MISRA C++ is a set of guidelines aimed at facilitating safe and maintainable C++ code in critical systems by enforcing best practices and preventing common programming errors.
Here's an example code snippet that follows MISRA C++ guidelines:
#include <iostream>
class MyClass {
public:
void display() const; // Function declaration
};
void MyClass::display() const {
std::cout << "Hello, MISRA C++!" << std::endl; // Avoiding side effects
}
int main() {
MyClass obj; // Object creation
obj.display(); // Function call
return 0; // Main must return an int
}
What is MISRA C++?
MISRA C++ (Motor Industry Software Reliability Association C++) is a set of software development guidelines aimed at facilitating safe and reliable programming practices in C++. Originally established for C in the automotive industry, MISRA has since evolved to address the unique challenges posed by C++ in safety-critical environments. Its primary purpose is to promote a disciplined approach to code writing, ensuring that software behaves predictably in challenging operational situations.
Why MISRA C++ is Important
MISRA C++ is critical in safety-critical systems, where software failure could lead to serious consequences, such as accidents or system malfunctions. Industries that heavily rely on MISRA C++ include:
- Automotive: Ensures vehicles are equipped with software that handles numerous safety features.
- Medical Devices: Guarantees that life-supporting devices operate correctly under all circumstances.
- Aerospace: Mandates strict reliability of flight systems to maintain safety in aviation.
By adhering to MISRA C++ standards, organizations can significantly enhance the reliability, maintainability, and safety of their software. This systematic approach reduces the risk of errors, ultimately improving user trust and satisfaction.
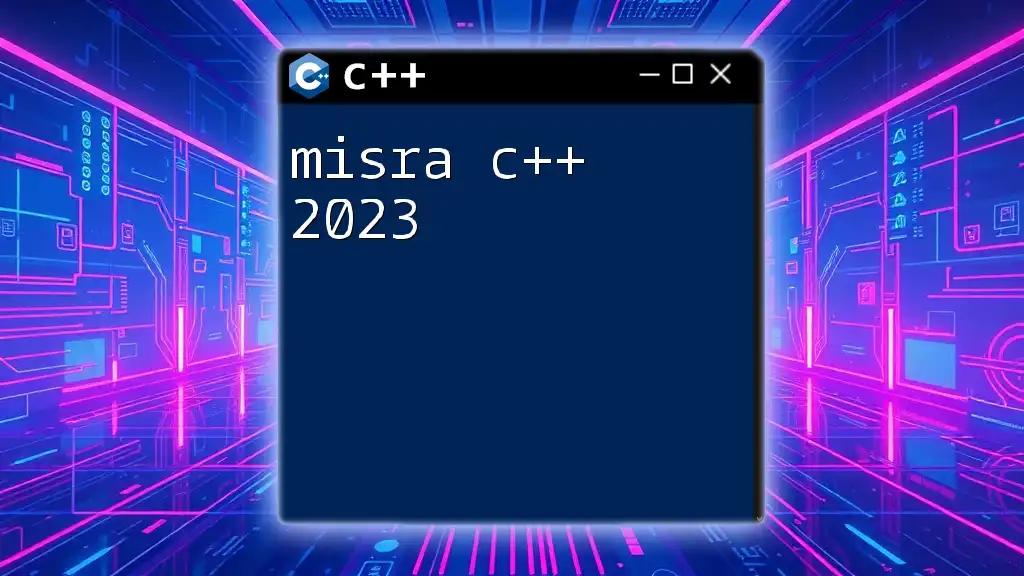
Understanding MISRA C++ Guidelines
Overview of MISRA Guidelines
The MISRA guidelines encompass a comprehensive set of rules tailored for C++ programming. These rules are categorized into three types: mandatory, required, and advisory. Each category has its significance:
-
Mandatory Rules: These are non-negotiable; failure to comply can lead to project rejection. For example, MISRA rule about avoiding the use of dynamic memory in safety-critical systems.
-
Required Rules: While not enforceable, compliance is highly recommended, as not following them may increase the risk of bugs. An instance might be the rule that discourages using certain operators in particular contexts.
-
Advisory Rules: These rules provide guidance to improve coding practices. They are not enforced strictly but adhering to them is beneficial.
Comparison with Other Standards
While MISRA C++ focuses on safety and predictability, other coding standards, like ISO C++, prioritize language features and performance. MISRA C++'s unique focus on limiting language complexity helps developers avoid undefined behavior and maintain control over the execution of the program, making it particularly appropriate for embedded systems.
