In C++, you can create an array of class objects to manage multiple instances of a class efficiently, allowing you to access and manipulate them using standard array indexing.
class MyClass {
public:
int value;
MyClass(int v) : value(v) {}
};
int main() {
MyClass objArray[3] = { MyClass(1), MyClass(2), MyClass(3) };
// Access first object and print its value
std::cout << objArray[0].value; // Output: 1
return 0;
}
What is an Array in C++?
An array in C++ is a collection of items stored at contiguous memory locations. The primary purpose of an array is to store multiple items of the same type together, such as integers or characters, allowing for efficient access and manipulation of data.
Here’s the basic syntax for an array declaration:
data_type array_name[array_size];
For example, declaring an integer array of size 5 would look like:
int numbers[5];
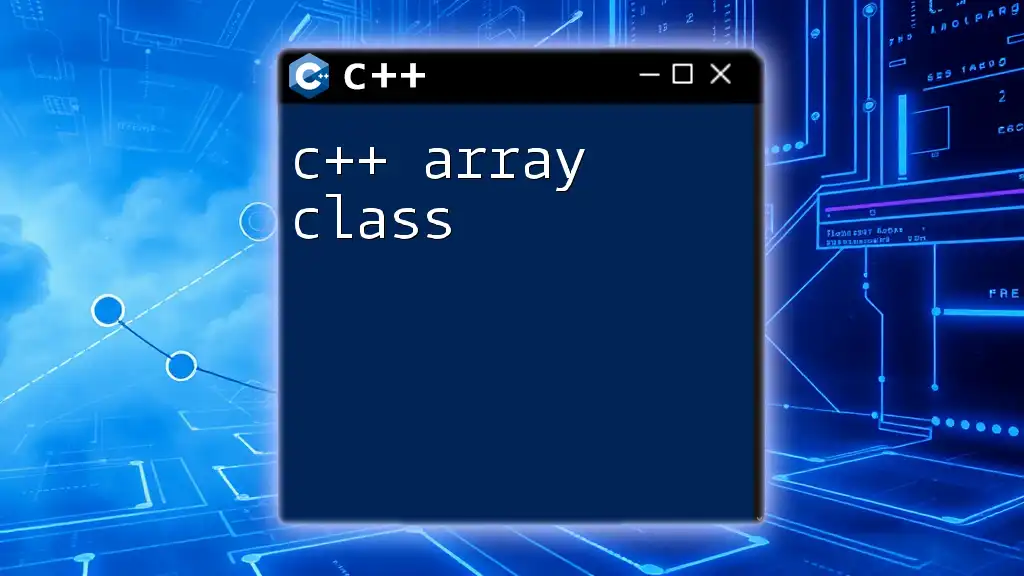
Understanding Classes in C++
A class in C++ acts as a blueprint for creating objects. Classes hold data in the form of attributes and define behavior through methods.
Key Components: Attributes and Methods
- Attributes: These are variables that hold the state of an object. For instance, a `Car` class might have attributes like `color`, `make`, and `model`.
- Methods: Functions defined within a class that describe the behaviors of the object. For example, a method `startEngine()` in a `Car` class would perform actions relevant to starting the car.
