C++ array methods provide a set of functions to manipulate, access, and perform operations on arrays efficiently.
Here’s a simple example demonstrating how to calculate the sum of elements in an array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int sum = 0;
for (int i = 0; i < 5; i++) {
sum += arr[i];
}
cout << "Sum: " << sum << endl;
return 0;
}
Understanding C++ Array Functions
Arrays in C++ are fundamental data structures that allow you to store a collection of elements of the same type. An array is particularly useful when you need to manage collections of data, such as a list of numbers or a set of objects. The main reason for utilizing C++ array methods is to optimize performance by efficiently interacting with these collections, allowing you to process data in a systematic way.
What are Array Functions?
Array functions are specific functions or operations performed on arrays to manipulate, access, or modify their contents. In C++, you can define your own user-defined functions or utilize built-in functions that handle arrays directly. Understanding how to use these functions effectively can significantly improve your coding efficiency.
Types of Array Functions in C++
C++ provides a range of functions that help to manage arrays. These include built-in functions offered by the Standard Library, as well as the ability to create custom functions tailored to specific needs.
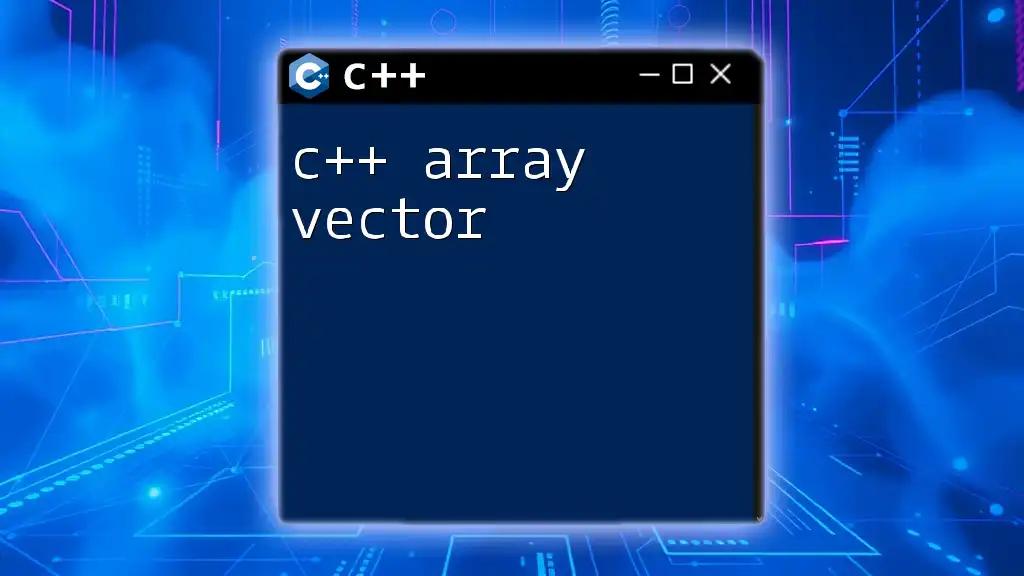
Creating and Initializing C++ Arrays
Basic Array Declaration
To work with arrays, the first step is to declare them. The syntax for declaring an array in C++ is simple:
int myArray[5]; // Declaration of an integer array
In this example, `myArray` will hold five integers. Remember, array sizes must be fixed at compile time.
Array Initialization
When declaring an array, you can also initialize its values immediately.
Static Initialization
You can initialize an array statically by providing its values directly:
int myArray[5] = {1, 2, 3, 4, 5};
This sets the elements of `myArray` to the specified integers upon creation.
Dynamic Initialization
For cases where array sizes are not known at compile time, you can use dynamic initialization with the `new` keyword:
int* myArray = new int[5]{1, 2, 3, 4, 5};
With this method, memory is allocated at runtime, allowing for more flexible programs.
