ADL (Argument-Dependent Lookup) in C++ is a feature that allows the compiler to automatically find the appropriate function to call based on the types of the arguments passed, rather than just the function name.
Here’s a simple example demonstrating ADL:
#include <iostream>
namespace Math {
void print(int value) {
std::cout << "Value: " << value << std::endl;
}
}
void displayValue(const Math::print&) {}
int main() {
displayValue(42); // Calls Math::print via ADL
return 0;
}
What is ADL?
Argument-Dependent Lookup (ADL) is a mechanism in C++ that facilitates the process of resolving function calls based on the types of arguments passed to those functions. Unlike traditional lookup methods that rely heavily on the scope in which a function resides, ADL takes into account the types of the arguments, significantly affecting how C++ resolves the names of functions. Understanding ADL can greatly improve your coding efficiency and clarity.
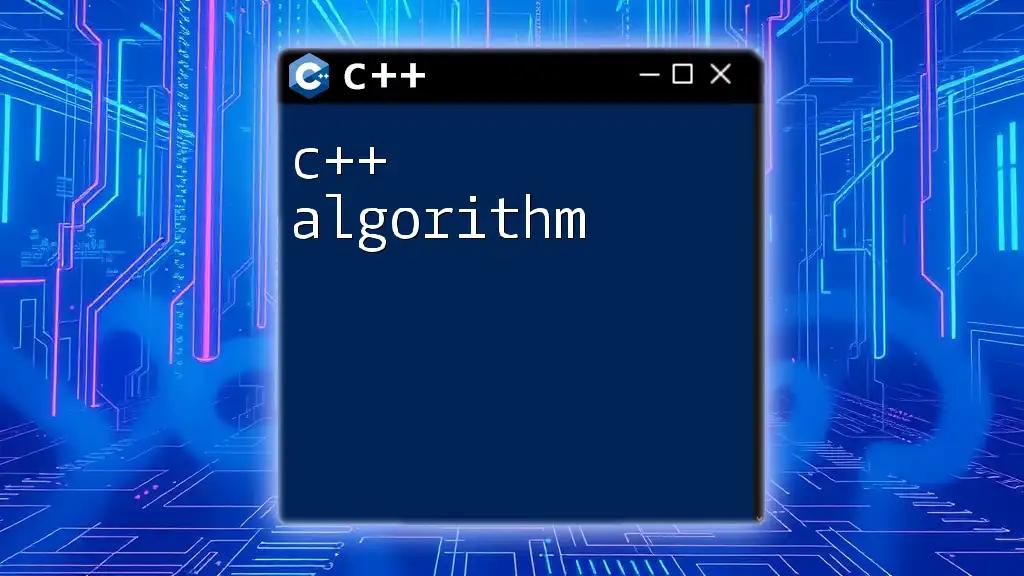
Why Use ADL?
The primary benefit of using C++ ADL is enhanced code readability. ADL allows functions that are defined within the same namespace as the argument types to be called without the need to explicitly specify their namespace. This leads to more concise and cleaner code. Moreover, ADL simplifies function resolution, so developers can call functions with less boilerplate code, making it easier to focus on core logic.
Practical scenarios where ADL can prove beneficial include:
- Overloaded Functions: It helps avoid naming clashes and ambiguities when multiple functions have the same name.
- Templates: When using templates, ADL allows functions tied to specific types to resolve seamlessly without fully qualifying their namespace.