Example of a Simple Class
Here’s how you can define a simple `Car` class in C++:
class Car {
public:
std::string make;
std::string model;
int year;
// Constructor
Car(std::string m, std::string mod, int y) : make(m), model(mod), year(y) {}
};
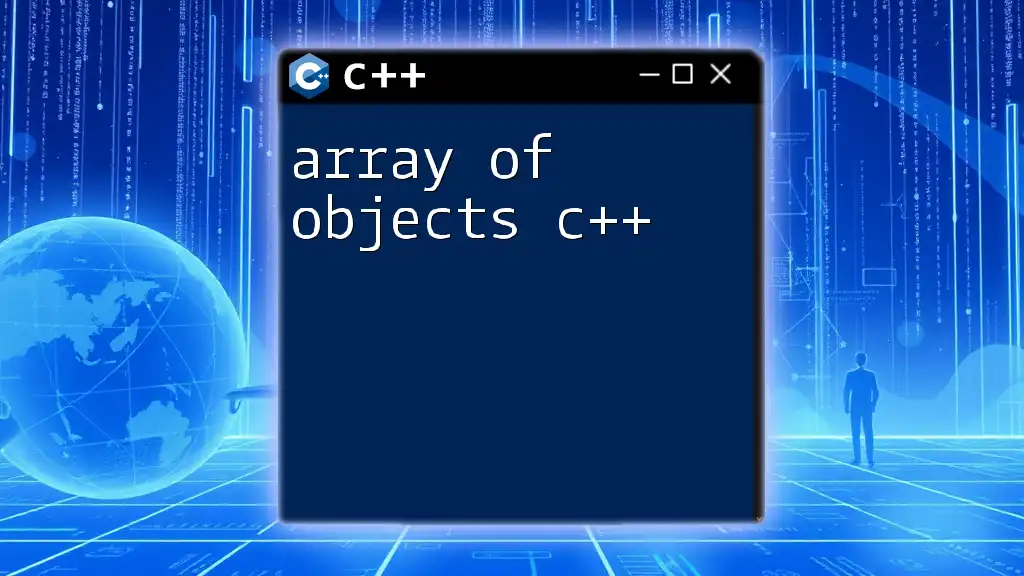
Creating a Class Object in C++
Defining a Class
Defining a class involves outlining its attributes and methods, along with access specifiers (public, private, protected). This encapsulation can help manage complexity.
Instantiating an Object from a Class
To create an object from a class, you use the class name followed by the object name and parentheses:
Car myCar("Toyota", "Camry", 2020);
Here, `myCar` is an instance of the `Car` class.
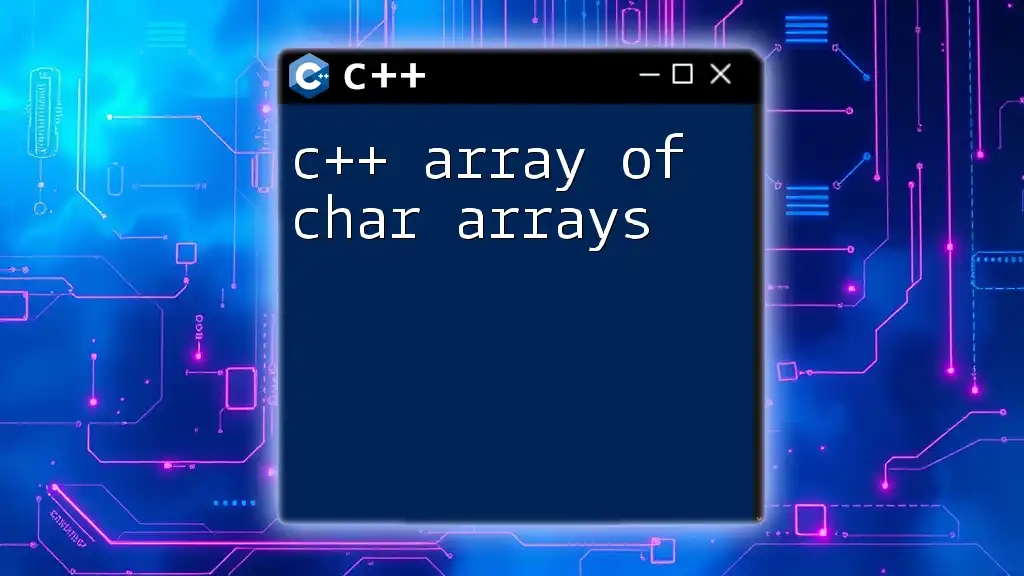
What is an Array of Class Objects?
An array of class objects holds multiple instances of a class in a single, contiguous memory block. This allows for batch processing and organized storage of similar entities.
