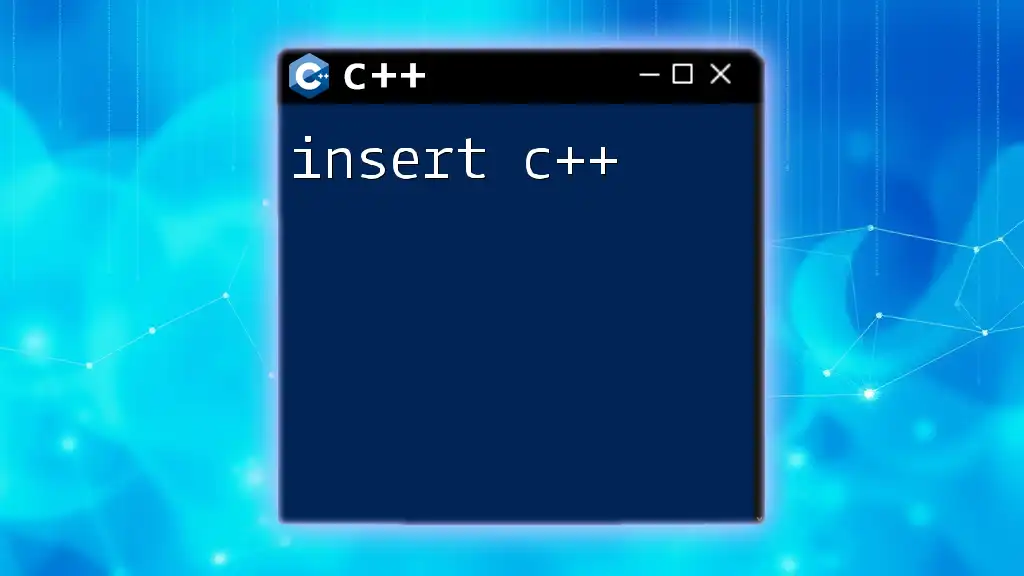
Basic Syntax for Importing in C++
The `#include` Directive
In C++, the `#include` directive is the primary means of importing files. There are two key syntactical forms you must know:
- Angle Brackets (`<>`): Used for including standard libraries.
- Double Quotes (`""`): Used for including your custom or user-defined libraries.
For example:
#include <iostream> // Standard library
#include "myHeader.h" // User-defined header
Practical Example: Including the iostream Library
The `iostream` library contains essential functionality for input and output operations in C++. To utilize its features, such as `std::cout` and `std::cin`, include it at the top of your code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In the above example, `std::cout` allows you to print messages to the console. This demonstrates the basic usage of importing libraries to perform standard tasks.
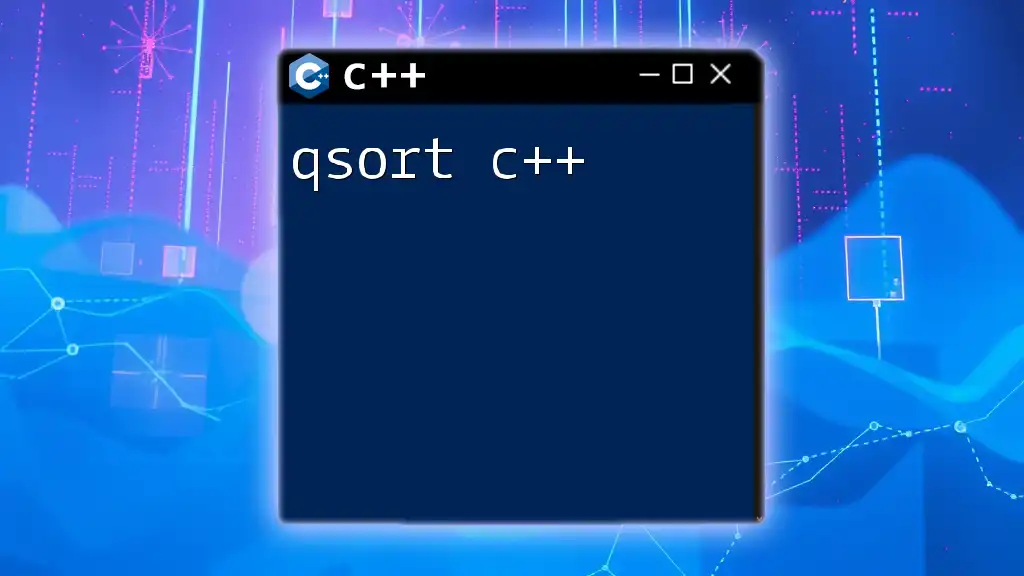
Delving Deeper: Libraries and Namespaces
Standard Libraries vs Custom Libraries
C++ offers a rich set of standard libraries that provide functionalities like data structures, algorithms, and file manipulation. However, you can also create your custom libraries to encapsulate and reuse specific functionalities across various projects.