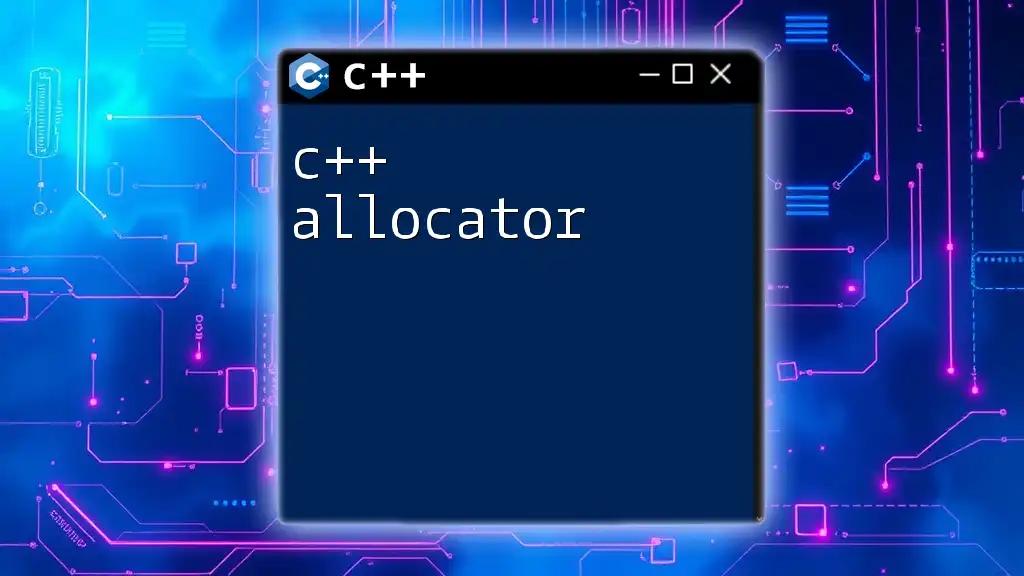
Understanding the Basics of C++ Functions
The Role of Namespaces in C++
Namespaces are a fundamental aspect of C++ programming, allowing developers to organize code in a way that prevents naming conflicts. Every function, variable, or class can belong to a namespace, which makes it easier to manage large codebases. When you use ADL, these namespaces play a critical role as they can dictate which functions can be resolved based on the arguments passed into a function call.
Function Overloading and Name Resolution
Function overloading enables multiple functions to have the same name but different parameter types. When a function call is made, C++ follows a strict set of name resolution rules to determine the appropriate function to invoke. ADL adds another layer by considering the types of the arguments provided to the function being called, which can sometimes lead to surprising behaviors. Thus, understanding name resolution rules becomes essential for utilizing C++ ADL effectively.

The Mechanism Behind Argument-Dependent Lookup
How ADL Works
When a function is called, C++ looks up the function in the scope of the caller first. If the function isn’t found, C++ considers the types of the function's arguments and performs a lookup in the namespaces associated with those types. This is where ADL shines, allowing separate namespaces to communicate based on argument types, facilitating smooth function resolution without fully qualified names.
Example of ADL in Action
Consider the following example:
#include <iostream>
namespace MyNamespace {
void greet() {
std::cout << "Hello from MyNamespace!" << std::endl;
}
}
void callGreet(); // Declaration to allow ADL
int main() {
callGreet(); // Explain why this works due to ADL
return 0;
}
void callGreet() {
// In this context, ADL is triggered due to the argument type
MyNamespace::greet(); // Resolves correctly because of ADL
}
In this example, when `callGreet` is called, ADL enables the invocation of `greet` defined in `MyNamespace` without needing to specify the namespace in the call directly.
