A C++ neural network is an implementation that allows the creation and training of artificial neural networks using the C++ programming language to perform tasks like classification and regression.
Here's a simple C++ code snippet for a basic feedforward neural network structure:
#include <iostream>
#include <vector>
#include <cmath>
class NeuralNetwork {
public:
NeuralNetwork(int input_nodes, int hidden_nodes, int output_nodes);
std::vector<double> feedforward(const std::vector<double>& input);
private:
std::vector<double> sigmoid(const std::vector<double>& x);
int input_nodes, hidden_nodes, output_nodes;
std::vector<std::vector<double>> weights_input_hidden;
std::vector<std::vector<double>> weights_hidden_output;
};
// Constructor and implementation details would follow...
Understanding the Basics of Neural Networks in C++
What is a Neural Network?
A neural network is a computational model inspired by the way biological neural networks in the human brain process information. It comprises interconnected groups of artificial neurons that work together to solve specific problems, including classification, regression, and even reinforcement learning. Unlike traditional algorithms which rely on explicit instructions, neural networks learn from data and improve their performance over time.
Components of a Neural Network
Neurons: The fundamental building blocks of neural networks, similar to biological neurons, each neuron receives input data, processes it, and produces an output.
Layers: Neural networks consist of three primary types of layers:
- Input Layer: This is where data enters the network.
- Hidden Layers: These layers perform the bulk of the computations and can vary in number depending on your architecture.
- Output Layer: This layer delivers the final result.
Weights and Biases: Each connection between neurons has an associated weight, determining the significance of the input. Biases help fine-tune the output along with weights. Adjusting weights and biases during training enables the network to learn complex patterns.
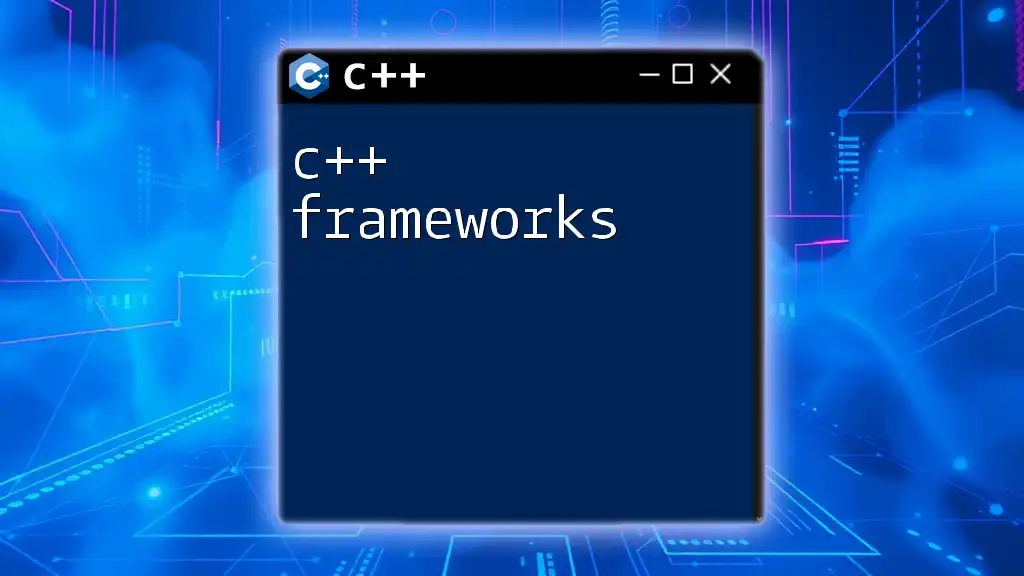
Setting Up Your C++ Environment for Neural Networks
Required Software and Libraries
To develop a C++ neural network, you will need suitable libraries and tools. Some popular libraries include:
- TensorFlow C++ API: Allows you to leverage the prominent TensorFlow machine learning library with C++.
- Armadillo: A high-quality linear algebra library for C++ that offers efficient data handling.
- FANN (Fast Artificial Neural Network Library): Designed specifically for creating neural networks.
To get started, follow installation instructions on the respective documentation pages for each library.
