In C++, you can allocate an array dynamically using the `new` operator, which allows for flexible memory allocation at runtime.
Here's a simple example:
int* myArray = new int[10]; // dynamically allocates an array of 10 integers
What is Array Allocation in C++
Array allocation in C++ refers to the method of allocating memory for arrays. Understanding how array allocation works is fundamental for effective programming in C++.
Definition
An array is a collection of variables of the same type, accessed using a single identifier and indexed using an integer. Memory for these arrays can be allocated either statically or dynamically, each having its own implications on performance and memory management.
Types of Arrays
-
Static Arrays
Static arrays have a fixed size determined at compile time. They are allocated on the stack, and their memory is automatically deallocated when they go out of scope. This makes them faster but less flexible. -
Dynamic Arrays
Dynamic arrays, in contrast, are allocated at runtime on the heap. This allows for greater flexibility as their size can change during the program's execution. However, with this flexibility comes the responsibility for manual memory management.
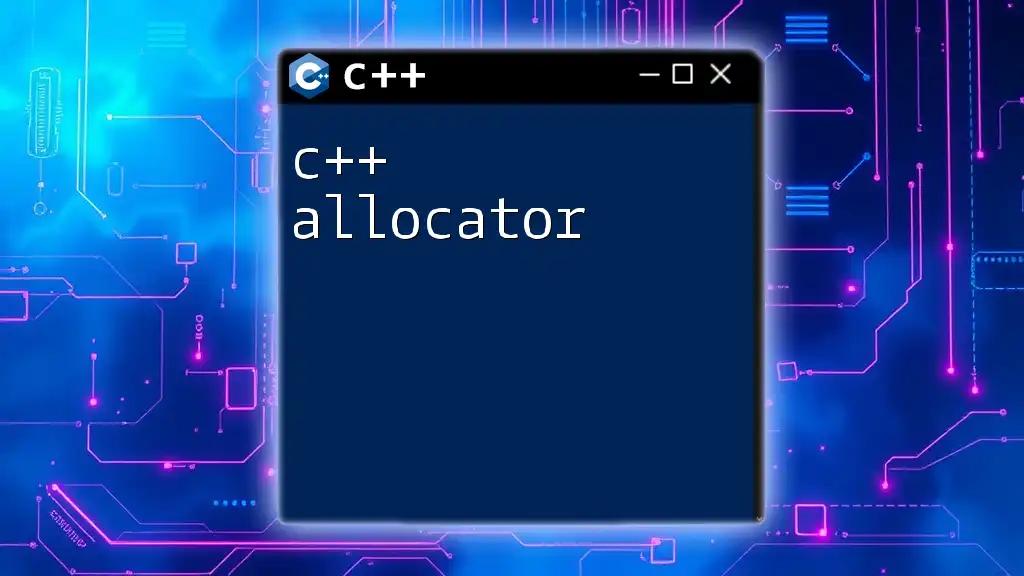
Why Use Dynamic Array Allocation?
Benefits of Dynamic Arrays
Dynamic arrays are advantageous in several ways:
-
Flexibility in Size: You can allocate or deallocate memory as needed. This is particularly useful in scenarios where the number of elements is not known beforehand, such as when reading input data from a file.
-
Efficient Memory Management: Unlike static arrays, which may allocate more memory than required, dynamic arrays allow developers to use memory more judiciously, allocating only what is necessary.
When to Choose Dynamic over Static Arrays
Dynamic arrays become an essential choice when:
- The size of the data set cannot be determined at compile time.
- You need to resize the array frequently.
- Memory usage needs to be optimized based on runtime conditions.