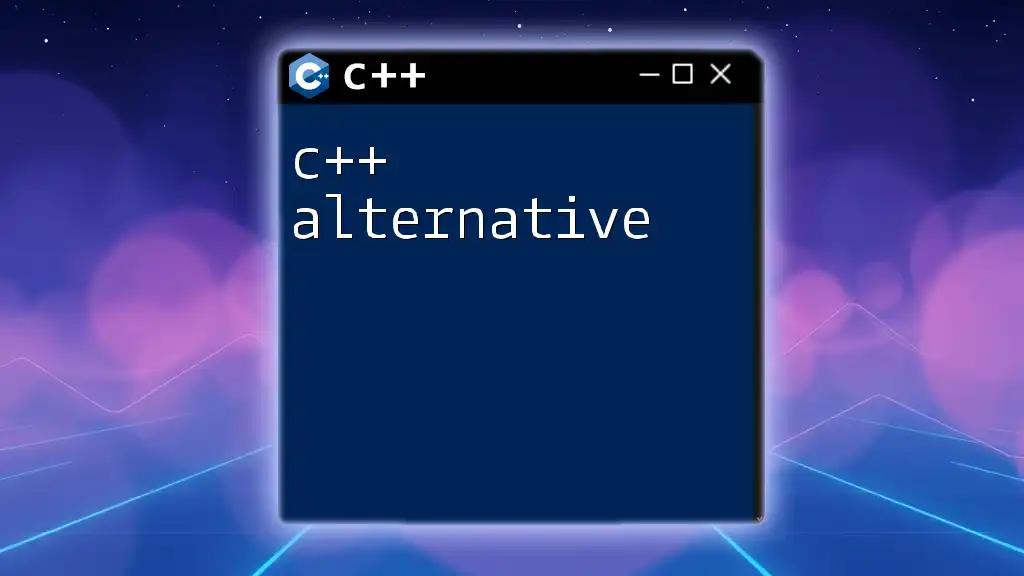
Conditions for ADL to Work
Lookup Behavior with Different Function Signatures
ADL operates under specific conditions, predominantly influenced by the function signatures. For a function to be found through ADL, the types of the arguments must be defined in the same namespace where the function is declared. If the types are not associated with the caller’s namespace, C++ will resort to traditional name resolution, leading to a failure to find the function.
Examples of ADL with Templates
ADL becomes especially powerful when combined with templates. When the type used as a template parameter is passed as an argument, ADL can automatically invoke the corresponding function within that type's namespace. Here’s a practical example:
#include <iostream>
namespace A {
struct Example {
static void func() {
std::cout << "ADL with Templates!" << std::endl;
}
};
}
template<typename T>
void callFunc() {
T::func(); // Will use ADL here
}
int main() {
callFunc<A::Example>(); // Explain why this works due to ADL
return 0;
}
In this snippet, `callFunc<A::Example>()` effectively demonstrates how the `func()` method is invoked through ADL because `Example` belongs to namespace `A`.
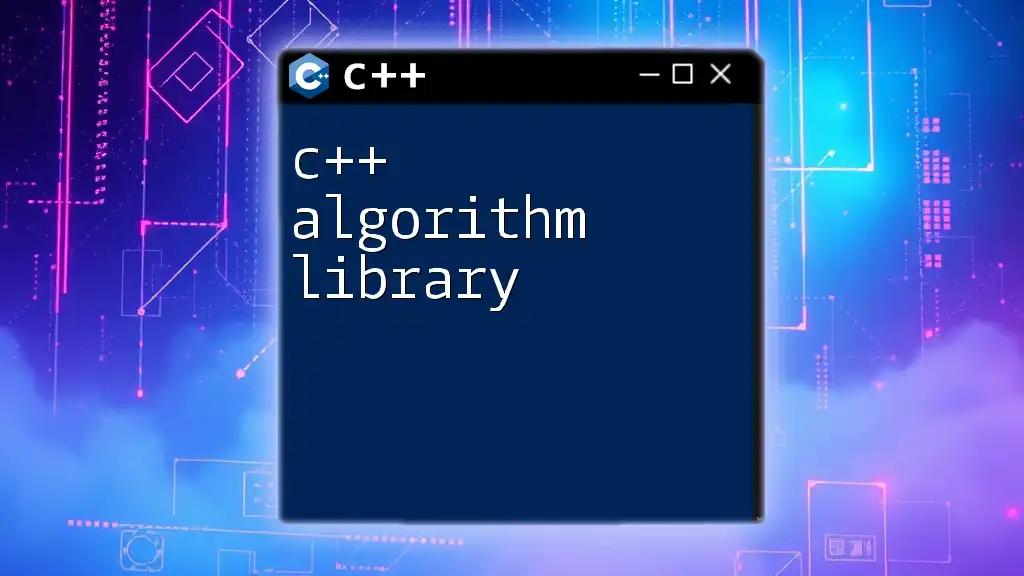
Common Pitfalls in Using ADL
Misunderstanding Function Scope
One of the most common pitfalls when using C++ ADL is misunderstanding how function scope interacts with namespaces. Developers may inadvertently assume that functions defined in a namespace will always be found when called from outside that namespace. However, this is not the case if the argument types don’t correspond to the namespace. Always be vigilant about the function’s scope and ensure your arguments are defined within the same namespace or adequately qualify the function call.