Difference Between an Array of Primitive Types and an Array of Class Objects
The main difference lies in the data being stored. Primitive types are simple data types like `int`, `char`, etc., while an array of class objects contains the complete structure and behaviors defined in a class. For instance:
int numbers[5]; // Array of primitive types
Car cars[5]; // Array of class objects
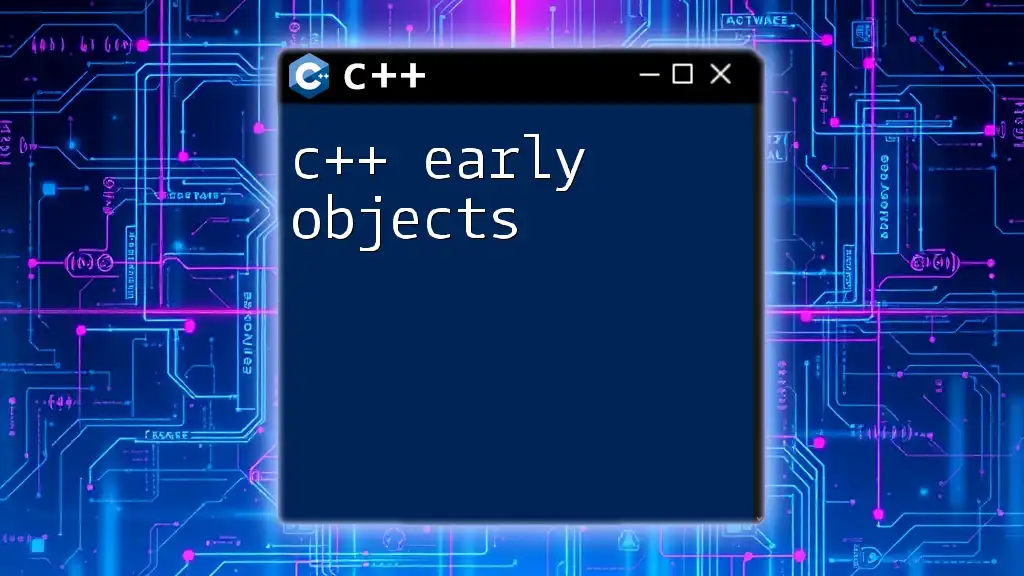
Declaring and Initializing an Array of Class Objects
Syntax for Declaring an Object Array
To declare an array of class objects, you simply mention the class name followed by the array name and the desired size:
Car cars[5];
Initializing an Array of Class Objects
You can initialize an array of class objects either statically or dynamically.
Static Initialization can be done at the point of declaration:
Car cars[3] = {
Car("Honda", "Accord", 2021),
Car("Ford", "Mustang", 2022),
Car("Tesla", "Model 3", 2023)
};
Dynamic Initialization uses `new` and pointers:
Car* cars = new Car[3]{
Car("Honda", "Accord", 2021),
Car("Ford", "Mustang", 2022),
Car("Tesla", "Model 3", 2023)
};
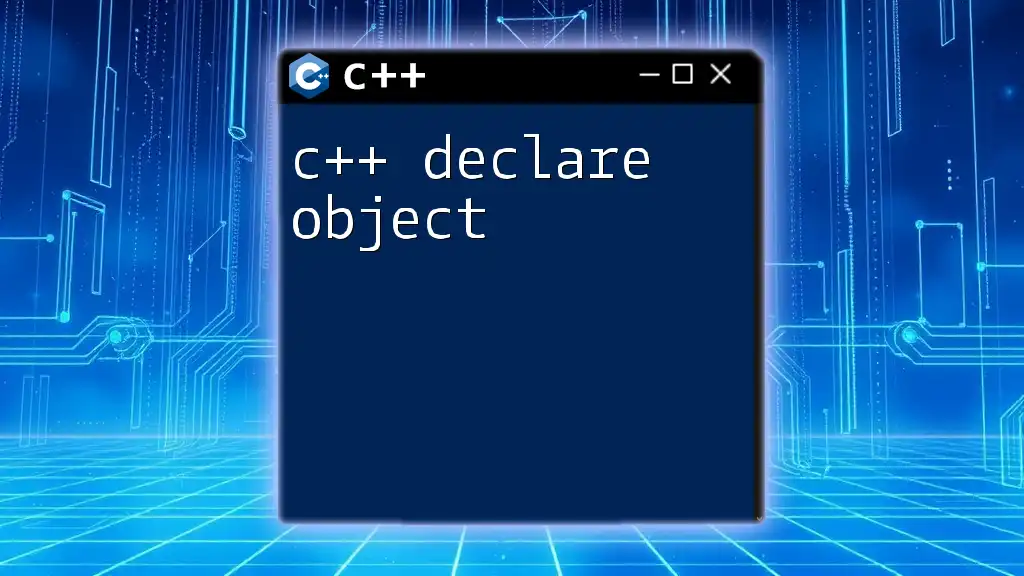
Accessing Class Objects in an Array
Using Indexes to Access Objects
Accessing elements in an array of class objects is performed using indices, much like primitive arrays. For example:
std::cout << cars[0].make; // Outputs "Honda"
Iterating Over an Array of Class Objects
You can iterate over an array using standard `for` loops or even range-based loops introduced in C++11:
for (int i = 0; i < 3; i++) {
std::cout << cars[i].make << std::endl; // Outputs the make of each car
}
// Using range-based for loop in C++11
for (const auto& car : cars) {
std::cout << car.make << std::endl;
}
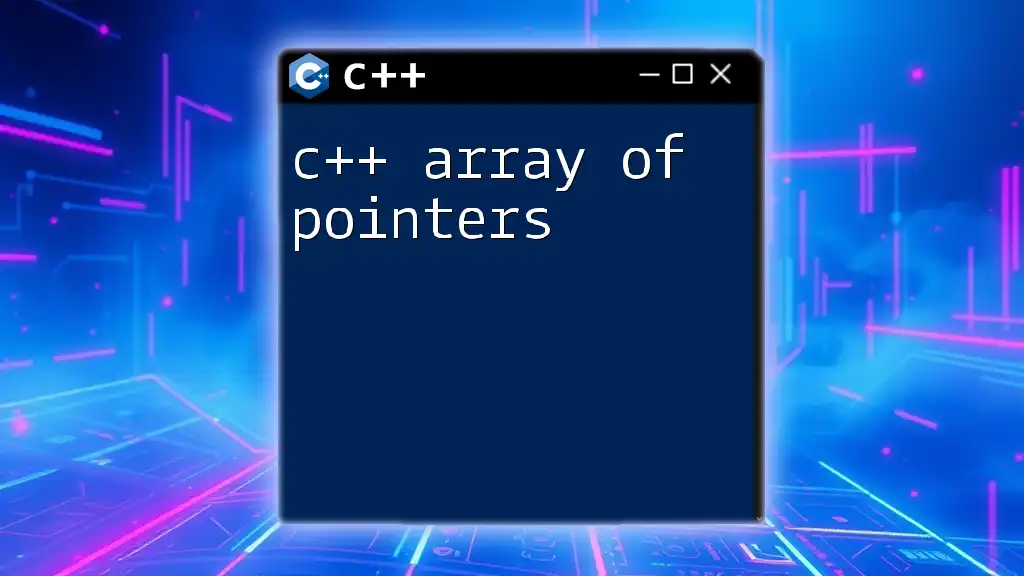
Manipulating Class Objects Within an Array
Modifying Object Properties
You can change the attributes of objects within the array using simple assignment:
cars[0].year = 2022; // Updates the year of the first car
Calling Object Methods from an Array
To invoke methods on objects in an array, simply use the dot operator:
cars[1].startEngine(); // Calls the method for the second car
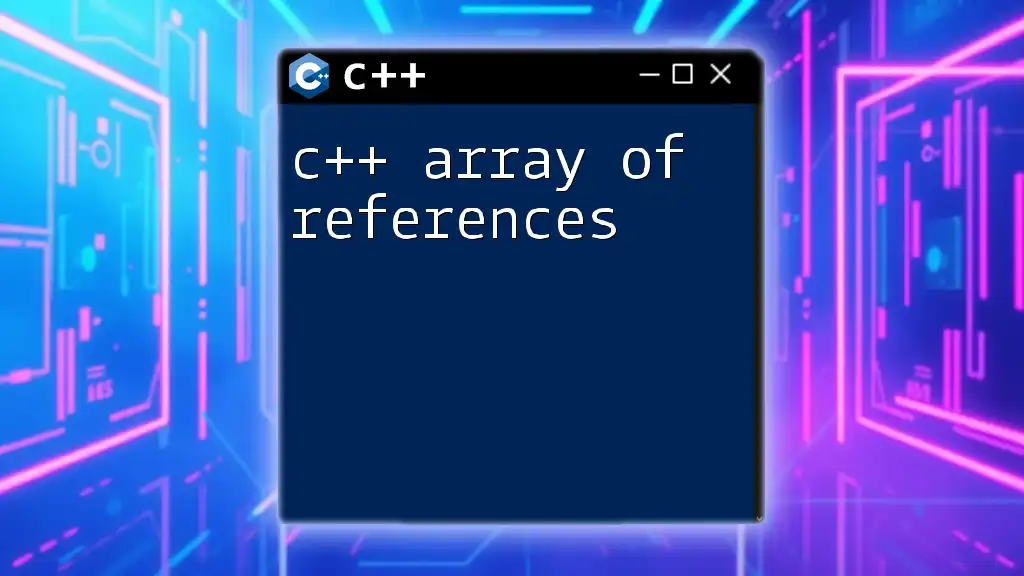
Practical Example: C++ Class Array
Creating a Simple Class for Example
Let’s create a `Book` class for our practical example:
class Book {
public:
std::string title;
std::string author;
int year;
Book(std::string t, std::string a, int y) : title(t), author(a), year(y) {}
};
Implementing an Array of Book Objects
Now, let’s initialize an array of `Book` objects:
Book books[3] = {
Book("1984", "George Orwell", 1949),
Book("The Great Gatsby", "F. Scott Fitzgerald", 1925),
Book("To Kill a Mockingbird", "Harper Lee", 1960)
};
You can print the details of each book using a loop:
for (const auto& book : books) {
std::cout << book.title << ", by " << book.author << ", published in " << book.year << std::endl;
}
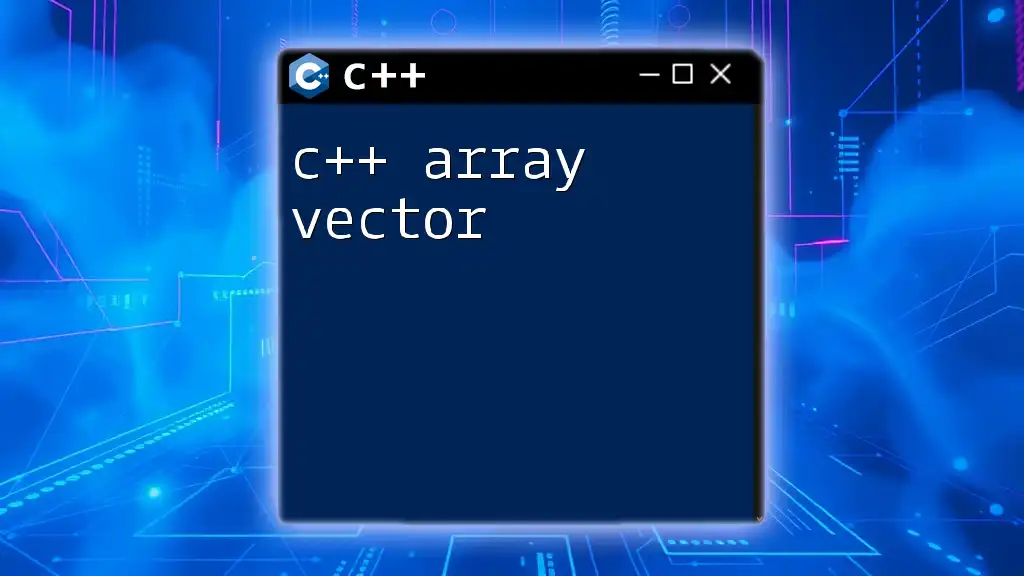
Common Pitfalls and Best Practices
Mistakes to Avoid When Using C++ Object Arrays
One common mistake is neglecting the proper use of constructors. If no constructors are defined, the objects may not be initialized correctly, leading to undefined behavior.
Another pitfall is not managing memory properly, especially when using dynamic arrays. Always ensure that you delete any dynamically allocated memory:
delete[] cars; // If using dynamic array for Car objects
Best Practices with C++ Arrays of Class Objects
- Efficient Memory Use: Prefer using standard containers, like `std::vector`, over raw arrays for better memory management.
- Encapsulation: Use private members and public methods for better access control.
- Using STL: The Standard Template Library (STL) provides containers like `std::vector` and `std::array`, which can simplify array management and provide additional functionality.