Getting Started with the Basics of C++
Before jumping into neural networks, securing a strong foundation in C++ is essential. Here are some must-know C++ concepts:
- Basic Syntax: Familiarize yourself with the syntax for variables, functions, loops, and conditions.
- Object-Oriented Programming (OOP): Understand key concepts such as classes, objects, inheritance, and polymorphism. OOP principles are fundamental when structuring a neural network.
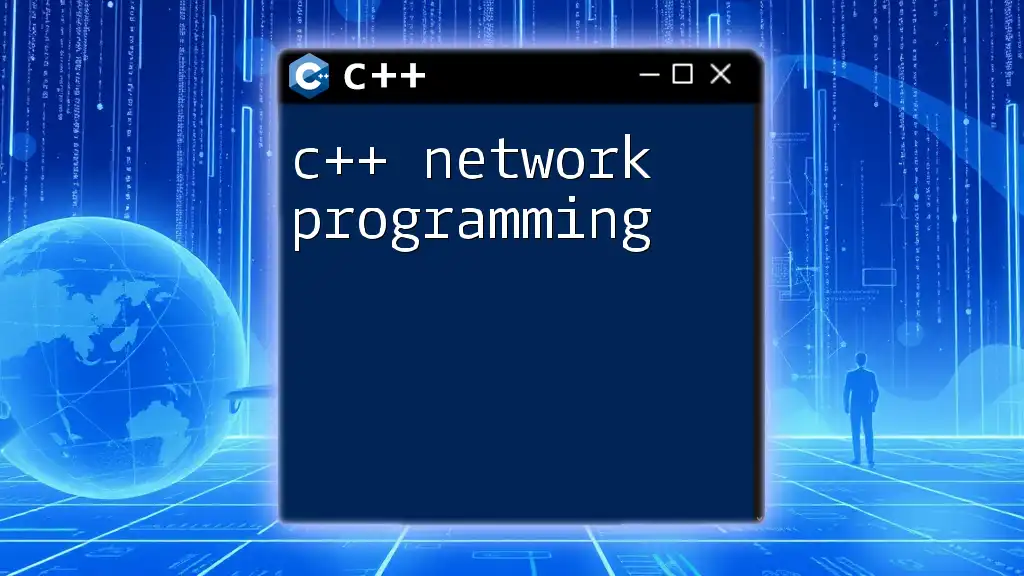
Building Your First Neural Network in C++
Structure of a Neural Network in C++
To create a neural network in C++, you can define a class that encapsulates user-defined parameters such as input nodes, hidden nodes, and output nodes. Here's a simple structure for a neural network class:
class NeuralNetwork {
public:
NeuralNetwork(int inputNodes, int hiddenNodes, int outputNodes);
void train(const std::vector<std::vector<double>>& trainingData);
std::vector<double> predict(const std::vector<double>& input);
private:
int inputNodes, hiddenNodes, outputNodes;
// Add necessary data structures to hold weights, biases, etc.
};
This class serves as a blueprint for creating multiple neural networks with varying configurations.
Forward Propagation
Forward propagation is the process by which input data is passed through the network to generate output. Each neuron computes its output based on the weighted sum of its inputs and applies an activation function.
Here’s a snippet demonstrating forward propagation in C++:
std::vector<double> NeuralNetwork::predict(const std::vector<double>& input) {
std::vector<double> hiddenOutput(hiddenNodes);
// Compute the output for hidden layers
for(int i = 0; i < hiddenNodes; ++i) {
double sum = 0.0;
for(int j = 0; j < inputNodes; ++j) {
sum += input[j] * weightsInputHidden[j][i]; // weightsInputHidden as a 2D vector
}
hiddenOutput[i] = activationFunction(sum + biasesHidden[i]); // activationFunction is a method to apply the activation
}
// Compute the output for the output layer
return output;
}
Backward Propagation
Backward propagation involves updating weights based on the error in prediction. It works by calculating gradients and making adjustments to minimize the loss function.