Ambiguity in Function Calls
Ambiguity can arise when multiple functions with the same name exist in different namespaces. For instance, if two overloaded functions share the same signature, and both namespaces are considered during the ADL process, C++ will produce a compilation error indicating ambiguity.
To resolve ambiguity, ensure that:
- Function arguments passed to overloaded functions have unique types or qualify names explicitly.
- Use `using` declarations judiciously to control which namespaces you’re exposing to avoid clashes.
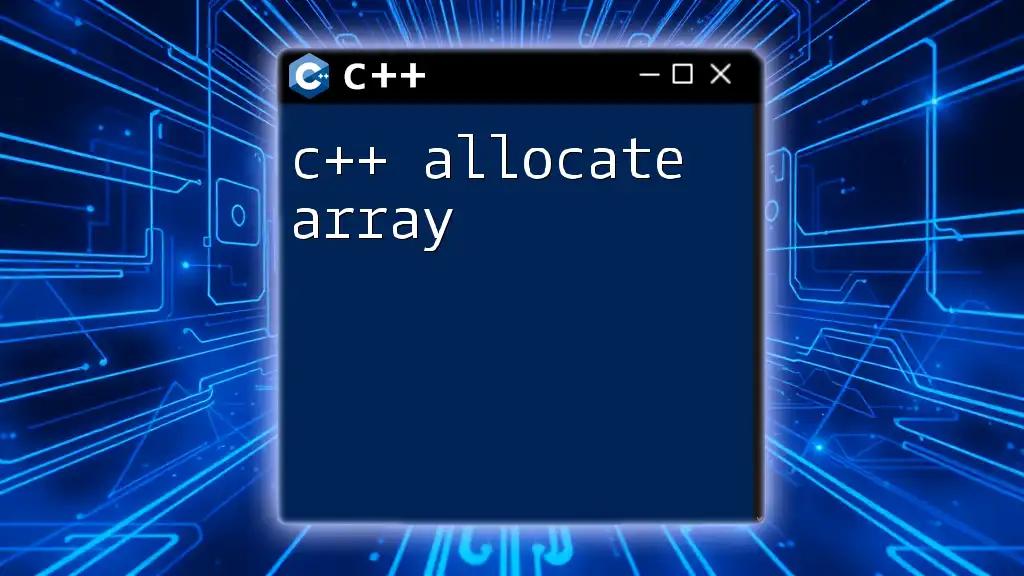
Best Practices for Using ADL
Using Clear Namespaces
To reap the full benefits of C++ ADL, use clear and organized namespaces. This simplifies maintainability and enhances readability. Namespaces should be logically related to the functionality they encompass, making it easy for others (and yourself) to understand your code at a glance.
Balancing Readability and Functionality
While ADL can reduce verbosity in your code, it is essential to balance its usage with readability. Excessive reliance on ADL may obfuscate function origins, especially in large codebases. Maintain clarity by not overusing ADL, particularly in complex functions. Explicitly qualify function calls when needed to prevent confusion.

Conclusion
In summary, understanding C++ ADL significantly enhances your coding efficiency by streamlining function resolution and reducing the need for verbose namespace specifications. By considering the types of arguments passed, you open doors to cleaner and more readable code. As you develop your C++ skills, integrating ADL into your programming style can lead to more elegant solutions and boost your proficiency. Remember, while ADL can be a powerful tool, always strive for clarity and maintainability in your code. Engage with the C++ community and continue to explore the various nuances of C++ features.

Additional Resources
Recommended Reading
For those looking to deepen their knowledge on advanced C++ topics, consider exploring reputable books and articles that focus on the intricacies of the language. Online courses that teach C++ concepts can help solidify your understanding and application of ADL.
Community and Forums
Engagement with programming communities can provide real-world insights into best practices and pitfalls related to C++ ADL. Learning from experienced developers can enhance your coding journey, offering practical advice that textbooks often overlook.