Using Namespaces
Namespaces in C++ are utilized to organize code better and prevent naming conflicts. The standard namespace is called `std`, which contains all the standard library features. You can use the following syntax to prevent the repetitive use of `std::`:
using namespace std; // Avoid prefixing library functions with std::
It's important to note that while using namespaces simplifies your code, it can also lead to ambiguity if similarly named entities exist in different namespaces. Therefore, cautiously managing namespaces is crucial.
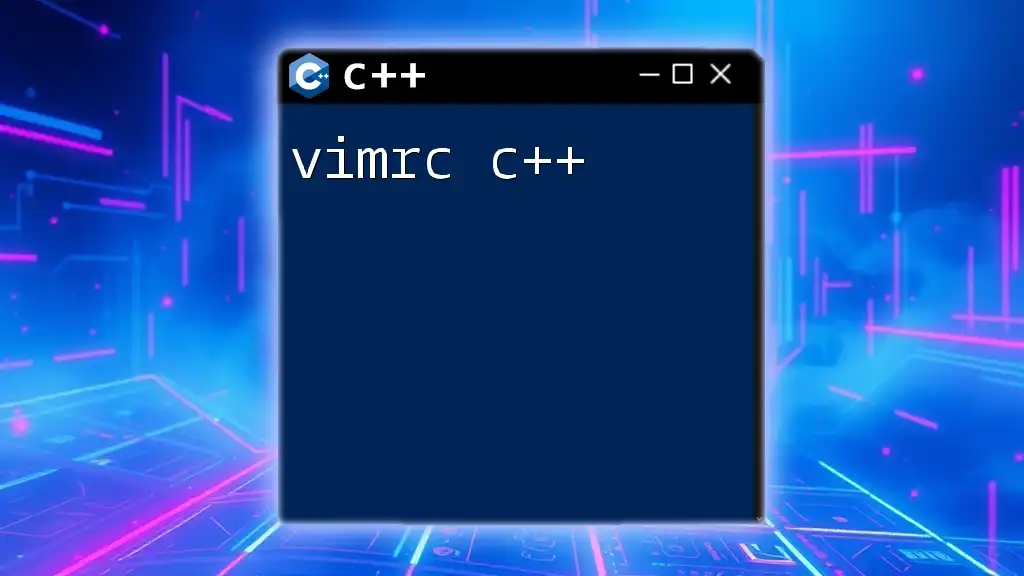
Advanced Import Techniques
Conditional Compilation
C++ allows for conditional compilation using directives like `#ifdef`. This enables certain parts of your code, including libraries, to be compiled only if specific conditions are met. This technique is particularly helpful for cross-platform development or customizing builds.
#ifdef SOME_MACRO
#include "myConditionalHeader.h"
#endif
Forward Declarations
Forward declarations are a technique where you declare the existence of a class before its implementation. It aids in avoiding circular dependencies between classes. Here’s how to use it:
class MyClass; // Forward declaration of MyClass
Implementing forward declarations correctly helps in reducing compile-time dependencies and improving the structure of your code.
