C++ can be challenging for beginners due to its complex syntax and concepts, but with practice and the right guidance, anyone can master it. Here's a simple example demonstrating a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is an extension of the C programming language and has become one of the most widely used programming languages in the world, particularly for system and application software, game development, and real-time systems. Developed in the early 1980s, its primary design focus is on performance and efficiency, allowing developers to have fine control over system resources. C++ is often chosen for its speed and ability to handle hardware-level manipulation, making it invaluable for developing performance-critical applications.
Key Features of C++
C++ boasts a set of powerful features that make it distinctive:
Object-Oriented Programming: C++ supports encapsulation, inheritance, and polymorphism, allowing for the creation of complex applications that are both modular and reusable.
Language Syntax and Structure: The syntax of C++ can be intricate, which may contribute to the perception of difficulty. Understanding the basic structure of C++ programs, including how to define classes, functions, and control structures, is crucial.
Memory Management and Pointers: Unlike languages with automatic memory management, C++ requires manual handling of memory allocation and deallocation. Understanding pointers, references, and dynamic memory is essential for creating efficient applications.
Standard Template Library (STL): The STL provides a set of ready-to-use classes and functions for common data structures and algorithms, allowing developers to write efficient and concise code.

How Hard is C++?
Learning Curve of C++
When considering is C++ hard, it is essential to evaluate the language's learning curve. Compared to languages like Python or Java, C++ can often seem more challenging for beginners. This is primarily due to its complexity and the necessity for manual memory management. However, many learners find that the effort is worth the payoff in functionality and performance.
Complexity in C++
Several factors contribute to the complexity of C++:
Syntax Complexity: The syntax of C++ is more verbose than that of many contemporary languages. An example is the definition of a simple class:
class Dog {
public:
string name;
void bark() {
cout << "Woof! Woof!" << endl;
}
};
When comparing this to a language like Python, where classes are defined with far less boilerplate code, it becomes clear why some learners struggle.
Memory Management: In C++, memory management is a double-edged sword; it provides developers with more control but also increases the risk of errors. Aspects such as dynamic allocation and the need to manage both constructors and destructors can overwhelm beginners. An example of dynamic memory allocation is:
int* arr = new int[10]; // Allocating an array of 10 integers
// Use arr...
delete[] arr; // Don't forget to deallocate
Object-Oriented Concepts: While object-oriented programming concepts such as classes and inheritance allow for powerful architecture, they can be daunting. Understanding how to effectively use these features is imperative and often requires practice.

Common Challenges in Learning C++
Syntax Errors and Debugging
Encountering syntax errors is a rite of passage for many C++ learners. Common issues include forgetting semicolons, mismatched brackets, or incorrect function signatures. These errors can be particularly frustrating, especially for those transitioning from languages with more lenient syntax requirements.
To ease the debugging process, utilizing tools such as GDB (GNU Debugger) or integrated development environment (IDE) debuggers can be incredibly helpful. These tools allow you to step through code, inspect variables, and trace logical flow, reducing the time spent hunting down bugs.
Advanced Features
As you delve deeper into C++, you will encounter advanced features that further complicate the landscape:
Templates: Templates enable generic programming and can be challenging to grasp. Understanding how to create and use function templates and class templates is fundamental:
template <typename T>
T add(T a, T b) {
return a + b;
}
Exception Handling: Managing errors through exception handling is crucial for building robust applications. C++ provides mechanisms for throwing and catching exceptions, but mastering this feature requires practice and careful consideration of how errors propagate through your code.
Pointers and References: Grasping the nuances of pointers and references often trips up beginners. For instance, understanding the difference between passing by value and passing by reference can drastically affect both performance and program behavior.
void modifyValue(int& ref) {
ref++;
}
int main() {
int x = 5;
modifyValue(x); // x will now be 6
}
Performance Optimization
While C++ offers unparalleled performance, with that power comes the responsibility of optimizing code. A solid understanding of the language and its features will aid in recognizing potential bottlenecks. Basic techniques include minimizing memory allocations, using the appropriate data structures from the STL, and avoiding unnecessary copying of objects.
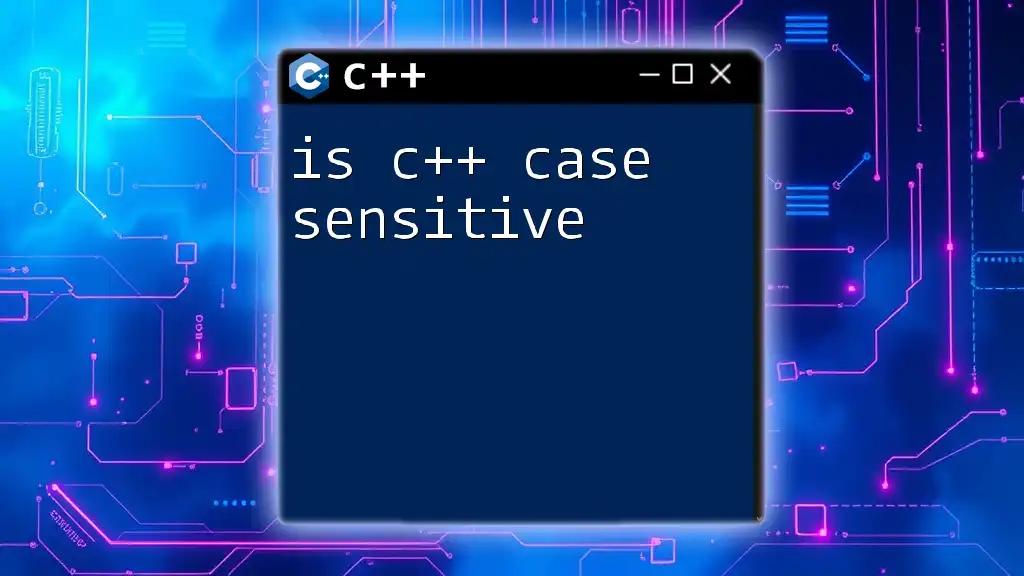
Is C++ Worth the Effort?
Industry Demand for C++ Skills
Given its prevalence in high-performance applications, the demand for C++ skills remains robust. Industries such as finance, game development, and systems programming value C++ expertise. Many companies, including tech giants and startups alike, seek individuals who can leverage C++ to create efficient and performant solutions.
Long-Term Benefits of Learning C++
Learning C++ is not merely about mastering a programming language; it’s about developing a mindset. The expertise gained through mastering C++ translates well to other languages, enhancing overall coding skills and problem-solving abilities. Moreover, C++ encourages good practices that are applicable across many programming paradigms.

