A C++ 2D array is essentially an array of arrays, allowing you to store and manage data in a grid-like structure, which can be utilized for various applications such as matrices or game boards.
Here's a simple code snippet demonstrating how to declare and initialize a 2D array in C++:
#include <iostream>
int main() {
int arr[3][4] = { {1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12} };
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 4; ++j) {
std::cout << arr[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
Understanding the Structure of a 2D Array
Definition
A 2D array is essentially an array of arrays, allowing you to handle data in a matrix format with a defined number of rows and columns. This structure is beneficial whenever you need to work with tabular data, such as grids, charts, or game boards.
When working with C++ 2D arrays, you can think of them as being indexed by two integers: one for the row and one for the column. This capability highlights the organization of data and eases operations on multiple dimensions.
Memory Representation
Row-Major Order
In C++, 2D arrays are stored in row-major order, which means that the elements of each row are stored in contiguous memory locations. This organization increases efficiency for most common applications, especially when traversing rows in loops.
For example, consider a 2D array defined as follows:
int array[2][3] = { {1, 2, 3}, {4, 5, 6} };
In memory, this array is laid out like this:
1 2 3 4 5 6
This layout can help improve cache locality when traversing rows, providing a performance advantage in many scenarios.
Column-Major Order
Some programming languages, like Fortran, utilize column-major order, which stores column elements contiguously. You might encounter this when interacting with different systems or applications, making it essential to understand how to transition between the two types of memory layouts.
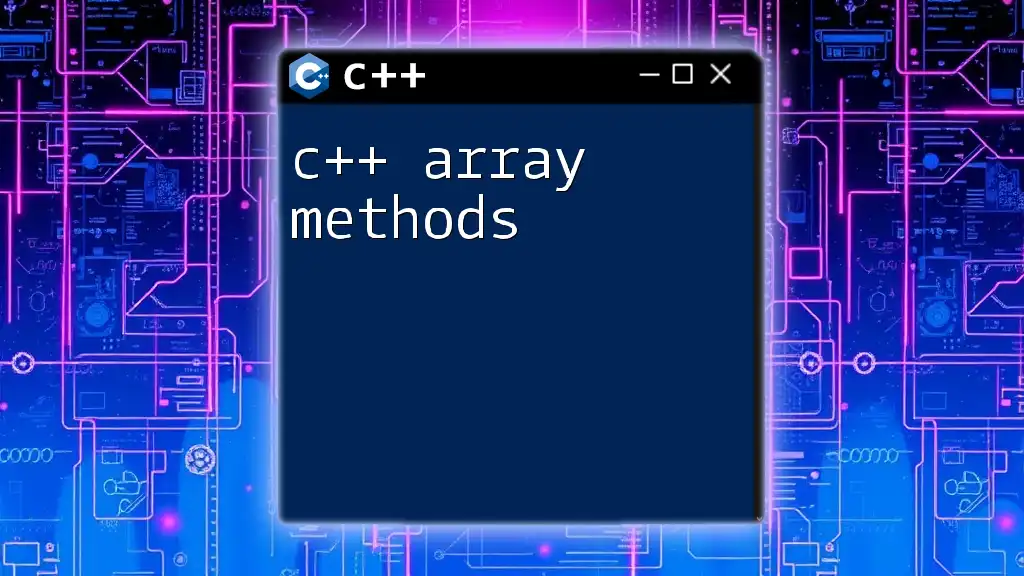
Declaring and Initializing 2D Arrays in C++
Syntax for Declaration
Declaring a 2D array in C++ is straightforward. You state the type followed by two dimensions. For instance:
int array[3][4]; // This declares a 2D array with 3 rows and 4 columns.
This syntax creates an empty 2D array in memory, ready for storing integers.
Initialization of 2D Arrays
Static Initialization
Static initialization allows you to specify values at the point of declaration. For example:
int array[3][4] = { {1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12} };
In this case, the array is filled with predefined values, significantly enhancing clarity and usability.
Dynamic Initialization
Alternatively, you can initialize a 2D array dynamically using loops:
int array[3][4];
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 4; ++j) {
array[i][j] = i + j; // This assigns the sum of indexes for initialization
}
}
This approach is beneficial for cases where the size is determined at runtime or when you want to calculate the values based on certain criteria.