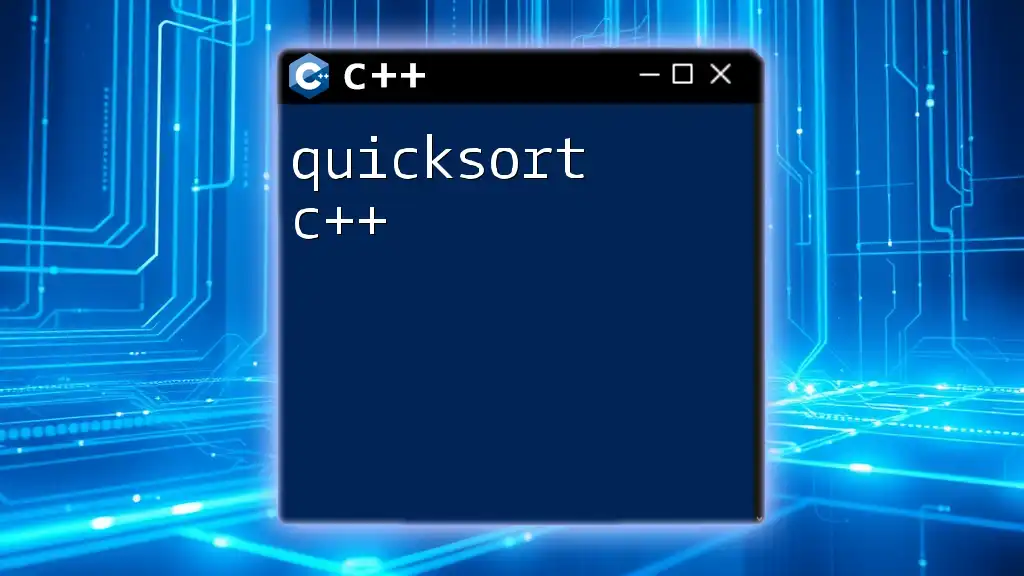
Optimizing Imports in C++
Best Practices for Importing
When it comes to importing in C++, following best practices is essential:
- Only include the libraries and headers that are necessary for your code. This keeps compilation times low and improves performance.
- Utilize forward declarations when possible to avoid excessive inclusions.
Common Pitfalls to Avoid
Importing can seem straightforward, but developers often fall into the following traps:
- Overusing `using namespace std;`: This can introduce naming conflicts, especially in larger projects. To mitigate this, aim to qualify standard library calls with `std::`.
- Circular includes: This occurs when two headers include each other, leading to compilation errors. Prevent this by structuring headers logically and using forward declarations when needed.
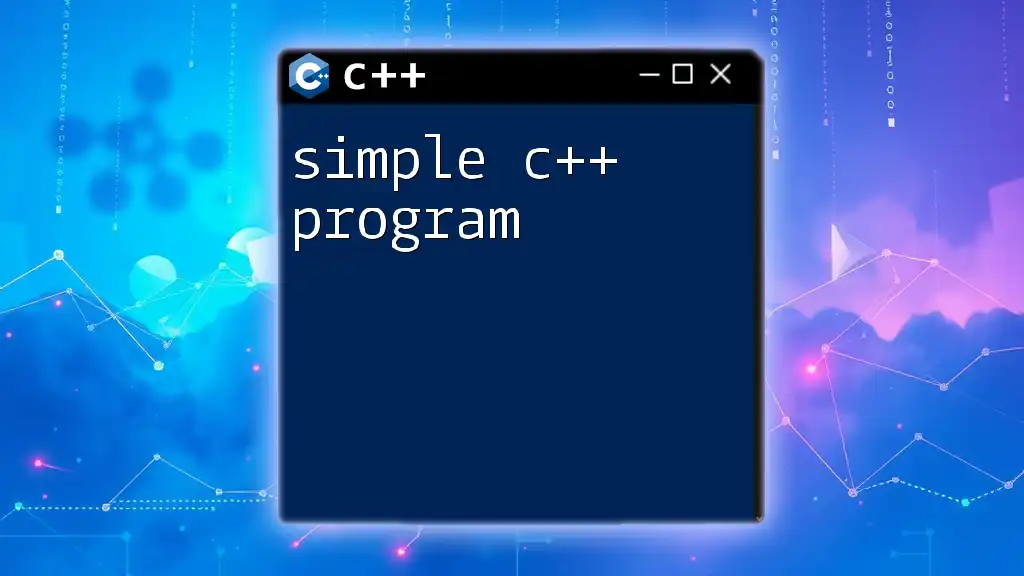
Frequently Asked Questions (FAQs)
How Do I Import Multiple Libraries?
Importing multiple libraries in C++ is straightforward. Simply list your `#include` statements, separating different libraries:
#include <iostream>
#include <vector>
This allows you to leverage both input/output functionalities and dynamic array management.
Can I Import Functions as Well?
In C++, while you do not "import functions" in the same way as classes or libraries, you can include header files that contain function declarations. When these headers are imported, you gain access to the declared functions.
// myFunctions.h
void myFunction();
// main.cpp
#include "myFunctions.h"
By this way, you organize your code and separate functionalities into different modules effectively.