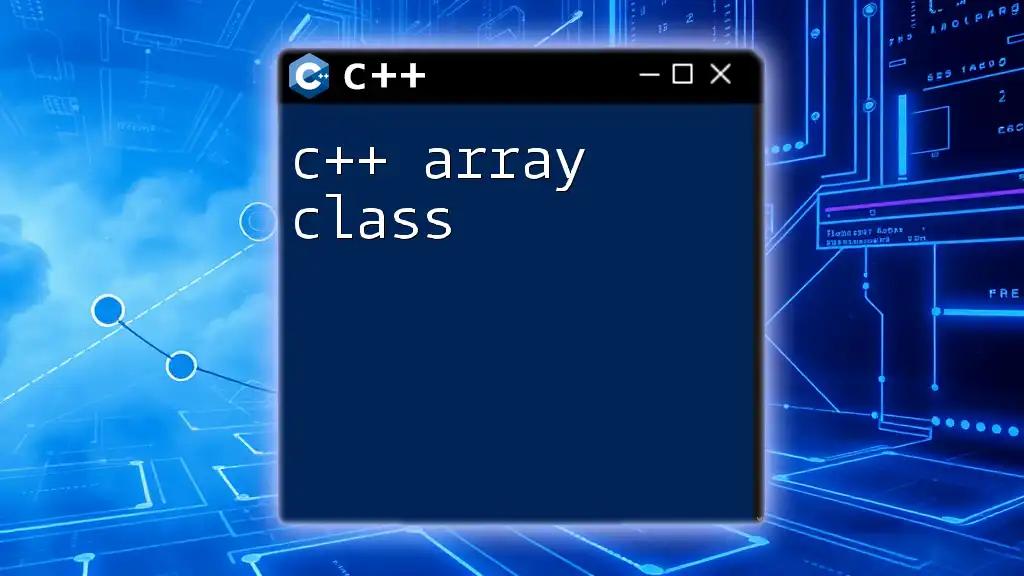
Accessing and Modifying C++ Array Elements
Indexing Basics
Accessing elements of an array is as straightforward as indexing into the array using square brackets. For example:
int value = myArray[2]; // Accesses the third element
In C++, indices start at 0, meaning that `myArray[2]` refers to the third element.
Modifying Array Elements
You can also modify values stored in an array by simply assigning a new value to a specific index:
myArray[1] = 10; // Changes the second element value from 2 to 10
This flexibility allows you to manage your data dynamically throughout the lifecycle of your program.
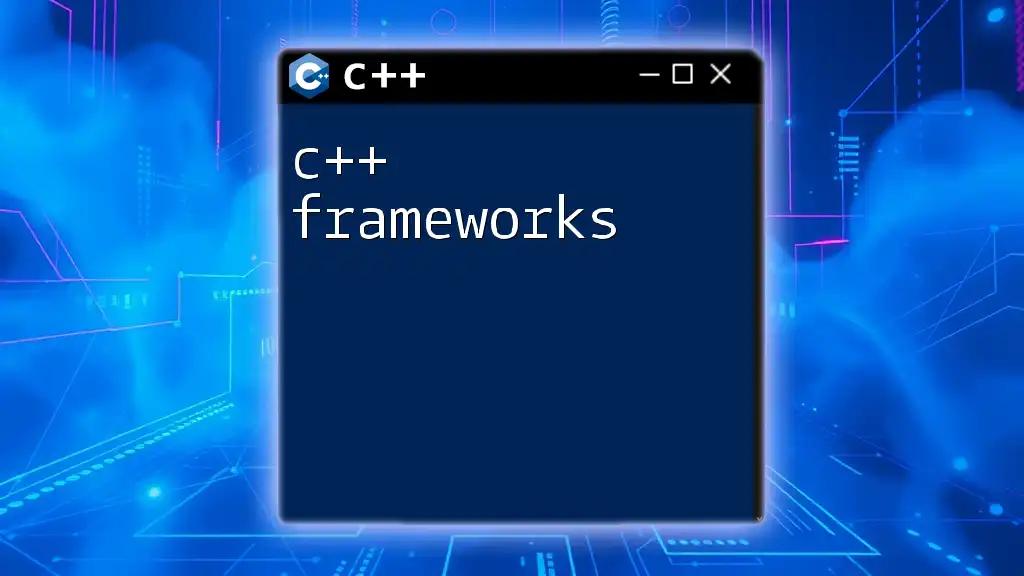
Common C++ Array Methods
Using Loop Structures with Arrays
Loops are essential when interacting with arrays, as they allow you to process many elements efficiently.