Here’s an overview of how you might implement backward propagation:
void NeuralNetwork::train(const std::vector<std::vector<double>>& trainingData) {
for (const auto& data : trainingData) {
std::vector<double> input = data; // Assume data is structured correctly
// Forward propagate the input
std::vector<double> output = predict(input);
// Calculate errors and gradients for each layer
// Update weights based on gradients
}
}
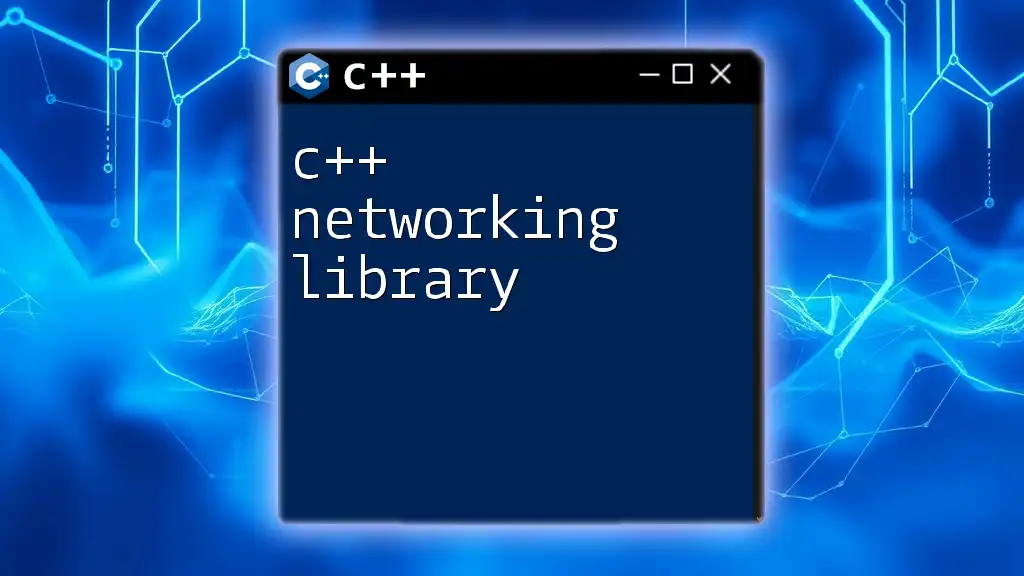
Training the Neural Network
Data Preparation and Preprocessing
Preparing your dataset is crucial. Normalize your data to ensure consistent scales across features. This leads to better, faster training. Split your data into training, validation, and test sets to evaluate your network properly.
Loss Function and Optimization
The loss function measures how well your neural network performs by comparing the predicted outputs against the true outputs. The Mean Squared Error (MSE) is a common choice for regression problems. The optimization algorithms, such as Gradient Descent, adjust weights and biases to minimize the loss function iteratively.
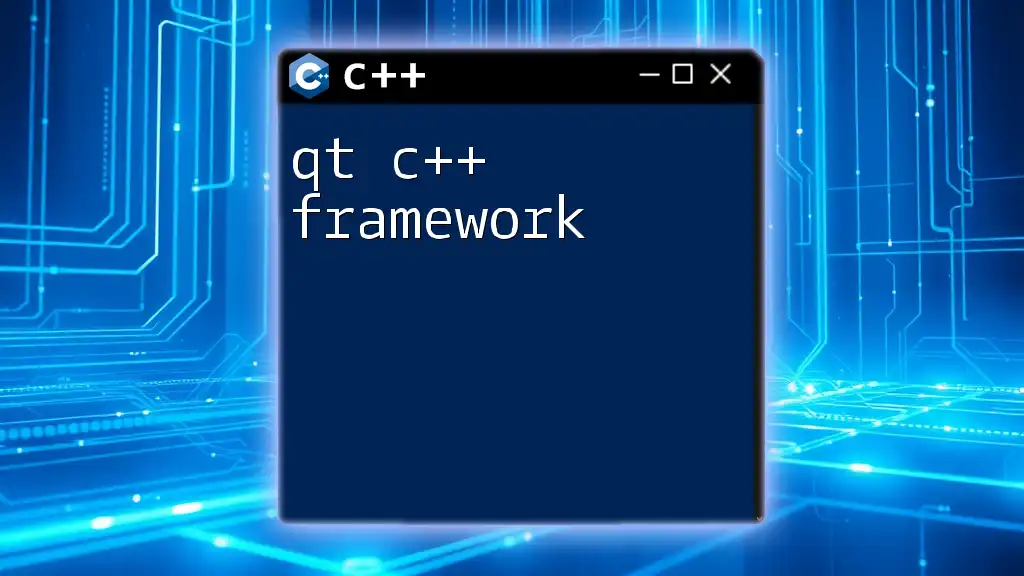
Enhancements and Advanced Techniques
Regularization Techniques
Regularization methods help prevent overfitting, which occurs when a model learns the training data too well, harming its performance on new data. Common techniques include L2 regularization, which adds a penalty based on the magnitude of weights.
