C++ loops allow you to iterate through elements in an array efficiently, enabling concise data processing.
Here's a simple example using a `for` loop to print elements of an array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int length = sizeof(arr) / sizeof(arr[0]);
for(int i = 0; i < length; i++) {
cout << arr[i] << " ";
}
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a collection of elements, all of the same type, stored in contiguous memory locations. They are particularly useful for storing multiple values under a single variable name, allowing for organized data management.
Arrays can be classified as either fixed-size or dynamic. Fixed-size arrays have a predetermined number of elements set at compile-time, while dynamic arrays can change in size at runtime, typically managed using pointers and the `new` keyword.
Memory allocation for arrays is a key concept to grasp. When you declare an array, the compiler allocates a fixed block of memory, making accessing elements fast and efficient.
Example: Declaring and initializing an array can be as simple as:
int numbers[5] = {1, 2, 3, 4, 5};
Accessing Array Elements
Accessing and modifying elements in an array can be done using the array index. Each element has a specific index, starting from zero. It’s critical to note that the index must be within the bounds of the array. Attempting to access an out-of-bounds index can lead to undefined behavior.
Code Snippet: The following demonstrates accessing an array element and modifying it:
cout << numbers[0]; // Output: 1
numbers[2] = 10; // Modifies the third element
Multi-Dimensional Arrays
Multi-dimensional arrays, such as 2D arrays (often used for matrices), extend the concept of arrays into multiple dimensions. These arrays are particularly useful in representing structures involving grids or tables.
Example: Declaring a two-dimensional array looks like this:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Here, `matrix` contains three rows and three columns, allowing for more complex data storage.
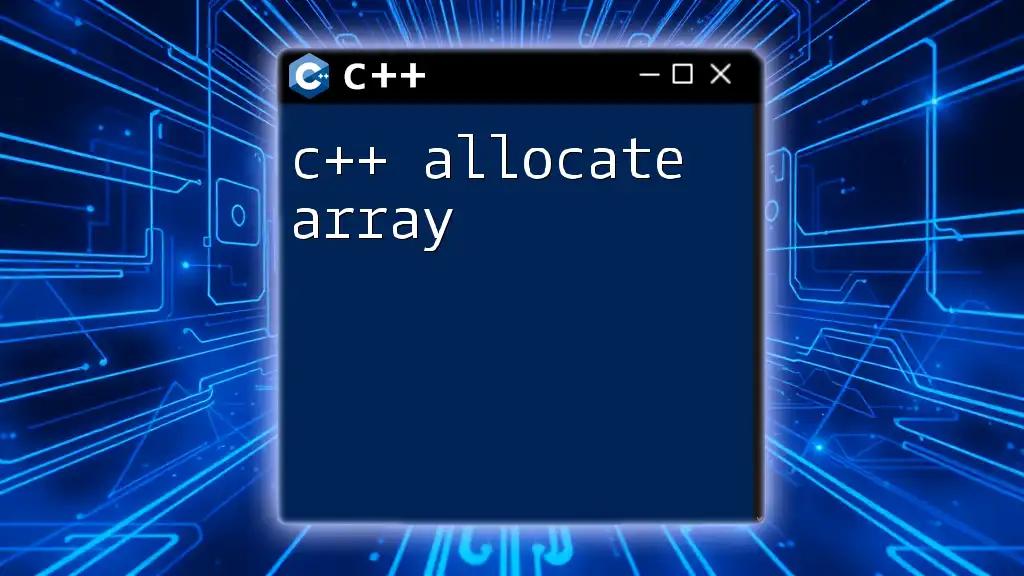
Understanding Loops in C++
What are Loops?
Loops are essential constructs in programming that allow for repeated execution of a block of code as long as a specified condition is met. They provide a mechanism for automating repetitive tasks, enhancing code efficiency and readability. Within C++, there are several types of loops, each suited for different use cases.
The `for` Loop
A `for` loop is particularly effective when the number of iterations is known ahead of time. It comprises initialization, a condition check, and an incrementation statement.
Code Snippet: The following demonstrates a basic `for` loop:
for (int i = 0; i < 5; i++) {
cout << i; // Output: 01234
}
This loop starts at `0` and iterates until it reaches `5`, incrementing `i` by `1` each time.