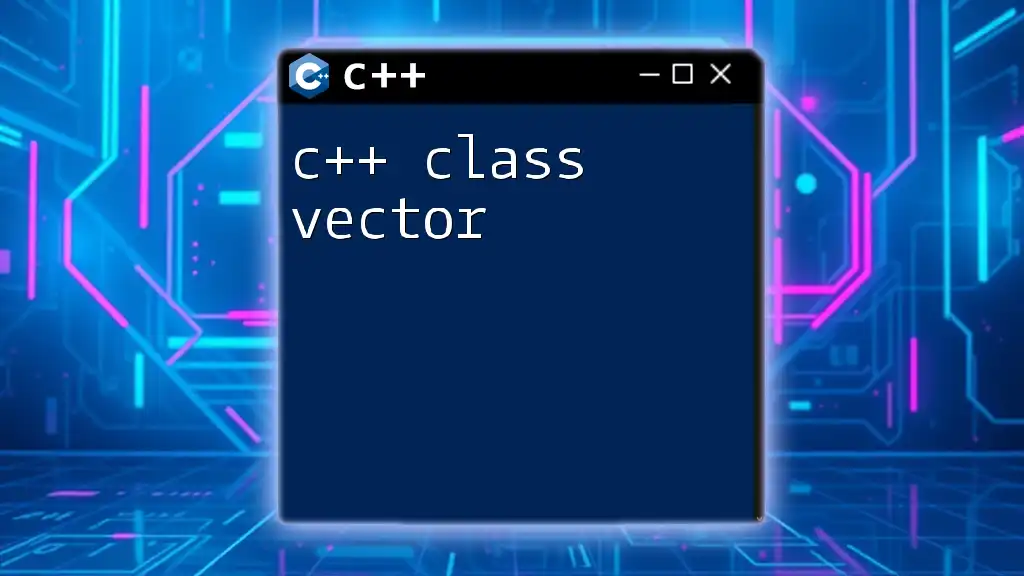
Conclusion
Understanding and using a C++ array of class objects can significantly enhance your programming abilities, enabling organized management of multiple instances of related data. The efficient manipulation and access of class objects facilitate cleaner, more maintainable code. Whether you are developing small applications or large-scale software, a firm grasp of this concept will elevate your programming skills and lead to more robust designs.
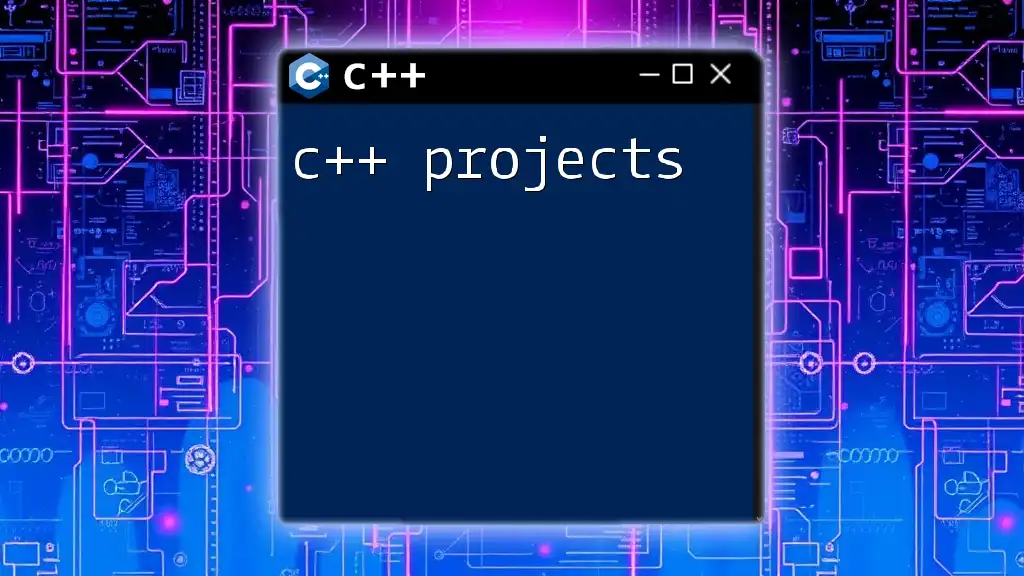
Additional Resources
For further learning, consider exploring recommended books, online tutorials, and forums that delve deeper into C++ programming, class management, and memory management techniques.