Implementing L2 regularization in your neural network might look something like this:
double NeuralNetwork::computeLoss(const std::vector<double>& predictions, const std::vector<double>& targets) {
double loss = meanSquaredError(predictions, targets);
// Add L2 penalty
for (const auto& weight : weightsInputHidden) {
loss += lambda * weight * weight; // where lambda is a constant for regularization influence
}
return loss;
}
Convolutional and Recurrent Neural Networks
For tasks such as image classification or time-series forecasting, consider using Convolutional Neural Networks (CNNs) or Recurrent Neural Networks (RNNs).
CNNs are effective in image processing due to their ability to capture spatial hierarchies, while RNNs excel in sequence prediction tasks, making them suitable for natural language processing. Each type requires a different architecture and careful tuning based on your application.
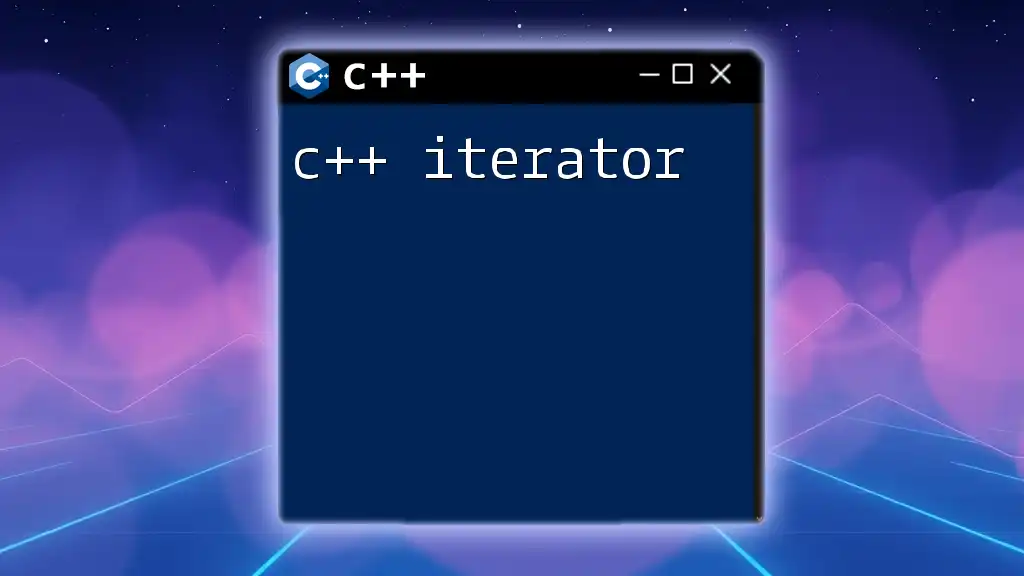
Real-World Applications of C++ Neural Networks
Industry Use Cases
C++ neural networks find applications across various sectors, including healthcare for disease prediction, finance for risk assessment, and automotive for autonomous systems. The performance efficiency of C++ makes it a popular choice in resource-constrained environments.
C++ Neural Network Libraries
Several libraries facilitate the development of C++ neural networks:
- FANN: Great for beginners with its straightforward API.
- dlib: Offers machine learning algorithms and tools, including neural networks.
- TensorFlow C++ API: Provides access to TensorFlow's robust functionalities in a C++ environment.
When choosing a library, consider the complexity of your project, ease of use, and community support.