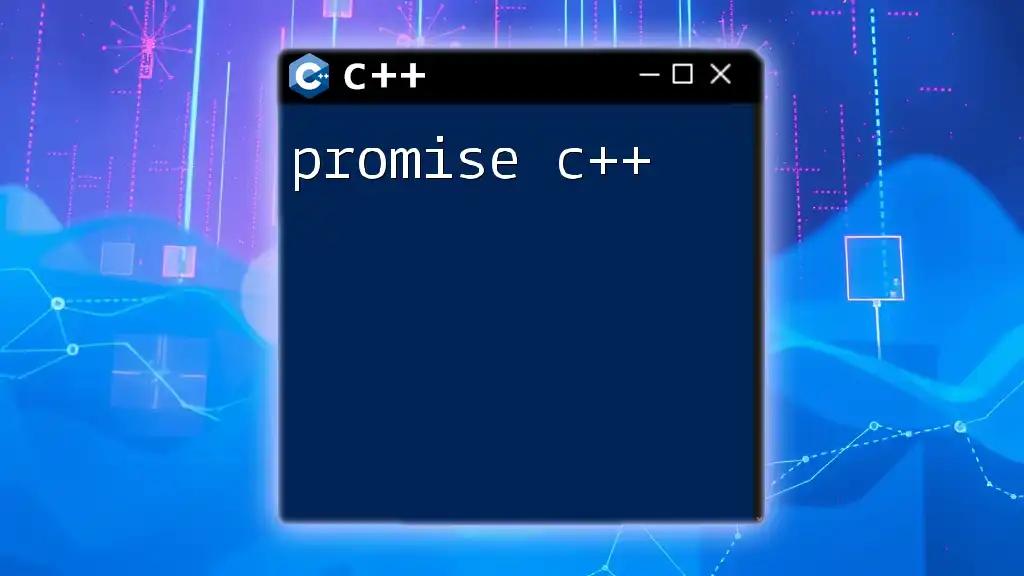
Key Concepts in MISRA C++
Major Topics Covered by MISRA C++
Type Safety is paramount in MISRA C++. This guideline emphasizes using compatible types, minimizing implicit conversions, and generally ensuring that type errors are minimized. For example, the following code snippet shows a proper approach to type usage:
int main() {
float f = 3.14;
int i = static_cast<int>(f); // Explicit conversion
}
Pointer and Reference Usage also receives special attention. The guidelines recommend avoiding outright pointer arithmetic in favor of safer alternatives, which help prevent memory access violations. An example of safe code is shown below:
void processArray(const int* array, size_t size) {
for (size_t index = 0; index < size; ++index) {
// Safe array access
std::cout << array[index] << std::endl;
}
}
When dealing with exception handling, MISRA C++ guides developers on conditions under which exceptions should be used, ensuring that exceptions do not propagate beyond appropriate boundaries. Non-compliant exception handling can be dangerous; thus, the guideline provides strategies to mitigate such risks.
Common Violations in C++
Many developers, especially those new to MISRA C++, may unintentionally violate guidelines. Common violations include:
- Using dynamic memory allocation in critical areas.
- Improper exception handling, leading to resource leaks.
To rectify non-compliant code, incorporating the appropriate guidelines into development processes is crucial. For instance, switching from dynamic memory allocations to statically allocated arrays can significantly enhance reliability.
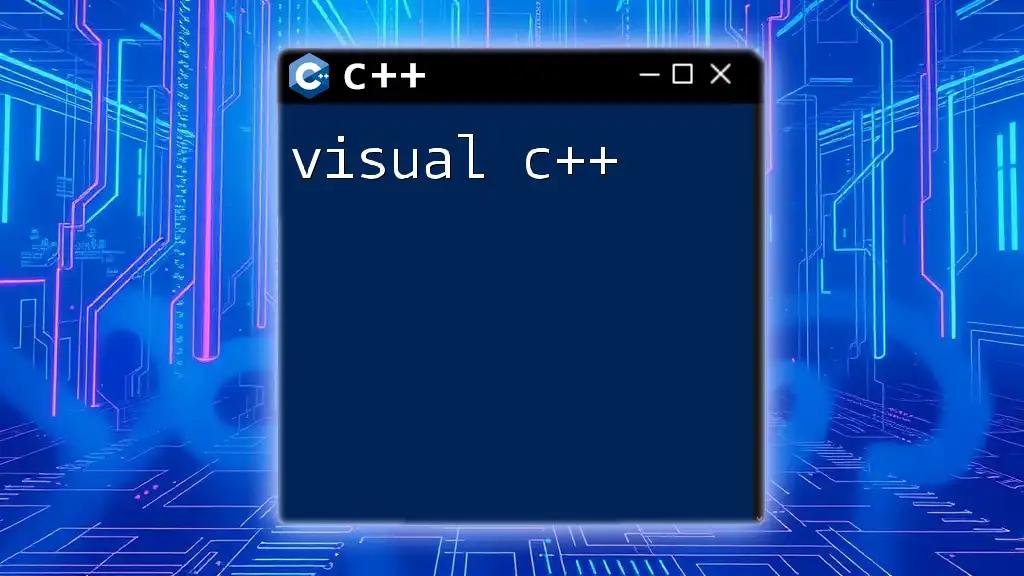
Implementing MISRA C++ in Your Projects
Steps to Integrate MISRA C++ Guidelines
To successfully integrate MISRA C++ in your projects, consider the following strategies:
-
Code Assessment Tools: Leverage tools designed to check compliance with MISRA standards. Popular choices include:
- PC-lint: Focused on static code analysis.