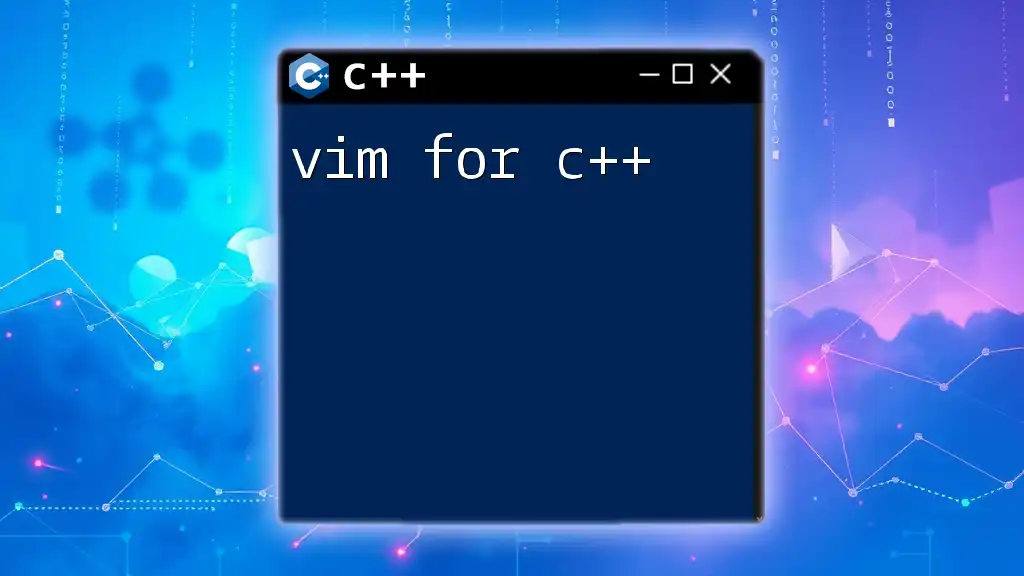
Conclusion
Importing in C++ is a vital skill for any developer looking to write efficient, modular, and maintainable code. Mastering the `#include` directive, understanding namespaces, and adhering to best practices will deepen your knowledge and improve your programming capabilities.
As you continue coding in C++, embrace the practice of importing libraries and resources that enhance your ability to solve complex problems while maintaining clean and readable code. Happy coding!
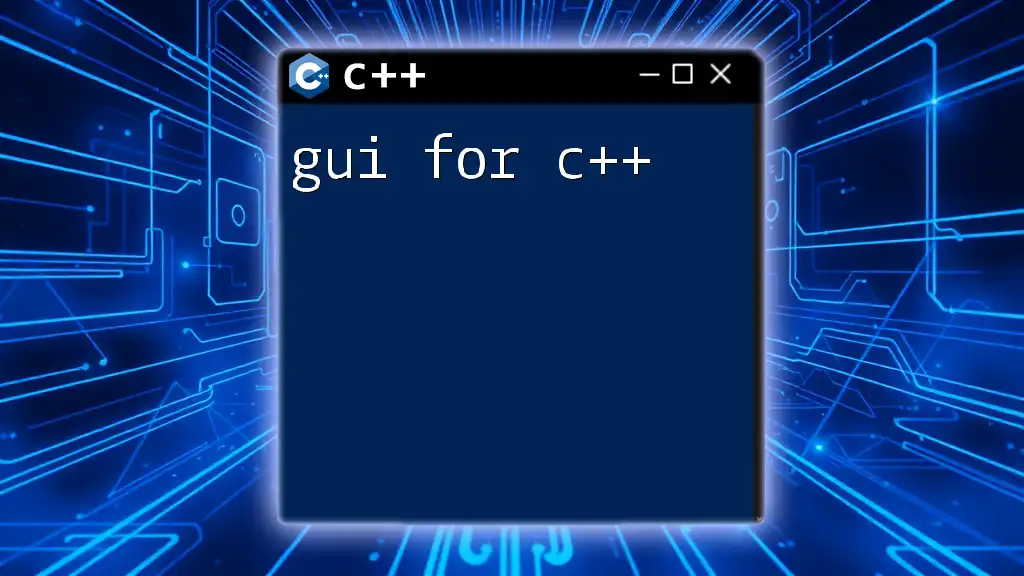
Additional Resources
For further exploration on the topic of importing in C++, consider checking out the following resources:
- C++ documentation (cppreference.com)
- Online C++ tutorials and coding platforms
- Community forums for C++ discussion and problem-solving