For Loops
A common way to iterate through an array is by using a for loop:
for(int i = 0; i < 5; i++) {
std::cout << myArray[i] << " ";
}
Here, we print each element in `myArray`. This method is predictable and straightforward.
While Loops
Alternatively, you can use a while loop to access array elements:
int i = 0;
while(i < 5) {
std::cout << myArray[i] << " ";
i++;
}
While loops can be beneficial when the termination condition is not necessarily based on index increments.
C++ Standard Library Functions for Arrays
C++ provides several useful functions in the Standard Library to perform operations on arrays, enhancing performance and usability.
Using `std::sort()` to Sort Arrays
Sorting is a fundamental task that can easily be accomplished with the Standard Library:
#include <algorithm>
std::sort(myArray, myArray + 5);
This function sorts the array in ascending order, showing C++'s built-in functionalities to simplify your work.
Finding Array Size with `sizeof`
Knowing the size of your array is crucial for many operations. You can determine the size of a statically allocated array using:
int size = sizeof(myArray) / sizeof(myArray[0]); // Size of the array
This simple formula calculates the number of elements by dividing the total byte size of the array by the size of a single element.
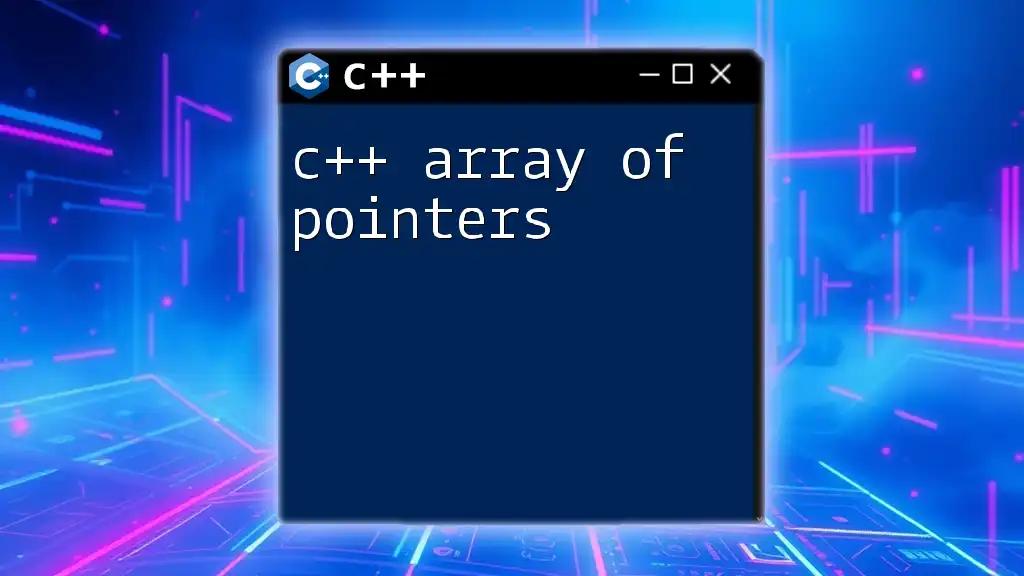
Advanced C++ Array Techniques
Multi-Dimensional Arrays
For complex data structures, multi-dimensional arrays can be valuable. They are declared similarly to single-dimensional arrays but with additional brackets:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
This creates a 3x3 matrix, useful for a variety of applications like graphics and scientific computing.
Accessing Elements in Multi-Dimensional Arrays
Accessing elements in a multi-dimensional array is done using two indices:
int value = matrix[1][2]; // Accesses the element at 2nd row, 3rd column
Understanding how to manipulate multi-dimensional arrays expands the potential for more intricate data handling.
C++ Array of Objects
You can also create arrays of class objects, allowing for powerful object-oriented programming practices:
class MyClass {
public:
int x;
};
MyClass objArray[10];
This code snippet creates an array capable of holding ten `MyClass` objects, demonstrating flexibility when managing collections of custom data types.