- CPPcheck: Open-source tool for checking coding standards compliance.
-
Developing a Compliance Strategy: Create a dedicated compliance strategy that incorporates training and regular code reviews. Setting achievable milestones can help track progress.
-
Training and Culture: Foster a coding culture that emphasizes the importance of adherence to coding standards. Regular workshops and training sessions can help keep the team updated about compliance strategies.
Best Practices for Compliance
Defining a shared coding style guide aligned with MISRA recommendations is vital. Key practices include:
-
Consistent Naming Conventions: Encourage uniformity by following specific naming conventions throughout the codebase.
-
Clear Code Comments: Documentation is vital for understanding code intentions, especially in cases of complex logic or exceptions.
Example Coding Style Guide Segment:
// Ensure all variable names begin with a lowercase letter
int count; // Correct
int Count; // Incorrect
Tools and Resources for Compliance
To maximize compliance with MISRA C++, utilize the following resources:
- Static Analysis Tools: Tools like Coverity and Klocwork can help in detecting compliance issues while developing software.
- Training Resources: Numerous online platforms offer courses designed specifically for MISRA C++.
- Community Support: Engage in forums and communities, such as Stack Overflow or specialized coding forums, to exchange knowledge and address compliance challenges.
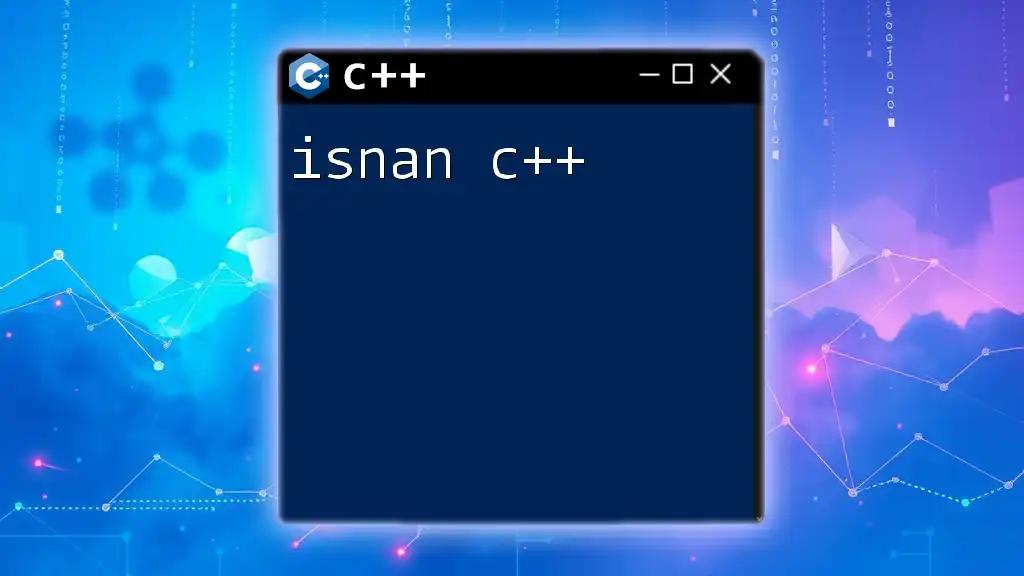
Real-World Applications of MISRA C++
Case Studies
Case Study 1: Automotive Industry
A notable example from the automotive industry demonstrates how adopting MISRA C++ principles led to a significant decrease in software errors. A major car manufacturer implemented a MISRA compliance approach and developed its vehicle control systems, leading to a car model that scored high in reliability and safety tests.
Case Study 2: Medical Devices
In the medical field, a company redesigned the software for a life-supporting ventilator. By implementing MISRA C++ guidelines, they were able to confirm that their system was not only efficient but also robust against potential software errors.
Benefits of Adhering to MISRA C++
The advantages of following MISRA C++ trends include enhanced code quality, increased team productivity, and reduced project timelines. Over the long term, the code becomes easier to maintain and extend, attracting new developers to the project without fear of introducing bugs or vulnerabilities.