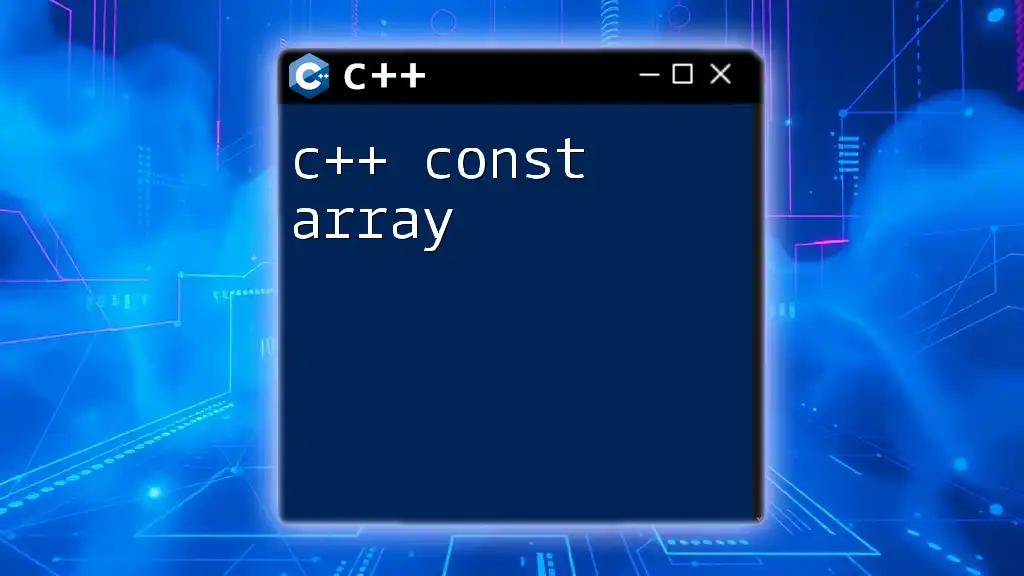
How to Allocate Arrays in C++
Using C++ Operators
Allocating Static Arrays
Static arrays are defined at compile time. The syntax is straightforward:
int numbers[5] = {1, 2, 3, 4, 5};
In the above example, an array named `numbers` is allocated with a size of 5 and initialized with values. The memory for this array is allocated on the stack.
Allocating Dynamic Arrays
Dynamic arrays are allocated using the `new` operator. This allows for the size to be defined at runtime:
int* dynamicArray = new int[5];
Here, `dynamicArray` points to an array of 5 integers that reside in the heap. Unlike static arrays, you must manage the memory yourself, including deallocation when the array is no longer needed.
Using Standard Library Features
std::vector
C++ Standard Library provides the `std::vector` class, which simplifies dynamic array management. It offers several powerful features, such as automatic resizing and memory management:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
With `std::vector`, there is no need to manually allocate or deallocate memory. Methods such as `push_back()` allow you to add elements efficiently, and the vector automatically manages memory and element copying.
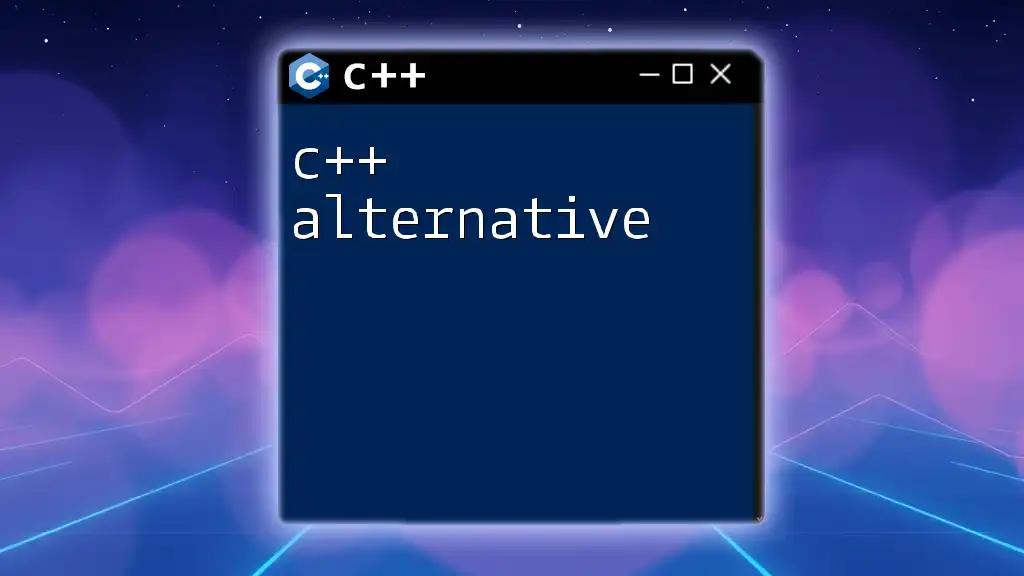
How to Deallocate Arrays in C++
Deallocating Dynamic Arrays
When you allocate a dynamic array using `new`, it is crucial to release the memory once you are done using it. Failing to do so leads to memory leaks:
delete[] dynamicArray;
This command frees up the memory allocated for `dynamicArray`. Always remember to match each `new` with a corresponding `delete` to ensure proper memory management.