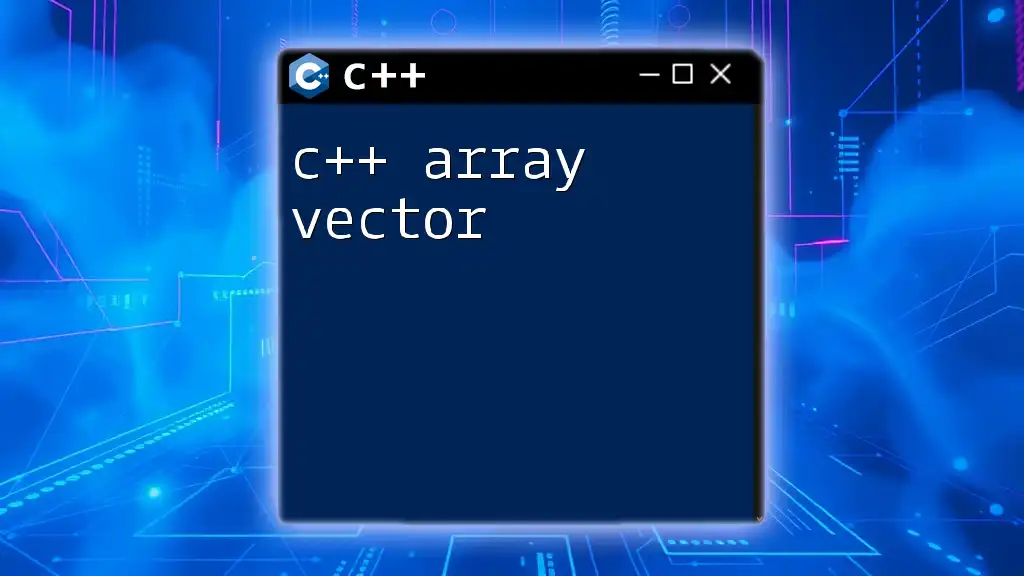
Accessing 2D Array Elements
Indexing Basics
Accessing elements in a C++ 2D array involves using two indices—one for the row and one for the column. For example, to access the element in the second row and third column:
int value = array[1][2]; // This accesses the element at row 1, column 2
It’s crucial to remember that indices start at 0 in C++, meaning that the first row and the first column are both at index 0.
Modifying Elements
You can easily change the values in a 2D array using the same indexing syntax. For instance, to update the value in the first row and first column:
array[0][0] = 100; // Changes the value to 100
This kind of operation allows for dynamic content management in applications, letting you modify data as needed while the program is executing.
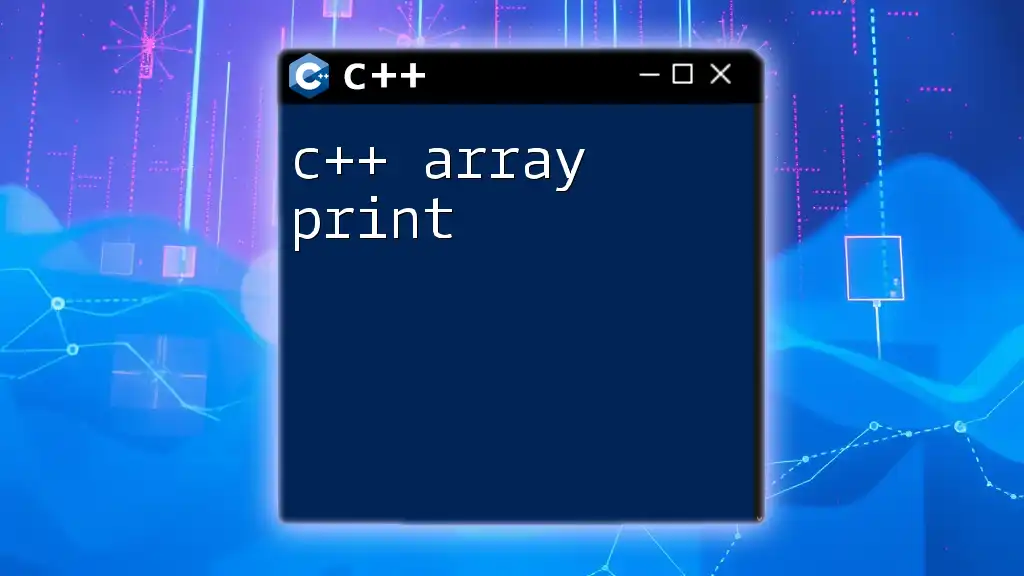
Using 2D Arrays in Functions
Passing 2D Arrays to Functions
When you need to work with 2D arrays in functions, the syntax requires you to specify the dimensions. For example:
void printArray(int arr[][4], int rowCount) {
for (int i = 0; i < rowCount; ++i) {
for (int j = 0; j < 4; ++j) {
std::cout << arr[i][j] << " ";
}
std::cout << std::endl; // Print a new line after each row
}
}
In this example, `printArray` accepts a 2D array (with a specific number of columns) and a count of how many rows to process. This structure allows you to work flexibly with matrices of different dimensions.