Tips for Overcoming the Perceived Difficulty
Start with Fundamentals
The journey of understanding is C++ hard becomes easier when you focus on mastering the fundamentals. Begin your learning journey with the basics: control structures, functions, and simple classes. Resources such as textbooks, online courses, and tutorials can provide structured learning paths and foundational knowledge.
Practice Regularly
Regular practice is key to becoming proficient in C++. Build simple projects to apply what you’ve learned. Start with basic console applications and gradually move to more complex systems or even games. Practical, hands-on experience solidifies learning and decreases the intimidation factor of the language.
Join the Community
Engaging with the C++ community can significantly enhance your learning experience. Participating in forums, attending coding meetups, or connecting with online groups can provide motivation, resources, and networking opportunities. Gleaning insights from experienced developers can demystify difficult concepts and offer practical tips.
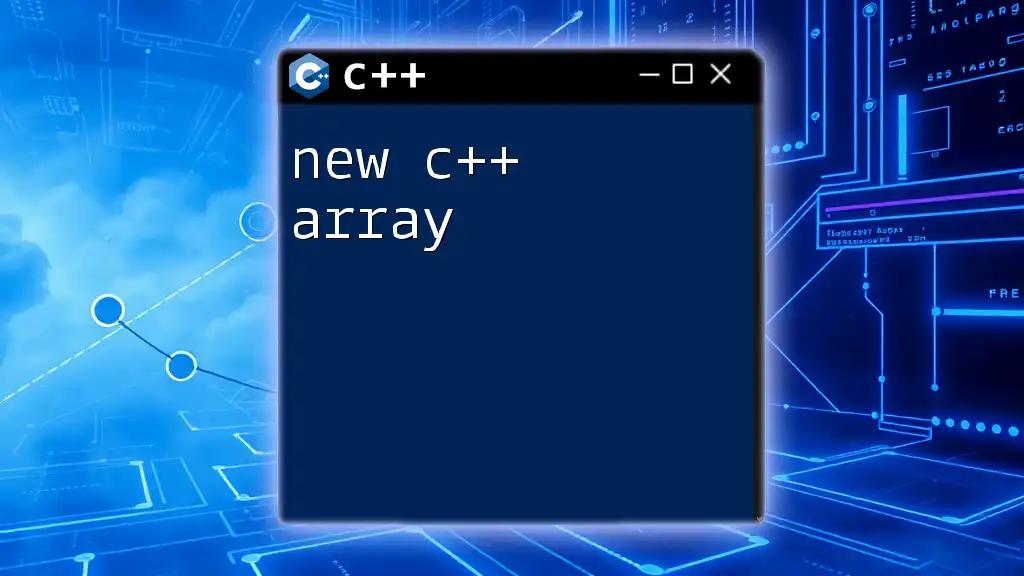
Conclusion
As we reflect on whether C++ is hard, it is important to recognize the complexity and challenges it presents to learners. However, with dedication and the right approach, anyone can overcome these hurdles. The investments made in learning C++ open doors to numerous opportunities and equip developers with invaluable skills. Embrace the challenge, and allow the rewards of becoming adept in C++ to propel your programming journey.
Take the first step—explore further resources or courses that will enhance your skills, and join a community that supports and motivates your learning. The journey may be challenging, but the learning and personal growth you gain will be well worth it.