The `while` Loop
In contrast, the `while` loop is more suitable when the number of iterations is not predetermined and relies on a condition evaluated before each loop iteration.
Code Snippet: Here’s a simple example of a `while` loop:
int i = 0;
while (i < 5) {
cout << i; // Output: 01234
i++;
}
This loop will continue iterating as long as `i` is less than `5`.
The `do-while` Loop
The `do-while` loop is similar to the `while` loop but guarantees at least one execution of the loop's body, as the condition is checked after the loop runs.
Code Snippet: A basic example of a `do-while` loop looks like this:
int i = 0;
do {
cout << i; // Output: 01234
i++;
} while (i < 5);
This loop will print the values of `i` and check the condition after executing the loop body.
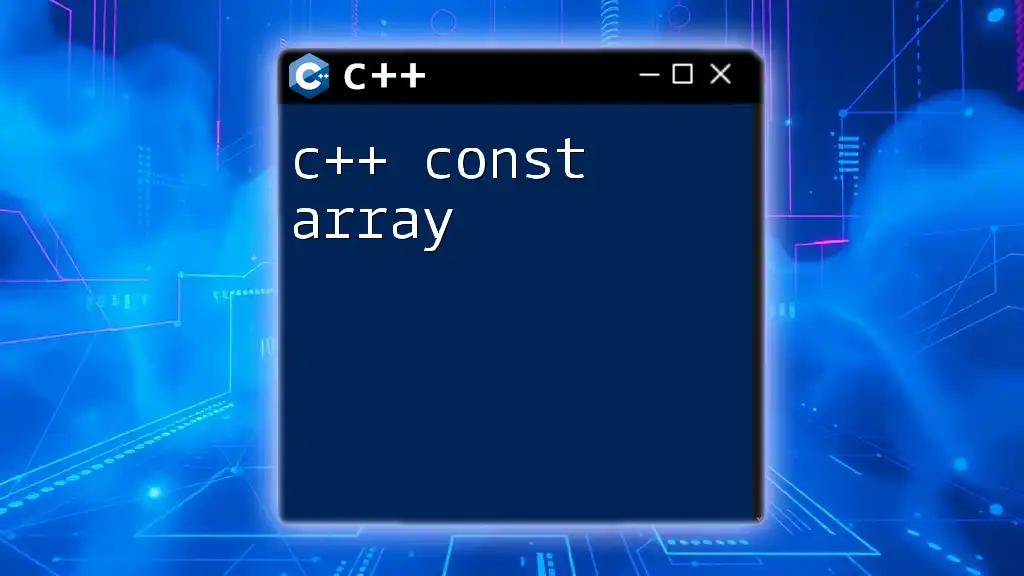
Using Loops with Arrays
Iterating Through an Array Using a `for` Loop
Utilizing loops with arrays allows for efficient data manipulation. A common use case is iterating through each element of an array using a `for` loop.
Code Snippet: Here’s how you can loop through an array:
for (int i = 0; i < 5; i++) {
cout << numbers[i]; // Output: 12345
}
This example demonstrates how to access each number in the `numbers` array sequentially.
Iterating Through a 2D Array
Nested loops are the best way to navigate multi-dimensional arrays, such as a 2D array. The outer loop typically iterates over the rows, while the inner loop iterates over the columns.
Code Snippet: This example shows how to iterate through a 2D array:
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << matrix[i][j] << " ";
}
cout << endl; // Outputs each row of the matrix
}
This will produce a structured output of the matrix’s content, effectively representing the grid-like pattern.