Return Types
Returning 2D arrays from functions requires using pointers. You cannot directly return an array type, but you can return a pointer to a dynamically allocated 2D array, which allows for more complex data management:
int** createArray(int rows, int columns) {
int** array = new int*[rows];
for (int i = 0; i < rows; ++i) {
array[i] = new int[columns];
}
return array; // Returns a pointer to the array
}
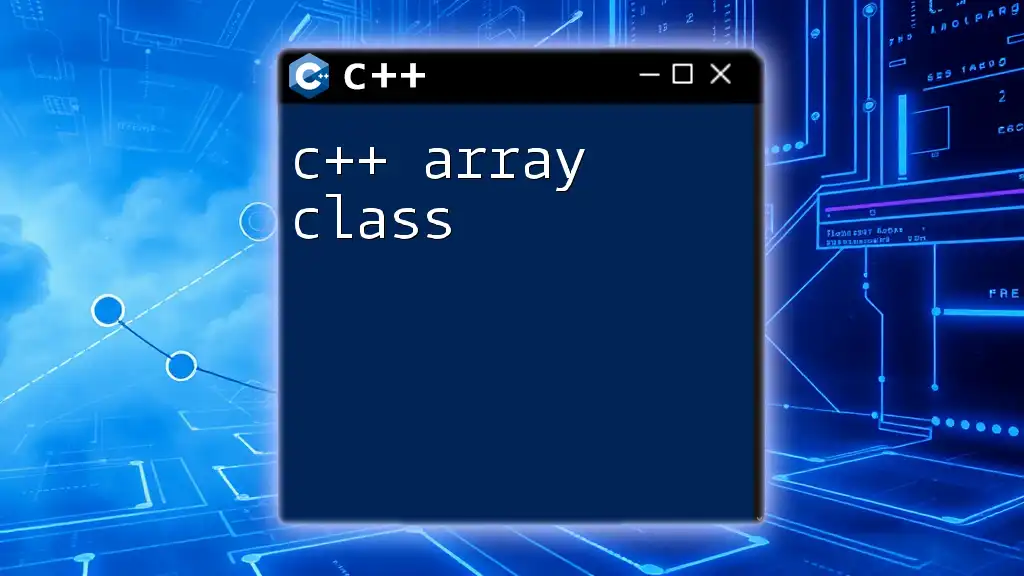
Common Operations on 2D Arrays
Traversing 2D Arrays
Looping through the elements of a C++ 2D array is typically done using nested loops. The outer loop iterates through the rows, while the inner loop goes through the columns. Here’s how it can be implemented:
for (int i = 0; i < rowCount; ++i) {
for (int j = 0; j < columnCount; ++j) {
std::cout << array[i][j] << " ";
}
}
This block of code efficiently prints all elements in the array, illustrating the ease of traversal in matrix-like structures.
Searching for an Element
You can implement a simple search algorithm, such as linear search, to find an element within a 2D array. For example:
bool found = false;
int target = 7;
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 4; ++j) {
if (array[i][j] == target) {
found = true;
break; // Breaks inner loop if the element is found
}
}
}
With this approach, you can efficiently locate a specific value, which is crucial for data processing applications.
Sorting a 2D Array
Sorting elements within a 2D array could involve sorting each row or column based on requirements. Here’s a simple implementation using `std::sort` for rows:
#include <algorithm>
// Function to sort each row of a 2D array
void sortRows(int array[][4], int rowCount) {
for (int i = 0; i < rowCount; ++i) {
std::sort(array[i], array[i] + 4); // Assumes 4 columns
}
}
This approach allows you to efficiently sort data, facilitating organized analysis or output.