Managing Memory with std::vector
Using `std::vector` simplifies memory management because vectors automatically handle memory allocation and deallocation. When a vector goes out of scope, its destructor is called, automatically freeing the associated memory.
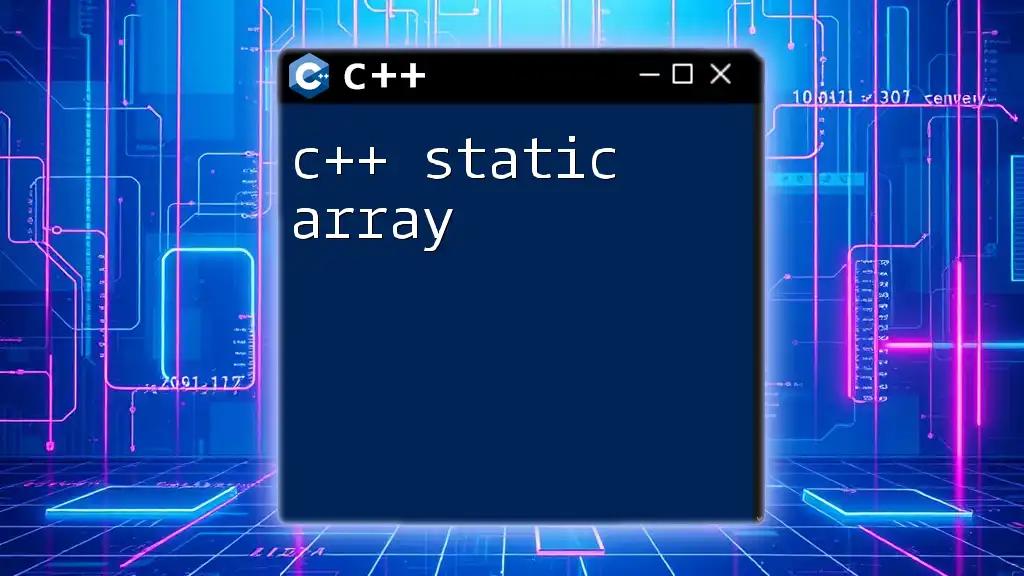
Best Practices in Array Allocation
Choosing the Right Type
When deciding between static and dynamic arrays, consider your needs:
- Use static arrays for fixed-size collections where performance is critical.
- Opt for dynamic arrays when size varies or performance specifics are less of a concern.
Preventing Memory Leaks
Preventing memory leaks is crucial for application stability. Ensure that every dynamically allocated array has a corresponding `delete` statement. It helps to establish guidelines for memory management in your codebase.
Using Smart Pointers
Smart pointers, like `std::unique_ptr`, can help manage dynamic arrays without the risks associated with manual memory management:
#include <memory>
std::unique_ptr<int[]> smartArray(new int[5]);
With smart pointers, memory is automatically deallocated when the pointer goes out of scope, reducing the risk of memory leaks.