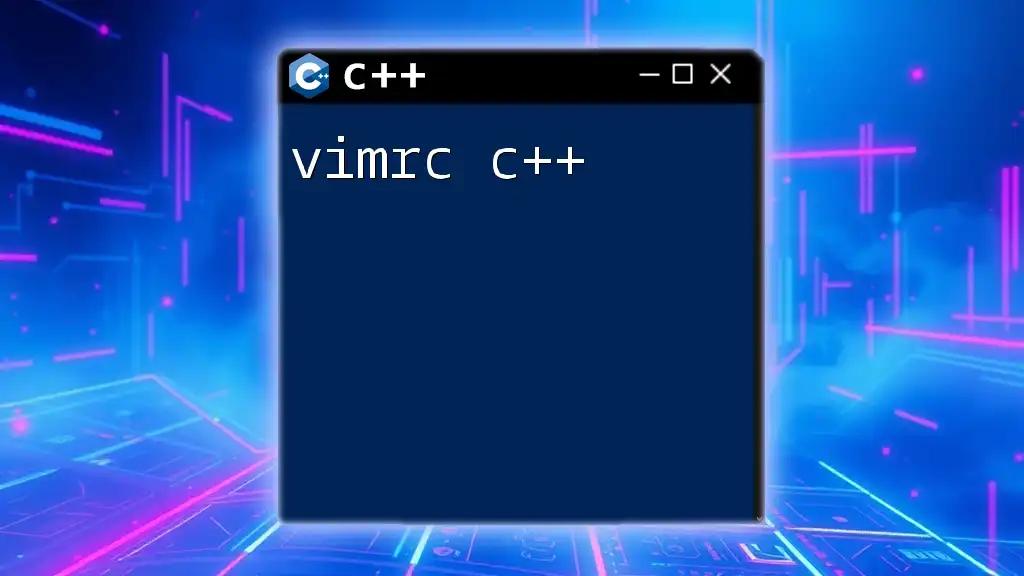
Conclusion
In summary, MISRA C++ provides a robust framework for ensuring safety and reliability in code development, particularly in critical systems. By adopting these guidelines, developers can significantly improve the quality of their software while minimizing the risk of errors. The importance of MISRA C++ cannot be overstated for organizations committed to delivering safe, efficient, and maintainable software.
As you explore ways to integrate MISRA C++ into your coding practice, consider embracing the tools, resources, and community support available to facilitate this essential journey. Adopting these guidelines is not just a compliance measure; it’s a commitment to quality and safety in programming.
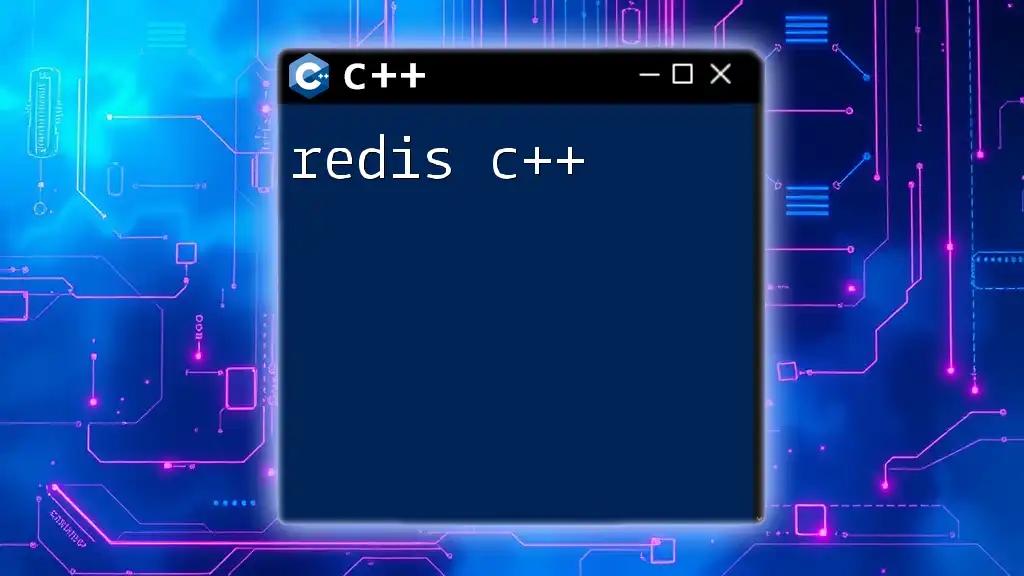
Additional Resources
Recommended Reading
For a deeper understanding of MISRA C++, consider these resources:
- "MISRA C++: 2008 Guidelines for the C++ Programming Language" - official documentation
- "Best Practices for C++ Programming" - insightful texts focusing on effective coding strategies.
Useful Links
Visit the official MISRA website for complete guidelines and detailed resources to enhance your software development practices in compliance with MISRA C++.