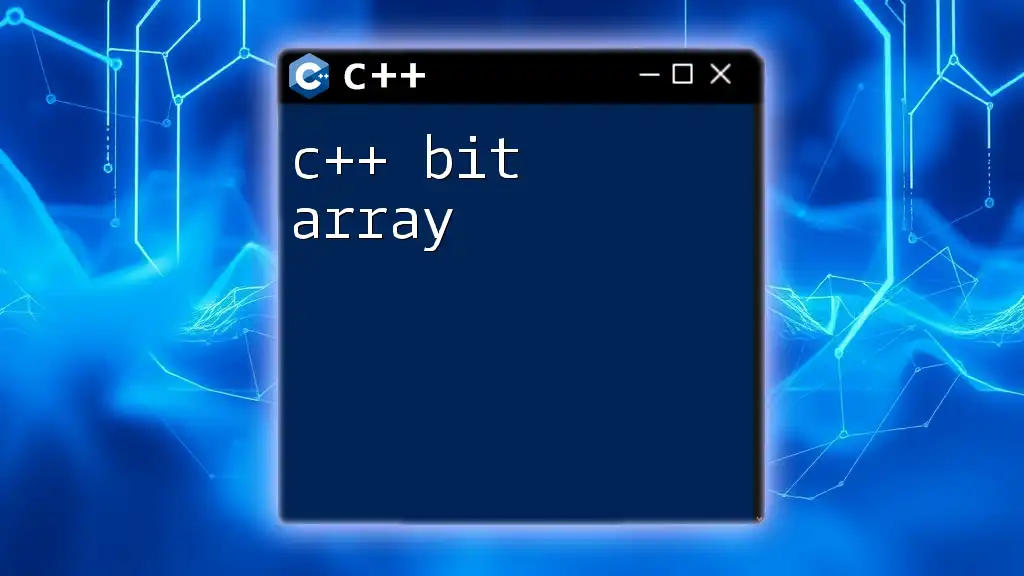
Advanced Concepts
Dynamic 2D Arrays
Dynamic 2D arrays offer flexibility in memory allocation, especially when the dimensions are not known at compile time. Creating a dynamic 2D array looks as follows:
int** array = new int*[rowCount];
for (int i = 0; i < rowCount; ++i) {
array[i] = new int[columnCount];
}
This method allows you to allocate the required memory on the heap, accommodating varying data sizes.
Memory Management
Proper memory management is vital when working with dynamic arrays to prevent memory leaks. After using a dynamically allocated 2D array, remember to free up the allocated memory:
for (int i = 0; i < rowCount; ++i) {
delete[] array[i]; // Release memory for each row
}
delete[] array; // Release memory for the array of pointers
Failing to deallocate memory could lead to increased consumption and possible program crashes.
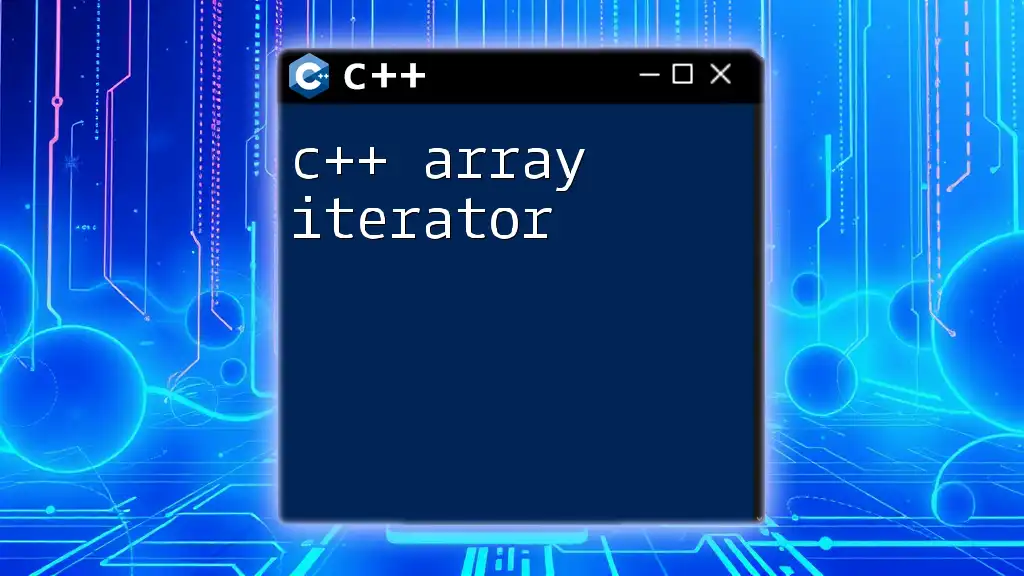
Conclusion
A comprehensive understanding of C++ 2D arrays equips you with the ability to handle complex data structures efficiently. Mastering their syntax, initialization, manipulation, and integration into functions adds to your overall programming proficiency. Implementing these concepts effectively will enable you to create sophisticated applications, whether you are dealing with graphics, mathematics, or data analysis.
Additional Resources
As you deepen your knowledge, consider exploring additional resources such as books, online tutorials, and coding challenges that focus on 2D arrays and their myriad uses in real-world programming. Your journey to mastering C++ will greatly benefit from continuous practice and exploration of related concepts.