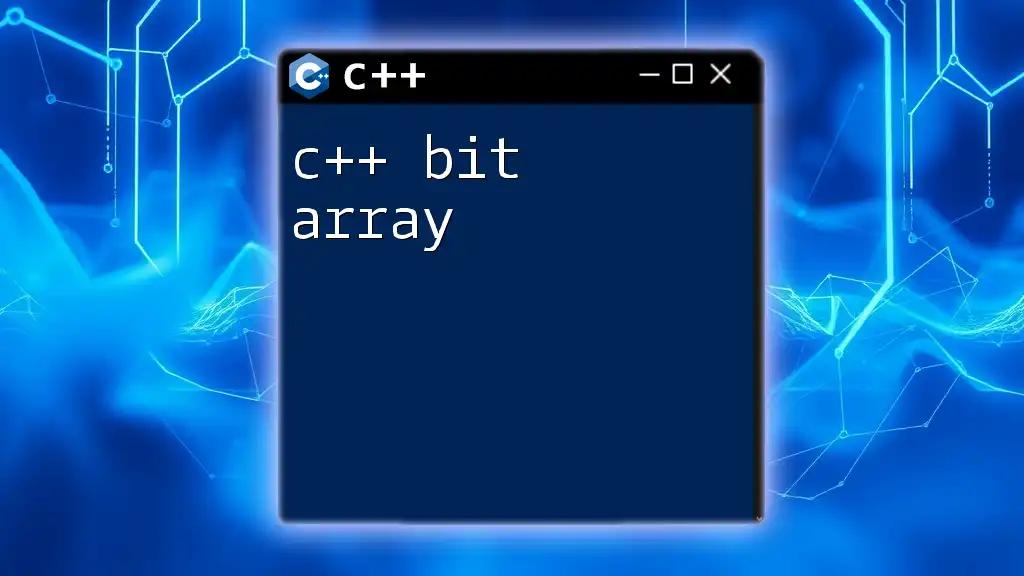
Common Pitfalls in Array Allocation
Off-by-One Errors
Off-by-one errors are prevalent when managing arrays. Always ensure that you account for zero-based indexing, leading to potential issues if you try to access an index outside the valid range.
Not Deallocating Memory
Forgetting to deallocate memory after using dynamic arrays can result in memory leaks, causing performance degradation or crashes in applications, especially in long-running processes.
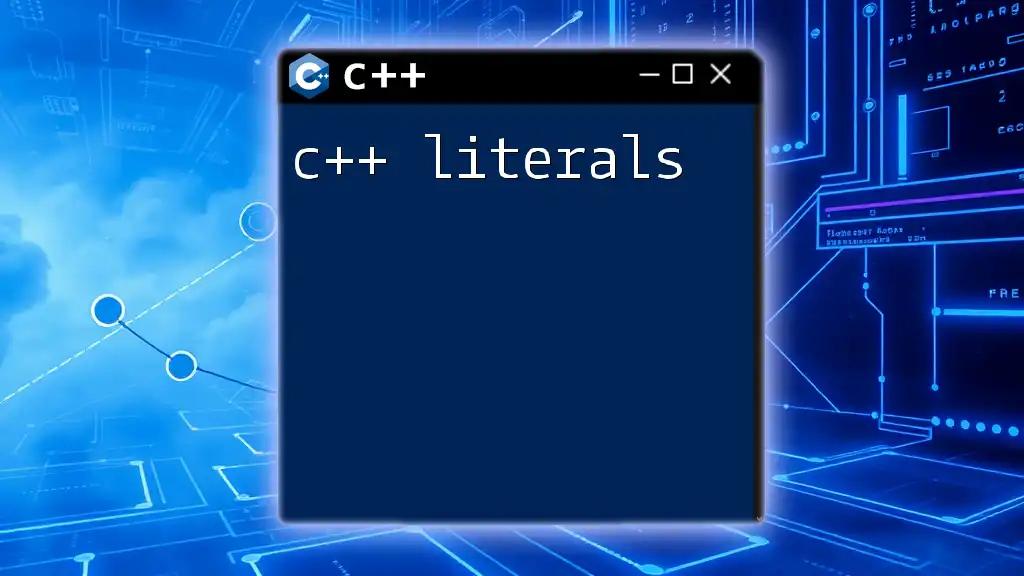
Conclusion
Understanding how to c++ allocate array effectively is a vital part of using C++. By knowing when and how to use static and dynamic arrays, along with best practices for memory management, you can craft more efficient and reliable applications. Practice these concepts in your coding projects to solidify your understanding and skills in array management.

Additional Resources
For more in-depth exploration of C++ array allocation and dynamic memory management, consider the following resources:
- C++ documentation
- Recommended books such as "Effective C++" by Scott Meyers
- Online courses or tutorials focusing on C++ programming.