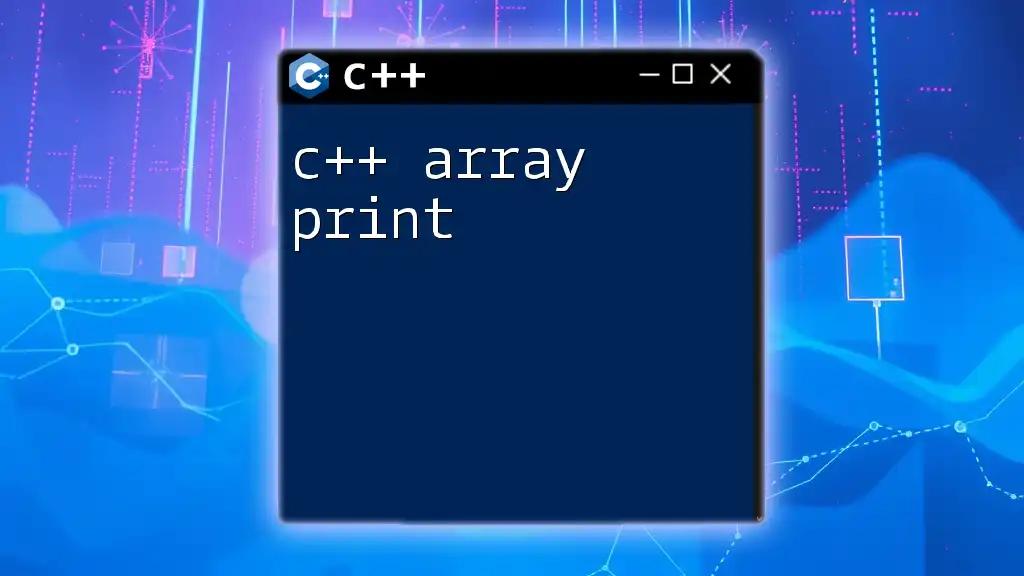
Conclusion
Understanding and utilizing C++ array methods is essential for any programmer looking to enhance their skills. Arrays provide a structured way to store and manipulate data, and knowing the various functions that work with them can streamline your programming workflow. Practice these techniques to become proficient in handling arrays in your future C++ projects.
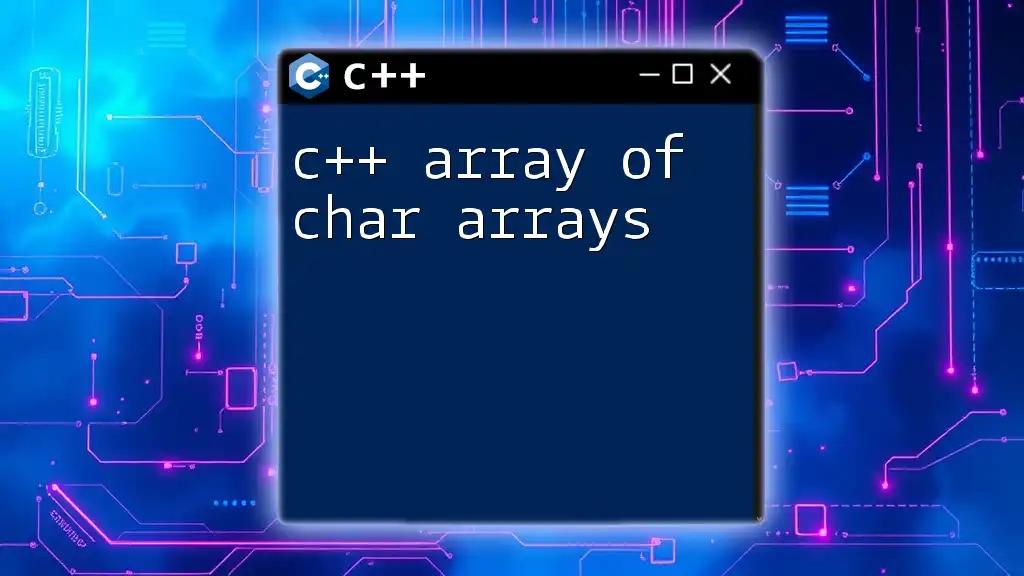
Additional Resources
For those interested in deepening their understanding of C++ arrays and their methods, consider exploring the official C++ documentation and joining online forums or study groups. Books focused on C++ programming principles can provide further context and advanced techniques to master arrays and data management.