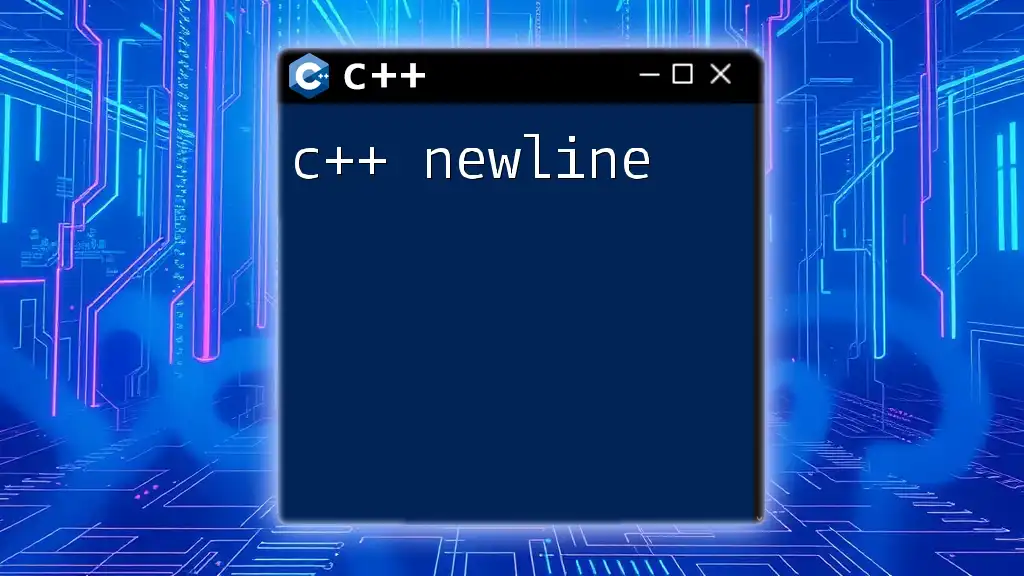
Conclusion
This guide has walked you through the essential concepts of C++ neural networks, from the foundational components to practical implementations. Using object-oriented principles allows for easier expansion and maintenance of your code, while leveraging C++'s performance makes it suitable for numerous applications.
For those eager to dive deeper into building neural networks, take the first step by experimenting with the code snippets provided and creating your own neural network projects. Embrace the learning process, and you will be designing efficient models in no time!
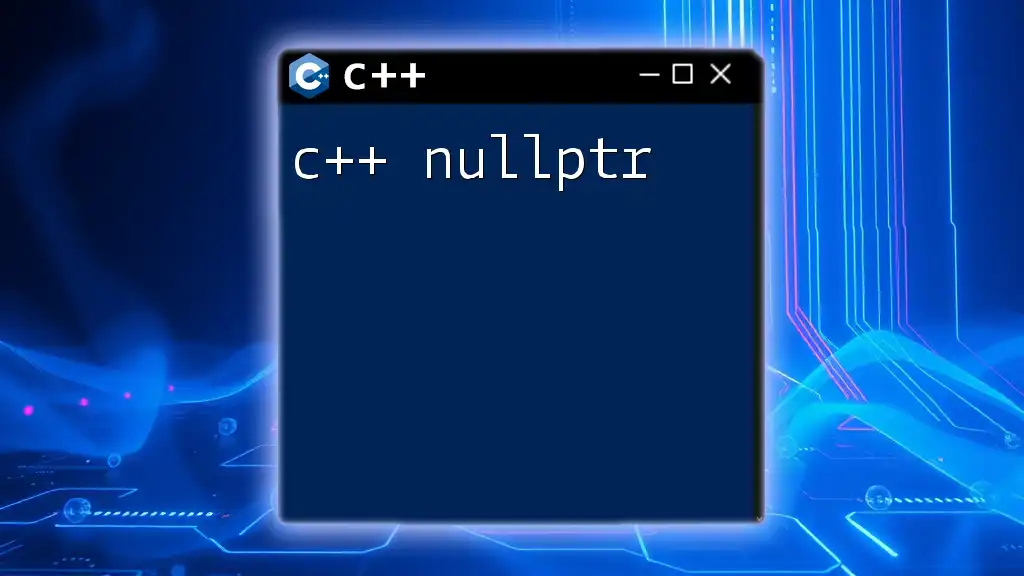
Resources and Further Reading
For those looking to expand their knowledge, consider checking out specialized books on neural networks, online courses that cover advanced topics, and reputable tutorials on C++ machine learning libraries. Engaging in community forums will also provide you with ongoing support and insights from other developers in the field.