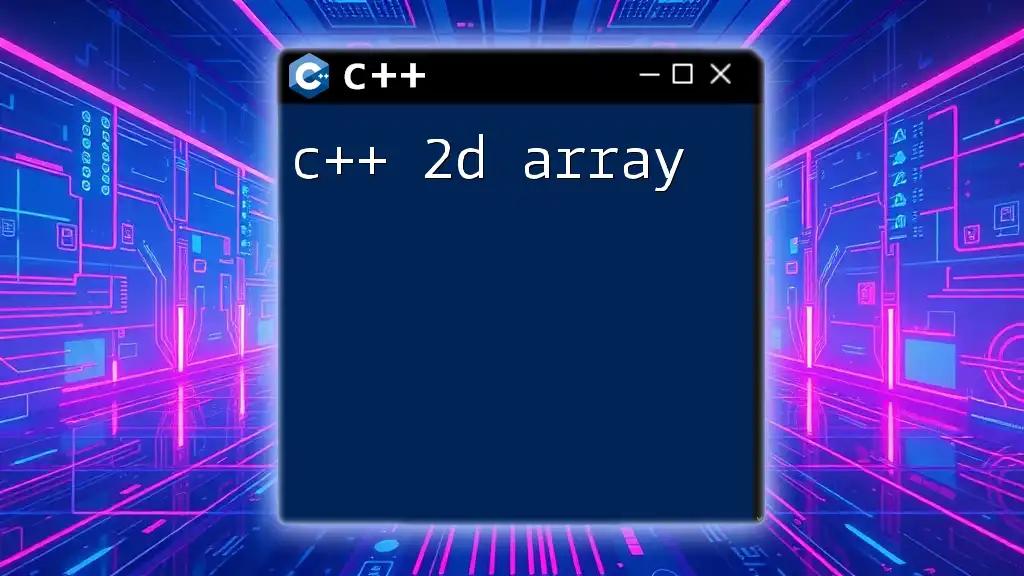
Common Mistakes and Best Practices
Common Mistakes with Arrays and Loops
As you work with arrays and loops in C++, several common pitfalls can arise:
- Off-by-one errors often occur in loop conditions, causing unintended iterations or missing elements.
- Failure to initialize variables can lead to garbage values in output or malfunctioning loops.
- Accidentally accessing out-of-bounds elements can result in crashes or undefined behavior.
Best Practices
To avoid these pitfalls, adhere to best practices:
- Use `const` for array sizes to promote clearer, safer code.
- When using dynamic arrays, prefer `std::vector` for flexibility and ease of use.
- Maintain proper commenting and code organization to improve readability and maintainability.
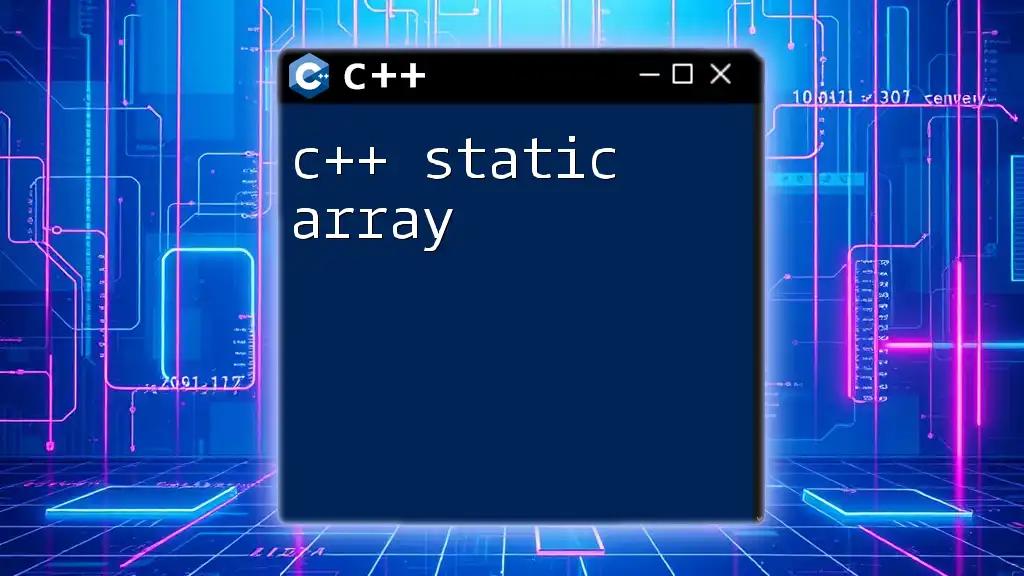
Conclusion
In conclusion, mastering C++ loops and arrays is essential for efficient programming. Understanding how to manipulate arrays with different types of loops not only streamlines your code but also enhances its overall functionality. Regular practice is key to becoming proficient, so take the time to experiment with different scenarios. Keep exploring and leveraging these concepts to create robust applications and solutions in your programming journey.